Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Automation / TransformProviderWrapper.cs / 1305600 / TransformProviderWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Transform pattern provider wrapper for WCP // // History: // 02/04/2004 : preid created // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TransformProviderWrapper: MarshalByRefObject, ITransformProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TransformProviderWrapper( AutomationPeer peer, ITransformProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface IWindowProvider // //----------------------------------------------------- #region Interface ITransformProvider public void Move( double x, double y ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Move ), new double [ ] { x, y } ); } public void Resize( double width, double height ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Resize ), new double [ ] { width, height } ); } public void Rotate( double degrees ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Rotate ), degrees ); } public bool CanMove { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanMove ), null ); } } public bool CanResize { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanResize ), null ); } } public bool CanRotate { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanRotate ), null ); } } #endregion Interface ITransformProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TransformProviderWrapper( peer, (ITransformProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object Move( object arg ) { double [ ] args = (double [ ]) arg; _iface.Move( args[ 0 ], args[ 1 ] ); return null; } private object Resize( object arg ) { double [ ] args = (double [ ]) arg; _iface.Resize( args[ 0 ], args[ 1 ] ); return null; } private object Rotate( object arg ) { _iface.Rotate( (double)arg ); return null; } private object GetCanMove( object unused ) { return _iface.CanMove; } private object GetCanResize( object unused ) { return _iface.CanResize; } private object GetCanRotate( object unused ) { return _iface.CanRotate; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITransformProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Transform pattern provider wrapper for WCP // // History: // 02/04/2004 : preid created // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TransformProviderWrapper: MarshalByRefObject, ITransformProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TransformProviderWrapper( AutomationPeer peer, ITransformProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface IWindowProvider // //----------------------------------------------------- #region Interface ITransformProvider public void Move( double x, double y ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Move ), new double [ ] { x, y } ); } public void Resize( double width, double height ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Resize ), new double [ ] { width, height } ); } public void Rotate( double degrees ) { ElementUtil.Invoke( _peer, new DispatcherOperationCallback( Rotate ), degrees ); } public bool CanMove { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanMove ), null ); } } public bool CanResize { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanResize ), null ); } } public bool CanRotate { get { return (bool) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetCanRotate ), null ); } } #endregion Interface ITransformProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TransformProviderWrapper( peer, (ITransformProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object Move( object arg ) { double [ ] args = (double [ ]) arg; _iface.Move( args[ 0 ], args[ 1 ] ); return null; } private object Resize( object arg ) { double [ ] args = (double [ ]) arg; _iface.Resize( args[ 0 ], args[ 1 ] ); return null; } private object Rotate( object arg ) { _iface.Rotate( (double)arg ); return null; } private object GetCanMove( object unused ) { return _iface.CanMove; } private object GetCanResize( object unused ) { return _iface.CanResize; } private object GetCanRotate( object unused ) { return _iface.CanRotate; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITransformProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
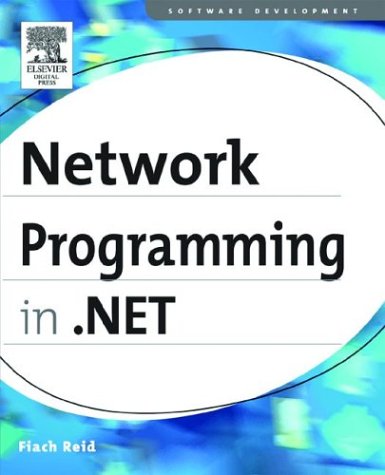
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseButtonEventArgs.cs
- ServiceManagerHandle.cs
- SigningCredentials.cs
- RestHandlerFactory.cs
- ContextProperty.cs
- ImageCodecInfoPrivate.cs
- ArrayConverter.cs
- _FtpDataStream.cs
- EpmCustomContentWriterNodeData.cs
- XmlDataImplementation.cs
- PointConverter.cs
- DataServiceContext.cs
- DataGridViewLayoutData.cs
- SoapClientProtocol.cs
- SessionStateModule.cs
- TextEditorSpelling.cs
- WebResourceAttribute.cs
- TdsParameterSetter.cs
- CheckableControlBaseAdapter.cs
- DataBoundControlHelper.cs
- DataGridTableStyleMappingNameEditor.cs
- Validator.cs
- TextEmbeddedObject.cs
- ColumnHeaderCollectionEditor.cs
- OperationContractAttribute.cs
- List.cs
- CacheEntry.cs
- KnownAssembliesSet.cs
- XmlSchemaImport.cs
- MenuItem.cs
- TextDecorationCollectionConverter.cs
- WebResourceUtil.cs
- EventPrivateKey.cs
- ImageMap.cs
- NetworkInformationException.cs
- DefaultProxySection.cs
- uribuilder.cs
- DragStartedEventArgs.cs
- SystemFonts.cs
- MultipartIdentifier.cs
- ErrorProvider.cs
- ControlDesigner.cs
- NetMsmqSecurityMode.cs
- LogStore.cs
- BindingNavigator.cs
- DateBoldEvent.cs
- BCryptHashAlgorithm.cs
- RadialGradientBrush.cs
- DocumentPage.cs
- BaseDataBoundControlDesigner.cs
- EntityTransaction.cs
- FrameAutomationPeer.cs
- ValidatedControlConverter.cs
- SchemaHelper.cs
- DynamicValueConverter.cs
- PeerContact.cs
- StyleHelper.cs
- ErrorReporting.cs
- TextTreeFixupNode.cs
- MonthCalendar.cs
- TagElement.cs
- RelativeSource.cs
- CodeSubDirectory.cs
- Tokenizer.cs
- QilTypeChecker.cs
- WebDisplayNameAttribute.cs
- XmlNodeWriter.cs
- COM2EnumConverter.cs
- KerberosTokenFactoryCredential.cs
- RunInstallerAttribute.cs
- HitTestFilterBehavior.cs
- StrokeNodeOperations2.cs
- EntityDataSourceState.cs
- ArithmeticException.cs
- CheckBoxList.cs
- JsonByteArrayDataContract.cs
- QueryRelOp.cs
- InputProcessorProfiles.cs
- AssociatedControlConverter.cs
- HostedHttpRequestAsyncResult.cs
- UnionCodeGroup.cs
- MergeFailedEvent.cs
- DetailsViewRow.cs
- XmlSerializationGeneratedCode.cs
- SmiRecordBuffer.cs
- GridViewRowEventArgs.cs
- Simplifier.cs
- HttpRequest.cs
- Padding.cs
- CacheMemory.cs
- _NtlmClient.cs
- RecognizerBase.cs
- BitmapData.cs
- HttpServerVarsCollection.cs
- TrustLevelCollection.cs
- ItemCheckedEvent.cs
- ReturnEventArgs.cs
- EventLogSession.cs
- CodeExpressionStatement.cs
- CurrentChangingEventArgs.cs