Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / WorkflowWebHostingModule.cs / 1305376 / WorkflowWebHostingModule.cs
/******************************************************************************** // Copyright (C) 2000-2001 Microsoft Corporation. All rights reserved. // // CONTENTS // Workflow Web Hosting Module. // DESCRIPTION // Implementation of Workflow Web Host Module. // REVISIONS // Date Ver By Remarks // ~~~~~~~~~~ ~~~ ~~~~~~~~ ~~~~~~~~~~~~~~ // 02/22/05 1.0 [....] Implementation. * ****************************************************************************/ #region Using directives using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Web; using System.Collections.Specialized; using System.Threading; #endregion namespace System.Workflow.Runtime.Hosting { ////// Cookie based rotuing module implementation /// public sealed class WorkflowWebHostingModule : IHttpModule { HttpApplication currentApplication; public WorkflowWebHostingModule() { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Workflow Web Hosting Module Created"); } ////// IHttpModule.Init() /// /// void IHttpModule.Init(HttpApplication application) { WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Workflow Web Hosting Module Initialized"); this.currentApplication = application; //Listen for Acquire and ReleaseRequestState event application.ReleaseRequestState += this.OnReleaseRequestState; application.AcquireRequestState += this.OnAcquireRequestState; } void IHttpModule.Dispose() { } void OnAcquireRequestState(Object sender, EventArgs e) { //Performs Cookie based routing. WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "WebHost Module Routing Begin"); HttpCookie routingCookie = HttpContext.Current.Request.Cookies.Get("WF_WorkflowInstanceId"); if (routingCookie != null) { HttpContext.Current.Items.Add("__WorkflowInstanceId__", new Guid(routingCookie.Value)); } //else no routing information found, it could be activation request or non workflow based request. } void OnReleaseRequestState(Object sender, EventArgs e) { //Saves cookie back to client. HttpCookie cookie = HttpContext.Current.Request.Cookies.Get("WF_WorkflowInstanceId"); if (cookie == null) { cookie = new HttpCookie("WF_WorkflowInstanceId"); Object workflowInstanceId = HttpContext.Current.Items["__WorkflowInstanceId__"]; if (workflowInstanceId != null) { cookie.Value = workflowInstanceId.ToString(); HttpContext.Current.Response.Cookies.Add(cookie); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
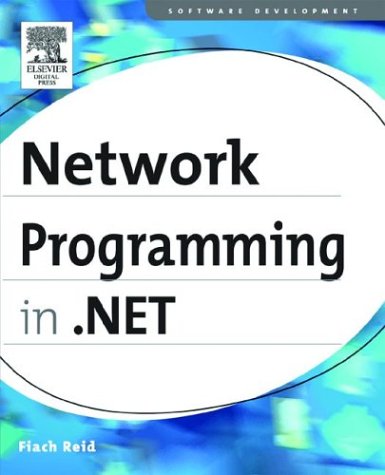
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- X509ChainElement.cs
- KeyBinding.cs
- StylusPlugin.cs
- InvalidProgramException.cs
- PropertyManager.cs
- Config.cs
- ParentQuery.cs
- MappingItemCollection.cs
- MultiBinding.cs
- QueryCursorEventArgs.cs
- Throw.cs
- GridViewColumn.cs
- NativeMethods.cs
- SpeechEvent.cs
- GenericTypeParameterBuilder.cs
- UIElementAutomationPeer.cs
- CallbackValidatorAttribute.cs
- ApplicationHost.cs
- DeviceSpecificChoiceCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- XPathScanner.cs
- ExtensionDataObject.cs
- HwndStylusInputProvider.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- OdbcConnectionStringbuilder.cs
- RelationshipFixer.cs
- BamlRecordReader.cs
- QilReference.cs
- DefaultExpressionVisitor.cs
- ImageDrawing.cs
- TextRangeBase.cs
- DataGridViewAddColumnDialog.cs
- FontDifferentiator.cs
- XPathAncestorQuery.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- RepeatButtonAutomationPeer.cs
- GridViewRow.cs
- WorkflowDebuggerSteppingAttribute.cs
- OleDbFactory.cs
- WebPartConnectionsCancelVerb.cs
- TypeGeneratedEventArgs.cs
- ComPlusDiagnosticTraceRecords.cs
- ImageCodecInfoPrivate.cs
- HttpListenerRequest.cs
- EditorAttribute.cs
- IndentTextWriter.cs
- EmptyTextWriter.cs
- FullTrustAssemblyCollection.cs
- ConvertBinder.cs
- ProxyGenerationError.cs
- SchemaTableOptionalColumn.cs
- MeasurementDCInfo.cs
- SqlExpander.cs
- TabletCollection.cs
- SecurityListenerSettingsLifetimeManager.cs
- TreeViewImageGenerator.cs
- QueryResponse.cs
- PipelineModuleStepContainer.cs
- KeyEvent.cs
- DataGridViewIntLinkedList.cs
- SymDocumentType.cs
- WebPartUserCapability.cs
- FixedTextSelectionProcessor.cs
- SqlHelper.cs
- ContentValidator.cs
- SafeNativeMethods.cs
- ExpressionServices.cs
- SingleAnimationBase.cs
- CompilerErrorCollection.cs
- ServiceConfigurationTraceRecord.cs
- WsatRegistrationHeader.cs
- PackWebResponse.cs
- WinFormsUtils.cs
- DomNameTable.cs
- Serializer.cs
- _OverlappedAsyncResult.cs
- CodeAccessPermission.cs
- x509utils.cs
- SqlUserDefinedAggregateAttribute.cs
- ReflectPropertyDescriptor.cs
- Int64Converter.cs
- CompositeActivityDesigner.cs
- DateTimePicker.cs
- SchemaRegistration.cs
- CacheDependency.cs
- LoadRetryStrategyFactory.cs
- XmlSchemaValidator.cs
- XPathSingletonIterator.cs
- HttpResponseHeader.cs
- StorageBasedPackageProperties.cs
- XmlSchemaAttributeGroup.cs
- DelegatingTypeDescriptionProvider.cs
- Rectangle.cs
- DeviceFiltersSection.cs
- ObjectAnimationBase.cs
- ChangeConflicts.cs
- InputBinding.cs
- ResourceLoader.cs
- IgnoreFileBuildProvider.cs
- TailCallAnalyzer.cs