Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / Primitives / IItemContainerGenerator.cs / 2 / IItemContainerGenerator.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: IItemContainerGenerator interface // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using MS.Internal.Data; namespace System.Windows.Controls.Primitives { ////// Interface through which a layout element (such as a panel) marked /// as an ItemsHost communicates with the ItemContainerGenerator of its /// items owner. /// public interface IItemContainerGenerator { ////// Return the ItemContainerGenerator appropriate for use by the given panel /// ItemContainerGenerator GetItemContainerGeneratorForPanel(Panel panel); ///Prepare the generator to generate, starting at the given position and direction ////// This method must be called before calling GenerateNext. It returns an /// IDisposable object that tracks the lifetime of the generation loop. /// This method sets the generator's status to GeneratingContent; when /// the IDisposable is disposed, the status changes to ContentReady or /// Error, as appropriate. /// IDisposable StartAt(GeneratorPosition position, GeneratorDirection direction); ///Prepare the generator to generate, starting at the given position and direction ////// This method must be called before calling GenerateNext. It returns an /// IDisposable object that tracks the lifetime of the generation loop. /// This method sets the generator's status to GeneratingContent; when /// the IDisposable is disposed, the status changes to ContentReady or /// Error, as appropriate. /// IDisposable StartAt(GeneratorPosition position, GeneratorDirection direction, bool allowStartAtRealizedItem); ///Return the container element used to display the next item. ////// This method must be called in the scope of the IDisposable returned by /// a previous call to StartAt. /// DependencyObject GenerateNext(); ///Return the container element used to display the next item. /// When the next item has not been realized, this method returns a container /// and sets isNewlyRealized to true. When the next item has been realized, /// this method returns the exisiting container and sets isNewlyRealized to /// false. /// ////// This method must be called in the scope of the IDisposable returned by /// a previous call to StartAt. /// DependencyObject GenerateNext(out bool isNewlyRealized); ////// Prepare the given element to act as the ItemUI for the /// corresponding item. This includes applying the ItemUI style, /// forwarding information from the host control (ItemTemplate, etc.), /// and other small adjustments. /// ////// This method must be called after the element has been added to the /// visual tree, so that resource references and inherited properties /// work correctly. /// /// The container to prepare. /// Normally this is the result of the previous call to GenerateNext. /// void PrepareItemContainer(DependencyObject container); ////// Remove all generated elements. /// void RemoveAll(); ////// Remove generated elements. /// ////// The position must refer to a previously generated item, i.e. its /// Offset must be 0. /// void Remove(GeneratorPosition position, int count); ////// Map an index into the items collection to a GeneratorPosition. /// GeneratorPosition GeneratorPositionFromIndex(int itemIndex); ////// Map a GeneratorPosition to an index into the items collection. /// int IndexFromGeneratorPosition(GeneratorPosition position); } ////// A user of the ItemContainerGenerator describes positions using this struct. /// Some examples: /// To start generating forward from the beginning of the item list, /// specify position (-1, 0) and direction Forward. /// To start generating backward from the end of the list, /// specify position (-1, 0) and direction Backward. /// To generate the items after the element with index k, specify /// position (k, 0) and direction Forward. /// public struct GeneratorPosition { ////// Index, with respect to realized elements. The special value -1 /// refers to a fictitious element at the beginning or end of the /// the list. /// public int Index { get { return _index; } set { _index = value; } } ////// Offset, with respect to unrealized items near the indexed element. /// An offset of 0 refers to the indexed element itself, an offset /// of 1 refers to the next (unrealized) item, and an offset of -1 /// refers to the previous item. /// public int Offset { get { return _offset; } set { _offset = value; } } ///Constructor public GeneratorPosition(int index, int offset) { _index = index; _offset = offset; } ///Return a hash code // This is required by FxCop. public override int GetHashCode() { return _index.GetHashCode() + _offset.GetHashCode(); } ///Returns a string representation of the GeneratorPosition public override string ToString() { return string.Concat("GeneratorPosition (", _index.ToString(System.Windows.Markup.TypeConverterHelper.EnglishUSCulture), ",", _offset.ToString(System.Windows.Markup.TypeConverterHelper.EnglishUSCulture), ")"); } // The remaining methods are present only because they are required by FxCop. ///Equality test // This is required by FxCop. public override bool Equals(object o) { if (o is GeneratorPosition) { GeneratorPosition that = (GeneratorPosition)o; return this._index == that._index && this._offset == that._offset; } return false; } ///Equality test // This is required by FxCop. public static bool operator==(GeneratorPosition gp1, GeneratorPosition gp2) { return gp1._index == gp2._index && gp1._offset == gp2._offset; } ///Inequality test // This is required by FxCop. public static bool operator!=(GeneratorPosition gp1, GeneratorPosition gp2) { return !(gp1 == gp2); } private int _index; private int _offset; } ////// This enum is used by the ItemContainerGenerator and its client to specify /// the direction in which the generator produces UI. /// public enum GeneratorDirection { ///generate forward through the item collection Forward, ///generate backward through the item collection Backward } ////// This enum is used by the ItemContainerGenerator to indicate its status. /// public enum GeneratorStatus { ///The generator has not tried to generate content NotStarted, ///The generator is generating containers GeneratingContainers, ///The generator has finished generating containers ContainersGenerated, ///The generator has finished generating containers, but encountered one or more errors Error } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: IItemContainerGenerator interface // // Specs: http://avalon/connecteddata/M5%20General%20Docs/Data%20Styling.mht // //--------------------------------------------------------------------------- using System; using MS.Internal.Data; namespace System.Windows.Controls.Primitives { ////// Interface through which a layout element (such as a panel) marked /// as an ItemsHost communicates with the ItemContainerGenerator of its /// items owner. /// public interface IItemContainerGenerator { ////// Return the ItemContainerGenerator appropriate for use by the given panel /// ItemContainerGenerator GetItemContainerGeneratorForPanel(Panel panel); ///Prepare the generator to generate, starting at the given position and direction ////// This method must be called before calling GenerateNext. It returns an /// IDisposable object that tracks the lifetime of the generation loop. /// This method sets the generator's status to GeneratingContent; when /// the IDisposable is disposed, the status changes to ContentReady or /// Error, as appropriate. /// IDisposable StartAt(GeneratorPosition position, GeneratorDirection direction); ///Prepare the generator to generate, starting at the given position and direction ////// This method must be called before calling GenerateNext. It returns an /// IDisposable object that tracks the lifetime of the generation loop. /// This method sets the generator's status to GeneratingContent; when /// the IDisposable is disposed, the status changes to ContentReady or /// Error, as appropriate. /// IDisposable StartAt(GeneratorPosition position, GeneratorDirection direction, bool allowStartAtRealizedItem); ///Return the container element used to display the next item. ////// This method must be called in the scope of the IDisposable returned by /// a previous call to StartAt. /// DependencyObject GenerateNext(); ///Return the container element used to display the next item. /// When the next item has not been realized, this method returns a container /// and sets isNewlyRealized to true. When the next item has been realized, /// this method returns the exisiting container and sets isNewlyRealized to /// false. /// ////// This method must be called in the scope of the IDisposable returned by /// a previous call to StartAt. /// DependencyObject GenerateNext(out bool isNewlyRealized); ////// Prepare the given element to act as the ItemUI for the /// corresponding item. This includes applying the ItemUI style, /// forwarding information from the host control (ItemTemplate, etc.), /// and other small adjustments. /// ////// This method must be called after the element has been added to the /// visual tree, so that resource references and inherited properties /// work correctly. /// /// The container to prepare. /// Normally this is the result of the previous call to GenerateNext. /// void PrepareItemContainer(DependencyObject container); ////// Remove all generated elements. /// void RemoveAll(); ////// Remove generated elements. /// ////// The position must refer to a previously generated item, i.e. its /// Offset must be 0. /// void Remove(GeneratorPosition position, int count); ////// Map an index into the items collection to a GeneratorPosition. /// GeneratorPosition GeneratorPositionFromIndex(int itemIndex); ////// Map a GeneratorPosition to an index into the items collection. /// int IndexFromGeneratorPosition(GeneratorPosition position); } ////// A user of the ItemContainerGenerator describes positions using this struct. /// Some examples: /// To start generating forward from the beginning of the item list, /// specify position (-1, 0) and direction Forward. /// To start generating backward from the end of the list, /// specify position (-1, 0) and direction Backward. /// To generate the items after the element with index k, specify /// position (k, 0) and direction Forward. /// public struct GeneratorPosition { ////// Index, with respect to realized elements. The special value -1 /// refers to a fictitious element at the beginning or end of the /// the list. /// public int Index { get { return _index; } set { _index = value; } } ////// Offset, with respect to unrealized items near the indexed element. /// An offset of 0 refers to the indexed element itself, an offset /// of 1 refers to the next (unrealized) item, and an offset of -1 /// refers to the previous item. /// public int Offset { get { return _offset; } set { _offset = value; } } ///Constructor public GeneratorPosition(int index, int offset) { _index = index; _offset = offset; } ///Return a hash code // This is required by FxCop. public override int GetHashCode() { return _index.GetHashCode() + _offset.GetHashCode(); } ///Returns a string representation of the GeneratorPosition public override string ToString() { return string.Concat("GeneratorPosition (", _index.ToString(System.Windows.Markup.TypeConverterHelper.EnglishUSCulture), ",", _offset.ToString(System.Windows.Markup.TypeConverterHelper.EnglishUSCulture), ")"); } // The remaining methods are present only because they are required by FxCop. ///Equality test // This is required by FxCop. public override bool Equals(object o) { if (o is GeneratorPosition) { GeneratorPosition that = (GeneratorPosition)o; return this._index == that._index && this._offset == that._offset; } return false; } ///Equality test // This is required by FxCop. public static bool operator==(GeneratorPosition gp1, GeneratorPosition gp2) { return gp1._index == gp2._index && gp1._offset == gp2._offset; } ///Inequality test // This is required by FxCop. public static bool operator!=(GeneratorPosition gp1, GeneratorPosition gp2) { return !(gp1 == gp2); } private int _index; private int _offset; } ////// This enum is used by the ItemContainerGenerator and its client to specify /// the direction in which the generator produces UI. /// public enum GeneratorDirection { ///generate forward through the item collection Forward, ///generate backward through the item collection Backward } ////// This enum is used by the ItemContainerGenerator to indicate its status. /// public enum GeneratorStatus { ///The generator has not tried to generate content NotStarted, ///The generator is generating containers GeneratingContainers, ///The generator has finished generating containers ContainersGenerated, ///The generator has finished generating containers, but encountered one or more errors Error } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
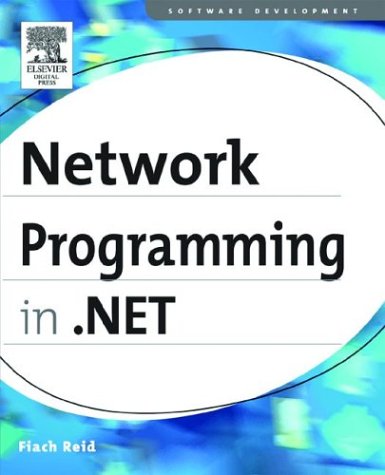
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RoleManagerSection.cs
- BitmapEffectState.cs
- TouchesOverProperty.cs
- KnowledgeBase.cs
- ProxyFragment.cs
- DataGridViewCell.cs
- AccessText.cs
- CustomAttributeFormatException.cs
- WebBrowserDocumentCompletedEventHandler.cs
- uribuilder.cs
- LocatorBase.cs
- HttpProfileBase.cs
- ComponentManagerBroker.cs
- ComponentResourceManager.cs
- SchemaImporterExtensionElement.cs
- ResXResourceSet.cs
- StringFreezingAttribute.cs
- SchemaDeclBase.cs
- OledbConnectionStringbuilder.cs
- ValidationRule.cs
- MethodExpression.cs
- CollectionChangedEventManager.cs
- ColorDialog.cs
- SplitterEvent.cs
- DbModificationClause.cs
- SQLByteStorage.cs
- QueryCacheKey.cs
- HttpListenerContext.cs
- SolidBrush.cs
- InfoCardSchemas.cs
- XD.cs
- SafeProcessHandle.cs
- UInt32.cs
- StreamWithDictionary.cs
- IdleTimeoutMonitor.cs
- SqlGatherProducedAliases.cs
- ConfigurationManagerHelper.cs
- MaskedTextBox.cs
- XmlSchemaComplexContent.cs
- DataObject.cs
- MenuItemBinding.cs
- LinkedResource.cs
- HttpException.cs
- ArcSegment.cs
- DockPattern.cs
- DSACryptoServiceProvider.cs
- DbSetClause.cs
- ParallelTimeline.cs
- CharEnumerator.cs
- MonikerHelper.cs
- SoundPlayerAction.cs
- Panel.cs
- PackageProperties.cs
- BitmapFrame.cs
- Msec.cs
- XPathConvert.cs
- PanelDesigner.cs
- TraceLog.cs
- BorderSidesEditor.cs
- CommonDialog.cs
- SlotInfo.cs
- TreeWalkHelper.cs
- CatalogPartChrome.cs
- DataGridViewCellStateChangedEventArgs.cs
- DbDataSourceEnumerator.cs
- DropTarget.cs
- ConnectorDragDropGlyph.cs
- PageClientProxyGenerator.cs
- GlyphTypeface.cs
- SystemException.cs
- SubstitutionResponseElement.cs
- SystemIPInterfaceProperties.cs
- SafeBitVector32.cs
- InputChannel.cs
- RenderDataDrawingContext.cs
- ListenerConnectionDemuxer.cs
- UnknownWrapper.cs
- CopyAction.cs
- TrackingWorkflowEventArgs.cs
- Package.cs
- MD5.cs
- ApplicationInterop.cs
- DocumentApplication.cs
- WindowsFormsLinkLabel.cs
- OdbcHandle.cs
- RectAnimationBase.cs
- DateTimeFormat.cs
- XmlNamespaceMappingCollection.cs
- DetailsView.cs
- CustomAttribute.cs
- ComponentCodeDomSerializer.cs
- ViewCellSlot.cs
- PrivacyNoticeBindingElementImporter.cs
- ReliabilityContractAttribute.cs
- ThreadLocal.cs
- WebSysDisplayNameAttribute.cs
- EntityAdapter.cs
- ListViewItemEventArgs.cs
- RNGCryptoServiceProvider.cs
- CompensatableTransactionScopeActivity.cs