Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / ArraySegment.cs / 1305376 / ArraySegment.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; using System.Diagnostics.Contracts; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); Contract.EndContractBlock(); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); Contract.EndContractBlock(); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment ** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; using System.Diagnostics.Contracts; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); Contract.EndContractBlock(); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); Contract.EndContractBlock(); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
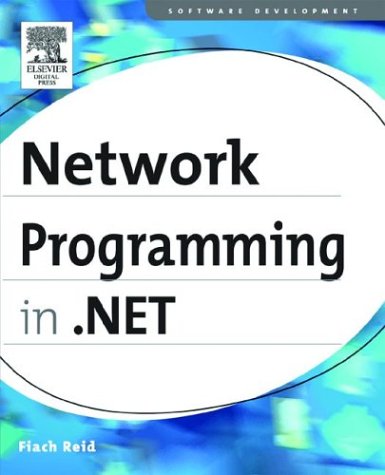
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- filewebrequest.cs
- CodeArrayIndexerExpression.cs
- Color.cs
- followingquery.cs
- graph.cs
- CompositeFontParser.cs
- Point3DCollectionConverter.cs
- Ref.cs
- DeferredTextReference.cs
- DockEditor.cs
- EnumConverter.cs
- PEFileReader.cs
- WindowsImpersonationContext.cs
- ProcessManager.cs
- DialogBaseForm.cs
- DbConnectionStringCommon.cs
- RenderingBiasValidation.cs
- Ppl.cs
- InvalidCastException.cs
- ClonableStack.cs
- DesignTimeVisibleAttribute.cs
- MemoryPressure.cs
- Content.cs
- QuadraticBezierSegment.cs
- SBCSCodePageEncoding.cs
- ProcessProtocolHandler.cs
- OdbcParameter.cs
- Int32EqualityComparer.cs
- ConstraintConverter.cs
- Stack.cs
- ZipFileInfo.cs
- RepeaterItem.cs
- XmlDocumentFragment.cs
- MonitoringDescriptionAttribute.cs
- Filter.cs
- ComboBoxAutomationPeer.cs
- TabRenderer.cs
- WebCategoryAttribute.cs
- UnsafeNativeMethods.cs
- autovalidator.cs
- IncrementalHitTester.cs
- XmlAttribute.cs
- DeviceSpecificDesigner.cs
- Matrix3DStack.cs
- BitmapEffectGroup.cs
- CheckBoxList.cs
- PropertyInformationCollection.cs
- IsolatedStorageFileStream.cs
- SuppressIldasmAttribute.cs
- CompositeActivityCodeGenerator.cs
- ProgressChangedEventArgs.cs
- WsdlInspector.cs
- FileInfo.cs
- SchemaNotation.cs
- ADMembershipProvider.cs
- counter.cs
- ArgumentOutOfRangeException.cs
- EpmCustomContentWriterNodeData.cs
- GetCertificateRequest.cs
- Point3D.cs
- GlyphInfoList.cs
- GetMemberBinder.cs
- PieceNameHelper.cs
- StringSorter.cs
- ResourceLoader.cs
- DbSourceParameterCollection.cs
- CustomAttributeFormatException.cs
- ShapingEngine.cs
- FormViewRow.cs
- SqlNotificationRequest.cs
- OperandQuery.cs
- Material.cs
- ProjectionPlan.cs
- BatchParser.cs
- ReferenceSchema.cs
- Block.cs
- PathSegmentCollection.cs
- PatternMatcher.cs
- LayoutUtils.cs
- CodeThrowExceptionStatement.cs
- ObjectPersistData.cs
- RelationshipConstraintValidator.cs
- JsonClassDataContract.cs
- ModelTreeManager.cs
- Floater.cs
- ResourceCategoryAttribute.cs
- isolationinterop.cs
- AsymmetricKeyExchangeDeformatter.cs
- XmlProcessingInstruction.cs
- DataSourceXmlTextReader.cs
- BuildDependencySet.cs
- AsyncResult.cs
- DetailsViewPagerRow.cs
- Msec.cs
- TypeFieldSchema.cs
- Sentence.cs
- ConfigurationStrings.cs
- ViewValidator.cs
- CommonDialog.cs
- CodeMemberMethod.cs