Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Markup / Primitives / MarkupProperty.cs / 1305600 / MarkupProperty.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: MarkupProperty.cs // // Contents: API for iterating a tree of objects for serialization // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- using System; using System.ComponentModel; using System.Collections.Generic; using System.Collections; using System.Reflection; using System.Text; using System.Windows; using MS.Internal.WindowsBase; namespace System.Windows.Markup.Primitives { ////// A property description used by serialiation to encapsulate access to properties and their values. A property is /// either representable as a string or a list of items. If the property can be respresented as a string /// IsComposite is false, otherwise, if IsComposite is true, the property is a list of items. /// public abstract class MarkupProperty { ////// Prevent external specialization /// [FriendAccessAllowed] // Used by MarkupPropertyWrapper internal MarkupProperty() { } ////// A name sutible for diagnostics and error reporting. A serializer should not use this value. It should use /// the PropertyDescriptor and/or DependencyProperty instead. /// public abstract string Name { get; } ////// The type of the property. /// public abstract Type PropertyType { get; } ////// whether the property type is a collection or not /// internal bool IsCollectionProperty { get { Type propertyType = PropertyType; return (typeof(IList).IsAssignableFrom(propertyType) || typeof(IDictionary).IsAssignableFrom(propertyType) || propertyType.IsArray); } } ////// The property descriptor for the markup property if this property is associated with a CLR property. /// public virtual PropertyDescriptor PropertyDescriptor { get { return null; } } ////// The dependency property for the markup property if this property is backed by a DependencyProperty. /// public virtual DependencyProperty DependencyProperty { get { return null; } } ////// Returns true when this propety is an attached dependency property. When true, PropertyDescriptor /// can be null (but is not required to be) and DependencyProperty is not null. /// public virtual bool IsAttached { get { return false; } } ////// Returns true when this property represents a constructor argument. When true, PropertyDescriptor and /// DependencyProperty are both null. XAML only uses this for represnting the constructor parameters of /// MarkupExtension instances. /// public virtual bool IsConstructorArgument { get { return false; } } ////// Returns true when this property represents the text to pass to the type's ValueConverter to create an /// instance instead of using a constructor. When true, PropertyDescriptor and DependencyProperty are both /// null. If a property is provided through MarkupItem.Properties that has this value set to true it is the /// only property the type will provide. /// public virtual bool IsValueAsString { get { return false; } } ////// Returns true when this property represents direct content of a collection (or dictionary) class. When true, /// PropertyDescriptor and DependencyProperty are both null. /// public virtual bool IsContent { get { return false; } } ////// Returns true when the property represents the key used by the MarkupItem's container to store the item in a /// dictionary. When true, the PropertyDescriptor and DependencyProperty will return null. XAML will emit this /// as an x:Key attribute. /// public virtual bool IsKey { get { return false; } } ////// If IsComposite is false Value and StringValue are valid to call, the property can be represented as a /// string. If is true, Items is valid to use and the property is one or more items. If the property is /// composite but has not items, the property isn't returned by MarkupItem.GetProperties. /// public virtual bool IsComposite { get { return false; } } ////// The current value of the property. /// public abstract object Value { get; } ////// The string value of the property using ValueSerializer classes when appropriate to convert the value to a /// string. Which ValueSerializer to invoke is determined by the IValueSerializerContext of the MarkupItem that /// returned this MarkupProperty. /// public abstract string StringValue { get; } ////// The list of types that this property will reference when it serializes its value as a string. This allows /// a serializer to ensure that the de-serializer has enough information to convert references to these type /// from the string representations. For example, ensuring there is an xmlns declaration for the XML namespace /// that defines the type. /// public abstract IEnumerableTypeReferences { get; } /// /// Enumerate the items that make up the value of this property. If the property is not an enumeration, this /// will only be one item in the enumeration. If the property is an enumeration (or enumerable) then all the /// items will be returned. At least one will always be returned since it MarkupItem will not create a /// MarkupProperty for properties with no items. /// public abstract IEnumerableItems { get; } /// /// The attributes associated with the markup property. /// public abstract AttributeCollection Attributes { get; } ////// Checks to see that each markup object is of a public type. Used in serialization. /// internal virtual void VerifyOnlySerializableTypes() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2005 // // File: MarkupProperty.cs // // Contents: API for iterating a tree of objects for serialization // // Created: 04/28/2005 [....] // //----------------------------------------------------------------------- using System; using System.ComponentModel; using System.Collections.Generic; using System.Collections; using System.Reflection; using System.Text; using System.Windows; using MS.Internal.WindowsBase; namespace System.Windows.Markup.Primitives { ////// A property description used by serialiation to encapsulate access to properties and their values. A property is /// either representable as a string or a list of items. If the property can be respresented as a string /// IsComposite is false, otherwise, if IsComposite is true, the property is a list of items. /// public abstract class MarkupProperty { ////// Prevent external specialization /// [FriendAccessAllowed] // Used by MarkupPropertyWrapper internal MarkupProperty() { } ////// A name sutible for diagnostics and error reporting. A serializer should not use this value. It should use /// the PropertyDescriptor and/or DependencyProperty instead. /// public abstract string Name { get; } ////// The type of the property. /// public abstract Type PropertyType { get; } ////// whether the property type is a collection or not /// internal bool IsCollectionProperty { get { Type propertyType = PropertyType; return (typeof(IList).IsAssignableFrom(propertyType) || typeof(IDictionary).IsAssignableFrom(propertyType) || propertyType.IsArray); } } ////// The property descriptor for the markup property if this property is associated with a CLR property. /// public virtual PropertyDescriptor PropertyDescriptor { get { return null; } } ////// The dependency property for the markup property if this property is backed by a DependencyProperty. /// public virtual DependencyProperty DependencyProperty { get { return null; } } ////// Returns true when this propety is an attached dependency property. When true, PropertyDescriptor /// can be null (but is not required to be) and DependencyProperty is not null. /// public virtual bool IsAttached { get { return false; } } ////// Returns true when this property represents a constructor argument. When true, PropertyDescriptor and /// DependencyProperty are both null. XAML only uses this for represnting the constructor parameters of /// MarkupExtension instances. /// public virtual bool IsConstructorArgument { get { return false; } } ////// Returns true when this property represents the text to pass to the type's ValueConverter to create an /// instance instead of using a constructor. When true, PropertyDescriptor and DependencyProperty are both /// null. If a property is provided through MarkupItem.Properties that has this value set to true it is the /// only property the type will provide. /// public virtual bool IsValueAsString { get { return false; } } ////// Returns true when this property represents direct content of a collection (or dictionary) class. When true, /// PropertyDescriptor and DependencyProperty are both null. /// public virtual bool IsContent { get { return false; } } ////// Returns true when the property represents the key used by the MarkupItem's container to store the item in a /// dictionary. When true, the PropertyDescriptor and DependencyProperty will return null. XAML will emit this /// as an x:Key attribute. /// public virtual bool IsKey { get { return false; } } ////// If IsComposite is false Value and StringValue are valid to call, the property can be represented as a /// string. If is true, Items is valid to use and the property is one or more items. If the property is /// composite but has not items, the property isn't returned by MarkupItem.GetProperties. /// public virtual bool IsComposite { get { return false; } } ////// The current value of the property. /// public abstract object Value { get; } ////// The string value of the property using ValueSerializer classes when appropriate to convert the value to a /// string. Which ValueSerializer to invoke is determined by the IValueSerializerContext of the MarkupItem that /// returned this MarkupProperty. /// public abstract string StringValue { get; } ////// The list of types that this property will reference when it serializes its value as a string. This allows /// a serializer to ensure that the de-serializer has enough information to convert references to these type /// from the string representations. For example, ensuring there is an xmlns declaration for the XML namespace /// that defines the type. /// public abstract IEnumerableTypeReferences { get; } /// /// Enumerate the items that make up the value of this property. If the property is not an enumeration, this /// will only be one item in the enumeration. If the property is an enumeration (or enumerable) then all the /// items will be returned. At least one will always be returned since it MarkupItem will not create a /// MarkupProperty for properties with no items. /// public abstract IEnumerableItems { get; } /// /// The attributes associated with the markup property. /// public abstract AttributeCollection Attributes { get; } ////// Checks to see that each markup object is of a public type. Used in serialization. /// internal virtual void VerifyOnlySerializableTypes() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
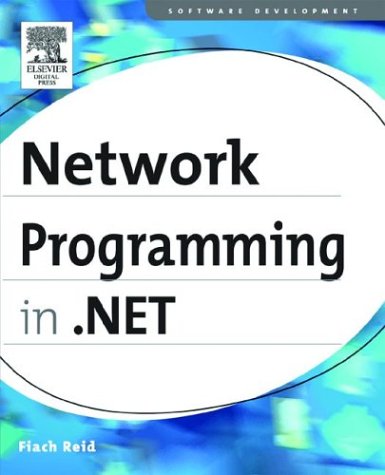
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TimeSpanStorage.cs
- CompilerError.cs
- EventManager.cs
- Command.cs
- InvokeProviderWrapper.cs
- ParserContext.cs
- DependencyObjectValidator.cs
- WeakReadOnlyCollection.cs
- GeometryGroup.cs
- FixedPosition.cs
- Encoder.cs
- CurrencyManager.cs
- ImageIndexConverter.cs
- KeyPullup.cs
- WindowsFormsSynchronizationContext.cs
- NamespaceQuery.cs
- MessageContractExporter.cs
- CodeMemberProperty.cs
- IntSecurity.cs
- ContentWrapperAttribute.cs
- HttpCacheVaryByContentEncodings.cs
- Queue.cs
- AlternationConverter.cs
- PersonalizationState.cs
- IndexedEnumerable.cs
- PeerNameResolver.cs
- UnmanagedMemoryAccessor.cs
- FullTextBreakpoint.cs
- TablePatternIdentifiers.cs
- DefaultValidator.cs
- PropertyDescriptorComparer.cs
- ParamArrayAttribute.cs
- ReversePositionQuery.cs
- HostTimeoutsElement.cs
- UxThemeWrapper.cs
- MouseGesture.cs
- FragmentQuery.cs
- DataObjectPastingEventArgs.cs
- Stroke2.cs
- TempFiles.cs
- ConfigurationException.cs
- StickyNote.cs
- JournalEntry.cs
- XmlSerializerNamespaces.cs
- ExtensionFile.cs
- SqlDelegatedTransaction.cs
- MatrixAnimationBase.cs
- AppDomainCompilerProxy.cs
- TemplateControlCodeDomTreeGenerator.cs
- AtomMaterializer.cs
- InputLanguageSource.cs
- OptimalBreakSession.cs
- ButtonChrome.cs
- ReferenceEqualityComparer.cs
- SQLInt16Storage.cs
- AnnotationAuthorChangedEventArgs.cs
- StateManagedCollection.cs
- UriParserTemplates.cs
- Enlistment.cs
- DocumentCollection.cs
- XPathDocumentBuilder.cs
- TripleDESCryptoServiceProvider.cs
- DetailsViewModeEventArgs.cs
- DateTimePicker.cs
- WorkerProcess.cs
- SequenceNumber.cs
- webclient.cs
- ExpressionEditorSheet.cs
- CellRelation.cs
- PropVariant.cs
- AutoResizedEvent.cs
- MenuItemAutomationPeer.cs
- TableDesigner.cs
- UIElement.cs
- TextBoxView.cs
- TraceInternal.cs
- ContextMarshalException.cs
- TextFormatter.cs
- DataColumn.cs
- ColorAnimationUsingKeyFrames.cs
- SqlDataSourceRefreshSchemaForm.cs
- COM2PropertyDescriptor.cs
- WebBrowserEvent.cs
- EventListenerClientSide.cs
- BamlRecords.cs
- Label.cs
- AccessedThroughPropertyAttribute.cs
- HMACSHA384.cs
- ToolStripSystemRenderer.cs
- CmsInterop.cs
- CommonObjectSecurity.cs
- SpeechUI.cs
- CapacityStreamGeometryContext.cs
- ValidatorUtils.cs
- HtmlControlPersistable.cs
- ProtocolElementCollection.cs
- RepeaterCommandEventArgs.cs
- HttpDictionary.cs
- assemblycache.cs
- TextServicesDisplayAttributePropertyRanges.cs