Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / XmlUtils / System / Xml / Xsl / QIL / QilNode.cs / 1305376 / QilNode.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Globalization; using System.Xml; using System.Xml.Schema; using System.Xml.Xsl; namespace System.Xml.Xsl.Qil { ////// A node in the QIL tree. /// ////// Don't construct QIL nodes directly; instead, use the internal class QilNode : IListQilFactory . /// This base internal class is not abstract and may be instantiated in some cases (for example, true/false boolean literals). ///{ protected QilNodeType nodeType; protected XmlQueryType xmlType; protected ISourceLineInfo sourceLine; protected object annotation; //----------------------------------------------- // Constructor //----------------------------------------------- /// /// Construct a new node /// public QilNode(QilNodeType nodeType) { this.nodeType = nodeType; } ////// Construct a new node /// public QilNode(QilNodeType nodeType, XmlQueryType xmlType) { this.nodeType = nodeType; this.xmlType = xmlType; } //----------------------------------------------- // QilNode methods //----------------------------------------------- ////// Access the QIL node type. /// public QilNodeType NodeType { get { return this.nodeType; } set { this.nodeType = value; } } ////// Access the QIL type. /// public virtual XmlQueryType XmlType { get { return this.xmlType; } set { this.xmlType = value; } } ////// Line info information for tools support. /// public ISourceLineInfo SourceLine { get { return this.sourceLine; } set { this.sourceLine = value; } } ////// Access an annotation which may have been attached to this node. /// public object Annotation { get { return this.annotation; } set { this.annotation = value; } } ////// Create a new deep copy of this node. /// public virtual QilNode DeepClone(QilFactory f) { return new QilCloneVisitor(f).Clone(this); } ////// Create a shallow copy of this node, copying all the fields. /// public virtual QilNode ShallowClone(QilFactory f) { QilNode n = (QilNode) MemberwiseClone(); f.TraceNode(n); return n; } //----------------------------------------------- // IListmethods -- override //----------------------------------------------- public virtual int Count { get { return 0; } } public virtual QilNode this[int index] { get { throw new IndexOutOfRangeException(); } set { throw new IndexOutOfRangeException(); } } public virtual void Insert(int index, QilNode node) { throw new NotSupportedException(); } public virtual void RemoveAt(int index) { throw new NotSupportedException(); } //----------------------------------------------- // IList methods -- no need to override //----------------------------------------------- public IEnumerator GetEnumerator() { return new IListEnumerator (this); } IEnumerator IEnumerable.GetEnumerator() { return new IListEnumerator (this); } public virtual bool IsReadOnly { get { return false; } } public virtual void Add(QilNode node) { Insert(Count, node); } public virtual void Add(IList list) { for (int i = 0; i < list.Count; i++) Insert(Count, list[i]); } public virtual void Clear() { for (int index = Count - 1; index >= 0; index--) RemoveAt(index); } public virtual bool Contains(QilNode node) { return IndexOf(node) != -1; } public virtual void CopyTo(QilNode[] array, int index) { for (int i = 0; i < Count; i++) array[index + i] = this[i]; } public virtual bool Remove(QilNode node) { int index = IndexOf(node); if (index >= 0) { RemoveAt(index); return true; } return false; } public virtual int IndexOf(QilNode node) { for (int i = 0; i < Count; i++) if (node.Equals(this[i])) return i; return -1; } //----------------------------------------------- // Debug //----------------------------------------------- #if QIL_TRACE_NODE_CREATION private int nodeId; private string nodeLoc; public int NodeId { get { return nodeId; } set { nodeId = value; } } public string NodeLocation { get { return nodeLoc; } set { nodeLoc = value; } } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
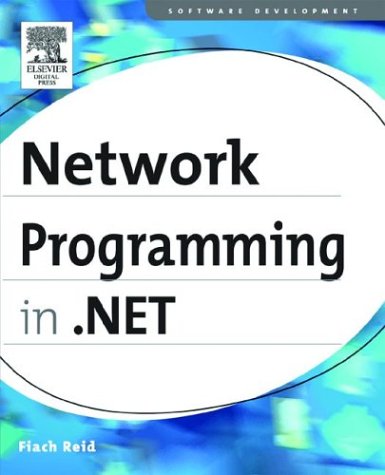
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RichTextBoxConstants.cs
- XmlJsonReader.cs
- SiteMapNode.cs
- BoundField.cs
- SafeRightsManagementSessionHandle.cs
- SQLResource.cs
- OperatorExpressions.cs
- TransactionProtocol.cs
- HttpCachePolicy.cs
- ChangeTracker.cs
- ApplicationDirectory.cs
- XslCompiledTransform.cs
- Grid.cs
- TraceHandler.cs
- ClientCultureInfo.cs
- PublishLicense.cs
- Rect3DConverter.cs
- WorkflowWebHostingModule.cs
- SqlDataSourceSelectingEventArgs.cs
- HttpServerUtilityWrapper.cs
- ValidationErrorEventArgs.cs
- DropShadowEffect.cs
- BaseDataBoundControl.cs
- EventRouteFactory.cs
- EntityParameterCollection.cs
- diagnosticsswitches.cs
- FeatureAttribute.cs
- LabelExpression.cs
- WindowsUpDown.cs
- IMembershipProvider.cs
- GridViewRowCollection.cs
- FontStretch.cs
- RangeValidator.cs
- RepeaterItemEventArgs.cs
- DataGridAddNewRow.cs
- MarkupExtensionReturnTypeAttribute.cs
- FrugalMap.cs
- PropertyTabAttribute.cs
- TextEndOfParagraph.cs
- UnsettableComboBox.cs
- LinqMaximalSubtreeNominator.cs
- GraphicsState.cs
- DeadCharTextComposition.cs
- BitmapMetadataEnumerator.cs
- DataGridViewComponentPropertyGridSite.cs
- HuffModule.cs
- XmlText.cs
- RelationshipWrapper.cs
- FirstMatchCodeGroup.cs
- WebResponse.cs
- DataAdapter.cs
- DiscoveryServiceExtension.cs
- WorkflowServiceHost.cs
- GregorianCalendarHelper.cs
- Module.cs
- DataViewManagerListItemTypeDescriptor.cs
- ProcessManager.cs
- _ScatterGatherBuffers.cs
- KeyEventArgs.cs
- DataTableMappingCollection.cs
- WindowsAuthenticationEventArgs.cs
- PermissionListSet.cs
- Normalizer.cs
- WebPartManagerInternals.cs
- DesignerProperties.cs
- XhtmlBasicSelectionListAdapter.cs
- MouseGesture.cs
- AudioFormatConverter.cs
- InheritanceContextHelper.cs
- Light.cs
- SocketAddress.cs
- SqlXmlStorage.cs
- ACE.cs
- dbdatarecord.cs
- FieldMetadata.cs
- GridLengthConverter.cs
- ResizeGrip.cs
- MenuItemBindingCollection.cs
- SystemIPv4InterfaceProperties.cs
- MessageSmuggler.cs
- CreateUserErrorEventArgs.cs
- DataColumnMapping.cs
- GcHandle.cs
- FlowDecisionDesigner.xaml.cs
- DbConnectionPoolOptions.cs
- WindowsImpersonationContext.cs
- DesignTimeTemplateParser.cs
- ItemsControl.cs
- iisPickupDirectory.cs
- CSharpCodeProvider.cs
- StrokeNodeData.cs
- HiddenFieldDesigner.cs
- LockedHandleGlyph.cs
- TdsValueSetter.cs
- ItemContainerGenerator.cs
- UnsafeNativeMethods.cs
- SplitContainer.cs
- DriveInfo.cs
- EndPoint.cs
- KnownIds.cs