Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / XsltOld / ElementAction.cs / 1 / ElementAction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ElementAction : ContainerAction { private const int NameDone = 2; private Avt nameAvt; private Avt nsAvt; private bool empty; private InputScopeManager manager; // Compile time precalculated AVTs private string name; private string nsUri; private PrefixQName qname; // When we not have AVTs at all we can do this. null otherwise. internal ElementAction() {} private static PrefixQName CreateElementQName(string name, string nsUri, InputScopeManager manager) { if (nsUri == Keywords.s_XmlnsNamespace) { throw XsltException.Create(Res.Xslt_ReservedNS, nsUri); } PrefixQName qname = new PrefixQName(); qname.SetQName(name); if (nsUri == null) { qname.Namespace = manager.ResolveXmlNamespace(qname.Prefix); } else { qname.Namespace = nsUri; } return qname; } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); this.name = PrecalculateAvt(ref this.nameAvt); this.nsUri = PrecalculateAvt(ref this.nsAvt ); // if both name and ns are not AVT we can calculate qname at compile time and will not need namespace manager anymore if (this.nameAvt == null && this.nsAvt == null) { if(this.name != Keywords.s_Xmlns) { this.qname = CreateElementQName(this.name, this.nsUri, compiler.CloneScopeManager()); } } else { this.manager = compiler.CloneScopeManager(); } if (compiler.Recurse()) { Debug.Assert(this.empty == false); CompileTemplate(compiler); compiler.ToParent(); } this.empty = (this.containedActions == null) ; } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.Namespace)) { this.nsAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.UseAttributeSets)) { AddAction(compiler.CreateUseAttributeSetsAction()); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.qname != null) { frame.CalulatedName = this.qname; } else { frame.CalulatedName = CreateElementQName( this.nameAvt == null ? this.name : this.nameAvt.Evaluate(processor, frame), this.nsAvt == null ? this.nsUri : this.nsAvt .Evaluate(processor, frame), this.manager ); } goto case NameDone; case NameDone: { PrefixQName qname = frame.CalulatedName; if (processor.BeginEvent(XPathNodeType.Element, qname.Prefix, qname.Name, qname.Namespace, this.empty) == false) { // Come back later frame.State = NameDone; break; } if (! this.empty) { processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run } else { goto case ProcessingChildren; } } case ProcessingChildren: if (processor.EndEvent(XPathNodeType.Element) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Xsl.XsltOld { using Res = System.Xml.Utils.Res; using System; using System.Diagnostics; using System.Xml; using System.Xml.XPath; internal class ElementAction : ContainerAction { private const int NameDone = 2; private Avt nameAvt; private Avt nsAvt; private bool empty; private InputScopeManager manager; // Compile time precalculated AVTs private string name; private string nsUri; private PrefixQName qname; // When we not have AVTs at all we can do this. null otherwise. internal ElementAction() {} private static PrefixQName CreateElementQName(string name, string nsUri, InputScopeManager manager) { if (nsUri == Keywords.s_XmlnsNamespace) { throw XsltException.Create(Res.Xslt_ReservedNS, nsUri); } PrefixQName qname = new PrefixQName(); qname.SetQName(name); if (nsUri == null) { qname.Namespace = manager.ResolveXmlNamespace(qname.Prefix); } else { qname.Namespace = nsUri; } return qname; } internal override void Compile(Compiler compiler) { CompileAttributes(compiler); CheckRequiredAttribute(compiler, this.nameAvt, Keywords.s_Name); this.name = PrecalculateAvt(ref this.nameAvt); this.nsUri = PrecalculateAvt(ref this.nsAvt ); // if both name and ns are not AVT we can calculate qname at compile time and will not need namespace manager anymore if (this.nameAvt == null && this.nsAvt == null) { if(this.name != Keywords.s_Xmlns) { this.qname = CreateElementQName(this.name, this.nsUri, compiler.CloneScopeManager()); } } else { this.manager = compiler.CloneScopeManager(); } if (compiler.Recurse()) { Debug.Assert(this.empty == false); CompileTemplate(compiler); compiler.ToParent(); } this.empty = (this.containedActions == null) ; } internal override bool CompileAttribute(Compiler compiler) { string name = compiler.Input.LocalName; string value = compiler.Input.Value; if (Keywords.Equals(name, compiler.Atoms.Name)) { this.nameAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.Namespace)) { this.nsAvt = Avt.CompileAvt(compiler, value); } else if (Keywords.Equals(name, compiler.Atoms.UseAttributeSets)) { AddAction(compiler.CreateUseAttributeSetsAction()); } else { return false; } return true; } internal override void Execute(Processor processor, ActionFrame frame) { Debug.Assert(processor != null && frame != null); switch (frame.State) { case Initialized: if(this.qname != null) { frame.CalulatedName = this.qname; } else { frame.CalulatedName = CreateElementQName( this.nameAvt == null ? this.name : this.nameAvt.Evaluate(processor, frame), this.nsAvt == null ? this.nsUri : this.nsAvt .Evaluate(processor, frame), this.manager ); } goto case NameDone; case NameDone: { PrefixQName qname = frame.CalulatedName; if (processor.BeginEvent(XPathNodeType.Element, qname.Prefix, qname.Name, qname.Namespace, this.empty) == false) { // Come back later frame.State = NameDone; break; } if (! this.empty) { processor.PushActionFrame(frame); frame.State = ProcessingChildren; break; // Allow children to run } else { goto case ProcessingChildren; } } case ProcessingChildren: if (processor.EndEvent(XPathNodeType.Element) == false) { frame.State = ProcessingChildren; break; } frame.Finished(); break; default: Debug.Fail("Invalid ElementAction execution state"); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
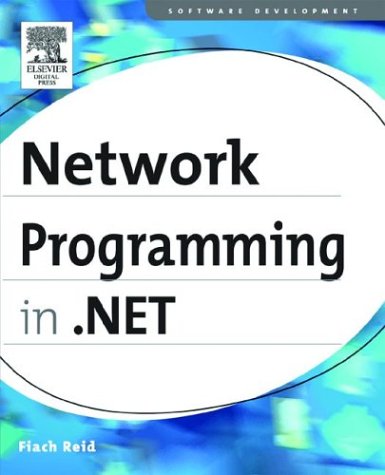
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SuppressIldasmAttribute.cs
- ReadOnlyKeyedCollection.cs
- InfoCardPolicy.cs
- HwndHostAutomationPeer.cs
- ValidationManager.cs
- VoiceSynthesis.cs
- RegionData.cs
- RowVisual.cs
- Certificate.cs
- BlurEffect.cs
- StreamInfo.cs
- AssemblyResourceLoader.cs
- RemotingHelper.cs
- ImportOptions.cs
- TcpConnectionPoolSettings.cs
- DisplayInformation.cs
- ParallelTimeline.cs
- PathStreamGeometryContext.cs
- StyleXamlTreeBuilder.cs
- TimeBoundedCache.cs
- StylusPoint.cs
- SourceLocation.cs
- OleStrCAMarshaler.cs
- RegularExpressionValidator.cs
- XmlSchemaInfo.cs
- BaseWebProxyFinder.cs
- RulePatternOps.cs
- CreateUserWizardStep.cs
- ImageSource.cs
- BinaryObjectReader.cs
- Interlocked.cs
- XmlKeywords.cs
- SizeAnimationUsingKeyFrames.cs
- Trace.cs
- ExceptionCollection.cs
- PermissionListSet.cs
- LongValidatorAttribute.cs
- KeyTime.cs
- BezierSegment.cs
- TextAutomationPeer.cs
- BindingContext.cs
- unitconverter.cs
- ClientType.cs
- InkCanvasInnerCanvas.cs
- XNodeNavigator.cs
- TransformConverter.cs
- StaticSiteMapProvider.cs
- MachineKey.cs
- ExpressionReplacer.cs
- StrokeNodeEnumerator.cs
- Package.cs
- XmlNotation.cs
- ExtenderControl.cs
- EFColumnProvider.cs
- OleDbDataAdapter.cs
- PageFunction.cs
- ConnectionManagementElementCollection.cs
- XmlConverter.cs
- Int64KeyFrameCollection.cs
- NullReferenceException.cs
- SessionStateContainer.cs
- PolicyStatement.cs
- CodeExporter.cs
- ScrollProviderWrapper.cs
- TemplateBindingExpressionConverter.cs
- DataGridCommandEventArgs.cs
- exports.cs
- ConditionalAttribute.cs
- SchemaElement.cs
- LicFileLicenseProvider.cs
- BindingList.cs
- SoapRpcServiceAttribute.cs
- UInt16.cs
- AddingNewEventArgs.cs
- TargetControlTypeCache.cs
- SiteMapProvider.cs
- Utils.cs
- ConfigurationProperty.cs
- WindowsListView.cs
- designeractionlistschangedeventargs.cs
- NameValueFileSectionHandler.cs
- FrameworkElementAutomationPeer.cs
- SmiConnection.cs
- XmlUTF8TextWriter.cs
- RawStylusSystemGestureInputReport.cs
- QueryExpr.cs
- SafeCoTaskMem.cs
- EndpointNameMessageFilter.cs
- XmlSchemaInfo.cs
- NamespaceQuery.cs
- login.cs
- UnicodeEncoding.cs
- SingleBodyParameterMessageFormatter.cs
- FilterException.cs
- CfgParser.cs
- ContextBase.cs
- HostingEnvironment.cs
- WebPartsSection.cs
- CustomError.cs
- QilSortKey.cs