Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Server / System / Data / Services / DataServiceException.cs / 1 / DataServiceException.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Base class for exceptions thrown by the web data services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; #endregion Namespaces. ////// The exception that is thrown when an error occurs while processing /// a web data service request. /// ////// The DataServiceException is thrown to indicate an error during /// request processing, specifying the appropriate response for /// the request. /// /// RFC2616 about the status code values: /// 1xx: Informational - Request received, continuing process /// "100" ; Section 10.1.1: Continue /// "101" ; Section 10.1.2: Switching Protocols /// /// 2xx: Success - The action was successfully received, understood, and accepted /// "200" ; Section 10.2.1: OK /// "201" ; Section 10.2.2: Created /// "202" ; Section 10.2.3: Accepted /// "203" ; Section 10.2.4: Non-Authoritative Information /// "204" ; Section 10.2.5: No Content /// "205" ; Section 10.2.6: Reset Content /// "206" ; Section 10.2.7: Partial Content /// /// 3xx: Redirection - Further action must be taken in order to complete the request /// "300" ; Section 10.3.1: Multiple Choices /// "301" ; Section 10.3.2: Moved Permanently /// "302" ; Section 10.3.3: Found /// "303" ; Section 10.3.4: See Other /// "304" ; Section 10.3.5: Not Modified /// "305" ; Section 10.3.6: Use Proxy /// "307" ; Section 10.3.8: Temporary Redirect /// /// 4xx: Client Error - The request contains bad syntax or cannot be fulfilled /// "400" ; Section 10.4.1: Bad Request /// "401" ; Section 10.4.2: Unauthorized /// "402" ; Section 10.4.3: Payment Required /// "403" ; Section 10.4.4: Forbidden /// "404" ; Section 10.4.5: Not Found /// "405" ; Section 10.4.6: Method Not Allowed /// "406" ; Section 10.4.7: Not Acceptable /// "407" ; Section 10.4.8: Proxy Authentication Required /// "408" ; Section 10.4.9: Request Time-out /// "409" ; Section 10.4.10: Conflict /// "410" ; Section 10.4.11: Gone /// "411" ; Section 10.4.12: Length Required /// "412" ; Section 10.4.13: Precondition Failed /// "413" ; Section 10.4.14: Request Entity Too Large /// "414" ; Section 10.4.15: Request-URI Too Large /// "415" ; Section 10.4.16: Unsupported Media Type /// "416" ; Section 10.4.17: Requested range not satisfiable /// "417" ; Section 10.4.18: Expectation Failed /// /// 5xx: Server Error - The server failed to fulfill an apparently valid request /// "500" ; Section 10.5.1: Internal Server Error /// "501" ; Section 10.5.2: Not Implemented /// "502" ; Section 10.5.3: Bad Gateway /// "503" ; Section 10.5.4: Service Unavailable /// "504" ; Section 10.5.5: Gateway Time-out /// "505" ; Section 10.5.6: HTTP Version not supported /// [Serializable] [DebuggerDisplay("{statusCode}: {Message}")] public sealed class DataServiceException : InvalidOperationException { #region Private fields. ///Language for the exception message. private readonly string messageLanguage; ///Error code to be used in payloads. private readonly string errorCode; ///HTTP response status code for this exception. private readonly int statusCode; ///'Allow' response for header. private string responseAllowHeader; #endregion Private fields. #region Constructors. ////// Initializes a new instance of the DataServiceException class. /// ////// The Message property is initialized to a system-supplied message /// that describes the error. This message takes into account the /// current system culture. The StatusCode property is set to 500 /// (Internal Server Error). /// public DataServiceException() : this(500, Strings.DataServiceException_GeneralError) { } ////// Initializes a new instance of the DataServiceException class. /// /// Plain text error message for this exception. ////// The StatusCode property is set to 500 (Internal Server Error). /// public DataServiceException(string message) : this(500, message) { } ////// Initializes a new instance of the DataServiceException class. /// /// Plain text error message for this exception. /// Exception that caused this exception to be thrown. ////// The StatusCode property is set to 500 (Internal Server Error). /// public DataServiceException(string message, Exception innerException) : this(500, null, message, null, innerException) { } ////// Initializes a new instance of the DataServiceException class. /// /// HTTP response status code for this exception. /// Plain text error message for this exception. public DataServiceException(int statusCode, string message) : this(statusCode, null, message, null, null) { } ////// Initializes a new instance of the DataServiceException class. /// /// HTTP response status code for this exception. /// Error code to be used in payloads. /// Plain text error message for this exception. /// Language of the. /// Exception that caused this exception to be thrown. public DataServiceException(int statusCode, string errorCode, string message, string messageXmlLang, Exception innerException) : base(message, innerException) { this.errorCode = errorCode ?? String.Empty; this.messageLanguage = messageXmlLang ?? CultureInfo.CurrentCulture.Name; this.statusCode = statusCode; } #pragma warning disable 0628 // Warning CS0628: // A sealed class cannot introduce a protected member because no other class will be able to inherit from the // sealed class and use the protected member. // // This method is used by the runtime when deserializing an exception. It follows the standard pattern, // which will also be necessary when this class is subclassed by DataServiceException . /// /// Initializes a new instance of the DataServiceException class from the /// specified SerializationInfo and StreamingContext instances. /// /// /// A SerializationInfo containing the information required to serialize /// the new DataServiceException. /// /// /// A StreamingContext containing the source of the serialized stream /// associated with the new DataServiceException. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1047", Justification = "Follows serialization info pattern.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032", Justification = "Follows serialization info pattern.")] protected DataServiceException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { if (serializationInfo != null) { this.errorCode = serializationInfo.GetString("errorCode"); this.messageLanguage = serializationInfo.GetString("messageXmlLang"); this.responseAllowHeader = serializationInfo.GetString("responseAllowHeader"); this.statusCode = serializationInfo.GetInt32("statusCode"); } } #pragma warning restore 0628 #endregion Constructors. #region Public properties. ///Error code to be used in payloads. public string ErrorCode { get { return this.errorCode; } } ///Language for the exception Message. public string MessageLanguage { get { return this.messageLanguage; } } ///Response status code for this exception. public int StatusCode { get { return this.statusCode; } } #endregion Public properties. #region Internal properties. ///'Allow' response for header. internal string ResponseAllowHeader { get { return this.responseAllowHeader; } } #endregion Internal properties. #region Methods. ////// Sets the SerializationInfo with information about the exception. /// /// The SerializationInfo that holds the serialized object data about the exception being thrown. /// The StreamingContext that contains contextual information about the source or destination. [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info != null) { info.AddValue("errorCode", this.errorCode); info.AddValue("messageXmlLang", this.messageLanguage); info.AddValue("responseAllowHeader", this.responseAllowHeader); info.AddValue("statusCode", this.statusCode); } base.GetObjectData(info, context); } ///Creates a new "Bad Request" exception for recursion limit exceeded. /// Recursion limit that was reaced. ///A new exception to indicate that the request is rejected. internal static DataServiceException CreateDeepRecursion(int recursionLimit) { return DataServiceException.CreateBadRequestError(Strings.BadRequest_DeepRecursion(recursionLimit)); } ///Creates a new "Forbidden" exception. ///A new exception to indicate that the request is forbidden. internal static DataServiceException CreateForbidden() { // 403: Forbidden return new DataServiceException(403, Strings.RequestUriProcessor_Forbidden); } ///Creates a new "Resource Not Found" exception. /// segment identifier information for which resource was not found. ///A new exception to indicate the requested resource cannot be found. internal static DataServiceException CreateResourceNotFound(string identifier) { // 404: Not Found return new DataServiceException(404, Strings.RequestUriProcessor_ResourceNotFound(identifier)); } ///Creates a new "Resource Not Found" exception. /// Plain text error message for this exception. ///A new exception to indicate the requested resource cannot be found. internal static DataServiceException ResourceNotFoundError(string errorMessage) { // 404: Not Found return new DataServiceException(404, errorMessage); } ///Creates a new exception to indicate a syntax error. ///A new exception to indicate a syntax error. internal static DataServiceException CreateSyntaxError() { return CreateSyntaxError(Strings.RequestUriProcessor_SyntaxError); } ///Creates a new exception to indicate a syntax error. /// Plain text error message for this exception. ///A new exception to indicate a syntax error. internal static DataServiceException CreateSyntaxError(string message) { return DataServiceException.CreateBadRequestError(message); } ////// Creates a new exception to indicate Precondition error. /// /// Plain text error message for this exception. ///A new exception to indicate a Precondition failed error. internal static DataServiceException CreatePreConditionFailedError(string message) { // 412 - Precondition failed return new DataServiceException(412, message); } ////// Creates a new exception to indicate BadRequest error. /// /// Plain text error message for this exception. ///A new exception to indicate a bad request error. internal static DataServiceException CreateBadRequestError(string message) { // 400 - Bad Request return new DataServiceException(400, message); } ////// Creates a new exception to indicate BadRequest error. /// /// Plain text error message for this exception. /// Inner Exception. ///A new exception to indicate a bad request error. internal static DataServiceException CreateBadRequestError(string message, Exception innerException) { // 400 - Bad Request return new DataServiceException(400, null, message, null, innerException); } ///Creates a new "Method Not Allowed" exception. /// Error message. /// String value for 'Allow' header in response. ///A new exception to indicate the requested method is not allowed on the response. internal static DataServiceException CreateMethodNotAllowed(string message, string allow) { // 405 - Method Not Allowed DataServiceException result = new DataServiceException(405, message); result.responseAllowHeader = allow; return result; } ////// Creates a new exception to indicate MethodNotImplemented error. /// /// Plain text error message for this exception. ///A new exception to indicate a MethodNotImplemented error. internal static DataServiceException CreateMethodNotImplemented(string message) { // 501 - Method Not Implemented return new DataServiceException(501, message); } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Base class for exceptions thrown by the web data services. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; using System.Security.Permissions; #endregion Namespaces. ////// The exception that is thrown when an error occurs while processing /// a web data service request. /// ////// The DataServiceException is thrown to indicate an error during /// request processing, specifying the appropriate response for /// the request. /// /// RFC2616 about the status code values: /// 1xx: Informational - Request received, continuing process /// "100" ; Section 10.1.1: Continue /// "101" ; Section 10.1.2: Switching Protocols /// /// 2xx: Success - The action was successfully received, understood, and accepted /// "200" ; Section 10.2.1: OK /// "201" ; Section 10.2.2: Created /// "202" ; Section 10.2.3: Accepted /// "203" ; Section 10.2.4: Non-Authoritative Information /// "204" ; Section 10.2.5: No Content /// "205" ; Section 10.2.6: Reset Content /// "206" ; Section 10.2.7: Partial Content /// /// 3xx: Redirection - Further action must be taken in order to complete the request /// "300" ; Section 10.3.1: Multiple Choices /// "301" ; Section 10.3.2: Moved Permanently /// "302" ; Section 10.3.3: Found /// "303" ; Section 10.3.4: See Other /// "304" ; Section 10.3.5: Not Modified /// "305" ; Section 10.3.6: Use Proxy /// "307" ; Section 10.3.8: Temporary Redirect /// /// 4xx: Client Error - The request contains bad syntax or cannot be fulfilled /// "400" ; Section 10.4.1: Bad Request /// "401" ; Section 10.4.2: Unauthorized /// "402" ; Section 10.4.3: Payment Required /// "403" ; Section 10.4.4: Forbidden /// "404" ; Section 10.4.5: Not Found /// "405" ; Section 10.4.6: Method Not Allowed /// "406" ; Section 10.4.7: Not Acceptable /// "407" ; Section 10.4.8: Proxy Authentication Required /// "408" ; Section 10.4.9: Request Time-out /// "409" ; Section 10.4.10: Conflict /// "410" ; Section 10.4.11: Gone /// "411" ; Section 10.4.12: Length Required /// "412" ; Section 10.4.13: Precondition Failed /// "413" ; Section 10.4.14: Request Entity Too Large /// "414" ; Section 10.4.15: Request-URI Too Large /// "415" ; Section 10.4.16: Unsupported Media Type /// "416" ; Section 10.4.17: Requested range not satisfiable /// "417" ; Section 10.4.18: Expectation Failed /// /// 5xx: Server Error - The server failed to fulfill an apparently valid request /// "500" ; Section 10.5.1: Internal Server Error /// "501" ; Section 10.5.2: Not Implemented /// "502" ; Section 10.5.3: Bad Gateway /// "503" ; Section 10.5.4: Service Unavailable /// "504" ; Section 10.5.5: Gateway Time-out /// "505" ; Section 10.5.6: HTTP Version not supported /// [Serializable] [DebuggerDisplay("{statusCode}: {Message}")] public sealed class DataServiceException : InvalidOperationException { #region Private fields. ///Language for the exception message. private readonly string messageLanguage; ///Error code to be used in payloads. private readonly string errorCode; ///HTTP response status code for this exception. private readonly int statusCode; ///'Allow' response for header. private string responseAllowHeader; #endregion Private fields. #region Constructors. ////// Initializes a new instance of the DataServiceException class. /// ////// The Message property is initialized to a system-supplied message /// that describes the error. This message takes into account the /// current system culture. The StatusCode property is set to 500 /// (Internal Server Error). /// public DataServiceException() : this(500, Strings.DataServiceException_GeneralError) { } ////// Initializes a new instance of the DataServiceException class. /// /// Plain text error message for this exception. ////// The StatusCode property is set to 500 (Internal Server Error). /// public DataServiceException(string message) : this(500, message) { } ////// Initializes a new instance of the DataServiceException class. /// /// Plain text error message for this exception. /// Exception that caused this exception to be thrown. ////// The StatusCode property is set to 500 (Internal Server Error). /// public DataServiceException(string message, Exception innerException) : this(500, null, message, null, innerException) { } ////// Initializes a new instance of the DataServiceException class. /// /// HTTP response status code for this exception. /// Plain text error message for this exception. public DataServiceException(int statusCode, string message) : this(statusCode, null, message, null, null) { } ////// Initializes a new instance of the DataServiceException class. /// /// HTTP response status code for this exception. /// Error code to be used in payloads. /// Plain text error message for this exception. /// Language of the. /// Exception that caused this exception to be thrown. public DataServiceException(int statusCode, string errorCode, string message, string messageXmlLang, Exception innerException) : base(message, innerException) { this.errorCode = errorCode ?? String.Empty; this.messageLanguage = messageXmlLang ?? CultureInfo.CurrentCulture.Name; this.statusCode = statusCode; } #pragma warning disable 0628 // Warning CS0628: // A sealed class cannot introduce a protected member because no other class will be able to inherit from the // sealed class and use the protected member. // // This method is used by the runtime when deserializing an exception. It follows the standard pattern, // which will also be necessary when this class is subclassed by DataServiceException . /// /// Initializes a new instance of the DataServiceException class from the /// specified SerializationInfo and StreamingContext instances. /// /// /// A SerializationInfo containing the information required to serialize /// the new DataServiceException. /// /// /// A StreamingContext containing the source of the serialized stream /// associated with the new DataServiceException. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1047", Justification = "Follows serialization info pattern.")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1032", Justification = "Follows serialization info pattern.")] protected DataServiceException(SerializationInfo serializationInfo, StreamingContext streamingContext) : base(serializationInfo, streamingContext) { if (serializationInfo != null) { this.errorCode = serializationInfo.GetString("errorCode"); this.messageLanguage = serializationInfo.GetString("messageXmlLang"); this.responseAllowHeader = serializationInfo.GetString("responseAllowHeader"); this.statusCode = serializationInfo.GetInt32("statusCode"); } } #pragma warning restore 0628 #endregion Constructors. #region Public properties. ///Error code to be used in payloads. public string ErrorCode { get { return this.errorCode; } } ///Language for the exception Message. public string MessageLanguage { get { return this.messageLanguage; } } ///Response status code for this exception. public int StatusCode { get { return this.statusCode; } } #endregion Public properties. #region Internal properties. ///'Allow' response for header. internal string ResponseAllowHeader { get { return this.responseAllowHeader; } } #endregion Internal properties. #region Methods. ////// Sets the SerializationInfo with information about the exception. /// /// The SerializationInfo that holds the serialized object data about the exception being thrown. /// The StreamingContext that contains contextual information about the source or destination. [SecurityPermissionAttribute(SecurityAction.Demand, SerializationFormatter = true)] public override void GetObjectData(SerializationInfo info, StreamingContext context) { if (info != null) { info.AddValue("errorCode", this.errorCode); info.AddValue("messageXmlLang", this.messageLanguage); info.AddValue("responseAllowHeader", this.responseAllowHeader); info.AddValue("statusCode", this.statusCode); } base.GetObjectData(info, context); } ///Creates a new "Bad Request" exception for recursion limit exceeded. /// Recursion limit that was reaced. ///A new exception to indicate that the request is rejected. internal static DataServiceException CreateDeepRecursion(int recursionLimit) { return DataServiceException.CreateBadRequestError(Strings.BadRequest_DeepRecursion(recursionLimit)); } ///Creates a new "Forbidden" exception. ///A new exception to indicate that the request is forbidden. internal static DataServiceException CreateForbidden() { // 403: Forbidden return new DataServiceException(403, Strings.RequestUriProcessor_Forbidden); } ///Creates a new "Resource Not Found" exception. /// segment identifier information for which resource was not found. ///A new exception to indicate the requested resource cannot be found. internal static DataServiceException CreateResourceNotFound(string identifier) { // 404: Not Found return new DataServiceException(404, Strings.RequestUriProcessor_ResourceNotFound(identifier)); } ///Creates a new "Resource Not Found" exception. /// Plain text error message for this exception. ///A new exception to indicate the requested resource cannot be found. internal static DataServiceException ResourceNotFoundError(string errorMessage) { // 404: Not Found return new DataServiceException(404, errorMessage); } ///Creates a new exception to indicate a syntax error. ///A new exception to indicate a syntax error. internal static DataServiceException CreateSyntaxError() { return CreateSyntaxError(Strings.RequestUriProcessor_SyntaxError); } ///Creates a new exception to indicate a syntax error. /// Plain text error message for this exception. ///A new exception to indicate a syntax error. internal static DataServiceException CreateSyntaxError(string message) { return DataServiceException.CreateBadRequestError(message); } ////// Creates a new exception to indicate Precondition error. /// /// Plain text error message for this exception. ///A new exception to indicate a Precondition failed error. internal static DataServiceException CreatePreConditionFailedError(string message) { // 412 - Precondition failed return new DataServiceException(412, message); } ////// Creates a new exception to indicate BadRequest error. /// /// Plain text error message for this exception. ///A new exception to indicate a bad request error. internal static DataServiceException CreateBadRequestError(string message) { // 400 - Bad Request return new DataServiceException(400, message); } ////// Creates a new exception to indicate BadRequest error. /// /// Plain text error message for this exception. /// Inner Exception. ///A new exception to indicate a bad request error. internal static DataServiceException CreateBadRequestError(string message, Exception innerException) { // 400 - Bad Request return new DataServiceException(400, null, message, null, innerException); } ///Creates a new "Method Not Allowed" exception. /// Error message. /// String value for 'Allow' header in response. ///A new exception to indicate the requested method is not allowed on the response. internal static DataServiceException CreateMethodNotAllowed(string message, string allow) { // 405 - Method Not Allowed DataServiceException result = new DataServiceException(405, message); result.responseAllowHeader = allow; return result; } ////// Creates a new exception to indicate MethodNotImplemented error. /// /// Plain text error message for this exception. ///A new exception to indicate a MethodNotImplemented error. internal static DataServiceException CreateMethodNotImplemented(string message) { // 501 - Method Not Implemented return new DataServiceException(501, message); } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
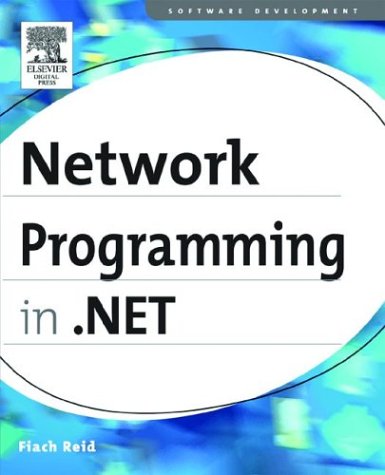
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlEntityReference.cs
- CollectionsUtil.cs
- ShaderRenderModeValidation.cs
- Debug.cs
- TextEditorThreadLocalStore.cs
- ButtonBaseAutomationPeer.cs
- Typography.cs
- COM2PictureConverter.cs
- TransformGroup.cs
- MutexSecurity.cs
- CustomTypeDescriptor.cs
- SmtpSpecifiedPickupDirectoryElement.cs
- PtsCache.cs
- backend.cs
- DesignerSerializerAttribute.cs
- SqlRetyper.cs
- ParseHttpDate.cs
- ControlTemplate.cs
- TableHeaderCell.cs
- LockCookie.cs
- WebServiceAttribute.cs
- SortQuery.cs
- CodeTypeDelegate.cs
- MailBnfHelper.cs
- TableRowGroup.cs
- ControlTemplate.cs
- TextPatternIdentifiers.cs
- DataGridViewColumnCollection.cs
- ConfigurationManagerHelperFactory.cs
- _SSPIWrapper.cs
- CultureInfo.cs
- FormViewInsertEventArgs.cs
- AsymmetricKeyExchangeFormatter.cs
- RtfControlWordInfo.cs
- HitTestWithPointDrawingContextWalker.cs
- Win32Exception.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- NumberFunctions.cs
- AutoGeneratedField.cs
- ServiceBehaviorAttribute.cs
- ProxyGenerationError.cs
- TokenBasedSetEnumerator.cs
- WebSysDescriptionAttribute.cs
- XmlSignatureProperties.cs
- SafeCoTaskMem.cs
- HashMembershipCondition.cs
- WindowsSolidBrush.cs
- Event.cs
- recordstatescratchpad.cs
- StylusDownEventArgs.cs
- TypedReference.cs
- CompositeFontParser.cs
- FixedHighlight.cs
- Module.cs
- AlphaSortedEnumConverter.cs
- TextPenaltyModule.cs
- PrtTicket_Public_Simple.cs
- InternalMappingException.cs
- OpenFileDialog.cs
- UnsafeNativeMethodsMilCoreApi.cs
- PersistenceContextEnlistment.cs
- PointAnimationBase.cs
- MDIClient.cs
- SmtpLoginAuthenticationModule.cs
- NotSupportedException.cs
- PropertyValueChangedEvent.cs
- SecurityTokenContainer.cs
- FileDialogCustomPlacesCollection.cs
- DynamicUpdateCommand.cs
- WebPartEditorOkVerb.cs
- FormViewInsertEventArgs.cs
- AutomationElement.cs
- XmlHierarchyData.cs
- WebResponse.cs
- ControlBuilder.cs
- SmtpNtlmAuthenticationModule.cs
- AncestorChangedEventArgs.cs
- StringUtil.cs
- ContainerVisual.cs
- DataObjectAttribute.cs
- OdbcCommandBuilder.cs
- PasswordDeriveBytes.cs
- Tile.cs
- TextRangeEdit.cs
- VisualProxy.cs
- MasterPageCodeDomTreeGenerator.cs
- DesignerAutoFormatStyle.cs
- StatusBar.cs
- XmlDictionaryReader.cs
- TemplateBamlTreeBuilder.cs
- IndexerReference.cs
- EndpointDiscoveryMetadata.cs
- CreateUserWizardStep.cs
- ColorAnimationBase.cs
- PageStatePersister.cs
- RC2.cs
- DataGridViewRowsRemovedEventArgs.cs
- TaskFileService.cs
- XmlSigningNodeWriter.cs
- SizeFConverter.cs