Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / TableItemPattern.cs / 1305600 / TableItemPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for TableItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Used to expose grid items with header information. /// #if (INTERNAL_COMPILE) internal class TableItemPattern: GridItemPattern #else public class TableItemPattern: GridItemPattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern, cached) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///TableItem pattern public static readonly new AutomationPattern Pattern = TableItemPatternIdentifiers.Pattern; ///Property ID: RowHeaderItems - Collection of all row headers for this cell public static readonly AutomationProperty RowHeaderItemsProperty = TableItemPatternIdentifiers.RowHeaderItemsProperty; ///Property ID: ColumnHeaderItems - Collection of all column headers for this cell public static readonly AutomationProperty ColumnHeaderItemsProperty = TableItemPatternIdentifiers.ColumnHeaderItemsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// new public TableItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new TableItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// new public TableItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new TableItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static new object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new TableItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct TableItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal TableItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } ////// Collection of all row headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetRowHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(RowHeaderItemsProperty, _useCache); } ////// Collection of all column headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetColumnHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(ColumnHeaderItemsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for TableItem Pattern // // History: // 06/23/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; namespace System.Windows.Automation { ////// Used to expose grid items with header information. /// #if (INTERNAL_COMPILE) internal class TableItemPattern: GridItemPattern #else public class TableItemPattern: GridItemPattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemPattern(AutomationElement el, SafePatternHandle hPattern, bool cached) : base(el, hPattern, cached) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///TableItem pattern public static readonly new AutomationPattern Pattern = TableItemPatternIdentifiers.Pattern; ///Property ID: RowHeaderItems - Collection of all row headers for this cell public static readonly AutomationProperty RowHeaderItemsProperty = TableItemPatternIdentifiers.RowHeaderItemsProperty; ///Property ID: ColumnHeaderItems - Collection of all column headers for this cell public static readonly AutomationProperty ColumnHeaderItemsProperty = TableItemPatternIdentifiers.ColumnHeaderItemsProperty; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods #endregion Public Methods //----------------------------------------------------- // // Public Properties // //------------------------------------------------------ #region Public Properties ////// This member allows access to previously requested /// cached properties for this element. The returned object /// has accessors for each property defined for this pattern. /// ////// Cached property values must have been previously requested /// using a CacheRequest. If you try to access a cached /// property that was not previously requested, an InvalidOperation /// Exception will be thrown. /// /// To get the value of a property at the current point in time, /// access the property via the Current accessor instead of /// Cached. /// new public TableItemPatternInformation Cached { get { Misc.ValidateCached(_cached); return new TableItemPatternInformation(_el, true); } } ////// This member allows access to current property values /// for this element. The returned object has accessors for /// each property defined for this pattern. /// ////// This pattern must be from an AutomationElement with a /// Full reference in order to get current values. If the /// AutomationElement was obtained using AutomationElementMode.None, /// then it contains only cached data, and attempting to get /// the current value of any property will throw an InvalidOperationException. /// /// To get the cached value of a property that was previously /// specified using a CacheRequest, access the property via the /// Cached accessor instead of Current. /// new public TableItemPatternInformation Current { get { Misc.ValidateCurrent(_hPattern); return new TableItemPatternInformation(_el, false); } } #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal static new object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new TableItemPattern(el, hPattern, cached); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields //----------------------------------------------------- // // Nested Classes // //------------------------------------------------------ #region Nested Classes ////// This class provides access to either Cached or Current /// properties on a pattern via the pattern's .Cached or /// .Current accessors. /// public struct TableItemPatternInformation { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors internal TableItemPatternInformation(AutomationElement el, bool useCache) { _el = el; _useCache = useCache; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// the row number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Row { get { return (int)_el.GetPatternPropertyValue(RowProperty, _useCache); } } ////// /// the column number of the element. This is zero based. /// /// ////// This API does not work inside the secure execution environment. /// public int Column { get { return (int)_el.GetPatternPropertyValue(ColumnProperty, _useCache); } } ////// /// count of how many rows the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int RowSpan { get { return (int)_el.GetPatternPropertyValue(RowSpanProperty, _useCache); } } ////// /// count of how many columns the element spans /// -- non merged cells should always return 1 /// /// ////// This API does not work inside the secure execution environment. /// public int ColumnSpan { get { return (int)_el.GetPatternPropertyValue(ColumnSpanProperty, _useCache); } } ////// /// The logical element that supports the GripPattern for this Item /// /// ////// This API does not work inside the secure execution environment. /// public AutomationElement ContainingGrid { get { return (AutomationElement)_el.GetPatternPropertyValue(ContainingGridProperty, _useCache); } } ////// Collection of all row headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetRowHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(RowHeaderItemsProperty, _useCache); } ////// Collection of all column headers for this cell /// ////// This API does not work inside the secure execution environment. /// public AutomationElement[] GetColumnHeaderItems() { return (AutomationElement[])_el.GetPatternPropertyValue(ColumnHeaderItemsProperty, _useCache); } #endregion Public Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationElement _el; // AutomationElement that contains the cache or live reference private bool _useCache; // true to use cache, false to use live reference to get current values #endregion Private Fields } #endregion Nested Classes } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
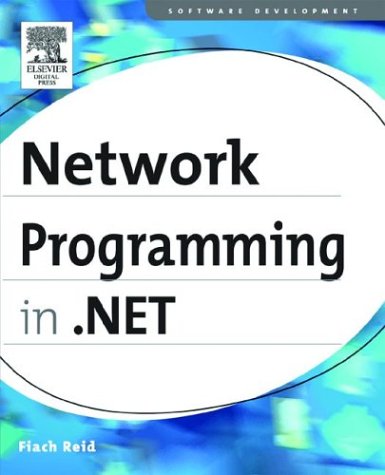
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuItemBinding.cs
- BaseParser.cs
- CatalogZoneBase.cs
- Triangle.cs
- ListViewItem.cs
- KnownAssembliesSet.cs
- Interlocked.cs
- ToolstripProfessionalRenderer.cs
- IdentityHolder.cs
- QueryStatement.cs
- HtmlElement.cs
- MarkupExtensionReturnTypeAttribute.cs
- ApplicationServicesHostFactory.cs
- xdrvalidator.cs
- OracleLob.cs
- SevenBitStream.cs
- DataGridViewSelectedRowCollection.cs
- EntitySetDataBindingList.cs
- WebUtil.cs
- SqlDataReaderSmi.cs
- Translator.cs
- CodeDOMUtility.cs
- Window.cs
- sortedlist.cs
- TreeChangeInfo.cs
- XmlSignatureProperties.cs
- OleDbCommandBuilder.cs
- ConfigurationLocation.cs
- WinCategoryAttribute.cs
- RuntimeConfigLKG.cs
- HtmlInputControl.cs
- SqlException.cs
- printdlgexmarshaler.cs
- LinearGradientBrush.cs
- WebPartMovingEventArgs.cs
- SendDesigner.xaml.cs
- XMLSchema.cs
- XmlSchemaProviderAttribute.cs
- MultiDataTrigger.cs
- XsltContext.cs
- FunctionImportMapping.cs
- HttpSysSettings.cs
- CodeAttributeDeclaration.cs
- XmlCDATASection.cs
- XmlDocumentFragment.cs
- SspiNegotiationTokenProvider.cs
- WinInet.cs
- TextContainerHelper.cs
- DefaultPrintController.cs
- InkSerializer.cs
- TextEncodedRawTextWriter.cs
- AdapterDictionary.cs
- coordinator.cs
- InnerItemCollectionView.cs
- ServiceDesigner.cs
- ProcessModuleCollection.cs
- base64Transforms.cs
- BitArray.cs
- RIPEMD160.cs
- EntityDataSourceChangedEventArgs.cs
- PcmConverter.cs
- Collection.cs
- CodePageUtils.cs
- ExpressionBuilderCollection.cs
- Marshal.cs
- DesignerSerializerAttribute.cs
- XmlSerializerNamespaces.cs
- WsatServiceAddress.cs
- SoapAttributes.cs
- OrderedDictionaryStateHelper.cs
- LateBoundChannelParameterCollection.cs
- ConstructorNeedsTagAttribute.cs
- CrossAppDomainChannel.cs
- Thickness.cs
- CreateBookmarkScope.cs
- Brush.cs
- BindingListCollectionView.cs
- TableRowCollection.cs
- SocketException.cs
- UnsafeNativeMethodsPenimc.cs
- Model3D.cs
- MonitoringDescriptionAttribute.cs
- ListCollectionView.cs
- KeyValueConfigurationElement.cs
- IOException.cs
- RelationshipConstraintValidator.cs
- Atom10FormatterFactory.cs
- DataBoundLiteralControl.cs
- ProfileInfo.cs
- ParameterDataSourceExpression.cs
- DataList.cs
- PolyBezierSegment.cs
- EnvelopedSignatureTransform.cs
- AstTree.cs
- Constants.cs
- LineBreak.cs
- SmtpCommands.cs
- SchemaTableOptionalColumn.cs
- Column.cs
- MemberPath.cs