Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Activities / Designers / EventSinkActivityDesigner.cs / 1305376 / EventSinkActivityDesigner.cs
namespace System.Workflow.Activities { using System; using System.Text; using System.Reflection; using System.Collections; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Drawing2D; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Compiler; using System.Workflow.Activities.Common; #region Class HandleExternalEventActivityDesigner [ActivityDesignerTheme(typeof(EventSinkDesignerTheme))] internal class HandleExternalEventActivityDesigner : ActivityDesigner { protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); object corrRefProperty = properties["CorrelationToken"]; HandleExternalEventActivity eventSink = Activity as HandleExternalEventActivity; AddRemoveCorrelationToken(eventSink.InterfaceType, properties, corrRefProperty); Type type = eventSink.InterfaceType; if (type == null) return; AddRemoveCorrelationToken(type, properties, corrRefProperty); eventSink.GetParameterPropertyDescriptors(properties); } private void AddRemoveCorrelationToken(Type interfaceType, IDictionary properties, object corrRefProperty) { if (interfaceType != null) { object[] corrProvAttribs = interfaceType.GetCustomAttributes(typeof(CorrelationProviderAttribute), false); object[] corrParamAttribs = interfaceType.GetCustomAttributes(typeof(CorrelationParameterAttribute), false); if (corrProvAttribs.Length != 0 || corrParamAttribs.Length != 0) { if (!properties.Contains("CorrelationToken")) properties.Add("CorrelationToken", corrRefProperty); return; } } if (properties.Contains("CorrelationToken")) properties.Remove("CorrelationToken"); } protected override void OnActivityChanged(ActivityChangedEventArgs e) { base.OnActivityChanged(e); if (e.Member != null) { if (e.Member.Name == "InterfaceType") { if (Activity.Site != null) { Type interfaceType = e.NewValue as Type; if (interfaceType != null) new ExternalDataExchangeInterfaceTypeFilterProvider(Activity.Site).CanFilterType(interfaceType, true); HandleExternalEventActivity eventSinkActivity = e.Activity as HandleExternalEventActivity; PropertyDescriptorUtils.SetPropertyValue(Activity.Site, TypeDescriptor.GetProperties(Activity)["EventName"], Activity, String.Empty); IExtendedUIService extUIService = (IExtendedUIService)Activity.Site.GetService(typeof(IExtendedUIService)); if (extUIService == null) throw new Exception(SR.GetString(SR.General_MissingService, typeof(IExtendedUIService).FullName)); } } else if ((e.Member.Name == "EventName") && e.Activity is HandleExternalEventActivity) { (e.Activity as HandleExternalEventActivity).ParameterBindings.Clear(); } if (e.Member.Name == "InterfaceType" || e.Member.Name == "EventName" || e.Member.Name == "CorrelationToken") TypeDescriptor.Refresh(e.Activity); } } } #endregion #region EventSinkDesignerTheme internal sealed class EventSinkDesignerTheme : ActivityDesignerTheme { public EventSinkDesignerTheme(WorkflowTheme theme) : base(theme) { this.ForeColor = Color.FromArgb(0xFF, 0x00, 0x00, 0x00); this.BorderColor = Color.FromArgb(0xFF, 0x9C, 0xAE, 0x73); this.BorderStyle = DashStyle.Solid; this.BackColorStart = Color.FromArgb(0xFF, 0xF5, 0xFB, 0xE1); this.BackColorEnd = Color.FromArgb(0xFF, 0xD6, 0xEB, 0x84); this.BackgroundStyle = LinearGradientMode.Horizontal; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.Activities { using System; using System.Text; using System.Reflection; using System.Collections; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Drawing.Drawing2D; using System.Workflow.ComponentModel; using System.Workflow.ComponentModel.Design; using System.Workflow.ComponentModel.Compiler; using System.Workflow.Activities.Common; #region Class HandleExternalEventActivityDesigner [ActivityDesignerTheme(typeof(EventSinkDesignerTheme))] internal class HandleExternalEventActivityDesigner : ActivityDesigner { protected override void PreFilterProperties(IDictionary properties) { base.PreFilterProperties(properties); object corrRefProperty = properties["CorrelationToken"]; HandleExternalEventActivity eventSink = Activity as HandleExternalEventActivity; AddRemoveCorrelationToken(eventSink.InterfaceType, properties, corrRefProperty); Type type = eventSink.InterfaceType; if (type == null) return; AddRemoveCorrelationToken(type, properties, corrRefProperty); eventSink.GetParameterPropertyDescriptors(properties); } private void AddRemoveCorrelationToken(Type interfaceType, IDictionary properties, object corrRefProperty) { if (interfaceType != null) { object[] corrProvAttribs = interfaceType.GetCustomAttributes(typeof(CorrelationProviderAttribute), false); object[] corrParamAttribs = interfaceType.GetCustomAttributes(typeof(CorrelationParameterAttribute), false); if (corrProvAttribs.Length != 0 || corrParamAttribs.Length != 0) { if (!properties.Contains("CorrelationToken")) properties.Add("CorrelationToken", corrRefProperty); return; } } if (properties.Contains("CorrelationToken")) properties.Remove("CorrelationToken"); } protected override void OnActivityChanged(ActivityChangedEventArgs e) { base.OnActivityChanged(e); if (e.Member != null) { if (e.Member.Name == "InterfaceType") { if (Activity.Site != null) { Type interfaceType = e.NewValue as Type; if (interfaceType != null) new ExternalDataExchangeInterfaceTypeFilterProvider(Activity.Site).CanFilterType(interfaceType, true); HandleExternalEventActivity eventSinkActivity = e.Activity as HandleExternalEventActivity; PropertyDescriptorUtils.SetPropertyValue(Activity.Site, TypeDescriptor.GetProperties(Activity)["EventName"], Activity, String.Empty); IExtendedUIService extUIService = (IExtendedUIService)Activity.Site.GetService(typeof(IExtendedUIService)); if (extUIService == null) throw new Exception(SR.GetString(SR.General_MissingService, typeof(IExtendedUIService).FullName)); } } else if ((e.Member.Name == "EventName") && e.Activity is HandleExternalEventActivity) { (e.Activity as HandleExternalEventActivity).ParameterBindings.Clear(); } if (e.Member.Name == "InterfaceType" || e.Member.Name == "EventName" || e.Member.Name == "CorrelationToken") TypeDescriptor.Refresh(e.Activity); } } } #endregion #region EventSinkDesignerTheme internal sealed class EventSinkDesignerTheme : ActivityDesignerTheme { public EventSinkDesignerTheme(WorkflowTheme theme) : base(theme) { this.ForeColor = Color.FromArgb(0xFF, 0x00, 0x00, 0x00); this.BorderColor = Color.FromArgb(0xFF, 0x9C, 0xAE, 0x73); this.BorderStyle = DashStyle.Solid; this.BackColorStart = Color.FromArgb(0xFF, 0xF5, 0xFB, 0xE1); this.BackColorEnd = Color.FromArgb(0xFF, 0xD6, 0xEB, 0x84); this.BackgroundStyle = LinearGradientMode.Horizontal; } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
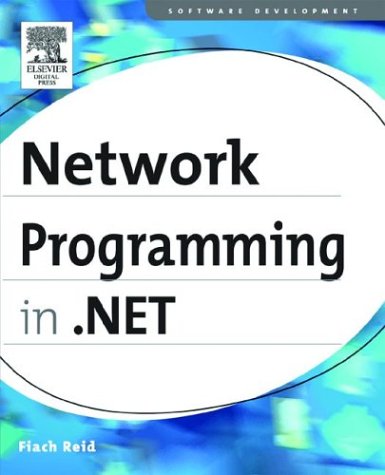
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultipartContentParser.cs
- Input.cs
- InfoCardRSACryptoProvider.cs
- _ContextAwareResult.cs
- RenderingEventArgs.cs
- GeneralTransform3DTo2DTo3D.cs
- LocalizabilityAttribute.cs
- EventSetter.cs
- MatrixConverter.cs
- SecurityPolicySection.cs
- ScriptingProfileServiceSection.cs
- CachingHintValidation.cs
- ComAwareEventInfo.cs
- MyContact.cs
- IChannel.cs
- TreeViewImageIndexConverter.cs
- GridViewCommandEventArgs.cs
- Win32KeyboardDevice.cs
- SequentialUshortCollection.cs
- SafeNativeMemoryHandle.cs
- BidOverLoads.cs
- _AutoWebProxyScriptHelper.cs
- InvalidOperationException.cs
- RotateTransform.cs
- Symbol.cs
- AnimatedTypeHelpers.cs
- CookieParameter.cs
- InvokeSchedule.cs
- BindingMAnagerBase.cs
- PropertyMapper.cs
- InputProviderSite.cs
- DbBuffer.cs
- StreamUpdate.cs
- DateTimeSerializationSection.cs
- KeyInfo.cs
- FunctionOverloadResolver.cs
- SiteIdentityPermission.cs
- activationcontext.cs
- SchemaDeclBase.cs
- ObjectConverter.cs
- ObjectDataSourceEventArgs.cs
- ProfessionalColors.cs
- Win32SafeHandles.cs
- AddInEnvironment.cs
- UriSection.cs
- Converter.cs
- CapabilitiesRule.cs
- TextEffectCollection.cs
- GetImportedCardRequest.cs
- TemplateKey.cs
- ListParagraph.cs
- DataTrigger.cs
- ObjectListFieldsPage.cs
- WindowProviderWrapper.cs
- ConfigurationLocationCollection.cs
- UrlMappingCollection.cs
- AppearanceEditorPart.cs
- InvalidCastException.cs
- LabelLiteral.cs
- PropertyMapper.cs
- EventMemberCodeDomSerializer.cs
- Aes.cs
- TextEditorDragDrop.cs
- XmlAttributeOverrides.cs
- GacUtil.cs
- TemplateControlCodeDomTreeGenerator.cs
- TextRunTypographyProperties.cs
- HostedImpersonationContext.cs
- HtmlControl.cs
- ScrollableControlDesigner.cs
- PartitionResolver.cs
- DbDataSourceEnumerator.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- DBCommand.cs
- WorkflowServiceBuildProvider.cs
- CodeTypeMember.cs
- GeneralTransform3DGroup.cs
- AssemblyHash.cs
- HyperLink.cs
- SiteMapDesignerDataSourceView.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- TextBox.cs
- InternalUserCancelledException.cs
- TextEditorMouse.cs
- ButtonBaseAdapter.cs
- SchemaCollectionCompiler.cs
- _HTTPDateParse.cs
- ModelVisual3D.cs
- PropertyManager.cs
- LoginUtil.cs
- DependencyObjectType.cs
- ISSmlParser.cs
- UnsafePeerToPeerMethods.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- RoleGroup.cs
- TextElement.cs
- ProcessManager.cs
- BrowserTree.cs
- TreeViewEvent.cs
- Activator.cs