Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / EnumType.cs / 1305376 / EnumType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- namespace System.Data.Metadata.Edm { using System.Data.Common; ////// Class representing a enumeration type /// internal sealed class EnumType : SimpleType { #region Constructors ////// The default constructor for EnumType: for bootstraping /// internal EnumType() { } ////// The constructor for EnumType. It takes the required information to identify this type. /// /// The name of this type /// The namespace name of this type /// The version of this type /// dataspace in which the enum belongs to ///Thrown if either name, namespace or version arguments are null internal EnumType(string name, string namespaceName, DataSpace dataSpace) : base(name, namespaceName, dataSpace) { } #endregion #region Fields private readonly ReadOnlyMetadataCollection_enumMembers = new ReadOnlyMetadataCollection (new MetadataCollection ()); #endregion #region Properties /// /// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.EnumType; } } ////// The collection of enumeration members belong to this enumeration type /// [MetadataProperty(BuiltInTypeKind.EnumMember, true)] public ReadOnlyMetadataCollectionEnumMembers { get { return _enumMembers; } } /// /// Sets this item to be readonly, once this is set, the item will never be writable again. /// internal override void SetReadOnly() { if (!IsReadOnly) { base.SetReadOnly(); this.EnumMembers.Source.SetReadOnly(); } } ////// Adds the given member to the member collection /// /// internal void AddMember(EnumMember enumMember) { this.EnumMembers.Source.Add(enumMember); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
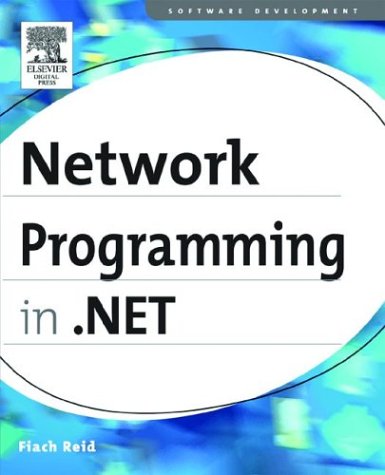
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OutputScope.cs
- Delay.cs
- MonitoringDescriptionAttribute.cs
- XPathAxisIterator.cs
- RoleService.cs
- ElementProxy.cs
- PropertyBuilder.cs
- MenuItemStyle.cs
- safePerfProviderHandle.cs
- ReadOnlyHierarchicalDataSource.cs
- ResolveNameEventArgs.cs
- SchemaMapping.cs
- PublisherMembershipCondition.cs
- PropertyBuilder.cs
- BitmapImage.cs
- DataControlField.cs
- DataGridViewBand.cs
- DiscoveryClientRequestChannel.cs
- CustomValidator.cs
- PropertyInformation.cs
- ImageButton.cs
- OptimizedTemplateContent.cs
- XslCompiledTransform.cs
- EntityChangedParams.cs
- MenuStrip.cs
- InteropAutomationProvider.cs
- SqlWebEventProvider.cs
- FactoryRecord.cs
- UriTemplateMatchException.cs
- SequenceFullException.cs
- WindowsImpersonationContext.cs
- FilteredDataSetHelper.cs
- mediapermission.cs
- X509ThumbprintKeyIdentifierClause.cs
- PixelFormats.cs
- XmlSerializerNamespaces.cs
- EditorPartDesigner.cs
- LayoutSettings.cs
- Attachment.cs
- TemplateContentLoader.cs
- AnimatedTypeHelpers.cs
- ArrayTypeMismatchException.cs
- GeometryHitTestResult.cs
- SerializationIncompleteException.cs
- WhiteSpaceTrimStringConverter.cs
- QueryCorrelationInitializer.cs
- ExpressionEditorAttribute.cs
- ChannelManagerBase.cs
- ContentTextAutomationPeer.cs
- ProcessRequestArgs.cs
- SqlClientPermission.cs
- RowToParametersTransformer.cs
- DecoratedNameAttribute.cs
- ClearTypeHintValidation.cs
- DistinctQueryOperator.cs
- InstanceDataCollectionCollection.cs
- DocumentXmlWriter.cs
- CustomWebEventKey.cs
- ReservationCollection.cs
- X509Utils.cs
- ImageUrlEditor.cs
- TextOnlyOutput.cs
- CompositeDataBoundControl.cs
- XmlSchemaGroupRef.cs
- ResourceReferenceExpression.cs
- TextServicesCompartment.cs
- RoleGroupCollectionEditor.cs
- StringKeyFrameCollection.cs
- HttpClientCertificate.cs
- AttachInfo.cs
- AnchorEditor.cs
- Stroke2.cs
- TextBlock.cs
- Container.cs
- DataBindEngine.cs
- BaseValidatorDesigner.cs
- QilInvoke.cs
- SplitContainer.cs
- EndpointAddressProcessor.cs
- RemotingConfigParser.cs
- HitTestParameters.cs
- XPathNodeList.cs
- DependencyPropertyKind.cs
- TextSelectionProcessor.cs
- QueryResponse.cs
- OpCodes.cs
- DataGridViewButtonCell.cs
- ResourceDisplayNameAttribute.cs
- SystemWebExtensionsSectionGroup.cs
- Socket.cs
- X509SecurityTokenProvider.cs
- Vertex.cs
- SystemUnicastIPAddressInformation.cs
- RegularExpressionValidator.cs
- DataGridViewRowEventArgs.cs
- safesecurityhelperavalon.cs
- ImportDesigner.xaml.cs
- TTSEngineTypes.cs
- HashRepartitionEnumerator.cs
- Geometry.cs