Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / Expression.cs / 1305600 / Expression.cs
using System; using System.ComponentModel; using MS.Internal.WindowsBase; namespace System.Windows { ////// Modes for expressions /// //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] internal enum ExpressionMode { ////// No options /// None = 0, ////// Expression may not be set in multiple places /// ////// Even if a non-shareable Expression has been attached and /// then detached, it still may not be reused /// NonSharable, ////// Expression forwards invalidations to the property on which it is set. /// ////// This option implies ForwardsInvalidations, ///as well. /// Whenever the expression is notified of an invalidation of one /// of its sources via OnPropertyInvalidation, it /// promises to invalidate the property on which it is set, so the /// property engine doesn't have to. /// /// The property engine does not /// need to keep a reference to the target /// in this case, which can allow the target to be garbage-collected /// when it is no longer in use. /// /// Expression supports DependencySources on a different Dispatcher. /// ////// This option implies SupportsUnboundSources } ///as well. /// When set, it suppresses the property engine's check that the /// DependencyObject to which the expression is attached belongs to /// the same Thread as all its DependencySources, and allows /// OnPropertyInvalidation notifications to arrive on the "wrong" /// Thread. It is the expression's responsibility to handle these /// correctly, typically by marshalling them to the right Thread. /// Note: The check is only suppressed when the source isn't owned /// by any Thread (i.e. source.Dispatcher == null). /// /// Expressions are used to define dependencies between properties /// ////// When a property's computed value is no longer considered valid, the /// property must be invalidated. The property engine propagates these /// invalidations to all dependents. //CASRemoval:[StrongNameIdentityPermission(SecurityAction.LinkDemand, PublicKey = Microsoft.Internal.BuildInfo.WCP_PUBLIC_KEY_STRING)] [TypeConverter(typeof(ExpressionConverter))] public class Expression { ////// /// An Expression can be set per-instance per-property via SetValue. /// /// Expression construction /// internal Expression() : this(ExpressionMode.None) { } ////// Expression construction /// internal Expression(ExpressionMode mode) { _flags = 0; switch(mode) { case ExpressionMode.None: break; case ExpressionMode.NonSharable: _flags |= InternalFlags.NonShareable; break; case ExpressionMode.ForwardsInvalidations: _flags |= InternalFlags.ForwardsInvalidations; _flags |= InternalFlags.NonShareable; break; case ExpressionMode.SupportsUnboundSources: _flags |= InternalFlags.ForwardsInvalidations; _flags |= InternalFlags.NonShareable; _flags |= InternalFlags.SupportsUnboundSources; break; default: throw new ArgumentException(SR.Get(SRID.UnknownExpressionMode)); } } // We need this Clone method to copy a binding during Freezable.Copy. We shouldn't be taking // the target object/dp parameters here, but Binding.ProvideValue requires it. (Binding // could probably be re-factored so that we don't need this). [FriendAccessAllowed] // Used by Freezables internal virtual Expression Copy( DependencyObject targetObject, DependencyProperty targetDP ) { // By default, just use the same copy. return this; } ////// List of sources of the Expression /// ///Sources list internal virtual DependencySource[] GetSources() { return null; } ////// Called to evaluate the Expression value /// /// DependencyObject being queried /// Property being queried ///Computed value. Unset if unavailable. internal virtual object GetValue(DependencyObject d, DependencyProperty dp) { return DependencyProperty.UnsetValue; } ////// Allows Expression to store set values /// /// DependencyObject being set /// Property being set /// Value being set ///true if Expression handled storing of the value internal virtual bool SetValue(DependencyObject d, DependencyProperty dp, object value) { return false; } ////// Notification that the Expression has been set as a property's value /// /// DependencyObject being set /// Property being set internal virtual void OnAttach(DependencyObject d, DependencyProperty dp) { } ////// Notification that the Expression has been removed as a property's value /// /// DependencyObject being cleared /// Property being cleared internal virtual void OnDetach(DependencyObject d, DependencyProperty dp) { } ////// Notification that a Dependent that this Expression established has /// been invalidated as a result of a Source invalidation /// /// DependencyObject that was invalidated /// Changed event args for the property that was invalidated internal virtual void OnPropertyInvalidation(DependencyObject d, DependencyPropertyChangedEventArgs args) { } ////// Dynamically change this Expression sources (availiable only for NonShareable /// Expressions) /// ////// Expression must be in use on provided DependencyObject/DependencyProperty. /// GetSources must reflect the old sources to be replaced by the provided newSources. /// /// DependencyObject whose sources are to be updated /// The property that the Expression is set to /// New sources internal void ChangeSources(DependencyObject d, DependencyProperty dp, DependencySource[] newSources) { if (d == null && !ForwardsInvalidations) { throw new ArgumentNullException("d"); } if (dp == null && !ForwardsInvalidations) { throw new ArgumentNullException("dp"); } if (Shareable) { throw new InvalidOperationException(SR.Get(SRID.ShareableExpressionsCannotChangeSources)); } DependencyObject.ValidateSources(d, newSources, this); // Additional validation in callee if (ForwardsInvalidations) { DependencyObject.ChangeExpressionSources(this, null, null, newSources); } else { DependencyObject.ChangeExpressionSources(this, d, dp, newSources); } } // Determines if Expression can be attached: // 1) If Shareable // 2) If NonShareable and not HasBeenAttached internal bool Attachable { // Once a NonShareable has been Attached, it can't be used anywhere // else again (even if it's been Detached) get { return Shareable || !HasBeenAttached; } } internal bool Shareable { get { return (_flags & InternalFlags.NonShareable) == 0; } } internal bool ForwardsInvalidations { get { return (_flags & InternalFlags.ForwardsInvalidations) != 0; } } internal bool SupportsUnboundSources { get { return (_flags & InternalFlags.SupportsUnboundSources) != 0; } } internal bool HasBeenAttached { get { return (_flags & InternalFlags.Attached) != 0; } } internal bool HasBeenDetached { get { return (_flags & InternalFlags.Detached) != 0; } } internal void MarkAttached() { _flags |= InternalFlags.Attached; } internal void MarkDetached() { _flags |= InternalFlags.Detached; } ////// Expression.GetValue can return NoValue to indicate that /// the property engine should obtain the value some other way. /// [FriendAccessAllowed] internal static readonly object NoValue = new object(); private InternalFlags _flags; [Flags] private enum InternalFlags { None = 0x0, NonShareable = 0x1, ForwardsInvalidations = 0x2, SupportsUnboundSources = 0x4, Attached = 0x8, Detached = 0x10, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
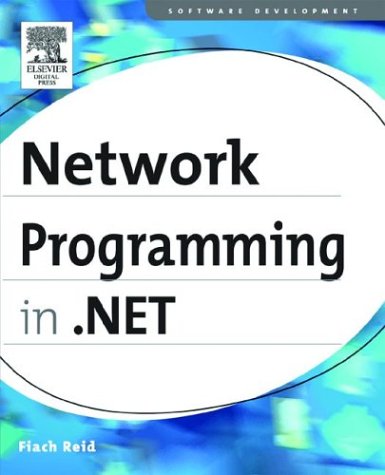
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InitiatorServiceModelSecurityTokenRequirement.cs
- TextFormatter.cs
- DataGridViewCellStyleConverter.cs
- UiaCoreTypesApi.cs
- DocumentViewer.cs
- SqlErrorCollection.cs
- ActivationArguments.cs
- TcpDuplicateContext.cs
- ClassicBorderDecorator.cs
- PackagePartCollection.cs
- EditorAttribute.cs
- VectorCollectionConverter.cs
- DataRecordInternal.cs
- Polygon.cs
- ValueTypePropertyReference.cs
- Switch.cs
- BufferModeSettings.cs
- StrokeCollection2.cs
- SpecularMaterial.cs
- ValidatorAttribute.cs
- ViewCellRelation.cs
- FixedPageProcessor.cs
- OdbcFactory.cs
- ArrayTypeMismatchException.cs
- DurableOperationContext.cs
- DoubleAnimationClockResource.cs
- MobileComponentEditorPage.cs
- nulltextcontainer.cs
- LocalValueEnumerator.cs
- StaticResourceExtension.cs
- CrossAppDomainChannel.cs
- ArrangedElementCollection.cs
- NavigateEvent.cs
- XmlRawWriter.cs
- GroupQuery.cs
- EventSourceCreationData.cs
- ToolBarOverflowPanel.cs
- Avt.cs
- DefaultValueMapping.cs
- SessionStateSection.cs
- EventEntry.cs
- StylusShape.cs
- ProtocolElementCollection.cs
- NativeMethods.cs
- PipelineModuleStepContainer.cs
- RegexCapture.cs
- D3DImage.cs
- XmlSchemaDocumentation.cs
- TypeConverter.cs
- CookielessHelper.cs
- Viewport3DVisual.cs
- DataGridColumnHeadersPresenterAutomationPeer.cs
- OutputBuffer.cs
- Vector3D.cs
- ValidationEventArgs.cs
- AnimatedTypeHelpers.cs
- CqlGenerator.cs
- TextHidden.cs
- SizeAnimation.cs
- CacheRequest.cs
- CompressionTransform.cs
- ExceptionRoutedEventArgs.cs
- Popup.cs
- Utils.cs
- ImageListUtils.cs
- FillBehavior.cs
- CellLabel.cs
- MultipleViewPatternIdentifiers.cs
- _DynamicWinsockMethods.cs
- LayoutInformation.cs
- DataViewManager.cs
- ExpressionVisitor.cs
- SecurityContextSecurityTokenParameters.cs
- loginstatus.cs
- TableLayoutPanelCellPosition.cs
- PersonalizableAttribute.cs
- ItemsControlAutomationPeer.cs
- TemplatePagerField.cs
- ButtonAutomationPeer.cs
- BitmapSourceSafeMILHandle.cs
- ExcludeFromCodeCoverageAttribute.cs
- CellParaClient.cs
- SessionStateItemCollection.cs
- validationstate.cs
- SequenceRange.cs
- ResourceAttributes.cs
- XmlSchemaSequence.cs
- ClientSponsor.cs
- LookupBindingPropertiesAttribute.cs
- StackSpiller.Generated.cs
- ProfilePropertyNameValidator.cs
- ActionNotSupportedException.cs
- CompiledQueryCacheKey.cs
- BinaryCommonClasses.cs
- ErrorTableItemStyle.cs
- Win32MouseDevice.cs
- MappingSource.cs
- PropertyInfo.cs
- MimeWriter.cs
- SimpleLine.cs