Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / TypeGenericEnumerableViewSchema.cs / 2 / TypeGenericEnumerableViewSchema.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// Represents a View's schema based on a generic IEnumerable. The /// strongly-typed row type is determined based on the generic argument /// of the enumerable. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] internal sealed class TypeGenericEnumerableViewSchema : BaseTypeViewSchema { public TypeGenericEnumerableViewSchema(string viewName, Type type) : base(viewName, type) { Debug.Assert(TypeSchema.IsBoundGenericEnumerable(type), String.Format(CultureInfo.InvariantCulture, "The type '{0}' does not implement System.Collections.Generic.IEnumerable, or the argument T is not bound to a type.", type.FullName)); } protected override Type GetRowType(Type objectType) { // Get the IEnumerable declaration Type enumerableType = null; if (objectType.IsInterface && objectType.IsGenericType && objectType.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { // If the type already is IEnumerable , that's the interface we want to inspect enumerableType = objectType; } else { // Otherwise we get a list of all the interafaces the type implements Type[] interfaces = objectType.GetInterfaces(); foreach (Type i in interfaces) { if (i.IsGenericType && i.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { enumerableType = i; break; } } } Debug.Assert(enumerableType != null, "Should have found an implementation of IEnumerable "); // Now return the value of the T argument Type[] genericArguments = enumerableType.GetGenericArguments(); Debug.Assert(genericArguments != null && genericArguments.Length == 1, "Expected IEnumerable to have a generic argument"); // If a type has IsGenericParameter=true that means it is not bound. if (genericArguments[0].IsGenericParameter) { Debug.Fail("Expected the type argument to IEnumerable to be bound"); return null; } else { return genericArguments[0]; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
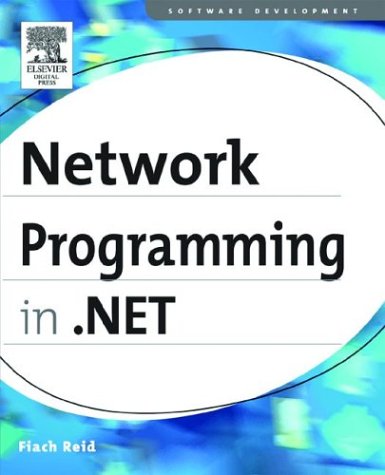
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SatelliteContractVersionAttribute.cs
- AudioDeviceOut.cs
- DependencyPropertyHelper.cs
- XsltQilFactory.cs
- DescendantOverDescendantQuery.cs
- AppDomainManager.cs
- PipelineModuleStepContainer.cs
- URLIdentityPermission.cs
- InternalPermissions.cs
- GridItemCollection.cs
- TableRow.cs
- XPathNavigator.cs
- Storyboard.cs
- _ConnectOverlappedAsyncResult.cs
- SmiMetaData.cs
- PrePostDescendentsWalker.cs
- CodeIterationStatement.cs
- XmlSignificantWhitespace.cs
- ArraySortHelper.cs
- DataError.cs
- MenuItemStyleCollection.cs
- Highlights.cs
- Transform3DGroup.cs
- ObjectQueryState.cs
- HttpRuntime.cs
- TextServicesDisplayAttribute.cs
- SetStateEventArgs.cs
- RewritingPass.cs
- CompositeActivityTypeDescriptorProvider.cs
- PointHitTestParameters.cs
- uribuilder.cs
- XPathDocumentBuilder.cs
- SimpleTypeResolver.cs
- AppSettingsReader.cs
- EventData.cs
- ActivityTypeDesigner.xaml.cs
- TextHidden.cs
- HttpVersion.cs
- SwitchDesigner.xaml.cs
- BuildProviderUtils.cs
- PropertyTabChangedEvent.cs
- Command.cs
- OLEDB_Enum.cs
- MetadataItem.cs
- WpfKnownTypeInvoker.cs
- TextElementCollectionHelper.cs
- TypeRestriction.cs
- RawTextInputReport.cs
- Margins.cs
- ContentPresenter.cs
- PolicyException.cs
- SamlAttributeStatement.cs
- CommandPlan.cs
- XmlCharCheckingWriter.cs
- ReadOnlyCollectionBuilder.cs
- SqlTypeConverter.cs
- WsdlImporterElement.cs
- UDPClient.cs
- WindowsSysHeader.cs
- PersonalizationStateInfoCollection.cs
- BaseCollection.cs
- XmlCompatibilityReader.cs
- PointValueSerializer.cs
- recordstate.cs
- filewebrequest.cs
- LinqDataSourceDeleteEventArgs.cs
- CounterCreationData.cs
- GenericNameHandler.cs
- CancelEventArgs.cs
- WebServiceReceiveDesigner.cs
- CellQuery.cs
- CapabilitiesAssignment.cs
- SerializationFieldInfo.cs
- ContainerActivationHelper.cs
- MdiWindowListStrip.cs
- AmbientValueAttribute.cs
- RegexMatchCollection.cs
- LinkConverter.cs
- Dictionary.cs
- XsdDataContractImporter.cs
- RetriableClipboard.cs
- EncryptedKey.cs
- BuildDependencySet.cs
- HttpCacheVaryByContentEncodings.cs
- XPathPatternBuilder.cs
- FtpCachePolicyElement.cs
- ErrorView.xaml.cs
- XmlUTF8TextReader.cs
- OrderByQueryOptionExpression.cs
- ConsoleTraceListener.cs
- UriGenerator.cs
- HttpProtocolImporter.cs
- Document.cs
- HasCopySemanticsAttribute.cs
- ToolStripSystemRenderer.cs
- QueryableDataSource.cs
- HttpWebRequestElement.cs
- MenuItemCollection.cs
- _KerberosClient.cs
- Tracking.cs