Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / OperatorExpressions.cs / 1305376 / OperatorExpressions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; namespace System.Data.Common.CommandTrees { #region Boolean Operators ////// Represents the logical And of two Boolean arguments. /// ///DbAndExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbAndExpression : DbBinaryExpression { internal DbAndExpression(TypeUsage booleanResultType, DbExpression left, DbExpression right) : base(DbExpressionKind.And, booleanResultType, left, right) { Debug.Assert(TypeSemantics.IsPrimitiveType(booleanResultType, PrimitiveTypeKind.Boolean), "DbAndExpression requires a Boolean result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Or of two Boolean arguments. /// ///DbOrExpression requires that both of its arguments have a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOrExpression : DbBinaryExpression { internal DbOrExpression(TypeUsage booleanResultType, DbExpression left, DbExpression right) : base(DbExpressionKind.Or, booleanResultType, left, right) { Debug.Assert(TypeSemantics.IsPrimitiveType(booleanResultType, PrimitiveTypeKind.Boolean), "DbOrExpression requires a Boolean result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the logical Not of a single Boolean argument. /// ///DbNotExpression requires that its argument has a Boolean result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbNotExpression : DbUnaryExpression { internal DbNotExpression(TypeUsage booleanResultType, DbExpression argument) : base(DbExpressionKind.Not, booleanResultType, argument) { Debug.Assert(TypeSemantics.IsPrimitiveType(booleanResultType, PrimitiveTypeKind.Boolean), "DbNotExpression requires a Boolean result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } #endregion /// /// Represents an arithmetic operation (addition, subtraction, multiplication, division, modulo or negation) applied to two numeric arguments. /// ///DbArithmeticExpression requires that its arguments have a common numeric result type [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbArithmeticExpression : DbExpression { private readonly DbExpressionList _args; internal DbArithmeticExpression(DbExpressionKind kind, TypeUsage numericResultType, DbExpressionList args) : base(kind, numericResultType) { Debug.Assert(TypeSemantics.IsNumericType(numericResultType), "DbArithmeticExpression result type must be numeric"); Debug.Assert( DbExpressionKind.Divide == kind || DbExpressionKind.Minus == kind || DbExpressionKind.Modulo == kind || DbExpressionKind.Multiply == kind || DbExpressionKind.Plus == kind || DbExpressionKind.UnaryMinus == kind, "Invalid DbExpressionKind used in DbArithmeticExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); Debug.Assert(args != null, "DbArithmeticExpression arguments cannot be null"); Debug.Assert( (DbExpressionKind.UnaryMinus == kind && 1 == args.Count) || 2 == args.Count, "Incorrect number of arguments specified to DbArithmeticExpression" ); this._args = args; } ////// Gets the list of expressions that define the current arguments. /// ////// The public IListArguments
property returns a fixed-size list ofelements. /// requires that all elements of it's Arguments
list /// have a common numeric result type. ///Arguments { get { return _args; } } /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a Case When...Then...Else logical operation. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCaseExpression : DbExpression { private readonly DbExpressionList _when; private readonly DbExpressionList _then; private readonly DbExpression _else; internal DbCaseExpression(TypeUsage commonResultType, DbExpressionList whens, DbExpressionList thens, DbExpression elseExpr) : base(DbExpressionKind.Case, commonResultType) { Debug.Assert(whens != null, "DbCaseExpression whens cannot be null"); Debug.Assert(thens != null, "DbCaseExpression thens cannot be null"); Debug.Assert(elseExpr != null, "DbCaseExpression else cannot be null"); Debug.Assert(whens.Count == thens.Count, "DbCaseExpression whens count must match thens count"); this._when = whens; this._then = thens; this._else = elseExpr; } ////// Gets the When clauses of this DbCaseExpression. /// public IListWhen { get { return _when; } } /// /// Gets the Then clauses of this DbCaseExpression. /// public IListThen { get { return _then; } } /// /// Gets the Else clause of this DbCaseExpression. /// public DbExpression Else { get { return _else; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a cast operation applied to a polymorphic argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbCastExpression : DbUnaryExpression { internal DbCastExpression(TypeUsage type, DbExpression argument) : base(DbExpressionKind.Cast, type, argument) { Debug.Assert(TypeSemantics.IsCastAllowed(argument.ResultType, type), "DbCastExpression represents an invalid cast"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a comparison operation (equality, greater than, greather than or equal, less than, less than or equal, inequality) applied to two arguments. /// ////// DbComparisonExpression requires that its arguments have a common result type /// that is equality comparable (for [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbComparisonExpression : DbBinaryExpression { internal DbComparisonExpression(DbExpressionKind kind, TypeUsage booleanResultType, DbExpression left, DbExpression right) : base(kind, booleanResultType, left, right) { Debug.Assert(left != null, "DbComparisonExpression left cannot be null"); Debug.Assert(right != null, "DbComparisonExpression right cannot be null"); Debug.Assert(TypeSemantics.IsBooleanType(booleanResultType), "DbComparisonExpression result type must be a Boolean type"); Debug.Assert( DbExpressionKind.Equals == kind || DbExpressionKind.LessThan == kind || DbExpressionKind.LessThanOrEquals == kind || DbExpressionKind.GreaterThan == kind || DbExpressionKind.GreaterThanOrEquals == kind || DbExpressionKind.NotEquals == kind, "Invalid DbExpressionKind used in DbComparisonExpression: " + Enum.GetName(typeof(DbExpressionKind), kind) ); } ///.Equals and .NotEquals), /// order comparable (for .GreaterThan and .LessThan), /// or both (for .GreaterThanOrEquals and .LessThanOrEquals). /// /// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents empty set determination applied to a single set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsEmptyExpression : DbUnaryExpression { internal DbIsEmptyExpression(TypeUsage booleanResultType, DbExpression argument) : base(DbExpressionKind.IsEmpty, booleanResultType, argument) { Debug.Assert(TypeSemantics.IsBooleanType(booleanResultType), "DbIsEmptyExpression requires a Boolean result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents null determination applied to a single argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsNullExpression : DbUnaryExpression { internal DbIsNullExpression(TypeUsage booleanResultType, DbExpression arg, bool isRowTypeArgumentAllowed) : base(DbExpressionKind.IsNull, booleanResultType, arg) { Debug.Assert(TypeSemantics.IsBooleanType(booleanResultType), "DbIsNullExpression requires a Boolean result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type comparison of a single argument against the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbIsOfExpression : DbUnaryExpression { private TypeUsage _ofType; internal DbIsOfExpression(DbExpressionKind isOfKind, TypeUsage booleanResultType, DbExpression argument, TypeUsage isOfType) : base(isOfKind, booleanResultType, argument) { Debug.Assert(DbExpressionKind.IsOf == this.ExpressionKind || DbExpressionKind.IsOfOnly == this.ExpressionKind, string.Format(CultureInfo.InvariantCulture, "Invalid DbExpressionKind used in DbIsOfExpression: {0}", Enum.GetName(typeof(DbExpressionKind), this.ExpressionKind))); Debug.Assert(TypeSemantics.IsBooleanType(booleanResultType), "DbIsOfExpression requires a Boolean result type"); this._ofType = isOfType; } ////// Gets the type metadata that the type metadata of the argument should be compared to. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of elements of the specified type from the given set argument. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbOfTypeExpression : DbUnaryExpression { private readonly TypeUsage _ofType; internal DbOfTypeExpression(DbExpressionKind ofTypeKind, TypeUsage collectionResultType, DbExpression argument, TypeUsage type) : base(ofTypeKind, collectionResultType, argument) { Debug.Assert(DbExpressionKind.OfType == ofTypeKind || DbExpressionKind.OfTypeOnly == ofTypeKind, "ExpressionKind for DbOfTypeExpression must be OfType or OfTypeOnly"); // // Assign the requested element type to the OfType property. // this._ofType = type; } ////// Gets the metadata of the type of elements that should be retrieved from the set argument. /// public TypeUsage OfType { get { return _ofType; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the type conversion of a single argument to the specified type. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbTreatExpression : DbUnaryExpression { internal DbTreatExpression(TypeUsage asType, DbExpression argument) : base(DbExpressionKind.Treat, asType, argument) { Debug.Assert(TypeSemantics.IsValidPolymorphicCast(argument.ResultType, asType), "DbTreatExpression represents an invalid treat"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents a string comparison against the specified pattern with an optional escape string /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbLikeExpression : DbExpression { private readonly DbExpression _argument; private readonly DbExpression _pattern; private readonly DbExpression _escape; internal DbLikeExpression(TypeUsage booleanResultType, DbExpression input, DbExpression pattern, DbExpression escape) : base(DbExpressionKind.Like, booleanResultType) { Debug.Assert(input != null, "DbLikeExpression argument cannot be null"); Debug.Assert(pattern != null, "DbLikeExpression pattern cannot be null"); Debug.Assert(escape != null, "DbLikeExpression escape cannot be null"); Debug.Assert(TypeSemantics.IsPrimitiveType(input.ResultType, PrimitiveTypeKind.String), "DbLikeExpression argument must have a string result type"); Debug.Assert(TypeSemantics.IsPrimitiveType(pattern.ResultType, PrimitiveTypeKind.String), "DbLikeExpression pattern must have a string result type"); Debug.Assert(TypeSemantics.IsPrimitiveType(escape.ResultType, PrimitiveTypeKind.String), "DbLikeExpression escape must have a string result type"); Debug.Assert(TypeSemantics.IsBooleanType(booleanResultType), "DbLikeExpression must have a Boolean result type"); this._argument = input; this._pattern = pattern; this._escape = escape; } ////// Gets the expression that specifies the string to compare against the given pattern /// public DbExpression Argument { get { return _argument; } } ////// Gets the expression that specifies the pattern against which the given string should be compared /// public DbExpression Pattern { get { return _pattern; } } ////// Gets the expression that provides an optional escape string to use for the comparison /// public DbExpression Escape { get { return _escape; } } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of a reference to the specified Entity as a Ref. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbEntityRefExpression : DbUnaryExpression { internal DbEntityRefExpression(TypeUsage refResultType, DbExpression entity) : base(DbExpressionKind.EntityRef, refResultType, entity) { Debug.Assert(TypeSemantics.IsReferenceType(refResultType), "DbEntityRefExpression requires a reference result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } /// /// Represents the retrieval of the key value of the specified Reference as a row. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbRefKeyExpression : DbUnaryExpression { internal DbRefKeyExpression(TypeUsage rowResultType, DbExpression reference) : base(DbExpressionKind.RefKey, rowResultType, reference) { Debug.Assert(TypeSemantics.IsRowType(rowResultType), "DbRefKeyExpression requires a row result type"); } ////// The visitor pattern method for expression visitors that do not produce a result value. /// /// An instance of DbExpressionVisitor. ///public override void Accept(DbExpressionVisitor visitor) { if (visitor != null) { visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } /// is null /// The visitor pattern method for expression visitors that produce a result value of a specific type. /// /// An instance of a typed DbExpressionVisitor that produces a result value of type TResultType. ///The type of the result produced by ////// is null An instance of public override TResultType Accept. (DbExpressionVisitor visitor) { if (visitor != null) { return visitor.Visit(this); } else { throw EntityUtil.ArgumentNull("visitor"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
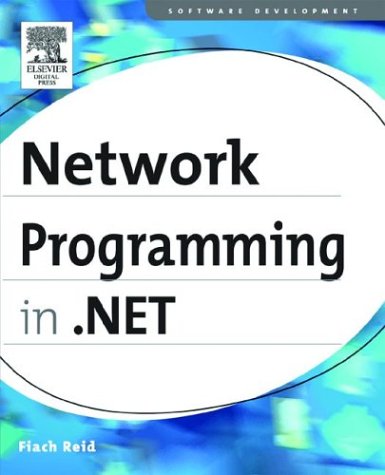
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SaveFileDialog.cs
- NumericExpr.cs
- FontConverter.cs
- BamlResourceDeserializer.cs
- TraceLevelStore.cs
- Scalars.cs
- SevenBitStream.cs
- PrintPreviewControl.cs
- HtmlPageAdapter.cs
- FormatConvertedBitmap.cs
- AdCreatedEventArgs.cs
- DeviceFilterEditorDialog.cs
- FunctionMappingTranslator.cs
- Setter.cs
- SqlParameter.cs
- JournalEntryListConverter.cs
- CustomSignedXml.cs
- ObjectStateFormatter.cs
- TreeViewDesigner.cs
- SendKeys.cs
- EntityDataSourceEntityTypeFilterItem.cs
- SelectionRangeConverter.cs
- ScriptManagerProxy.cs
- DllNotFoundException.cs
- ComponentEditorForm.cs
- XmlIlVisitor.cs
- BaseDataBoundControlDesigner.cs
- HtmlContainerControl.cs
- newinstructionaction.cs
- DataGridToolTip.cs
- InvalidWMPVersionException.cs
- ResolvedKeyFrameEntry.cs
- GPRECTF.cs
- DeclarativeExpressionConditionDeclaration.cs
- MessageDecoder.cs
- MetadataItem_Static.cs
- sapiproxy.cs
- XmlAttributeCache.cs
- GridViewDeleteEventArgs.cs
- InstanceOwnerQueryResult.cs
- Header.cs
- XmlUrlResolver.cs
- Quaternion.cs
- Scene3D.cs
- CustomErrorsSection.cs
- AQNBuilder.cs
- Transform3DGroup.cs
- DataTableCollection.cs
- LogicalExpr.cs
- Visual3D.cs
- NativeMethods.cs
- ReflectEventDescriptor.cs
- OperationPerformanceCounters.cs
- HttpRequestBase.cs
- GeneratedCodeAttribute.cs
- PolyLineSegmentFigureLogic.cs
- ControlPaint.cs
- ControlPropertyNameConverter.cs
- TypeForwardedToAttribute.cs
- InstanceLockException.cs
- SqlMethods.cs
- glyphs.cs
- TextDecoration.cs
- XmlNavigatorFilter.cs
- CodeConstructor.cs
- WmfPlaceableFileHeader.cs
- NativeMethods.cs
- OledbConnectionStringbuilder.cs
- mactripleDES.cs
- BuildProvider.cs
- ObjectSet.cs
- StyleBamlRecordReader.cs
- XmlDataSource.cs
- WebPartUserCapability.cs
- TableColumnCollection.cs
- SortExpressionBuilder.cs
- ToolStripGrip.cs
- TransformValueSerializer.cs
- PropertyIDSet.cs
- DataGridColumnFloatingHeader.cs
- SqlDataAdapter.cs
- ReferencedType.cs
- TypeSystem.cs
- CatalogPart.cs
- SortAction.cs
- GenericXmlSecurityToken.cs
- ComplexPropertyEntry.cs
- FrameworkElementFactory.cs
- ObjectQueryProvider.cs
- LocationSectionRecord.cs
- InternalBufferManager.cs
- _NestedSingleAsyncResult.cs
- FixedDSBuilder.cs
- ConsoleTraceListener.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- EnterpriseServicesHelper.cs
- InfiniteIntConverter.cs
- SerializationTrace.cs
- ExceptionCollection.cs
- SyntaxCheck.cs