Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Markup / XmlLanguageConverter.cs / 1305600 / XmlLanguageConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: XmlLanguageConverter.cs // // Description: Contains the XmlLanuageConverter: TypeConverter for the CultureInfo class. // // History: // 11/21/2005 : jerryd - Initial implementation // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Markup { ////// XmlLanuageConverter - Converter class for converting instances of other types to and from XmlLanguage /// in a way that does not depend on the current user's language settings. /// public class XmlLanguageConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string ietfLanguageTag = source as string; if (ietfLanguageTag != null) { return XmlLanguage.GetLanguage(ietfLanguageTag); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The XmlLanguage to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for XmlLanguage.IetfLanguageTag, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } XmlLanguage xmlLanguage = value as XmlLanguage; if (xmlLanguage != null) { if (destinationType == typeof(string)) { return xmlLanguage.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(XmlLanguage).GetMethod( "GetLanguage", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { xmlLanguage.IetfLanguageTag }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: XmlLanguageConverter.cs // // Description: Contains the XmlLanuageConverter: TypeConverter for the CultureInfo class. // // History: // 11/21/2005 : jerryd - Initial implementation // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows.Markup { ////// XmlLanuageConverter - Converter class for converting instances of other types to and from XmlLanguage /// in a way that does not depend on the current user's language settings. /// public class XmlLanguageConverter: TypeConverter { //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// CanConvertFrom - Returns whether or not this class can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext typeDescriptorContext, Type sourceType) { // We can only handle strings. return sourceType == typeof(string); } ////// CanConvertTo - Returns whether or not this class can convert to a given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext typeDescriptorContext, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. return destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string); } ////// ConvertFrom - Attempt to convert to a CultureInfo from the given object /// ////// A CultureInfo object based on the specified culture name. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a valid type /// which can be converted to a CultureInfo. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to a CultureInfo. public override object ConvertFrom(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object source) { string ietfLanguageTag = source as string; if (ietfLanguageTag != null) { return XmlLanguage.GetLanguage(ietfLanguageTag); } throw GetConvertFromException(source); } ////// ConvertTo - Attempt to convert a CultureInfo to the given type /// ////// The object which was constructed. /// ////// An ArgumentNullException is thrown if the example object is null. /// ////// An ArgumentException is thrown if the example object is not null and is not a CultureInfo, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The XmlLanguage to convert. /// The type to which to convert the CultureInfo. ////// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for XmlLanguage.IetfLanguageTag, not an arbitrary class/method /// [SecurityCritical] public override object ConvertTo(ITypeDescriptorContext typeDescriptorContext, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } XmlLanguage xmlLanguage = value as XmlLanguage; if (xmlLanguage != null) { if (destinationType == typeof(string)) { return xmlLanguage.IetfLanguageTag; } else if (destinationType == typeof(InstanceDescriptor)) { MethodInfo method = typeof(XmlLanguage).GetMethod( "GetLanguage", BindingFlags.Static | BindingFlags.InvokeMethod | BindingFlags.Public, null, // use default binder new Type[] { typeof(string) }, null // default binder doesn't use parameter modifiers ); return new InstanceDescriptor(method, new object[] { xmlLanguage.IetfLanguageTag }); } } throw GetConvertToException(value, destinationType); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
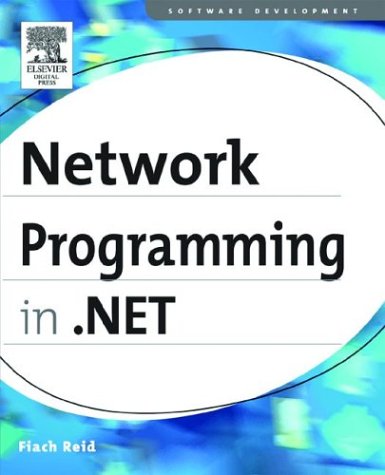
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlSerializerUtil.cs
- ClientOptions.cs
- Hex.cs
- Compensate.cs
- TimelineGroup.cs
- BuildProviderCollection.cs
- PropertyEmitterBase.cs
- ChildrenQuery.cs
- XmlSiteMapProvider.cs
- Int16Converter.cs
- ToggleButtonAutomationPeer.cs
- QueryableFilterRepeater.cs
- XAMLParseException.cs
- COM2ColorConverter.cs
- CorrelationHandle.cs
- XpsDigitalSignature.cs
- StrongTypingException.cs
- basevalidator.cs
- OleTxTransactionInfo.cs
- DateTimeConverter.cs
- XmlAnyElementAttributes.cs
- DefaultPropertyAttribute.cs
- Paragraph.cs
- EventWaitHandle.cs
- UserValidatedEventArgs.cs
- InternalConfigConfigurationFactory.cs
- XmlSchemaAny.cs
- XsltArgumentList.cs
- SID.cs
- ViewStateException.cs
- ErrorInfoXmlDocument.cs
- Blend.cs
- IPEndPointCollection.cs
- SQLDouble.cs
- DataGridViewCellParsingEventArgs.cs
- CodeIndexerExpression.cs
- ReadContentAsBinaryHelper.cs
- FlowSwitch.cs
- PEFileEvidenceFactory.cs
- SqlDataSourceWizardForm.cs
- PropertiesTab.cs
- LineInfo.cs
- OdbcInfoMessageEvent.cs
- AuthenticatingEventArgs.cs
- StorageConditionPropertyMapping.cs
- DataGridViewSortCompareEventArgs.cs
- WebBrowsableAttribute.cs
- Error.cs
- ComplexPropertyEntry.cs
- SendMailErrorEventArgs.cs
- DependencyPropertyHelper.cs
- NativeActivityTransactionContext.cs
- SizeF.cs
- ArgumentNullException.cs
- Pair.cs
- _TimerThread.cs
- ConfigXmlWhitespace.cs
- PointAnimationClockResource.cs
- ChannelSinkStacks.cs
- EncoderBestFitFallback.cs
- UIAgentAsyncParams.cs
- EventDescriptorCollection.cs
- Int32Rect.cs
- RpcAsyncResult.cs
- EmulateRecognizeCompletedEventArgs.cs
- MediaElementAutomationPeer.cs
- ToolboxBitmapAttribute.cs
- SqlMethodCallConverter.cs
- WebPartEditorApplyVerb.cs
- SqlUdtInfo.cs
- CFStream.cs
- LinearKeyFrames.cs
- Int32.cs
- StrongNameMembershipCondition.cs
- clipboard.cs
- RowCache.cs
- StringFunctions.cs
- DelegatedStream.cs
- PublisherMembershipCondition.cs
- SelectionChangedEventArgs.cs
- DivideByZeroException.cs
- SID.cs
- TextPointerBase.cs
- BufferedGraphics.cs
- LambdaExpression.cs
- DataKeyCollection.cs
- WebSysDefaultValueAttribute.cs
- DataColumnChangeEvent.cs
- KeyValueConfigurationElement.cs
- StructuredTypeInfo.cs
- Attributes.cs
- ToolStripRenderer.cs
- SchemaMerger.cs
- Configuration.cs
- SqlClientWrapperSmiStream.cs
- NavigationPropertySingletonExpression.cs
- ColorDialog.cs
- LambdaCompiler.Address.cs
- Predicate.cs
- HttpListenerRequest.cs