Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Mapping / ViewValidator.cs / 2 / ViewValidator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Globalization; using System.Data.Common.CommandTrees; using System.Collections.Generic; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping { ////// Verifies that only legal expressions exist in a user-defined query mapping view. /// /// - ViewExpr = (see ViewExpressionValidator) /// Project(Projection, ViewExpr) | /// Project(Value, ViewExpr) | /// Filter(ViewExpr, ViewExpr) | /// Join(ViewExpr, ViewExpr, ViewExpr) | /// UnionAll(ViewExpr, ViewExpr) | /// Scan(S-Space EntitySet) /// Constant | /// Property{StructuralProperty}(ViewExpr) | /// Null | /// VariableReference | /// Cast(ViewExpr) | /// Case([Predicate, ViewExpr]*, ViewExpr) | /// Comparison(ViewExpr, ViewExpr) | /// Not(ViewExpr) | /// And(ViewExpr, ViewExpr) | /// Or(ViewExpr, ViewExpr) | /// IsNull(ViewExpr) /// - Projection = (see ViewExpressionValidator.Visit(DbProjectExpression) /// NewInstance{TargetEntity}(ViewExpr*) | /// NewInstance{Row}(ViewExpr*) /// internal static class ViewValidator { ////// Determines whether the given view is valid. /// /// Query view to validate. /// Store item collection. /// Mapping in which view is declared. ///Errors in view definition. internal static IEnumerableValidateQueryView(DbQueryCommandTree view, StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { ViewExpressionValidator validator = new ViewExpressionValidator(storeItemCollection, setMapping, elementType, includeSubtypes); validator.VisitExpression(view.Query); return validator.Errors; } private sealed class ViewExpressionValidator : BasicExpressionVisitor { private readonly StorageSetMapping _setMapping; private readonly StoreItemCollection _storeItemCollection; private readonly List _errors; private readonly EntityTypeBase _elementType; private readonly bool _includeSubtypes; internal ViewExpressionValidator(StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { Debug.Assert(null != setMapping); Debug.Assert(null != storeItemCollection); _setMapping = setMapping; _storeItemCollection = storeItemCollection; _errors = new List (); _elementType = elementType; _includeSubtypes = includeSubtypes; } internal IEnumerable Errors { get { return _errors; } } public override void VisitExpression(DbExpression expression) { if (null != expression) { ValidateExpressionKind(expression.ExpressionKind); } base.VisitExpression(expression); } private void ValidateExpressionKind(DbExpressionKind expressionKind) { switch (expressionKind) { // Supported expression kinds case DbExpressionKind.Constant: case DbExpressionKind.Property: case DbExpressionKind.Null: case DbExpressionKind.VariableReference: case DbExpressionKind.Cast: case DbExpressionKind.Case: case DbExpressionKind.Not: case DbExpressionKind.Or: case DbExpressionKind.And: case DbExpressionKind.IsNull: case DbExpressionKind.Equals: case DbExpressionKind.NotEquals: case DbExpressionKind.LessThan: case DbExpressionKind.LessThanOrEquals: case DbExpressionKind.GreaterThan: case DbExpressionKind.GreaterThanOrEquals: case DbExpressionKind.Project: case DbExpressionKind.NewInstance: case DbExpressionKind.Filter: case DbExpressionKind.Ref: case DbExpressionKind.UnionAll: case DbExpressionKind.Scan: case DbExpressionKind.FullOuterJoin: case DbExpressionKind.LeftOuterJoin: case DbExpressionKind.InnerJoin: case DbExpressionKind.EntityRef: break; default: string elementString = (_includeSubtypes)?"IsTypeOf("+_elementType.ToString()+")":_elementType.ToString(); _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedExpressionKind_QueryView_2( _setMapping.Set.Name, elementString, expressionKind), (int)StorageMappingErrorCode.MappingUnsupportedExpressionKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); break; } } public override void Visit(DbPropertyExpression expression) { base.Visit(expression); if (expression.Property.BuiltInTypeKind != BuiltInTypeKind.EdmProperty) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedPropertyKind_QueryView_3( _setMapping.Set.Name, expression.Property.Name, expression.Property.BuiltInTypeKind), (int)StorageMappingErrorCode.MappingUnsupportedPropertyKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } public override void Visit(DbNewInstanceExpression expression) { base.Visit(expression); EdmType type = expression.ResultType.EdmType; if (type.BuiltInTypeKind != BuiltInTypeKind.RowType) { // restrict initialization of non-row types to the target of the view if (!_setMapping.Set.ElementType.IsAssignableFrom(type)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedInitialization_QueryView_2( _setMapping.Set.Name, type.FullName), (int)StorageMappingErrorCode.MappingUnsupportedInitializationQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } public override void Visit(DbScanExpression expression) { base.Visit(expression); Debug.Assert(null != expression.Target); // verify scan target is in S-space EntitySetBase target = expression.Target; EntityContainer targetContainer = target.EntityContainer; Debug.Assert(null != target.EntityContainer); if ((targetContainer.DataSpace != DataSpace.SSpace)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedScanTarget_QueryView_2( _setMapping.Set.Name, target.Name), (int)StorageMappingErrorCode.MappingUnsupportedScanTargetQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System.Data.Metadata.Edm; using System.Globalization; using System.Data.Common.CommandTrees; using System.Collections.Generic; using System.Diagnostics; using System.Data.Entity; namespace System.Data.Mapping { ////// Verifies that only legal expressions exist in a user-defined query mapping view. /// /// - ViewExpr = (see ViewExpressionValidator) /// Project(Projection, ViewExpr) | /// Project(Value, ViewExpr) | /// Filter(ViewExpr, ViewExpr) | /// Join(ViewExpr, ViewExpr, ViewExpr) | /// UnionAll(ViewExpr, ViewExpr) | /// Scan(S-Space EntitySet) /// Constant | /// Property{StructuralProperty}(ViewExpr) | /// Null | /// VariableReference | /// Cast(ViewExpr) | /// Case([Predicate, ViewExpr]*, ViewExpr) | /// Comparison(ViewExpr, ViewExpr) | /// Not(ViewExpr) | /// And(ViewExpr, ViewExpr) | /// Or(ViewExpr, ViewExpr) | /// IsNull(ViewExpr) /// - Projection = (see ViewExpressionValidator.Visit(DbProjectExpression) /// NewInstance{TargetEntity}(ViewExpr*) | /// NewInstance{Row}(ViewExpr*) /// internal static class ViewValidator { ////// Determines whether the given view is valid. /// /// Query view to validate. /// Store item collection. /// Mapping in which view is declared. ///Errors in view definition. internal static IEnumerableValidateQueryView(DbQueryCommandTree view, StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { ViewExpressionValidator validator = new ViewExpressionValidator(storeItemCollection, setMapping, elementType, includeSubtypes); validator.VisitExpression(view.Query); return validator.Errors; } private sealed class ViewExpressionValidator : BasicExpressionVisitor { private readonly StorageSetMapping _setMapping; private readonly StoreItemCollection _storeItemCollection; private readonly List _errors; private readonly EntityTypeBase _elementType; private readonly bool _includeSubtypes; internal ViewExpressionValidator(StoreItemCollection storeItemCollection, StorageSetMapping setMapping, EntityTypeBase elementType, bool includeSubtypes) { Debug.Assert(null != setMapping); Debug.Assert(null != storeItemCollection); _setMapping = setMapping; _storeItemCollection = storeItemCollection; _errors = new List (); _elementType = elementType; _includeSubtypes = includeSubtypes; } internal IEnumerable Errors { get { return _errors; } } public override void VisitExpression(DbExpression expression) { if (null != expression) { ValidateExpressionKind(expression.ExpressionKind); } base.VisitExpression(expression); } private void ValidateExpressionKind(DbExpressionKind expressionKind) { switch (expressionKind) { // Supported expression kinds case DbExpressionKind.Constant: case DbExpressionKind.Property: case DbExpressionKind.Null: case DbExpressionKind.VariableReference: case DbExpressionKind.Cast: case DbExpressionKind.Case: case DbExpressionKind.Not: case DbExpressionKind.Or: case DbExpressionKind.And: case DbExpressionKind.IsNull: case DbExpressionKind.Equals: case DbExpressionKind.NotEquals: case DbExpressionKind.LessThan: case DbExpressionKind.LessThanOrEquals: case DbExpressionKind.GreaterThan: case DbExpressionKind.GreaterThanOrEquals: case DbExpressionKind.Project: case DbExpressionKind.NewInstance: case DbExpressionKind.Filter: case DbExpressionKind.Ref: case DbExpressionKind.UnionAll: case DbExpressionKind.Scan: case DbExpressionKind.FullOuterJoin: case DbExpressionKind.LeftOuterJoin: case DbExpressionKind.InnerJoin: case DbExpressionKind.EntityRef: break; default: string elementString = (_includeSubtypes)?"IsTypeOf("+_elementType.ToString()+")":_elementType.ToString(); _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedExpressionKind_QueryView_2( _setMapping.Set.Name, elementString, expressionKind), (int)StorageMappingErrorCode.MappingUnsupportedExpressionKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); break; } } public override void Visit(DbPropertyExpression expression) { base.Visit(expression); if (expression.Property.BuiltInTypeKind != BuiltInTypeKind.EdmProperty) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedPropertyKind_QueryView_3( _setMapping.Set.Name, expression.Property.Name, expression.Property.BuiltInTypeKind), (int)StorageMappingErrorCode.MappingUnsupportedPropertyKindQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } public override void Visit(DbNewInstanceExpression expression) { base.Visit(expression); EdmType type = expression.ResultType.EdmType; if (type.BuiltInTypeKind != BuiltInTypeKind.RowType) { // restrict initialization of non-row types to the target of the view if (!_setMapping.Set.ElementType.IsAssignableFrom(type)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedInitialization_QueryView_2( _setMapping.Set.Name, type.FullName), (int)StorageMappingErrorCode.MappingUnsupportedInitializationQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } public override void Visit(DbScanExpression expression) { base.Visit(expression); Debug.Assert(null != expression.Target); // verify scan target is in S-space EntitySetBase target = expression.Target; EntityContainer targetContainer = target.EntityContainer; Debug.Assert(null != target.EntityContainer); if ((targetContainer.DataSpace != DataSpace.SSpace)) { _errors.Add(new EdmSchemaError(System.Data.Entity.Strings.Mapping_UnsupportedScanTarget_QueryView_2( _setMapping.Set.Name, target.Name), (int)StorageMappingErrorCode.MappingUnsupportedScanTargetQueryView, EdmSchemaErrorSeverity.Error, _setMapping.EntityContainerMapping.SourceLocation, _setMapping.StartLineNumber, _setMapping.StartLinePosition)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
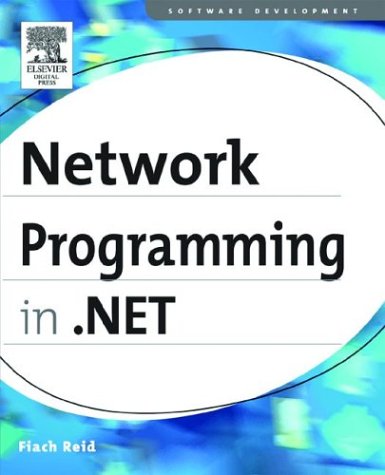
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormClosedEvent.cs
- ToolboxItemFilterAttribute.cs
- PartialCachingControl.cs
- RoleGroup.cs
- PageBuildProvider.cs
- SettingsPropertyIsReadOnlyException.cs
- XmlReflectionMember.cs
- OleDbMetaDataFactory.cs
- X509SecurityTokenProvider.cs
- SafeNativeMethods.cs
- WebSysDefaultValueAttribute.cs
- ConfigUtil.cs
- Line.cs
- SHA512Managed.cs
- DataGridViewRowPostPaintEventArgs.cs
- Size3D.cs
- SynchronizationLockException.cs
- WebControlAdapter.cs
- PrtTicket_Public.cs
- TextDecorationLocationValidation.cs
- SchemaSetCompiler.cs
- ObjectTypeMapping.cs
- ColorTransform.cs
- PointHitTestParameters.cs
- MetaChildrenColumn.cs
- CodeAccessPermission.cs
- ProfilePropertySettings.cs
- SecUtil.cs
- _ConnectStream.cs
- PipeSecurity.cs
- MetadataException.cs
- XamlToRtfParser.cs
- KeyFrames.cs
- WebPartTransformerCollection.cs
- LinqDataSourceInsertEventArgs.cs
- ConfigXmlDocument.cs
- Proxy.cs
- HtmlControlPersistable.cs
- ResourceContainer.cs
- NumericUpDownAcceleration.cs
- CalendarDataBindingHandler.cs
- Attributes.cs
- WebDescriptionAttribute.cs
- PropertyTab.cs
- XmlAttributeHolder.cs
- Expressions.cs
- NamespaceEmitter.cs
- Decoder.cs
- SetterBase.cs
- WorkflowLayouts.cs
- AlphaSortedEnumConverter.cs
- OleDbParameter.cs
- XMLSyntaxException.cs
- DataTransferEventArgs.cs
- _LazyAsyncResult.cs
- EnumValidator.cs
- RelatedView.cs
- CancellationState.cs
- XmlRootAttribute.cs
- InfoCardTraceRecord.cs
- ValueConversionAttribute.cs
- XmlNamespaceManager.cs
- BadImageFormatException.cs
- SQLMoney.cs
- _SSPIWrapper.cs
- DoubleConverter.cs
- Win32SafeHandles.cs
- ScriptControl.cs
- LocalizabilityAttribute.cs
- ExpressionEvaluator.cs
- FragmentQueryKB.cs
- ApplicationFileCodeDomTreeGenerator.cs
- MetadataItemEmitter.cs
- NTAccount.cs
- OdbcConnectionFactory.cs
- AssemblyResourceLoader.cs
- GregorianCalendarHelper.cs
- HttpUnhandledOperationInvoker.cs
- TemplatePartAttribute.cs
- XmlReaderSettings.cs
- IfJoinedCondition.cs
- DigitalSignature.cs
- Style.cs
- TextEditorCharacters.cs
- ActiveXSite.cs
- Sequence.cs
- WhitespaceRule.cs
- ReadOnlyTernaryTree.cs
- GPPOINTF.cs
- FixedPosition.cs
- NamedPipeTransportElement.cs
- ConfigDefinitionUpdates.cs
- GradientBrush.cs
- TdsParameterSetter.cs
- SymbolType.cs
- RetrieveVirtualItemEventArgs.cs
- FixedSOMElement.cs
- CommonProperties.cs
- TemplateBindingExtension.cs
- EntityCommandExecutionException.cs