Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Interaction / Model / ModelFactory.cs / 1305376 / ModelFactory.cs
namespace System.Activities.Presentation.Model { using System.Activities.Presentation.Services; using System.Activities.Presentation; using System; ////// The ModelFactory class should be used to create instances /// of items in the designer. ModelFactory is designed to be /// a static API for convenience. The underlying implementation /// of this API simply calls through to the ModelService�s /// CreateItem method. /// public static class ModelFactory { ////// Creates a new item for the given item type. /// /// /// The designer's editing context. /// /// /// The type of item to create. /// /// /// An optional array of arguments that should be passed to the constructor of the item. /// ////// The newly created item type. /// ///if itemType or context is null. ///if there is no editing model in the context that can create new items. public static ModelItem CreateItem(EditingContext context, Type itemType, params object[] arguments) { return CreateItem(context, itemType, CreateOptions.None, arguments); } ////// Creates a new item for the given item type. /// /// /// The designer's editing context. /// /// /// The type of item to create. /// /// /// A set of create options to use when creating the item. The default value is CreateOptions.None. /// /// /// An optional array of arguments that should be passed to the constructor of the item. /// ////// The newly created item type. /// ///if itemType or context is null. ///if there is no editing model in the context that can create new items. public static ModelItem CreateItem(EditingContext context, Type itemType, CreateOptions options, params object[] arguments) { if (context == null) throw FxTrace.Exception.ArgumentNull("context"); if (itemType == null) throw FxTrace.Exception.ArgumentNull("itemType"); if (!EnumValidator.IsValid(options)) throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("options")); ModelService ms = context.Services.GetRequiredService(); return ms.InvokeCreateItem(itemType, options, arguments); } /// /// Creates a new model item by creating a deep copy of the isntance provided. /// /// /// The designer's editing context. /// /// /// The item to clone. /// ////// The newly created item. /// public static ModelItem CreateItem(EditingContext context, object item) { if (context == null) throw FxTrace.Exception.ArgumentNull("context"); if (item == null) throw FxTrace.Exception.ArgumentNull("item"); ModelService ms = context.Services.GetRequiredService(); return ms.InvokeCreateItem(item); } /// /// Create a new model item that represents a the value of a static member of a the given class. /// For example, to add a reference to Brushes.Red to the model call this methods with /// typeof(Brushes) and the string "Red". This will be serialized into XAML as /// {x:Static Brushes.Red}. /// /// /// The designer's editing context. /// /// /// The type that contains the static member being referenced. /// /// /// The name of the static member being referenced. /// ///public static ModelItem CreateStaticMemberItem(EditingContext context, Type type, string memberName) { if (context == null) throw FxTrace.Exception.ArgumentNull("context"); if (type == null) throw FxTrace.Exception.ArgumentNull("type"); if (memberName == null) throw FxTrace.Exception.ArgumentNull("memberName"); ModelService ms = context.Services.GetRequiredService (); return ms.InvokeCreateStaticMemberItem(type, memberName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
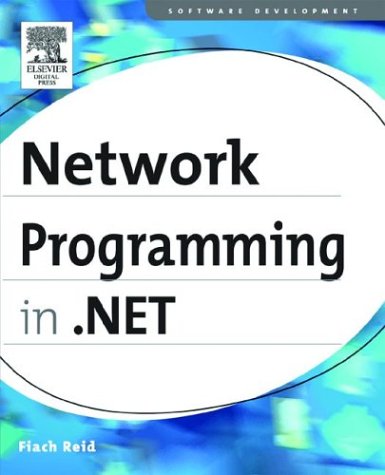
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewInsertionMark.cs
- AvTrace.cs
- TextServicesCompartment.cs
- ObjectTypeMapping.cs
- DataMemberFieldConverter.cs
- InstanceData.cs
- ButtonBase.cs
- ManagementObjectCollection.cs
- QueryOpeningEnumerator.cs
- ConfigurationValidatorBase.cs
- columnmapfactory.cs
- HttpHandlersInstallComponent.cs
- ToolStripItemDataObject.cs
- XmlDataSourceView.cs
- Version.cs
- DispatcherSynchronizationContext.cs
- MouseGesture.cs
- VoiceObjectToken.cs
- SynchronizationLockException.cs
- ZipPackagePart.cs
- FormCollection.cs
- CodeIterationStatement.cs
- securitymgrsite.cs
- ZipIOLocalFileDataDescriptor.cs
- ConstantCheck.cs
- InputProcessorProfilesLoader.cs
- ToolStripMenuItem.cs
- TextRangeEditTables.cs
- KeyConstraint.cs
- StringComparer.cs
- DropDownList.cs
- CallbackTimeoutsBehavior.cs
- WebContentFormatHelper.cs
- System.Data_BID.cs
- shaperfactoryquerycachekey.cs
- ILGenerator.cs
- ExtendedProtectionPolicyTypeConverter.cs
- AutomationFocusChangedEventArgs.cs
- PrinterResolution.cs
- ChtmlLinkAdapter.cs
- UIPermission.cs
- GregorianCalendar.cs
- BooleanSwitch.cs
- FormViewUpdatedEventArgs.cs
- Repeater.cs
- HotSpotCollection.cs
- DelayedRegex.cs
- DataObjectMethodAttribute.cs
- DataDesignUtil.cs
- _BasicClient.cs
- Effect.cs
- FontFamilyConverter.cs
- MapPathBasedVirtualPathProvider.cs
- HashMembershipCondition.cs
- ListView.cs
- ServiceHandle.cs
- TemplateXamlParser.cs
- DmlSqlGenerator.cs
- ResumeStoryboard.cs
- DesignerCategoryAttribute.cs
- KeyInstance.cs
- Int64AnimationBase.cs
- EditorResources.cs
- Operator.cs
- VScrollProperties.cs
- PositiveTimeSpanValidator.cs
- ToolConsole.cs
- HyperLinkField.cs
- ExceptionUtil.cs
- ScrollContentPresenter.cs
- RenderData.cs
- HtmlWindow.cs
- NavigationEventArgs.cs
- ExpandSegment.cs
- WhiteSpaceTrimStringConverter.cs
- HelloOperationCD1AsyncResult.cs
- RoleGroup.cs
- BorderGapMaskConverter.cs
- SemanticAnalyzer.cs
- HttpProfileGroupBase.cs
- SqlReorderer.cs
- JournalNavigationScope.cs
- SmtpAuthenticationManager.cs
- Crc32.cs
- ChtmlImageAdapter.cs
- CompoundFileStreamReference.cs
- EditorZone.cs
- TypeForwardedToAttribute.cs
- DateTimeConverter2.cs
- CmsUtils.cs
- HwndMouseInputProvider.cs
- TextRunTypographyProperties.cs
- ValueQuery.cs
- CatalogZoneBase.cs
- SecurityTokenTypes.cs
- String.cs
- Application.cs
- SqlParameter.cs
- NonClientArea.cs
- XDeferredAxisSource.cs