Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Tools / WSATConfig / Configuration / FirewallWrapper.cs / 1305376 / FirewallWrapper.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.Tools.ServiceModel.WsatConfig { using System; using System.Collections.Generic; using System.Text; using System.Runtime.InteropServices; using System.Collections; using System.Security.Permissions; class FirewallWrapper { const string FwMgrClassId = "{304CE942-6E39-40D8-943A-B913C40C9CD4}"; const string FwOpenPortClassId = "{0CA545C6-37AD-4A6C-BF92-9F7610067EF5}"; INetFirewallMgr manager = null; INetFirewallOpenPortsCollection openPorts = null; INetFirewallPolicy localPolicy = null; INetFirewallProfile currentProfile = null; [SecurityPermission(SecurityAction.LinkDemand, UnmanagedCode = true)] internal FirewallWrapper() { try { this.manager = (INetFirewallMgr)Activator.CreateInstance(Type.GetTypeFromCLSID(new Guid(FwMgrClassId))); this.localPolicy = this.manager.LocalPolicy; this.currentProfile = this.localPolicy.CurrentProfile; this.openPorts = this.currentProfile.GloballyOpenPorts; } catch (COMException) { this.manager = null; this.localPolicy = null; this.currentProfile = null; this.openPorts = null; } catch (MethodAccessException ex) { throw new WsatAdminException(WsatAdminErrorCode.FIREWALL_ACCESS_DENIED, SR.GetString(SR.FirewallAccessDenied), ex); } } bool IsHttpsPortOpened(int port) { foreach (INetFirewallOpenPort openPort in this.openPorts) { if (openPort.Port == port) { return true; } } return false; } internal void AddHttpsPort(int portToAdd) { // //if portToAdd is already opened, adding it anyway will remove the old entry // if (portToAdd < 0 || this.openPorts == null || IsHttpsPortOpened(portToAdd)) { return; } try { INetFirewallOpenPort openPort = (INetFirewallOpenPort)Activator.CreateInstance(Type.GetTypeFromCLSID(new Guid(FwOpenPortClassId))); openPort.Enabled = true; openPort.IPVersion = NetFirewallIPVersion.Any; openPort.Name = SR.GetString(SR.HTTPSPortName); openPort.Port = portToAdd; openPort.Protocol = NetFirewallIPProtocol.Tcp; openPort.Scope = NetFirewallScope.All; this.openPorts.Add(openPort); } catch (COMException e) { throw new WsatAdminException(WsatAdminErrorCode.UNEXPECTED_FIREWALL_CONFIG_ERROR, SR.GetString(SR.UnexpectedFirewallError, e.Message), e); } catch (MethodAccessException e) { throw new WsatAdminException(WsatAdminErrorCode.FIREWALL_ACCESS_DENIED, SR.GetString(SR.FirewallAccessDenied), e); } catch (UnauthorizedAccessException e) { throw new WsatAdminException(WsatAdminErrorCode.FIREWALL_ACCESS_DENIED, SR.GetString(SR.FirewallAccessDenied), e); } } internal void RemoveHttpsPort(int portToRemove) { if (portToRemove<0 || this.openPorts == null) { return; } Listports = new List (); foreach (INetFirewallOpenPort port in this.openPorts) { if (port.Port == portToRemove && Utilities.SafeCompare(port.Name, SR.GetString(SR.HTTPSPortName))) { ports.Add(port); // continue to remove other ports under the WSAT port name to minimize security attack // surface for the machine, but throw out an exception in the end } } bool accessDenied = false; foreach (INetFirewallOpenPort port in ports) { try { this.openPorts.Remove(port.Port, port.Protocol); } catch (UnauthorizedAccessException) { accessDenied = true; } } if (accessDenied) { // at least one port could not be removed due to permission denied throw new WsatAdminException(WsatAdminErrorCode.FIREWALL_ACCESS_DENIED, SR.GetString(SR.FirewallAccessDenied)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
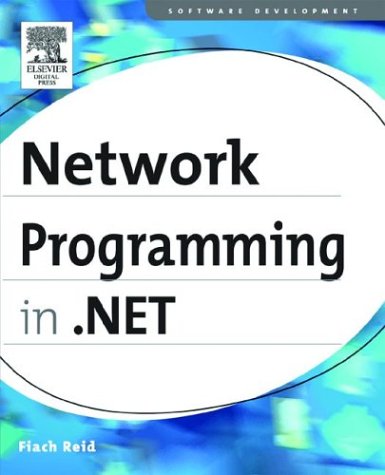
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CoreChannel.cs
- ReturnEventArgs.cs
- Transform.cs
- SelectingProviderEventArgs.cs
- CompositeActivityCodeGenerator.cs
- HorizontalAlignConverter.cs
- ExpressionBuilderContext.cs
- ChtmlTextWriter.cs
- ObjectDataSourceDisposingEventArgs.cs
- HuffmanTree.cs
- SecUtil.cs
- _Events.cs
- WindowsScrollBarBits.cs
- HttpAsyncResult.cs
- CharUnicodeInfo.cs
- StrokeCollectionDefaultValueFactory.cs
- DateTimePicker.cs
- DNS.cs
- TypeCollectionPropertyEditor.cs
- ArithmeticException.cs
- LocatorGroup.cs
- InlineCollection.cs
- WorkflowEnvironment.cs
- ElementNotEnabledException.cs
- AcceleratedTokenProviderState.cs
- MatrixTransform.cs
- LineUtil.cs
- FixedFlowMap.cs
- SHA1.cs
- XmlIgnoreAttribute.cs
- SafeArchiveContext.cs
- WebPartHelpVerb.cs
- CommentGlyph.cs
- TerminatorSinks.cs
- ElementAction.cs
- HwndProxyElementProvider.cs
- XmlSortKeyAccumulator.cs
- NumericUpDown.cs
- WebServiceReceiveDesigner.cs
- ComponentCodeDomSerializer.cs
- InOutArgument.cs
- PropertyToken.cs
- EdmProperty.cs
- IssuedSecurityTokenProvider.cs
- XPathNavigator.cs
- Transform3DGroup.cs
- MobileControlDesigner.cs
- IconBitmapDecoder.cs
- DesignerExtenders.cs
- Material.cs
- OutputWindow.cs
- LogSwitch.cs
- TransformerInfoCollection.cs
- LayoutEditorPart.cs
- InvalidDataContractException.cs
- LinkedList.cs
- BuildResult.cs
- IgnoreSectionHandler.cs
- ISAPIApplicationHost.cs
- ResourceWriter.cs
- BuildDependencySet.cs
- PrintEvent.cs
- SamlSubjectStatement.cs
- ConfigurationSectionHelper.cs
- WorkflowServiceOperationListItem.cs
- PasswordRecoveryDesigner.cs
- MethodBody.cs
- FilteredDataSetHelper.cs
- SchemaLookupTable.cs
- KeysConverter.cs
- DbConnectionOptions.cs
- ProviderConnectionPointCollection.cs
- XmlILConstructAnalyzer.cs
- ToolStripSystemRenderer.cs
- TranslateTransform.cs
- CounterCreationDataConverter.cs
- Tokenizer.cs
- AsyncCodeActivityContext.cs
- ScriptResourceInfo.cs
- AVElementHelper.cs
- Pens.cs
- ValidatorAttribute.cs
- Expressions.cs
- XmlSchemaInclude.cs
- DataGridViewElement.cs
- ProvidersHelper.cs
- InfocardInteractiveChannelInitializer.cs
- HostedImpersonationContext.cs
- WebPartActionVerb.cs
- PropertyIDSet.cs
- TableCellsCollectionEditor.cs
- CatalogZoneAutoFormat.cs
- Formatter.cs
- Point3DValueSerializer.cs
- ToolStripHighContrastRenderer.cs
- IndentedWriter.cs
- FocusManager.cs
- HttpResponse.cs
- EditorZone.cs
- MouseButtonEventArgs.cs