Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities.DurableInstancing / System / Activities / DurableInstancing / LoadRetryAsyncResult.cs / 1305376 / LoadRetryAsyncResult.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.DurableInstancing { using System; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Runtime; using System.Runtime.DurableInstancing; class LoadRetryAsyncResult : AsyncResult { static AsyncCallback onTryCommandCallback = Fx.ThunkCallback(new AsyncCallback(OnTryCommandCallback)); bool commandSuccess; TimeoutHelper commandTimeout; InstanceLockedException lastInstanceLockedException; int retryCount; public LoadRetryAsyncResult(SqlWorkflowInstanceStore store, InstancePersistenceContext context, InstancePersistenceCommand command, TimeSpan timeout, AsyncCallback callback, object state) : base(callback, state) { this.InstanceStore = store; this.InstancePersistenceContext = context; this.InstancePersistenceCommand = command; this.commandTimeout = new TimeoutHelper(timeout); InstanceStore.BeginTryCommandInternal(this.InstancePersistenceContext, this.InstancePersistenceCommand, this.commandTimeout.RemainingTime(), LoadRetryAsyncResult.onTryCommandCallback, this); } public SqlWorkflowInstanceStore InstanceStore { get; private set; } public TimeSpan RetryTimeout { get; private set; } InstancePersistenceCommand InstancePersistenceCommand { get; set; } InstancePersistenceContext InstancePersistenceContext { get; set; } public static bool End(IAsyncResult result) { LoadRetryAsyncResult thisPtr = AsyncResult.End(result); return thisPtr.commandSuccess; } public void AbortRetry() { Fx.Assert(this.lastInstanceLockedException != null, "no last instance lock exception"); this.Complete(false, this.lastInstanceLockedException); } public void Retry() { InstanceStore.BeginTryCommandInternal(this.InstancePersistenceContext, this.InstancePersistenceCommand, this.commandTimeout.RemainingTime(), LoadRetryAsyncResult.onTryCommandCallback, this); } [SuppressMessage(FxCop.Category.Design, FxCop.Rule.DoNotCatchGeneralExceptionTypes, Justification = "Standard AsyncResult callback pattern.")] static void OnTryCommandCallback(IAsyncResult result) { LoadRetryAsyncResult tryCommandAsyncResult = (LoadRetryAsyncResult)(result.AsyncState); Exception completeException = null; bool completeFlag = true; try { tryCommandAsyncResult.CompleteTryCommand(result); } catch (InstanceLockedException instanceLockedException) { TimeSpan retryDelay = tryCommandAsyncResult.InstanceStore.GetNextRetryDelay(++tryCommandAsyncResult.retryCount); if (retryDelay < tryCommandAsyncResult.commandTimeout.RemainingTime()) { tryCommandAsyncResult.RetryTimeout = retryDelay; if (tryCommandAsyncResult.InstanceStore.EnqueueRetry(tryCommandAsyncResult)) { tryCommandAsyncResult.lastInstanceLockedException = instanceLockedException; completeFlag = false; } } else if (TD.LockRetryTimeoutIsEnabled()) { TD.LockRetryTimeout(tryCommandAsyncResult.commandTimeout.OriginalTimeout.ToString()); } if (completeFlag) { completeException = instanceLockedException; } } catch (Exception exception) { if (Fx.IsFatal(exception)) { throw; } completeException = exception; } if (completeFlag) { tryCommandAsyncResult.Complete(false, completeException); } } void CompleteTryCommand(IAsyncResult result) { this.commandSuccess = this.InstanceStore.EndTryCommand(result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
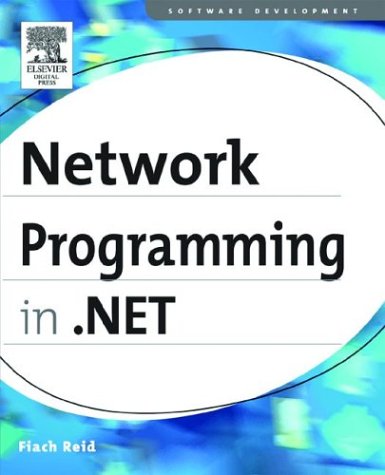
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextServicesDisplayAttribute.cs
- WebPartConnectionsDisconnectVerb.cs
- controlskin.cs
- CryptoHelper.cs
- InvalidEnumArgumentException.cs
- InstanceKeyNotReadyException.cs
- SafeNativeMethodsCLR.cs
- MatrixStack.cs
- BitArray.cs
- ColorConverter.cs
- WebPartVerbCollection.cs
- CharacterString.cs
- ToolStripKeyboardHandlingService.cs
- TypeLibConverter.cs
- Char.cs
- ScalarType.cs
- TranslateTransform3D.cs
- TextSchema.cs
- MobileCategoryAttribute.cs
- VirtualDirectoryMapping.cs
- ProjectedWrapper.cs
- ChildDocumentBlock.cs
- MarshalByRefObject.cs
- EmissiveMaterial.cs
- RuntimeTrackingProfile.cs
- CalendarAutomationPeer.cs
- ConfigXmlSignificantWhitespace.cs
- DiscreteKeyFrames.cs
- ResourceExpression.cs
- DataGridViewAutoSizeModeEventArgs.cs
- EncoderExceptionFallback.cs
- Array.cs
- TimeSpan.cs
- GregorianCalendar.cs
- CacheOutputQuery.cs
- AssemblyUtil.cs
- CompilerScopeManager.cs
- SecurityElement.cs
- Logging.cs
- OpacityConverter.cs
- SchemaEntity.cs
- HwndSourceKeyboardInputSite.cs
- CheckBoxField.cs
- ConnectionInterfaceCollection.cs
- ConfigXmlComment.cs
- DesignRelationCollection.cs
- WebChannelFactory.cs
- ServiceNameCollection.cs
- AttachedPropertyBrowsableAttribute.cs
- Effect.cs
- StreamWithDictionary.cs
- ObjectCacheHost.cs
- WmlListAdapter.cs
- _HelperAsyncResults.cs
- CellQuery.cs
- EventWaitHandle.cs
- ApplicationActivator.cs
- GridViewColumnCollection.cs
- CrossSiteScriptingValidation.cs
- SqlStream.cs
- AppDomainGrammarProxy.cs
- WindowsUserNameSecurityTokenAuthenticator.cs
- OpenTypeLayoutCache.cs
- ClientSettingsStore.cs
- MDIClient.cs
- SiteMapDataSourceView.cs
- BaseCodeDomTreeGenerator.cs
- ServiceInstallComponent.cs
- FileUtil.cs
- CodeGotoStatement.cs
- MultiPageTextView.cs
- WebPartsPersonalization.cs
- WorkflowView.cs
- XmlILStorageConverter.cs
- BaseCodePageEncoding.cs
- FunctionUpdateCommand.cs
- XmlEntityReference.cs
- CultureInfo.cs
- TextFormatterContext.cs
- GZipDecoder.cs
- StaticExtension.cs
- MailMessage.cs
- DatatypeImplementation.cs
- FocusChangedEventArgs.cs
- XmlCharacterData.cs
- RegexCode.cs
- StyleHelper.cs
- AbstractSvcMapFileLoader.cs
- LeftCellWrapper.cs
- CaretElement.cs
- ActiveXHost.cs
- MethodBuilderInstantiation.cs
- DateTimeFormatInfo.cs
- Baml6ConstructorInfo.cs
- xsdvalidator.cs
- ToolStripItemImageRenderEventArgs.cs
- TemplateControl.cs
- Common.cs
- WebConfigurationFileMap.cs
- LinkedResourceCollection.cs