Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / SystemNet / Net / PeerToPeer / PnrpPermission.cs / 1305376 / PnrpPermission.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net.PeerToPeer { using System.Security; using System.Security.Permissions; using System.Globalization; ////// PnrpPermission atrribute /// [AttributeUsage(AttributeTargets.Method | AttributeTargets.Constructor | AttributeTargets.Class | AttributeTargets.Struct | AttributeTargets.Assembly, AllowMultiple = true, Inherited = false)] [Serializable()] public sealed class PnrpPermissionAttribute : CodeAccessSecurityAttribute { ////// Just call base constructor /// /// public PnrpPermissionAttribute(SecurityAction action) : base(action) { } ////// As required by the SecurityAttribute class. /// ///public override IPermission CreatePermission() { if (Unrestricted) { return new PnrpPermission(PermissionState.Unrestricted); } else { return new PnrpPermission(PermissionState.None); } } } /// /// Currently we only support two levels - Unrestrictred or none /// [Serializable] public sealed class PnrpPermission : CodeAccessPermission, IUnrestrictedPermission { private bool m_noRestriction; internal static readonly PnrpPermission UnrestrictedPnrpPermission = new PnrpPermission(PermissionState.Unrestricted); ////// public PnrpPermission(PermissionState state) { m_noRestriction = (state == PermissionState.Unrestricted); } internal PnrpPermission(bool free) { m_noRestriction = free; } // IUnrestrictedPermission interface methods ////// Creates a new instance of the ////// class that passes all demands or that fails all demands. /// /// public bool IsUnrestricted() { return m_noRestriction; } // IPermission interface methods ////// Checks the overall permission state of the object. /// ////// public override IPermission Copy() { return new PnrpPermission(m_noRestriction); } ////// Creates a copy of a ///instance. /// /// public override IPermission Union(IPermission target) { // Pattern suggested by Security engine if (target == null) { return this.Copy(); } PnrpPermission other = target as PnrpPermission; if (other == null) { throw new ArgumentException( SR.GetString(SR.PnrpPermission_CantUnionWithNonPnrpPermission), "target"); } return new PnrpPermission(m_noRestriction || other.m_noRestriction); } ///Returns the logical union between two ///instances. /// public override IPermission Intersect(IPermission target) { // Pattern suggested by Security engine if (target == null) { return null; } PnrpPermission other = target as PnrpPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.PnrpPermission_CantIntersectWithNonPnrpPermission), "target"); } // return null if resulting permission is restricted and empty // Hence, the only way for a bool permission will be. if (this.m_noRestriction && other.m_noRestriction) { return new PnrpPermission(true); } return null; } ///Returns the logical intersection between two ///instances. /// public override bool IsSubsetOf(IPermission target) { // Pattern suggested by Security engine if (target == null) { return m_noRestriction == false; } PnrpPermission other = target as PnrpPermission; if (other == null) { throw new ArgumentException(SR.GetString(SR.PnrpPermission_TargetNotAPnrpPermission), "target"); } //Here is the matrix of result based on m_noRestriction for me and she // me.noRestriction she.noRestriction me.isSubsetOf(she) // 0 0 1 // 0 1 1 // 1 0 0 // 1 1 1 return (!m_noRestriction || other.m_noRestriction); } ///Compares two ///instances. /// Cinstrcy from a security element /// /// public override void FromXml(SecurityElement e) { if (e == null) { throw new ArgumentNullException(SR.GetString(SR.InvalidSecurityElem)); } // SecurityElement must be a permission element if (!e.Tag.Equals("IPermission")) { throw new ArgumentException(SR.GetString(SR.InvalidSecurityElem), "securityElement"); } string className = e.Attribute("class"); // SecurityElement must be a permission element for this type if (className == null) { throw new ArgumentException(SR.GetString(SR.InvalidSecurityElem), "securityElement"); } if (className.IndexOf(this.GetType().FullName, StringComparison.Ordinal) < 0) { throw new ArgumentException(SR.GetString(SR.InvalidSecurityElem), "securityElement"); } string str = e.Attribute("Unrestricted"); m_noRestriction = (str != null ? (0 == string.Compare(str, "true", StringComparison.OrdinalIgnoreCase)) : false); } ////// Copyto a security element /// ///public override SecurityElement ToXml() { SecurityElement securityElement = new SecurityElement("IPermission"); securityElement.AddAttribute("class", this.GetType().FullName + ", " + this.GetType().Module.Assembly.FullName.Replace('\"', '\'')); securityElement.AddAttribute("version", "1"); if (m_noRestriction) { securityElement.AddAttribute("Unrestricted", "true"); } return securityElement; } } // class PnrpPermission } // namespace System.Net.PeerToPeer // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
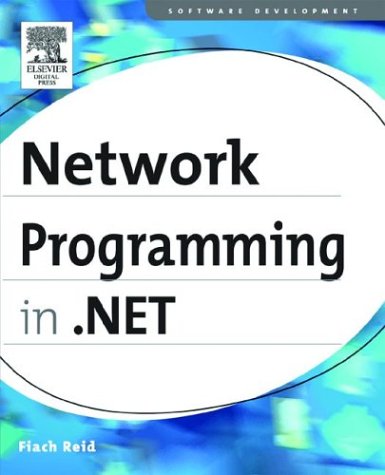
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateKeyConverter.cs
- UnescapedXmlDiagnosticData.cs
- metadatamappinghashervisitor.cs
- Timer.cs
- SqlDataReaderSmi.cs
- ButtonRenderer.cs
- StringExpressionSet.cs
- BaseUriHelper.cs
- Profiler.cs
- OleDbParameterCollection.cs
- ListViewItemMouseHoverEvent.cs
- CompilerCollection.cs
- DataRowChangeEvent.cs
- WizardDesigner.cs
- MouseOverProperty.cs
- TcpHostedTransportConfiguration.cs
- RootNamespaceAttribute.cs
- CollectionViewSource.cs
- login.cs
- StylusPointPropertyInfoDefaults.cs
- HashSetDebugView.cs
- StyleModeStack.cs
- InfoCardSchemas.cs
- ToolboxBitmapAttribute.cs
- Line.cs
- BufferBuilder.cs
- TemplateComponentConnector.cs
- DrawToolTipEventArgs.cs
- ResetableIterator.cs
- DivideByZeroException.cs
- DataSourceView.cs
- ListViewInsertedEventArgs.cs
- OrderedDictionaryStateHelper.cs
- LiteralControl.cs
- SQLDecimal.cs
- SqlNodeTypeOperators.cs
- TableItemStyle.cs
- ClientRolePrincipal.cs
- ArgumentOutOfRangeException.cs
- ConfigurationErrorsException.cs
- KeyFrames.cs
- ChildDocumentBlock.cs
- XmlSchemaSet.cs
- FontFamilyIdentifier.cs
- _ConnectionGroup.cs
- TdsParserStaticMethods.cs
- KeyValuePairs.cs
- Error.cs
- MembershipValidatePasswordEventArgs.cs
- DataGridViewLinkColumn.cs
- NavigationCommands.cs
- UnmanagedMarshal.cs
- NativeMethods.cs
- QueryExecutionOption.cs
- DurableInstanceProvider.cs
- CompModSwitches.cs
- InvalidAsynchronousStateException.cs
- TrustLevelCollection.cs
- ClickablePoint.cs
- CompatibleIComparer.cs
- FontStretches.cs
- NominalTypeEliminator.cs
- WindowsFormsHelpers.cs
- WhitespaceSignificantCollectionAttribute.cs
- RootBuilder.cs
- ObjectStorage.cs
- DataGridItem.cs
- MetadataHelper.cs
- ProxyManager.cs
- TypefaceMap.cs
- ActivityExecutorOperation.cs
- CodeCommentStatementCollection.cs
- SiteMap.cs
- ErrorHandler.cs
- ImmComposition.cs
- Color.cs
- WeakEventTable.cs
- StoreAnnotationsMap.cs
- PriorityQueue.cs
- DataViewListener.cs
- NativeRecognizer.cs
- Psha1DerivedKeyGenerator.cs
- MissingSatelliteAssemblyException.cs
- FlowPosition.cs
- EntityStoreSchemaFilterEntry.cs
- CryptoApi.cs
- ExternalFile.cs
- XmlNodeList.cs
- DataColumnChangeEvent.cs
- CollectionBase.cs
- TraceSection.cs
- RowCache.cs
- XPathAncestorQuery.cs
- BuildResult.cs
- DataGridAutoFormat.cs
- Root.cs
- StackSpiller.Temps.cs
- DataSourceCacheDurationConverter.cs
- StreamUpgradeAcceptor.cs
- SuppressMessageAttribute.cs