Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Stylus / RawStylusInputReport.cs / 1 / RawStylusInputReport.cs
using System; using System.ComponentModel; using System.Windows; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Input.StylusPlugIns; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; // SecurityHelper using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// ////// The RawStylusInputReport class encapsulates the raw input provided /// from a stylus. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawStylusInputReport : InputReport { ///////////////////////////////////////////////////////////////////// ////// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The PenContext. /// /// /// The set of actions being reported. /// /// /// Tablet device id. /// /// /// Stylus device id. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, PenContext penContext, RawStylusActions actions, int tabletDeviceId, int stylusDeviceId, int[] data) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (data == null && actions != RawStylusActions.InRange) { throw new ArgumentNullException("data"); } _penContext = new SecurityCriticalDataClass(penContext); _actions = actions; _tabletDeviceId = tabletDeviceId; _stylusDeviceId = stylusDeviceId; _data = data; _isSynchronize = false; } ///////////////////////////////////////////////////////////////////// /// /// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The set of actions being reported. /// /// /// Raw stylus data StylusPointDescription. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, RawStylusActions actions, StylusPointDescription mousePointDescription, int[] mouseData) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (mouseData == null) { throw new ArgumentNullException("mouseData"); } _penContext = new SecurityCriticalDataClass(null); _actions = actions; _tabletDeviceId = 0; _stylusDeviceId = 0; _data = mouseData; _isSynchronize = false; _mousePointDescription = mousePointDescription; _isMouseInput = true; } ///////////////////////////////////////////////////////////////////// /// /// Read-only access to the set of actions that were reported. /// internal RawStylusActions Actions { get { return _actions; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// internal int TabletDeviceId { get { return _tabletDeviceId; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: provides access to critical member _penContext /// internal PenContext PenContext { [SecurityCritical] get { return _penContext.Value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: This handles critical data _penContext /// TreatAsSafe: We're returning safe data /// internal StylusPointDescription StylusPointDescription { [SecurityCritical, SecurityTreatAsSafe] get { return _isMouseInput ? _mousePointDescription : _penContext.Value.StylusPointDescription; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus device id that reported the data. /// internal int StylusDeviceId { get { return _stylusDeviceId; } } ///////////////////////////////////////////////////////////////////// internal StylusDevice StylusDevice { get { return _stylusDevice; } set { _stylusDevice = value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the raw data that was reported. /// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to a clone. /// [SecurityCritical, SecurityTreatAsSafe] internal int[] GetRawPacketData() { if (_data == null) return null; return (int[])_data.Clone(); } ///////////////////////////////////////////////////////////////////// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to return the last tablet point. /// [SecurityCritical, SecurityTreatAsSafe] internal Point GetLastTabletPoint() { int packetLength = StylusPointDescription.GetInputArrayLengthPerPoint(); int lastXIndex = _data.Length - packetLength; return new Point(_data[lastXIndex], _data[lastXIndex + 1]); } ///////////////////////////////////////////////////////////////////// ////// Critical - Hands out ref to internal data that can be used to spoof input. /// internal int[] Data { [SecurityCritical] get { return _data; } } ///////////////////////////////////////////////////////////////////// ////// Critical - Setting property can be used to spoof input. /// internal RawStylusInput RawStylusInput { get { return _rawStylusInput.Value; } [SecurityCritical] set { _rawStylusInput.Value = value; } } ///////////////////////////////////////////////////////////////////// internal bool Synchronized { get { return _isSynchronize; } set { _isSynchronize = value; } } ///////////////////////////////////////////////////////////////////// RawStylusActions _actions; int _tabletDeviceId; ////// Critical: Marked critical to prevent inadvertant spread to transparent code /// private SecurityCriticalDataClass_penContext; int _stylusDeviceId; /// /// Critical to prevent accidental spread to transparent code /// [SecurityCritical] int[] _data; StylusDevice _stylusDevice; // cached value looked up from _stylusDeviceId SecurityCriticalDataForSet_rawStylusInput; bool _isSynchronize; // Set from StylusDevice.Synchronize. bool _isMouseInput; // Special case of mouse input being sent to plugins StylusPointDescription _mousePointDescription; // layout for mouse points } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.ComponentModel; using System.Windows; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Input.StylusPlugIns; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationCore; // SecurityHelper using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Input { ///////////////////////////////////////////////////////////////////////// /// /// The RawStylusInputReport class encapsulates the raw input provided /// from a stylus. /// ////// It is important to note that the InputReport class only contains /// blittable types. This is required so that the report can be /// marshalled across application domains. /// internal class RawStylusInputReport : InputReport { ///////////////////////////////////////////////////////////////////// ////// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The PenContext. /// /// /// The set of actions being reported. /// /// /// Tablet device id. /// /// /// Stylus device id. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, PenContext penContext, RawStylusActions actions, int tabletDeviceId, int stylusDeviceId, int[] data) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (data == null && actions != RawStylusActions.InRange) { throw new ArgumentNullException("data"); } _penContext = new SecurityCriticalDataClass(penContext); _actions = actions; _tabletDeviceId = tabletDeviceId; _stylusDeviceId = stylusDeviceId; _data = data; _isSynchronize = false; } ///////////////////////////////////////////////////////////////////// /// /// Constructs an instance of the RawStylusInputReport class. /// /// /// The mode in which the input is being provided. /// /// /// The time when the input occured. /// /// /// The PresentationSource over which the stylus moved. /// /// /// The set of actions being reported. /// /// /// Raw stylus data StylusPointDescription. /// /// /// Raw stylus data. /// ////// Critical: This handles critical data in the form of PresentationSource. /// TreatAsSafe:There are demands on the critical data(PresentationSource) /// [SecurityCritical,SecurityTreatAsSafe] internal RawStylusInputReport( InputMode mode, int timestamp, PresentationSource inputSource, RawStylusActions actions, StylusPointDescription mousePointDescription, int[] mouseData) : base(inputSource, InputType.Stylus, mode, timestamp) { // Validate parameters if (!RawStylusActionsHelper.IsValid(actions)) { throw new InvalidEnumArgumentException(SR.Get(SRID.Enum_Invalid, "actions")); } if (mouseData == null) { throw new ArgumentNullException("mouseData"); } _penContext = new SecurityCriticalDataClass(null); _actions = actions; _tabletDeviceId = 0; _stylusDeviceId = 0; _data = mouseData; _isSynchronize = false; _mousePointDescription = mousePointDescription; _isMouseInput = true; } ///////////////////////////////////////////////////////////////////// /// /// Read-only access to the set of actions that were reported. /// internal RawStylusActions Actions { get { return _actions; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// internal int TabletDeviceId { get { return _tabletDeviceId; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: provides access to critical member _penContext /// internal PenContext PenContext { [SecurityCritical] get { return _penContext.Value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus context id that reported the data. /// ////// Critical: This handles critical data _penContext /// TreatAsSafe: We're returning safe data /// internal StylusPointDescription StylusPointDescription { [SecurityCritical, SecurityTreatAsSafe] get { return _isMouseInput ? _mousePointDescription : _penContext.Value.StylusPointDescription; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to stylus device id that reported the data. /// internal int StylusDeviceId { get { return _stylusDeviceId; } } ///////////////////////////////////////////////////////////////////// internal StylusDevice StylusDevice { get { return _stylusDevice; } set { _stylusDevice = value; } } ///////////////////////////////////////////////////////////////////// ////// Read-only access to the raw data that was reported. /// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to a clone. /// [SecurityCritical, SecurityTreatAsSafe] internal int[] GetRawPacketData() { if (_data == null) return null; return (int[])_data.Clone(); } ///////////////////////////////////////////////////////////////////// ////// Critical: Access the critical field - _data /// TreatAsSafe: No input is taken. It's safe to return the last tablet point. /// [SecurityCritical, SecurityTreatAsSafe] internal Point GetLastTabletPoint() { int packetLength = StylusPointDescription.GetInputArrayLengthPerPoint(); int lastXIndex = _data.Length - packetLength; return new Point(_data[lastXIndex], _data[lastXIndex + 1]); } ///////////////////////////////////////////////////////////////////// ////// Critical - Hands out ref to internal data that can be used to spoof input. /// internal int[] Data { [SecurityCritical] get { return _data; } } ///////////////////////////////////////////////////////////////////// ////// Critical - Setting property can be used to spoof input. /// internal RawStylusInput RawStylusInput { get { return _rawStylusInput.Value; } [SecurityCritical] set { _rawStylusInput.Value = value; } } ///////////////////////////////////////////////////////////////////// internal bool Synchronized { get { return _isSynchronize; } set { _isSynchronize = value; } } ///////////////////////////////////////////////////////////////////// RawStylusActions _actions; int _tabletDeviceId; ////// Critical: Marked critical to prevent inadvertant spread to transparent code /// private SecurityCriticalDataClass_penContext; int _stylusDeviceId; /// /// Critical to prevent accidental spread to transparent code /// [SecurityCritical] int[] _data; StylusDevice _stylusDevice; // cached value looked up from _stylusDeviceId SecurityCriticalDataForSet_rawStylusInput; bool _isSynchronize; // Set from StylusDevice.Synchronize. bool _isMouseInput; // Special case of mouse input being sent to plugins StylusPointDescription _mousePointDescription; // layout for mouse points } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
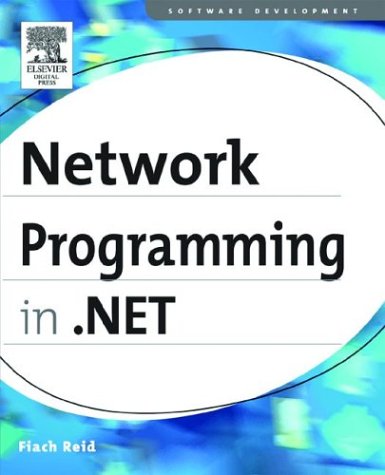
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlEncoding.cs
- TextTreeInsertElementUndoUnit.cs
- AnnotationComponentChooser.cs
- XmlElementAttributes.cs
- dataprotectionpermission.cs
- View.cs
- GenericTypeParameterBuilder.cs
- DbFunctionCommandTree.cs
- QueryStringParameter.cs
- ResourceReader.cs
- CounterCreationData.cs
- Package.cs
- SynchronizationLockException.cs
- ISAPIRuntime.cs
- OutputCacheProviderCollection.cs
- WebPartTransformerCollection.cs
- SoapIgnoreAttribute.cs
- Thumb.cs
- MetadataSerializer.cs
- GetLedgerRequest.cs
- ClassImporter.cs
- UserControl.cs
- ViewStateException.cs
- DefinitionBase.cs
- WSHttpSecurity.cs
- CodeArrayCreateExpression.cs
- WindowsListViewGroupSubsetLink.cs
- TakeOrSkipWhileQueryOperator.cs
- LogWriteRestartAreaState.cs
- BridgeDataRecord.cs
- XmlHierarchicalEnumerable.cs
- AtlasWeb.Designer.cs
- RootContext.cs
- MsmqUri.cs
- HandleCollector.cs
- DisplayInformation.cs
- HuffCodec.cs
- DoubleCollection.cs
- RegexCapture.cs
- OleDbReferenceCollection.cs
- JumpItem.cs
- safelink.cs
- ListViewGroupItemCollection.cs
- WSTransactionSection.cs
- MailMessageEventArgs.cs
- ScrollViewer.cs
- VideoDrawing.cs
- ToolStripDropDownClosedEventArgs.cs
- DataGridViewCell.cs
- CodeDelegateInvokeExpression.cs
- CommunicationObjectManager.cs
- Walker.cs
- PaperSource.cs
- ImageIndexEditor.cs
- RootBrowserWindowProxy.cs
- GridViewSelectEventArgs.cs
- unsafenativemethodsother.cs
- Icon.cs
- EntityDataSourceEntityTypeFilterConverter.cs
- BooleanKeyFrameCollection.cs
- TypeConstant.cs
- ScrollBarRenderer.cs
- ContentType.cs
- RunWorkerCompletedEventArgs.cs
- RegistrySecurity.cs
- HostedHttpRequestAsyncResult.cs
- ProfileProvider.cs
- ButtonChrome.cs
- ISO2022Encoding.cs
- ServiceBehaviorElementCollection.cs
- externdll.cs
- UITypeEditor.cs
- SQLInt64Storage.cs
- ChtmlPageAdapter.cs
- SoapAttributeAttribute.cs
- RowBinding.cs
- TextServicesManager.cs
- OracleDateTime.cs
- HtmlControl.cs
- FlowDocumentPaginator.cs
- XamlToRtfParser.cs
- AnnotationAuthorChangedEventArgs.cs
- CollectionViewGroupRoot.cs
- DataObjectCopyingEventArgs.cs
- OleDbFactory.cs
- WindowsPrincipal.cs
- XslCompiledTransform.cs
- HtmlForm.cs
- MembershipUser.cs
- WCFServiceClientProxyGenerator.cs
- FactoryGenerator.cs
- securitycriticaldata.cs
- WeakReferenceEnumerator.cs
- _PooledStream.cs
- StylusPlugin.cs
- SafeEventLogReadHandle.cs
- Symbol.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- HtmlWindowCollection.cs
- EmptyStringExpandableObjectConverter.cs