Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / PtsHost / OptimalBreakSession.cs / 1305600 / OptimalBreakSession.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: OptimalBreakSession // // Description: OptimalBreakSession is unmanaged resouce handle to TextParagraphCache // // History: // 06/07/2005 : ghermann - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Security; // SecurityCritical using System.Windows.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; using System.Windows.Media.TextFormatting; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Break record for line - holds decoration information // --------------------------------------------------------------------- internal sealed class OptimalBreakSession : UnmanagedHandle { // ------------------------------------------------------------------ // Constructor. // // PtsContext - Context // TextParagraphCache - Contained line break // ----------------------------------------------------------------- internal OptimalBreakSession(TextParagraph textParagraph, TextParaClient textParaClient, TextParagraphCache TextParagraphCache, OptimalTextSource optimalTextSource) : base(textParagraph.PtsContext) { _textParagraph = textParagraph; _textParaClient = textParaClient; _textParagraphCache = TextParagraphCache; _optimalTextSource = optimalTextSource; } ////// Dispose the break session / paragraph cache /// public override void Dispose() { try { if(_textParagraphCache != null) { _textParagraphCache.Dispose(); } if(_optimalTextSource != null) { _optimalTextSource.Dispose(); } } finally { _textParagraphCache = null; _optimalTextSource = null; } base.Dispose(); } #region Internal Properties internal TextParagraphCache TextParagraphCache { get { return _textParagraphCache; } } internal TextParagraph TextParagraph { get { return _textParagraph; } } internal TextParaClient TextParaClient { get { return _textParaClient; } } internal OptimalTextSource OptimalTextSource { get { return _optimalTextSource; } } #endregion Internal Properties #region Private Fields private TextParagraphCache _textParagraphCache; private TextParagraph _textParagraph; private TextParaClient _textParaClient; private OptimalTextSource _optimalTextSource; #endregion Private Fields } // ---------------------------------------------------------------------- // LineBreakpoint - Unmanaged handle for TextBreakpoint / optimal break session // ---------------------------------------------------------------------- internal sealed class LineBreakpoint : UnmanagedHandle { // ----------------------------------------------------------------- // Constructor. // // PtsContext - Context // TextBreakpoint - Contained breakpoint // ------------------------------------------------------------------ internal LineBreakpoint(OptimalBreakSession optimalBreakSession, TextBreakpoint textBreakpoint) : base(optimalBreakSession.PtsContext) { _textBreakpoint = textBreakpoint; _optimalBreakSession = optimalBreakSession; } ////// Dispose the text breakpoint /// public override void Dispose() { if(_textBreakpoint != null) { _textBreakpoint.Dispose(); } base.Dispose(); } #region Internal Properties internal OptimalBreakSession OptimalBreakSession { get { return _optimalBreakSession; } } #endregion Internal Properties #region Private Fields private TextBreakpoint _textBreakpoint; private OptimalBreakSession _optimalBreakSession; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: OptimalBreakSession // // Description: OptimalBreakSession is unmanaged resouce handle to TextParagraphCache // // History: // 06/07/2005 : ghermann - created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Windows; using System.Security; // SecurityCritical using System.Windows.Documents; using MS.Internal.Text; using MS.Internal.PtsHost.UnsafeNativeMethods; using System.Windows.Media.TextFormatting; namespace MS.Internal.PtsHost { // --------------------------------------------------------------------- // Break record for line - holds decoration information // --------------------------------------------------------------------- internal sealed class OptimalBreakSession : UnmanagedHandle { // ------------------------------------------------------------------ // Constructor. // // PtsContext - Context // TextParagraphCache - Contained line break // ----------------------------------------------------------------- internal OptimalBreakSession(TextParagraph textParagraph, TextParaClient textParaClient, TextParagraphCache TextParagraphCache, OptimalTextSource optimalTextSource) : base(textParagraph.PtsContext) { _textParagraph = textParagraph; _textParaClient = textParaClient; _textParagraphCache = TextParagraphCache; _optimalTextSource = optimalTextSource; } ////// Dispose the break session / paragraph cache /// public override void Dispose() { try { if(_textParagraphCache != null) { _textParagraphCache.Dispose(); } if(_optimalTextSource != null) { _optimalTextSource.Dispose(); } } finally { _textParagraphCache = null; _optimalTextSource = null; } base.Dispose(); } #region Internal Properties internal TextParagraphCache TextParagraphCache { get { return _textParagraphCache; } } internal TextParagraph TextParagraph { get { return _textParagraph; } } internal TextParaClient TextParaClient { get { return _textParaClient; } } internal OptimalTextSource OptimalTextSource { get { return _optimalTextSource; } } #endregion Internal Properties #region Private Fields private TextParagraphCache _textParagraphCache; private TextParagraph _textParagraph; private TextParaClient _textParaClient; private OptimalTextSource _optimalTextSource; #endregion Private Fields } // ---------------------------------------------------------------------- // LineBreakpoint - Unmanaged handle for TextBreakpoint / optimal break session // ---------------------------------------------------------------------- internal sealed class LineBreakpoint : UnmanagedHandle { // ----------------------------------------------------------------- // Constructor. // // PtsContext - Context // TextBreakpoint - Contained breakpoint // ------------------------------------------------------------------ internal LineBreakpoint(OptimalBreakSession optimalBreakSession, TextBreakpoint textBreakpoint) : base(optimalBreakSession.PtsContext) { _textBreakpoint = textBreakpoint; _optimalBreakSession = optimalBreakSession; } ////// Dispose the text breakpoint /// public override void Dispose() { if(_textBreakpoint != null) { _textBreakpoint.Dispose(); } base.Dispose(); } #region Internal Properties internal OptimalBreakSession OptimalBreakSession { get { return _optimalBreakSession; } } #endregion Internal Properties #region Private Fields private TextBreakpoint _textBreakpoint; private OptimalBreakSession _optimalBreakSession; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
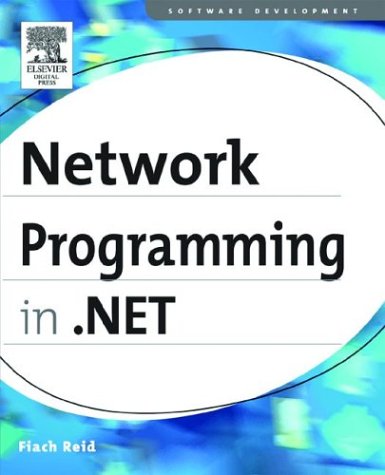
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DragEventArgs.cs
- XmlSchemas.cs
- AnnotationAuthorChangedEventArgs.cs
- FtpWebRequest.cs
- Type.cs
- SplitterPanelDesigner.cs
- RTTypeWrapper.cs
- RegularExpressionValidator.cs
- ToolCreatedEventArgs.cs
- FactorySettingsElement.cs
- SerializationException.cs
- DataSourceSelectArguments.cs
- Error.cs
- EntityDesignPluralizationHandler.cs
- OdbcEnvironment.cs
- ClientSettingsProvider.cs
- OdbcConnectionHandle.cs
- XmlHierarchicalEnumerable.cs
- ProcessProtocolHandler.cs
- VBCodeProvider.cs
- _ProxyChain.cs
- IdentifierCollection.cs
- AutomationElementIdentifiers.cs
- BamlVersionHeader.cs
- StyleXamlParser.cs
- ClientBuildManager.cs
- StyleTypedPropertyAttribute.cs
- ReflectPropertyDescriptor.cs
- DataListItem.cs
- PassportAuthenticationModule.cs
- ListViewInsertionMark.cs
- AutomationPeer.cs
- InputBuffer.cs
- SqlTrackingService.cs
- ProcessHostFactoryHelper.cs
- MenuItemStyleCollection.cs
- NumberFunctions.cs
- XmlWrappingReader.cs
- StringUtil.cs
- IListConverters.cs
- InheritanceRules.cs
- InfoCardPolicy.cs
- AsnEncodedData.cs
- ParenthesizePropertyNameAttribute.cs
- StackOverflowException.cs
- ConfigXmlAttribute.cs
- XmlCustomFormatter.cs
- ResourceReferenceExpression.cs
- CollectionViewGroupRoot.cs
- WindowsListViewSubItem.cs
- BlockUIContainer.cs
- Misc.cs
- FixedPageProcessor.cs
- OneOfConst.cs
- AssemblyCacheEntry.cs
- DataColumnCollection.cs
- Color.cs
- AnnotationMap.cs
- FormCollection.cs
- ValueTypeFixupInfo.cs
- NetworkInterface.cs
- ToolStripLabel.cs
- _Win32.cs
- AddInEnvironment.cs
- DataKey.cs
- AuthenticationException.cs
- HttpHandlersSection.cs
- InputBinding.cs
- CroppedBitmap.cs
- DesignSurfaceEvent.cs
- IisTraceWebEventProvider.cs
- IdentifierService.cs
- TableAdapterManagerGenerator.cs
- ISFClipboardData.cs
- Pointer.cs
- DeviceFilterEditorDialog.cs
- RelationshipConverter.cs
- DataServiceExpressionVisitor.cs
- FormsAuthenticationUserCollection.cs
- QueryComponents.cs
- EmulateRecognizeCompletedEventArgs.cs
- HttpProtocolReflector.cs
- Transactions.cs
- KoreanLunisolarCalendar.cs
- TreeNodeClickEventArgs.cs
- TemplateXamlParser.cs
- Thickness.cs
- ResourcePool.cs
- InputScopeManager.cs
- CounterSampleCalculator.cs
- PopOutPanel.cs
- SQLChars.cs
- FileAccessException.cs
- HotCommands.cs
- Array.cs
- AnnotationComponentManager.cs
- DesignerAdRotatorAdapter.cs
- ToolStripOverflowButton.cs
- TreeIterators.cs
- HwndStylusInputProvider.cs