Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / EntityDesignerUtils.cs / 1305376 / EntityDesignerUtils.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.IO; using System.Text; using System.Xml; using System.Data.Metadata.Edm; using System.Data.Mapping; namespace System.Data.Entity.Design { internal static class EntityDesignerUtils { private static readonly EFNamespaceSet v1Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2007/06/edmx", Csdl = XmlConstants.ModelNamespace_1, Msl = StorageMslConstructs.NamespaceUriV1, Ssdl = XmlConstants.TargetNamespace_1 }; private static readonly EFNamespaceSet v2Namespaces = new EFNamespaceSet { Edmx = "http://schemas.microsoft.com/ado/2008/10/edmx", Csdl = XmlConstants.ModelNamespace_2, Msl = StorageMslConstructs.NamespaceUriV2, Ssdl = XmlConstants.TargetNamespace_2 }; internal static readonly string _edmxFileExtension = ".edmx"; ////// Extract the Conceptual, Mapping and Storage nodes from an EDMX input streams, and extract the value of the metadataArtifactProcessing property. /// /// /// /// /// /// internal static void ExtractConceptualMappingAndStorageNodes(StreamReader edmxInputStream, out XmlElement conceptualSchemaNode, out XmlElement mappingNode, out XmlElement storageSchemaNode, out string metadataArtifactProcessingValue) { // load up an XML document representing the edmx file XmlDocument xmlDocument = new XmlDocument(); xmlDocument.Load(edmxInputStream); EFNamespaceSet set = v2Namespaces; if (xmlDocument.DocumentElement.NamespaceURI == v1Namespaces.Edmx) { set = v1Namespaces; } XmlNamespaceManager nsMgr = new XmlNamespaceManager(xmlDocument.NameTable); nsMgr.AddNamespace("edmx", set.Edmx); nsMgr.AddNamespace("edm", set.Csdl); nsMgr.AddNamespace("ssdl", set.Ssdl); nsMgr.AddNamespace("map", set.Msl); // find the ConceptualModel Schema node conceptualSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:ConceptualModels/edm:Schema", nsMgr); // find the StorageModel Schema node storageSchemaNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:StorageModels/ssdl:Schema", nsMgr); // find the Mapping node mappingNode = (XmlElement)xmlDocument.SelectSingleNode( "/edmx:Edmx/edmx:Runtime/edmx:Mappings/map:Mapping", nsMgr); // find the Connection node metadataArtifactProcessingValue = String.Empty; XmlNodeList connectionProperties = xmlDocument.SelectNodes( "/edmx:Edmx/edmx:Designer/edmx:Connection/edmx:DesignerInfoPropertySet/edmx:DesignerProperty", nsMgr); if (connectionProperties != null) { foreach (XmlNode propertyNode in connectionProperties) { foreach (XmlAttribute a in propertyNode.Attributes) { // treat attribute names case-sensitive (since it is xml), but attribute value case-insensitive to be accommodating . if (a.Name.Equals("Name", StringComparison.Ordinal) && a.Value.Equals("MetadataArtifactProcessing", StringComparison.OrdinalIgnoreCase)) { foreach (XmlAttribute a2 in propertyNode.Attributes) { if (a2.Name.Equals("Value", StringComparison.Ordinal)) { metadataArtifactProcessingValue = a2.Value; break; } } } } } } } // utility method to ensure an XmlElement (containing the C, M or S element // from the Edmx file) is sent out to a stream in the same format internal static void OutputXmlElementToStream(XmlElement xmlElement, Stream stream) { XmlWriterSettings settings = new XmlWriterSettings(); settings.Encoding = Encoding.UTF8; settings.Indent = true; // set up output document XmlDocument outputXmlDoc = new XmlDocument(); XmlNode importedElement = outputXmlDoc.ImportNode(xmlElement, true); outputXmlDoc.AppendChild(importedElement); // write out XmlDocument XmlWriter writer = null; try { writer = XmlWriter.Create(stream, settings); outputXmlDoc.WriteTo(writer); } finally { if (writer != null) { writer.Close(); } } } private struct EFNamespaceSet { public string Edmx; public string Csdl; public string Msl; public string Ssdl; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
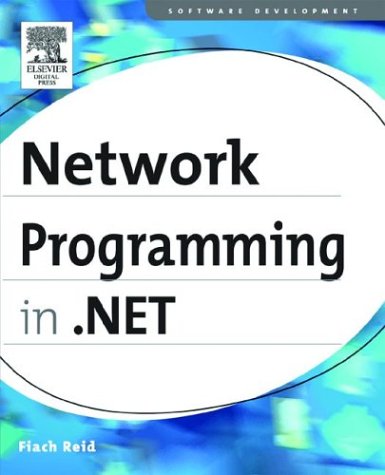
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RelatedEnd.cs
- TokenBasedSet.cs
- DataSourceCacheDurationConverter.cs
- AuthorizationRuleCollection.cs
- VirtualPathUtility.cs
- ClassImporter.cs
- WebPartAddingEventArgs.cs
- BStrWrapper.cs
- ServiceReference.cs
- VisualStyleInformation.cs
- VirtualPathUtility.cs
- AsymmetricSecurityProtocolFactory.cs
- AsymmetricSignatureFormatter.cs
- COM2AboutBoxPropertyDescriptor.cs
- OleDbException.cs
- NamedPipeChannelFactory.cs
- MimeMultiPart.cs
- UrlMappingsSection.cs
- ThemeDirectoryCompiler.cs
- RuleRef.cs
- ValueSerializerAttribute.cs
- PersonalizationProviderCollection.cs
- SlotInfo.cs
- Page.cs
- TracedNativeMethods.cs
- ToolStripGrip.cs
- Delegate.cs
- ToolBar.cs
- CallbackException.cs
- PrintPageEvent.cs
- TraceHandlerErrorFormatter.cs
- XmlReader.cs
- ToolStripPanelRow.cs
- Tag.cs
- SqlMultiplexer.cs
- HttpCapabilitiesEvaluator.cs
- AdornerHitTestResult.cs
- IdnElement.cs
- RichTextBox.cs
- SecurityManager.cs
- Tag.cs
- MailWebEventProvider.cs
- PropertyDescriptorCollection.cs
- SynchronizationLockException.cs
- DesignSurfaceEvent.cs
- XmlHelper.cs
- CodeStatement.cs
- HttpListenerRequest.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- SchemaElementDecl.cs
- LinkedResource.cs
- KnownTypesProvider.cs
- ApplicationSecurityInfo.cs
- ObjectDataSourceFilteringEventArgs.cs
- PerformanceCounterLib.cs
- RelationshipDetailsRow.cs
- IdentityNotMappedException.cs
- SmtpMail.cs
- FlowDocumentPage.cs
- CodeConditionStatement.cs
- Mouse.cs
- CacheOutputQuery.cs
- FontStretches.cs
- CultureInfoConverter.cs
- FileDialog_Vista_Interop.cs
- ObjectViewFactory.cs
- DiscoveryReference.cs
- XsdDateTime.cs
- FormView.cs
- NodeInfo.cs
- RectangleHotSpot.cs
- MatrixKeyFrameCollection.cs
- CommonDialog.cs
- ExternalDataExchangeService.cs
- MeasureItemEvent.cs
- GlyphRunDrawing.cs
- EncodingFallbackAwareXmlTextWriter.cs
- HTMLTagNameToTypeMapper.cs
- EventLevel.cs
- XmlStringTable.cs
- RuleAction.cs
- KeyPullup.cs
- DataStreams.cs
- SortFieldComparer.cs
- TogglePatternIdentifiers.cs
- BulletDecorator.cs
- InternalControlCollection.cs
- SoapTypeAttribute.cs
- SerializationStore.cs
- OneOfConst.cs
- BlockUIContainer.cs
- WorkerProcess.cs
- XmlConvert.cs
- FastEncoderStatics.cs
- OptionUsage.cs
- LocatorPart.cs
- DbExpressionRules.cs
- TimerElapsedEvenArgs.cs
- RegexRunnerFactory.cs
- SchemaImporter.cs