Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / Interop / Restrictions.cs / 1305376 / Restrictions.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging.Interop { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using Microsoft.Win32; internal class Restrictions { private MQRESTRICTION restrictionStructure; public const int PRLT = 0; public const int PRLE = 1; public const int PRGT = 2; public const int PRGE = 3; public const int PREQ = 4; public const int PRNE = 5; public Restrictions(int maxRestrictions) { this.restrictionStructure = new MQRESTRICTION(maxRestrictions); } public virtual void AddGuid(int propertyId, int op, Guid value) { IntPtr data = Marshal.AllocHGlobal(16); Marshal.Copy(value.ToByteArray(), 0 , data, 16); this.AddItem(propertyId, op, MessagePropertyVariants.VT_CLSID, data); } public virtual void AddGuid(int propertyId, int op) { IntPtr data = Marshal.AllocHGlobal(16); this.AddItem(propertyId, op, MessagePropertyVariants.VT_CLSID, data); } private void AddItem(int id,int op, short vt, IntPtr data) { Marshal.WriteInt32(restrictionStructure.GetNextValidPtr(0) , op); Marshal.WriteInt32(restrictionStructure.GetNextValidPtr(4), id); Marshal.WriteInt16(restrictionStructure.GetNextValidPtr(8), vt); Marshal.WriteInt16(restrictionStructure.GetNextValidPtr(10), (short)0); Marshal.WriteInt16(restrictionStructure.GetNextValidPtr(12), (short)0); Marshal.WriteInt16(restrictionStructure.GetNextValidPtr(14), (short)0); Marshal.WriteIntPtr(restrictionStructure.GetNextValidPtr(16), data); Marshal.WriteIntPtr(restrictionStructure.GetNextValidPtr(16 + IntPtr.Size), (IntPtr)0); ++restrictionStructure.restrictionCount; } public virtual void AddI4(int propertyId, int op, int value) { this.AddItem(propertyId, op, MessagePropertyVariants.VT_I4, (IntPtr)value); } public virtual void AddString(int propertyId, int op, string value) { if (value == null) this.AddItem(propertyId, op, MessagePropertyVariants.VT_NULL, (IntPtr)0); else { IntPtr data = Marshal.StringToHGlobalUni(value); this.AddItem(propertyId, op, MessagePropertyVariants.VT_LPWSTR, data); } } public virtual MQRESTRICTION GetRestrictionsRef() { return this.restrictionStructure; } [StructLayout(LayoutKind.Sequential)] public class MQRESTRICTION { public int restrictionCount; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr restrinctions; public MQRESTRICTION(int maxCount) { this.restrinctions = Marshal.AllocHGlobal(maxCount * GetRestrictionSize()); } ~MQRESTRICTION() { if (this.restrinctions != (IntPtr)0) { for (int index= 0; index < this.restrictionCount; ++ index) { short vt = Marshal.ReadInt16((IntPtr)((long)this.restrinctions + (index * GetRestrictionSize()) + 8)); if (vt != MessagePropertyVariants.VT_I4) { IntPtr dataPtr = (IntPtr)((long)this.restrinctions + (index * GetRestrictionSize()) + 16); IntPtr data = Marshal.ReadIntPtr(dataPtr); Marshal.FreeHGlobal(data); } } Marshal.FreeHGlobal(this.restrinctions); this.restrinctions = (IntPtr)0; } } public IntPtr GetNextValidPtr(int offset) { return (IntPtr)((long)restrinctions + restrictionCount * GetRestrictionSize() + offset); } public static int GetRestrictionSize() { return 16 + (IntPtr.Size * 2); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
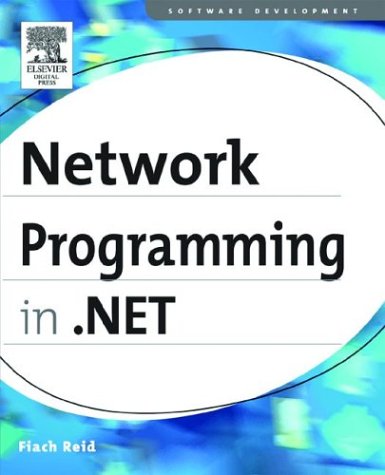
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScrollPattern.cs
- DescendantOverDescendantQuery.cs
- SudsWriter.cs
- XmlSchemaAnyAttribute.cs
- _SingleItemRequestCache.cs
- Variant.cs
- DesignTimeParseData.cs
- FormViewPageEventArgs.cs
- QueryUtil.cs
- AuthorizationSection.cs
- DataGridViewColumnCollectionEditor.cs
- OperatingSystem.cs
- OleDbConnectionInternal.cs
- ToolStrip.cs
- VectorValueSerializer.cs
- JapaneseLunisolarCalendar.cs
- DictionaryKeyPropertyAttribute.cs
- WindowsToolbar.cs
- TreeBuilderBamlTranslator.cs
- GifBitmapDecoder.cs
- StylusPointCollection.cs
- ScalarOps.cs
- DatatypeImplementation.cs
- SqlRowUpdatingEvent.cs
- UnsafeNativeMethods.cs
- AnnotationResourceCollection.cs
- XmlSchemaObject.cs
- PolicyVersionConverter.cs
- MsmqIntegrationProcessProtocolHandler.cs
- ScriptResourceHandler.cs
- HtmlWindowCollection.cs
- PreviewKeyDownEventArgs.cs
- AuthenticationServiceManager.cs
- TableProviderWrapper.cs
- TraceHwndHost.cs
- SHA1Managed.cs
- RowType.cs
- WorkflowOperationBehavior.cs
- ActivityStateQuery.cs
- GeometryCombineModeValidation.cs
- XmlSchemaProviderAttribute.cs
- DesignerSerializerAttribute.cs
- WebPartUserCapability.cs
- MailWebEventProvider.cs
- FileDialogCustomPlace.cs
- RuntimeConfigurationRecord.cs
- ReversePositionQuery.cs
- TextEditorMouse.cs
- NativeWrapper.cs
- DesignerDataSourceView.cs
- HScrollProperties.cs
- BrushMappingModeValidation.cs
- CanExecuteRoutedEventArgs.cs
- MethodBody.cs
- SplitterCancelEvent.cs
- Statements.cs
- XamlPoint3DCollectionSerializer.cs
- mediaclock.cs
- FontFamilyConverter.cs
- DateTimeUtil.cs
- MobileResource.cs
- LayoutSettings.cs
- MobileListItem.cs
- XmlElementAttribute.cs
- NamedPipeConnectionPool.cs
- IIS7WorkerRequest.cs
- RuntimeConfigLKG.cs
- ProxyAttribute.cs
- Latin1Encoding.cs
- WindowsScrollBarBits.cs
- MemoryPressure.cs
- QueryOutputWriter.cs
- DataGridViewRowsRemovedEventArgs.cs
- ApplicationSettingsBase.cs
- SequentialUshortCollection.cs
- ProviderCollection.cs
- InfiniteIntConverter.cs
- TaskExceptionHolder.cs
- ApplicationInfo.cs
- PackageRelationship.cs
- HostUtils.cs
- WebPartConnectionsCancelEventArgs.cs
- MulticastNotSupportedException.cs
- OutputCacheProfile.cs
- Wildcard.cs
- SecureEnvironment.cs
- CustomTypeDescriptor.cs
- ILGenerator.cs
- SafeRightsManagementEnvironmentHandle.cs
- SelectedDatesCollection.cs
- QilBinary.cs
- WindowsSlider.cs
- WinEventHandler.cs
- OleDbParameterCollection.cs
- AssemblyBuilder.cs
- UnsupportedPolicyOptionsException.cs
- WebPartDisplayModeCancelEventArgs.cs
- InlineCollection.cs
- TrackBarRenderer.cs
- CompiledQuery.cs