Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / Workflow / ComponentModel / Design / FindSimilarActivitiesVerb.cs / 1305376 / FindSimilarActivitiesVerb.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Workflow.ComponentModel.Design { using System; using System.Collections.Generic; using System.ComponentModel; using System.ComponentModel.Design; using System.Runtime; using System.ServiceModel; using System.Workflow.ComponentModel; //// This the the class that implements the Search by menu item displayed on the activityDesigner // this is responsible for finding the matching activity designers and displaying them using // the acticvitydesigner hightlighter. // internal class FindSimilarActivitiesVerb: ActivityDesignerVerb where TActivity : Activity { List matchingActivityDesigner; ActivityComparer matchMaker; ActivityDesigner owner; public FindSimilarActivitiesVerb(ActivityDesigner designer, ActivityComparer matchMaker, string displayText) : base(designer, DesignerVerbGroup.Misc, displayText, new EventHandler(OnInvoke)) { Fx.Assert(designer != null, "Received null for designer parameter to FindSimilarActivitiesVerb ctor."); Fx.Assert(matchMaker != null, "Received null for matchMaker parameter to FindSimilarActivitiesVerb ctor."); this.owner = designer; this.matchMaker = matchMaker; } private static void OnInvoke(object source, EventArgs e) { FindSimilarActivitiesVerb designerVerb = source as FindSimilarActivitiesVerb ; ActivityDesigner activityDesigner = designerVerb.owner; List highlightedDesigners = designerVerb.GetMatchingActivityDesigners(activityDesigner); ActivityDesignerHighlighter hightlighter = new ActivityDesignerHighlighter(activityDesigner); hightlighter.Highlight(highlightedDesigners); } private ActivityDesigner GetDesigner(Activity activity) { IDesignerHost designerHost = this.GetService(typeof(IDesignerHost)) as IDesignerHost; return designerHost.GetDesigner(activity as IComponent) as ActivityDesigner; } private List GetMatchingActivityDesigners(ActivityDesigner activityDesigner) { CompositeActivityDesigner rootDesigner = DesignerPainter.GetRootDesigner(activityDesigner); matchingActivityDesigner = new List (); Walker activityTreeWalker = new Walker(); activityTreeWalker.FoundActivity += new WalkerEventHandler(OnWalkerFoundActivity); activityTreeWalker.Walk(rootDesigner.Activity); return matchingActivityDesigner; } private object GetService(Type serviceType) { if (serviceType == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("serviceType"); } if (owner.Activity != null && owner.Activity.Site != null) { return owner.Activity.Site.GetService(serviceType); } else { return null; } } private void OnWalkerFoundActivity(Walker walker, WalkerEventArgs eventArgs) { TActivity foundActivity = eventArgs.CurrentActivity as TActivity; if (foundActivity != null) { if (this.matchMaker((TActivity) owner.Activity, foundActivity)) { matchingActivityDesigner.Add(GetDesigner(eventArgs.CurrentActivity)); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
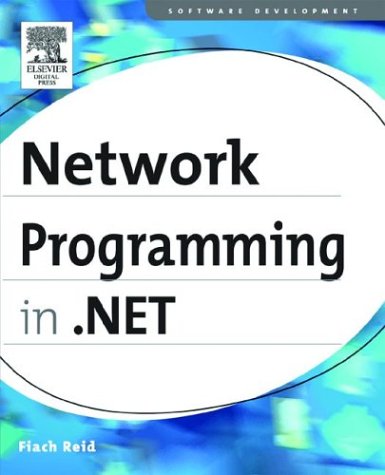
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CommonDialog.cs
- AsyncResult.cs
- StrongNameIdentityPermission.cs
- HwndSource.cs
- AspProxy.cs
- _NTAuthentication.cs
- ConfigurationLockCollection.cs
- CharKeyFrameCollection.cs
- Span.cs
- _SSPIWrapper.cs
- StylusTip.cs
- DynamicILGenerator.cs
- CompositeCollectionView.cs
- PropertyBuilder.cs
- TargetConverter.cs
- XPathArrayIterator.cs
- ListItemCollection.cs
- OperationExecutionFault.cs
- TabControlAutomationPeer.cs
- ControlTemplate.cs
- WebPartConnectionsCloseVerb.cs
- VersionConverter.cs
- TreeViewBindingsEditor.cs
- MsmqChannelFactory.cs
- DataSetMappper.cs
- PrintController.cs
- SelfIssuedAuthRSACryptoProvider.cs
- XPathScanner.cs
- PackageProperties.cs
- UserUseLicenseDictionaryLoader.cs
- JsonFormatMapping.cs
- SqlBuilder.cs
- TimersDescriptionAttribute.cs
- SerializerProvider.cs
- CodeCatchClauseCollection.cs
- HttpChannelListener.cs
- TransactionManager.cs
- BitmapEffectGroup.cs
- ProcessModelSection.cs
- SeekStoryboard.cs
- SpeechSynthesizer.cs
- BaseAddressElementCollection.cs
- WebPartsPersonalizationAuthorization.cs
- ImageEditor.cs
- HtmlTableRowCollection.cs
- RegistryKey.cs
- CodeIdentifiers.cs
- LinqDataSourceDeleteEventArgs.cs
- AlternateViewCollection.cs
- DebugInfoExpression.cs
- HttpClientCertificate.cs
- MemberAccessException.cs
- TreeNodeBinding.cs
- DesignerCategoryAttribute.cs
- LineServicesCallbacks.cs
- DataServiceRequestException.cs
- PrimitiveCodeDomSerializer.cs
- exports.cs
- ReliabilityContractAttribute.cs
- ApplicationDirectory.cs
- WmlPhoneCallAdapter.cs
- SettingsPropertyIsReadOnlyException.cs
- GenerateTemporaryTargetAssembly.cs
- ToolStripGrip.cs
- updatecommandorderer.cs
- Utils.cs
- CodeTypeParameter.cs
- FreeFormDragDropManager.cs
- CookieProtection.cs
- VirtualPathUtility.cs
- EFColumnProvider.cs
- ScriptBehaviorDescriptor.cs
- ValuePattern.cs
- CompositeActivityTypeDescriptorProvider.cs
- UnsafeNativeMethods.cs
- XPathSelfQuery.cs
- ItemMap.cs
- FixedTextView.cs
- ServiceMetadataContractBehavior.cs
- DynamicDiscoveryDocument.cs
- Inflater.cs
- TableItemPattern.cs
- BindingBase.cs
- ChineseLunisolarCalendar.cs
- SingleAnimationBase.cs
- OleDbCommand.cs
- ClockGroup.cs
- SimpleTypeResolver.cs
- X509Certificate2Collection.cs
- PropertyManager.cs
- CompilerHelpers.cs
- Table.cs
- ETagAttribute.cs
- NativeWindow.cs
- Token.cs
- ObjectQueryProvider.cs
- KnownTypesHelper.cs
- GenericsInstances.cs
- ForEachAction.cs
- DataBinder.cs