Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Unary / IndexedSelectQueryOperator.cs / 1305376 / IndexedSelectQueryOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IndexedSelectQueryOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A variant of the Select operator that supplies element index while performing the /// projection operation. This requires cooperation with partitioning and merging to /// guarantee ordering is preserved. /// /// @ internal sealed class IndexedSelectQueryOperator : UnaryQueryOperator { // Selector function. Used to project elements to a transformed view during execution. private Func m_selector; private bool m_prematureMerge = false; // Whether to prematurely merge the input of this operator. //---------------------------------------------------------------------------------------- // Initializes a new select operator. // // Arguments: // child - the child operator or data source from which to pull data // selector - a delegate representing the selector function // // Assumptions: // selector must be non null. // internal IndexedSelectQueryOperator(IEnumerable child, Func selector) :base(child) { Contract.Assert(child != null, "child data source cannot be null"); Contract.Assert(selector != null, "need a selector function"); m_selector = selector; // In an indexed Select, elements must be returned in the order in which // indices were assigned. m_outputOrdered = true; InitOrdinalIndexState(); } private void InitOrdinalIndexState() { OrdinalIndexState indexState = Child.OrdinalIndexState; if (ExchangeUtilities.IsWorseThan(Child.OrdinalIndexState, OrdinalIndexState.Correct)) { m_prematureMerge = true; indexState = OrdinalIndexState.Correct; } Contract.Assert(!ExchangeUtilities.IsWorseThan(indexState, OrdinalIndexState.Correct)); SetOrdinalIndexState(indexState); } //--------------------------------------------------------------------------------------- // Just opens the current operator, including opening the child and wrapping it with // partitions as needed. // internal override QueryResults Open( QuerySettings settings, bool preferStriping) { QueryResults childQueryResults = Child.Open(settings, preferStriping); return IndexedSelectQueryOperatorResults.NewResults(childQueryResults, this, settings, preferStriping); } internal override void WrapPartitionedStream ( PartitionedStream inputStream, IPartitionedStreamRecipient recipient, bool preferStriping, QuerySettings settings) { int partitionCount = inputStream.PartitionCount; // If the index is not correct, we need to reindex. PartitionedStream inputStreamInt; if (m_prematureMerge) { ListQueryResults listResults = QueryOperator .ExecuteAndCollectResults( inputStream, partitionCount, Child.OutputOrdered, preferStriping, settings); inputStreamInt = listResults.GetPartitionedStream(); } else { Contract.Assert(typeof(TKey) == typeof(int)); inputStreamInt = (PartitionedStream )(object)inputStream; } // Since the index is correct, the type of the index must be int PartitionedStream outputStream = new PartitionedStream (partitionCount, Util.GetDefaultComparer (), OrdinalIndexState); for (int i = 0; i < partitionCount; i++) { outputStream[i] = new IndexedSelectQueryOperatorEnumerator(inputStreamInt[i], m_selector); } recipient.Receive(outputStream); } //--------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return m_prematureMerge; } } //--------------------------------------------------------------------------------------- // The enumerator type responsible for projecting elements as it is walked. // class IndexedSelectQueryOperatorEnumerator : QueryOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The data source to enumerate. private readonly Func m_selector; // The actual select function. //---------------------------------------------------------------------------------------- // Instantiates a new select enumerator. // internal IndexedSelectQueryOperatorEnumerator(QueryOperatorEnumerator source, Func selector) { Contract.Assert(source != null); Contract.Assert(selector != null); m_source = source; m_selector = selector; } //--------------------------------------------------------------------------------------- // Straightforward IEnumerator methods. // internal override bool MoveNext(ref TOutput currentElement, ref int currentKey) { // So long as the source has a next element, we have an element. TInput element = default(TInput); if (m_source.MoveNext(ref element, ref currentKey)) { Contract.Assert(m_selector != null, "expected a compiled selection function"); currentElement = m_selector(element, currentKey); return true; } return false; } protected override void Dispose(bool disposing) { m_source.Dispose(); } } //---------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { return Child.AsSequentialQuery(token).Select(m_selector); } //------------------------------------------------------------------------------------ // Query results for an indexed Select operator. The results are indexible if the child // results were indexible. // class IndexedSelectQueryOperatorResults : UnaryQueryOperatorResults { IndexedSelectQueryOperator m_selectOp; // Operator that generated the results int m_childCount; // The number of elements in child results public static QueryResults NewResults( QueryResults childQueryResults, IndexedSelectQueryOperator op, QuerySettings settings, bool preferStriping) { if (childQueryResults.IsIndexible) { return new IndexedSelectQueryOperatorResults( childQueryResults, op, settings, preferStriping); } else { return new UnaryQueryOperatorResults( childQueryResults, op, settings, preferStriping); } } private IndexedSelectQueryOperatorResults( QueryResults childQueryResults, IndexedSelectQueryOperator op, QuerySettings settings, bool preferStriping) : base(childQueryResults, op, settings, preferStriping) { m_selectOp = op; Contract.Assert(m_childQueryResults.IsIndexible); m_childCount = m_childQueryResults.ElementsCount; } internal override int ElementsCount { get { Contract.Assert(m_childCount >= 0); return m_childQueryResults.ElementsCount; } } internal override bool IsIndexible { get { return true; } } internal override TOutput GetElement(int index) { return m_selectOp.m_selector(m_childQueryResults.GetElement(index), index); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // IndexedSelectQueryOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// A variant of the Select operator that supplies element index while performing the /// projection operation. This requires cooperation with partitioning and merging to /// guarantee ordering is preserved. /// /// @ internal sealed class IndexedSelectQueryOperator : UnaryQueryOperator { // Selector function. Used to project elements to a transformed view during execution. private Func m_selector; private bool m_prematureMerge = false; // Whether to prematurely merge the input of this operator. //---------------------------------------------------------------------------------------- // Initializes a new select operator. // // Arguments: // child - the child operator or data source from which to pull data // selector - a delegate representing the selector function // // Assumptions: // selector must be non null. // internal IndexedSelectQueryOperator(IEnumerable child, Func selector) :base(child) { Contract.Assert(child != null, "child data source cannot be null"); Contract.Assert(selector != null, "need a selector function"); m_selector = selector; // In an indexed Select, elements must be returned in the order in which // indices were assigned. m_outputOrdered = true; InitOrdinalIndexState(); } private void InitOrdinalIndexState() { OrdinalIndexState indexState = Child.OrdinalIndexState; if (ExchangeUtilities.IsWorseThan(Child.OrdinalIndexState, OrdinalIndexState.Correct)) { m_prematureMerge = true; indexState = OrdinalIndexState.Correct; } Contract.Assert(!ExchangeUtilities.IsWorseThan(indexState, OrdinalIndexState.Correct)); SetOrdinalIndexState(indexState); } //--------------------------------------------------------------------------------------- // Just opens the current operator, including opening the child and wrapping it with // partitions as needed. // internal override QueryResults Open( QuerySettings settings, bool preferStriping) { QueryResults childQueryResults = Child.Open(settings, preferStriping); return IndexedSelectQueryOperatorResults.NewResults(childQueryResults, this, settings, preferStriping); } internal override void WrapPartitionedStream ( PartitionedStream inputStream, IPartitionedStreamRecipient recipient, bool preferStriping, QuerySettings settings) { int partitionCount = inputStream.PartitionCount; // If the index is not correct, we need to reindex. PartitionedStream inputStreamInt; if (m_prematureMerge) { ListQueryResults listResults = QueryOperator .ExecuteAndCollectResults( inputStream, partitionCount, Child.OutputOrdered, preferStriping, settings); inputStreamInt = listResults.GetPartitionedStream(); } else { Contract.Assert(typeof(TKey) == typeof(int)); inputStreamInt = (PartitionedStream )(object)inputStream; } // Since the index is correct, the type of the index must be int PartitionedStream outputStream = new PartitionedStream (partitionCount, Util.GetDefaultComparer (), OrdinalIndexState); for (int i = 0; i < partitionCount; i++) { outputStream[i] = new IndexedSelectQueryOperatorEnumerator(inputStreamInt[i], m_selector); } recipient.Receive(outputStream); } //--------------------------------------------------------------------------------------- // Whether this operator performs a premature merge. // internal override bool LimitsParallelism { get { return m_prematureMerge; } } //--------------------------------------------------------------------------------------- // The enumerator type responsible for projecting elements as it is walked. // class IndexedSelectQueryOperatorEnumerator : QueryOperatorEnumerator { private readonly QueryOperatorEnumerator m_source; // The data source to enumerate. private readonly Func m_selector; // The actual select function. //---------------------------------------------------------------------------------------- // Instantiates a new select enumerator. // internal IndexedSelectQueryOperatorEnumerator(QueryOperatorEnumerator source, Func selector) { Contract.Assert(source != null); Contract.Assert(selector != null); m_source = source; m_selector = selector; } //--------------------------------------------------------------------------------------- // Straightforward IEnumerator methods. // internal override bool MoveNext(ref TOutput currentElement, ref int currentKey) { // So long as the source has a next element, we have an element. TInput element = default(TInput); if (m_source.MoveNext(ref element, ref currentKey)) { Contract.Assert(m_selector != null, "expected a compiled selection function"); currentElement = m_selector(element, currentKey); return true; } return false; } protected override void Dispose(bool disposing) { m_source.Dispose(); } } //---------------------------------------------------------------------------------------- // Returns an enumerable that represents the query executing sequentially. // internal override IEnumerable AsSequentialQuery(CancellationToken token) { return Child.AsSequentialQuery(token).Select(m_selector); } //------------------------------------------------------------------------------------ // Query results for an indexed Select operator. The results are indexible if the child // results were indexible. // class IndexedSelectQueryOperatorResults : UnaryQueryOperatorResults { IndexedSelectQueryOperator m_selectOp; // Operator that generated the results int m_childCount; // The number of elements in child results public static QueryResults NewResults( QueryResults childQueryResults, IndexedSelectQueryOperator op, QuerySettings settings, bool preferStriping) { if (childQueryResults.IsIndexible) { return new IndexedSelectQueryOperatorResults( childQueryResults, op, settings, preferStriping); } else { return new UnaryQueryOperatorResults( childQueryResults, op, settings, preferStriping); } } private IndexedSelectQueryOperatorResults( QueryResults childQueryResults, IndexedSelectQueryOperator op, QuerySettings settings, bool preferStriping) : base(childQueryResults, op, settings, preferStriping) { m_selectOp = op; Contract.Assert(m_childQueryResults.IsIndexible); m_childCount = m_childQueryResults.ElementsCount; } internal override int ElementsCount { get { Contract.Assert(m_childCount >= 0); return m_childQueryResults.ElementsCount; } } internal override bool IsIndexible { get { return true; } } internal override TOutput GetElement(int index) { return m_selectOp.m_selector(m_childQueryResults.GetElement(index), index); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
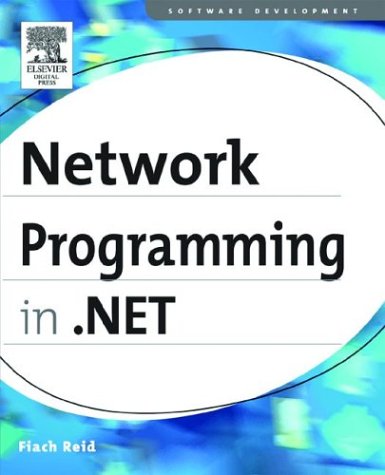
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataListItemEventArgs.cs
- ToggleButton.cs
- SizeLimitedCache.cs
- StreamGeometry.cs
- AlignmentYValidation.cs
- CodeExporter.cs
- CornerRadius.cs
- ContextProperty.cs
- CurrentChangingEventManager.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- HWStack.cs
- TransactionFormatter.cs
- SqlConnectionFactory.cs
- PageRouteHandler.cs
- xdrvalidator.cs
- TextParagraphView.cs
- StringComparer.cs
- SiteMapDataSourceView.cs
- FormatterConverter.cs
- DynamicFilterExpression.cs
- CompiledAction.cs
- PolicyLevel.cs
- EventProperty.cs
- LinearGradientBrush.cs
- TemplateColumn.cs
- DesignerHelpers.cs
- SqlTrackingWorkflowInstance.cs
- SqlProviderServices.cs
- WindowsFormsLinkLabel.cs
- RegexWriter.cs
- TdsParserStaticMethods.cs
- BoundColumn.cs
- PocoPropertyAccessorStrategy.cs
- MatrixTransform3D.cs
- LogLogRecordHeader.cs
- DiscoveryClientDocuments.cs
- RotateTransform.cs
- CommonRemoteMemoryBlock.cs
- OleAutBinder.cs
- RowParagraph.cs
- BrushMappingModeValidation.cs
- KeyboardNavigation.cs
- UInt32Converter.cs
- CodeCatchClause.cs
- GridViewRowPresenter.cs
- SecurityManager.cs
- ExtensionQuery.cs
- rsa.cs
- GroupedContextMenuStrip.cs
- TreeWalkHelper.cs
- DynamicActivityProperty.cs
- CodeDOMUtility.cs
- HttpStreamXmlDictionaryReader.cs
- SizeConverter.cs
- ApplyImportsAction.cs
- TrackingConditionCollection.cs
- EventProxy.cs
- InkCanvasSelection.cs
- Ray3DHitTestResult.cs
- HttpResponseHeader.cs
- CLSCompliantAttribute.cs
- FragmentNavigationEventArgs.cs
- StatusStrip.cs
- Literal.cs
- CodeGeneratorOptions.cs
- TextAction.cs
- CompatibleComparer.cs
- PointKeyFrameCollection.cs
- LinearKeyFrames.cs
- DateTimePicker.cs
- SubpageParagraph.cs
- FlagsAttribute.cs
- Metadata.cs
- OutputCacheProviderCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- SerializationObjectManager.cs
- TraceContextEventArgs.cs
- Empty.cs
- PartialTrustHelpers.cs
- TemplateNameScope.cs
- IDispatchConstantAttribute.cs
- TextBoxDesigner.cs
- Function.cs
- ExceptQueryOperator.cs
- GifBitmapDecoder.cs
- IItemContainerGenerator.cs
- cookie.cs
- SimpleRecyclingCache.cs
- Registry.cs
- HwndStylusInputProvider.cs
- SegmentInfo.cs
- UnsafeNativeMethods.cs
- PieceNameHelper.cs
- ColorConvertedBitmap.cs
- RawStylusInputReport.cs
- CodeDirectiveCollection.cs
- OdbcStatementHandle.cs
- PlatformNotSupportedException.cs
- FileDialog_Vista_Interop.cs
- FieldToken.cs