Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / clr / src / BCL / System / Threading / Interlocked.cs / 2 / Interlocked.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System.Threading { using System; using System.Security.Permissions; using System.Runtime.CompilerServices; using System.Runtime.ConstrainedExecution; // After much discussion, we decided the Interlocked class doesn't need // any HPA's for synchronization or external threading. They hurt C#'s // codegen for the yield keyword, and arguably they didn't protect much. // Instead, they penalized people (and compilers) for writing threadsafe // code. public static class Interlocked { /****************************** * Increment * Implemented: int * long *****************************/ [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int Increment(ref int location); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long Increment(ref long location); /****************************** * Decrement * Implemented: int * long *****************************/ [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int Decrement(ref int location); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long Decrement(ref long location); /****************************** * Exchange * Implemented: int * long * float * double * Object * IntPtr *****************************/ [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int Exchange(ref int location1, int value); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long Exchange(ref long location1, long value); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern float Exchange(ref float location1, float value); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern double Exchange(ref double location1, double value); [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern Object Exchange(ref Object location1, Object value); [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern IntPtr Exchange(ref IntPtr location1, IntPtr value); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [System.Runtime.InteropServices.ComVisible(false)] public static T Exchange(ref T location1, T value) where T : class { _Exchange(__makeref(location1), __makeref(value)); //Since value is a local we use trash its data on return // The Exchange replaces the data with new data // so after the return "value" contains the original location1 //See ExchangeGeneric for more details return value; } [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _Exchange(TypedReference location1, TypedReference value); /****************************** * CompareExchange * Implemented: int * long * float * double * Object * IntPtr *****************************/ [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern int CompareExchange(ref int location1, int value, int comparand); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern long CompareExchange(ref long location1, long value, long comparand); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern float CompareExchange(ref float location1, float value, float comparand); [MethodImplAttribute(MethodImplOptions.InternalCall)] public static extern double CompareExchange(ref double location1, double value, double comparand); [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern Object CompareExchange(ref Object location1, Object value, Object comparand); [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static extern IntPtr CompareExchange(ref IntPtr location1, IntPtr value, IntPtr comparand); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] [System.Runtime.InteropServices.ComVisible(false)] public static T CompareExchange (ref T location1, T value, T comparand) where T : class { _CompareExchange(__makeref(location1), __makeref(value), comparand); //Since value is a local we use trash its data on return // The Exchange replaces the data with new data // so after the return "value" contains the original location1 //See CompareExchangeGeneric for more details return value; } [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] private static extern void _CompareExchange(TypedReference location1, TypedReference value, Object comparand); /****************************** * Add * Implemented: int * long *****************************/ [MethodImplAttribute(MethodImplOptions.InternalCall)] [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] internal static extern int ExchangeAdd(ref int location1, int value); [MethodImplAttribute(MethodImplOptions.InternalCall)] internal static extern long ExchangeAdd(ref long location1, long value); [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static int Add(ref int location1, int value) { return ExchangeAdd(ref location1, value) + value; } [ReliabilityContract(Consistency.WillNotCorruptState, Cer.Success)] public static long Add(ref long location1, long value) { return ExchangeAdd(ref location1, value) + value; } /****************************** * Read *****************************/ public static long Read(ref long location) { return Interlocked.CompareExchange(ref location,0,0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
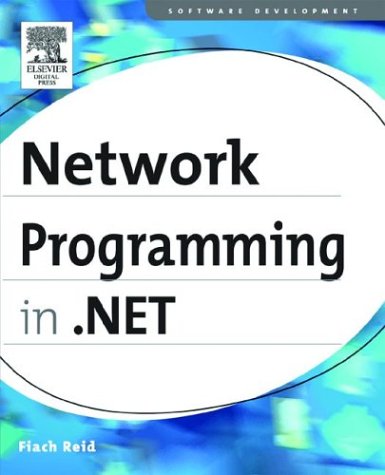
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceInfo.cs
- BaseCollection.cs
- ConfigurationSectionGroup.cs
- HttpPostServerProtocol.cs
- HashCryptoHandle.cs
- FixedPageProcessor.cs
- SafeRightsManagementSessionHandle.cs
- OutputWindow.cs
- RenderOptions.cs
- QueryPageSettingsEventArgs.cs
- UnsafeMethods.cs
- SmiEventSink.cs
- LinqExpressionNormalizer.cs
- DataGridCaption.cs
- BooleanSwitch.cs
- SqlLiftIndependentRowExpressions.cs
- AssemblyUtil.cs
- StrongNameIdentityPermission.cs
- VSWCFServiceContractGenerator.cs
- HyperLinkDataBindingHandler.cs
- WhiteSpaceTrimStringConverter.cs
- XmlQueryStaticData.cs
- AttachInfo.cs
- PropertyInfoSet.cs
- ResponseStream.cs
- GiveFeedbackEventArgs.cs
- ActivityBindForm.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- GridProviderWrapper.cs
- VisualTreeUtils.cs
- PrePrepareMethodAttribute.cs
- TextBreakpoint.cs
- Sentence.cs
- DataSourceDesigner.cs
- DataSourceCache.cs
- XmlReflectionMember.cs
- SoapParser.cs
- DecoderReplacementFallback.cs
- Vector3DAnimationBase.cs
- AutomationAttributeInfo.cs
- basenumberconverter.cs
- Size3D.cs
- SpeechUI.cs
- BrowserCapabilitiesFactory35.cs
- HttpApplicationFactory.cs
- XamlInt32CollectionSerializer.cs
- SqlDependencyUtils.cs
- WebHttpBindingCollectionElement.cs
- safemediahandle.cs
- DataList.cs
- PowerEase.cs
- WMIInterop.cs
- SpeakProgressEventArgs.cs
- RMEnrollmentPage2.cs
- Configuration.cs
- NavigationPropertyAccessor.cs
- HandlerFactoryWrapper.cs
- IODescriptionAttribute.cs
- DependencyObject.cs
- RawKeyboardInputReport.cs
- SessionStateContainer.cs
- CodeTryCatchFinallyStatement.cs
- SizeKeyFrameCollection.cs
- WebAdminConfigurationHelper.cs
- ConfigXmlText.cs
- ChannelCacheDefaults.cs
- FixedDocument.cs
- webeventbuffer.cs
- Directory.cs
- ParallelEnumerable.cs
- TypeLoadException.cs
- QueryRewriter.cs
- GeneralTransform3D.cs
- HtmlHead.cs
- UriSection.cs
- RegexCharClass.cs
- TrackingConditionCollection.cs
- ThicknessAnimationBase.cs
- Deflater.cs
- CatalogZone.cs
- PartitionedStream.cs
- FieldInfo.cs
- ResourceAssociationSetEnd.cs
- SupportingTokenProviderSpecification.cs
- AlphabeticalEnumConverter.cs
- UiaCoreApi.cs
- DataServiceRequestArgs.cs
- _RequestCacheProtocol.cs
- FontStyleConverter.cs
- DriveInfo.cs
- GlobalizationSection.cs
- DependencyObject.cs
- HScrollBar.cs
- UnconditionalPolicy.cs
- Rijndael.cs
- ContentTypeSettingDispatchMessageFormatter.cs
- ISAPIWorkerRequest.cs
- DocumentReference.cs
- XmlAnyElementAttribute.cs
- XmlSchemaAnyAttribute.cs