Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / IlGen / TailCallAnalyzer.cs / 1305376 / TailCallAnalyzer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Xml.Xsl.Qil; namespace System.Xml.Xsl.IlGen { ////// This analyzer walks each function in the graph and annotates Invoke nodes which can /// be compiled using the IL .tailcall instruction. This instruction will discard the /// current stack frame before calling the new function. /// internal static class TailCallAnalyzer { ////// Perform tail-call analysis on the functions in the specified QilExpression. /// public static void Analyze(QilExpression qil) { foreach (QilFunction ndFunc in qil.FunctionList) { // Only analyze functions which are pushed to the writer, since otherwise code // is generated after the call instruction in order to process cached results if (XmlILConstructInfo.Read(ndFunc).ConstructMethod == XmlILConstructMethod.Writer) AnalyzeDefinition(ndFunc.Definition); } } ////// Recursively analyze the definition of a function. /// private static void AnalyzeDefinition(QilNode nd) { Debug.Assert(XmlILConstructInfo.Read(nd).PushToWriterLast, "Only need to analyze expressions which will be compiled in push mode."); switch (nd.NodeType) { case QilNodeType.Invoke: // Invoke node can either be compiled as IteratorThenWriter, or Writer. // Since IteratorThenWriter involves caching the results of the function call // and iterating over them, .tailcall cannot be used if (XmlILConstructInfo.Read(nd).ConstructMethod == XmlILConstructMethod.Writer) OptimizerPatterns.Write(nd).AddPattern(OptimizerPatternName.TailCall); break; case QilNodeType.Loop: { // Recursively analyze Loop return value QilLoop ndLoop = (QilLoop) nd; if (ndLoop.Variable.NodeType == QilNodeType.Let || !ndLoop.Variable.Binding.XmlType.MaybeMany) AnalyzeDefinition(ndLoop.Body); break; } case QilNodeType.Sequence: { // Recursively analyze last expression in Sequence QilList ndSeq = (QilList) nd; if (ndSeq.Count > 0) AnalyzeDefinition(ndSeq[ndSeq.Count - 1]); break; } case QilNodeType.Choice: { // Recursively analyze Choice branches QilChoice ndChoice = (QilChoice) nd; for (int i = 0; i < ndChoice.Branches.Count; i++) AnalyzeDefinition(ndChoice.Branches[i]); break; } case QilNodeType.Conditional: { // Recursively analyze Conditional branches QilTernary ndCond = (QilTernary) nd; AnalyzeDefinition(ndCond.Center); AnalyzeDefinition(ndCond.Right); break; } case QilNodeType.Nop: AnalyzeDefinition(((QilUnary) nd).Child); break; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
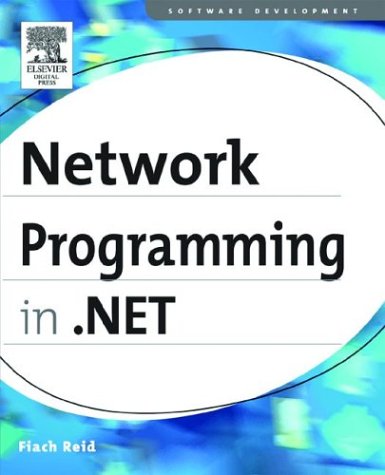
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ModuleElement.cs
- DrawingBrush.cs
- ClonableStack.cs
- PauseStoryboard.cs
- DialogResultConverter.cs
- ImageFormat.cs
- StringFunctions.cs
- SqlDataSourceCommandEventArgs.cs
- ClassicBorderDecorator.cs
- XmlSchemaFacet.cs
- Message.cs
- BounceEase.cs
- CodeDelegateInvokeExpression.cs
- FixedBufferAttribute.cs
- ContentType.cs
- SiteMapDataSourceView.cs
- HitTestFilterBehavior.cs
- ObjectTypeMapping.cs
- UserPreferenceChangingEventArgs.cs
- HostedTcpTransportManager.cs
- GridLength.cs
- HtmlForm.cs
- DataGridViewAccessibleObject.cs
- EventBookmark.cs
- InternalConfigRoot.cs
- ConsoleKeyInfo.cs
- ExpressionVisitorHelpers.cs
- DoubleKeyFrameCollection.cs
- BinaryObjectReader.cs
- ContentTextAutomationPeer.cs
- Normalization.cs
- BitmapMetadataEnumerator.cs
- CommonRemoteMemoryBlock.cs
- ProcessDesigner.cs
- SynthesizerStateChangedEventArgs.cs
- ValidationErrorCollection.cs
- TextTreeUndo.cs
- DictionaryBase.cs
- Debugger.cs
- SecureUICommand.cs
- FaultDescription.cs
- ComponentChangedEvent.cs
- ObfuscateAssemblyAttribute.cs
- SqlNotificationEventArgs.cs
- ConditionalDesigner.cs
- TypeLoadException.cs
- DesignTimeSiteMapProvider.cs
- FormattedText.cs
- PartialList.cs
- SingleResultAttribute.cs
- ProcessModelSection.cs
- XmlChildEnumerator.cs
- SocketPermission.cs
- TransformedBitmap.cs
- ChildDocumentBlock.cs
- DefaultExpressionVisitor.cs
- ReflectionPermission.cs
- DateTimeFormat.cs
- ScrollableControl.cs
- MobileFormsAuthentication.cs
- SessionStateItemCollection.cs
- AbstractExpressions.cs
- ServiceConfigurationTraceRecord.cs
- CodeBlockBuilder.cs
- OracleBoolean.cs
- DefaultValueAttribute.cs
- InkCanvasSelection.cs
- ColorAnimation.cs
- MetadataItemSerializer.cs
- SqlBooleanMismatchVisitor.cs
- CompositeScriptReferenceEventArgs.cs
- MasterPageParser.cs
- WebPartTracker.cs
- Options.cs
- QueryResultOp.cs
- DataControlFieldCell.cs
- ConnectorMovedEventArgs.cs
- EdmConstants.cs
- String.cs
- TextPattern.cs
- infer.cs
- MetricEntry.cs
- ButtonStandardAdapter.cs
- FlowNode.cs
- ClaimTypes.cs
- TemplateEditingService.cs
- UserControl.cs
- NativeActivity.cs
- OdbcEnvironment.cs
- FloaterBaseParagraph.cs
- VisualBrush.cs
- DynamicControl.cs
- NativeMethods.cs
- SecureUICommand.cs
- SQLBytes.cs
- UnsafeNativeMethods.cs
- ReadOnlyDataSourceView.cs
- SqlBinder.cs
- RecognizerBase.cs
- GeneralTransform3DTo2DTo3D.cs