Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / Mobile / MobileDeviceCapabilitiesSectionHandler.cs / 1305376 / MobileDeviceCapabilitiesSectionHandler.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Mobile { using System.Collections; using System.Configuration; using System.Reflection; using System.Xml; using System.Security.Permissions; ///[AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class MobileDeviceCapabilitiesSectionHandler : IConfigurationSectionHandler { // IConfigurationSectionHandler Methods /// /// Object IConfigurationSectionHandler.Create(Object parent, Object context, XmlNode node) { return Create(parent, context, node); } protected object Create(Object parent, Object context, XmlNode node) { // see ASURT 123738 if (context == null || context.GetType() != typeof(System.Web.Configuration.HttpConfigurationContext)) { return null; } DeviceFilterDictionary currentFilterDictionary; if(parent == null) { currentFilterDictionary = new DeviceFilterDictionary(); } else { currentFilterDictionary = new DeviceFilterDictionary( (DeviceFilterDictionary)parent); } ConfigurationSectionHelper helper = new ConfigurationSectionHelper(); foreach(XmlNode child in node.ChildNodes) { helper.Node = child; // skip whitespace and comments if(helper.IsWhitespaceOrComment()) { continue; } // reject nonelements helper.RejectNonElement(); // handle tags if(child.Name.Equals("filter")) { String name = helper.RemoveStringAttribute("name", true); String className = helper.RemoveStringAttribute("type", false); if(className != null) { const String methodAttributeName = "method"; String methodName = helper.RemoveStringAttribute(methodAttributeName, false); String capabilityName = helper.RemoveStringAttribute("compare", false); String argumentValue = helper.RemoveStringAttribute("argument", false); helper.CheckForUnrecognizedAttributes(); if(className == String.Empty) { throw new ConfigurationErrorsException(SR.GetString(SR.DevCapSect_EmptyClass), child); } if(methodName == null) { throw new ConfigurationErrorsException(SR.GetString(SR.ConfigSect_MissingAttr, methodAttributeName), child); } if(methodName == String.Empty) { throw new ConfigurationErrorsException(SR.GetString(SR.ConfigSect_MissingValue, methodAttributeName), child); } if(capabilityName != null || argumentValue != null) { String msg; if (capabilityName != null) { msg = SR.GetString(SR.DevCapSect_ExtraCompareDelegator); } else { msg = SR.GetString(SR.DevCapSect_ExtraArgumentDelegator); } throw new ConfigurationErrorsException(msg, child); } MobileCapabilities.EvaluateCapabilitiesDelegate evaluator; Type evaluatorClass = Type.GetType(className); if(null == evaluatorClass) { String msg = SR.GetString(SR.DevCapSect_NoTypeInfo, className); throw new ConfigurationErrorsException(msg, child); } try { evaluator = (MobileCapabilities.EvaluateCapabilitiesDelegate) MobileCapabilities.EvaluateCapabilitiesDelegate.CreateDelegate( typeof(MobileCapabilities.EvaluateCapabilitiesDelegate), evaluatorClass, methodName); } catch(Exception e) { String msg = SR.GetString(SR.DevCapSect_NoCapabilityEval, methodName, e.Message); throw new ConfigurationErrorsException(msg, child); } currentFilterDictionary.AddCapabilityDelegate(name, evaluator); } else { String capabilityName = helper.RemoveStringAttribute("compare", false); String argumentValue = helper.RemoveStringAttribute("argument", false); String methodName = helper.RemoveStringAttribute("method", false); helper.CheckForUnrecognizedAttributes(); if(String.IsNullOrEmpty(capabilityName)) { throw new ConfigurationErrorsException( SR.GetString(SR.DevCapSect_MustSpecify), child); } if(methodName != null) { throw new ConfigurationErrorsException( SR.GetString(SR.DevCapSect_ComparisonAlreadySpecified), child); } try { currentFilterDictionary.AddComparisonDelegate(name, capabilityName, argumentValue); } catch(Exception e) { String msg = SR.GetString(SR.DevCapSect_UnableAddDelegate, name, e.Message); throw new ConfigurationErrorsException(msg, child); } } } else { String msg = SR.GetString(SR.DevCapSect_UnrecognizedTag, child.Name); throw new ConfigurationErrorsException(msg, child); } helper.Node = null; } return currentFilterDictionary; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
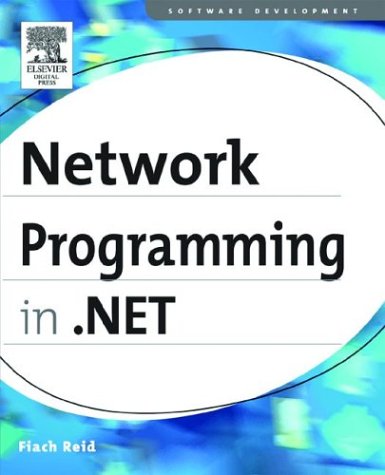
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfessionalColorTable.cs
- TemplateControl.cs
- MachineKeySection.cs
- MenuItem.cs
- ClockGroup.cs
- Binding.cs
- XPathDocumentIterator.cs
- DataConnectionHelper.cs
- SerializationUtility.cs
- AuthenticateEventArgs.cs
- DateTimeOffsetAdapter.cs
- LinkedResource.cs
- SecurityKeyIdentifierClause.cs
- ToolStripPanelRow.cs
- Expression.cs
- OdbcConnectionString.cs
- DesignerLoader.cs
- ValidationErrorCollection.cs
- StickyNoteAnnotations.cs
- OleDbMetaDataFactory.cs
- CellIdBoolean.cs
- DSGeneratorProblem.cs
- Panel.cs
- TypeDelegator.cs
- Bitmap.cs
- DateRangeEvent.cs
- RTLAwareMessageBox.cs
- ObjectAnimationBase.cs
- DataStreamFromComStream.cs
- MenuEventArgs.cs
- WinEventWrap.cs
- WebReferencesBuildProvider.cs
- XmlLanguageConverter.cs
- RecognitionResult.cs
- ThreadExceptionDialog.cs
- SymmetricKey.cs
- ComplexObject.cs
- Span.cs
- PropertyFilter.cs
- RequestChannelBinder.cs
- BitmapFrame.cs
- PasswordTextNavigator.cs
- StreamSecurityUpgradeProvider.cs
- PersonalizableTypeEntry.cs
- TrimSurroundingWhitespaceAttribute.cs
- GridLengthConverter.cs
- MouseActionValueSerializer.cs
- ImportContext.cs
- Imaging.cs
- WindowsGraphics.cs
- InputScopeAttribute.cs
- TraceListeners.cs
- diagnosticsswitches.cs
- PersonalizationAdministration.cs
- ApplicationGesture.cs
- BinaryReader.cs
- TraceSwitch.cs
- StorageEntityContainerMapping.cs
- TextEndOfSegment.cs
- DropSource.cs
- ConditionValidator.cs
- ThicknessAnimationBase.cs
- MediaContext.cs
- DispatchWrapper.cs
- GeometryValueSerializer.cs
- DataGridViewControlCollection.cs
- RIPEMD160Managed.cs
- SqlSupersetValidator.cs
- Update.cs
- ActivityBuilderHelper.cs
- NavigationWindow.cs
- Pointer.cs
- StringStorage.cs
- ClientCultureInfo.cs
- WindowsUpDown.cs
- EnumBuilder.cs
- UInt64.cs
- TextTreeTextElementNode.cs
- DataColumnSelectionConverter.cs
- CreatingCookieEventArgs.cs
- FormViewUpdatedEventArgs.cs
- CodeVariableReferenceExpression.cs
- DemultiplexingClientMessageFormatter.cs
- AdornerHitTestResult.cs
- FolderLevelBuildProvider.cs
- XmlSchemaSimpleContent.cs
- WindowsStartMenu.cs
- NativeRecognizer.cs
- SmiContextFactory.cs
- RadioButtonList.cs
- ErrorFormatterPage.cs
- SmtpNetworkElement.cs
- TrustSection.cs
- ButtonField.cs
- _FtpDataStream.cs
- JoinSymbol.cs
- TrustManagerMoreInformation.cs
- ErrorWebPart.cs
- Canvas.cs
- TypeResolvingOptions.cs