Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Navigation / JournalEntryListConverter.cs / 1 / JournalEntryListConverter.cs
//---------------------------------------------------------------------------- // // File: JournalConverter.cs // // Description: Implements the Converter to limit journal views to 9 items. // // Created: 1/31/05 by [....] // // Copyright (C) 2005 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- // Disable unknown #pragma warning for pragmas we use to exclude certain PreSharp violations #pragma warning disable 1634, 1691 using System; using System.Collections; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Windows.Data; using MS.Internal; namespace System.Windows.Navigation { ////// This class returns an IEnumerable that is limited in length - it is used by the NavigationWindow /// style to limit how many entries are shown in the back and forward drop down buttons. Not /// intended to be used in any other situations. /// public sealed class JournalEntryListConverter : IValueConverter { ////// This method from IValueConverter returns an IEnumerable which in turn will yield the /// ViewLimit limited collection of journal back and forward stack entries. /// public object Convert(object value, Type targetType, object parameter, CultureInfo culture) { #pragma warning disable 6506 return (value != null) ? ((JournalEntryStack)value).GetLimitedJournalEntryStackEnumerable() : null; #pragma warning restore 6506 } ////// This method from IValueConverter returns an IEnumerable which was originally passed to Convert /// public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture) { return Binding.DoNothing; } } ////// Describes the position of the journal entry relative to the current page. /// public enum JournalEntryPosition { ////// The entry is on the BackStack /// Back, ////// The current page /// Current, ////// The entry on the ForwardStack /// Forward, } ////// Puts all of the journal entries into a single list, for an IE7-style menu. /// public sealed class JournalEntryUnifiedViewConverter : IMultiValueConverter { ////// The DependencyProperty for the JournalEntryPosition property. /// public static readonly DependencyProperty JournalEntryPositionProperty = DependencyProperty.RegisterAttached( "JournalEntryPosition", typeof(JournalEntryPosition), typeof(JournalEntryUnifiedViewConverter), new PropertyMetadata(JournalEntryPosition.Current)); ////// Helper for reading the JournalEntryPosition property. /// public static JournalEntryPosition GetJournalEntryPosition(DependencyObject element) { if (element == null) { throw new ArgumentNullException("element"); } return ((JournalEntryPosition)element.GetValue(JournalEntryPositionProperty)); } ////// Helper for setting the JournalEntryPosition property. /// public static void SetJournalEntryPosition(DependencyObject element, JournalEntryPosition position) { if (element == null) { throw new ArgumentNullException("element"); } element.SetValue(JournalEntryPositionProperty, position); } ////// This method from IValueConverter returns an IEnumerable which in turn will yield the /// single list containing all of the menu items. /// public object Convert(object[] values, Type targetType, object parameter, System.Globalization.CultureInfo culture) { if (values != null && values.Length == 2) { JournalEntryStack backStack = values[0] as JournalEntryStack; JournalEntryStack forwardStack = values[1] as JournalEntryStack; if (backStack != null && forwardStack != null) { LimitedJournalEntryStackEnumerable limitedBackStack = (LimitedJournalEntryStackEnumerable)backStack.GetLimitedJournalEntryStackEnumerable(); LimitedJournalEntryStackEnumerable limitedForwardStack = (LimitedJournalEntryStackEnumerable)forwardStack.GetLimitedJournalEntryStackEnumerable(); return new UnifiedJournalEntryStackEnumerable(limitedBackStack, limitedForwardStack); } } return null; } ////// This method is unused. /// public object[] ConvertBack(object value, Type[] targetTypes, object parameter, System.Globalization.CultureInfo culture) { return new object[] { Binding.DoNothing }; } } // Merges LimitedBack and Forward Stack into one list and internal class UnifiedJournalEntryStackEnumerable : IEnumerable, INotifyCollectionChanged { internal UnifiedJournalEntryStackEnumerable(LimitedJournalEntryStackEnumerable backStack, LimitedJournalEntryStackEnumerable forwardStack) { _backStack = backStack; _backStack.CollectionChanged += new NotifyCollectionChangedEventHandler(StacksChanged); _forwardStack = forwardStack; _forwardStack.CollectionChanged += new NotifyCollectionChangedEventHandler(StacksChanged); } public IEnumerator GetEnumerator() { if (_items == null) { // Reserve space so this will not have to reallocate. The most it will ever be is // 9 for the forward stack, 9 for the back stack, 1 for the title bar _items = new ArrayList(19); // Add ForwardStack in reverse order foreach (JournalEntry o in _forwardStack) { _items.Insert(0, o); JournalEntryUnifiedViewConverter.SetJournalEntryPosition(o, JournalEntryPosition.Forward); } DependencyObject current = new DependencyObject(); current.SetValue(JournalEntry.NameProperty, SR.Get(SRID.NavWindowMenuCurrentPage)); // "Current Page" _items.Add(current); foreach (JournalEntry o in _backStack) { _items.Add(o); JournalEntryUnifiedViewConverter.SetJournalEntryPosition(o, JournalEntryPosition.Back); } } return _items.GetEnumerator(); } internal void StacksChanged(object sender, NotifyCollectionChangedEventArgs e) { _items = null; if (CollectionChanged != null) { CollectionChanged(this, new NotifyCollectionChangedEventArgs(NotifyCollectionChangedAction.Reset)); } } public event NotifyCollectionChangedEventHandler CollectionChanged; private LimitedJournalEntryStackEnumerable _backStack, _forwardStack; private ArrayList _items; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
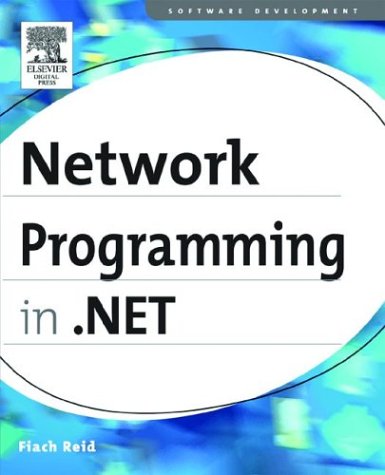
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MultiSelector.cs
- FormViewPagerRow.cs
- SmiSettersStream.cs
- Utils.cs
- DependencyPropertyHelper.cs
- WindowsRichEditRange.cs
- CellTreeNodeVisitors.cs
- MultiBindingExpression.cs
- EventEntry.cs
- Page.cs
- DiscoveryMessageSequenceCD1.cs
- ResolveNextArgumentWorkItem.cs
- AutomationFocusChangedEventArgs.cs
- OleDbStruct.cs
- UITypeEditor.cs
- EnvelopedPkcs7.cs
- Encoder.cs
- SmuggledIUnknown.cs
- SamlEvidence.cs
- MouseBinding.cs
- MailBnfHelper.cs
- SimpleMailWebEventProvider.cs
- _NestedSingleAsyncResult.cs
- TextSelection.cs
- OrderByQueryOptionExpression.cs
- HostedTransportConfigurationBase.cs
- CompositeScriptReference.cs
- FullTextLine.cs
- BindingElement.cs
- CustomAttributeSerializer.cs
- EventHandlerList.cs
- RelationshipFixer.cs
- MulticastIPAddressInformationCollection.cs
- DefaultProxySection.cs
- WebScriptServiceHost.cs
- RequiredAttributeAttribute.cs
- ForEachAction.cs
- util.cs
- ExpressionBuilderContext.cs
- SpellerInterop.cs
- ImpersonateTokenRef.cs
- ResourceKey.cs
- PreservationFileWriter.cs
- ContactManager.cs
- DataGridTable.cs
- GroupByQueryOperator.cs
- Vector3DIndependentAnimationStorage.cs
- WindowsSecurityTokenAuthenticator.cs
- NetSectionGroup.cs
- RequestQueue.cs
- ControlCommandSet.cs
- Int32Converter.cs
- BulletDecorator.cs
- BitmapSource.cs
- AndAlso.cs
- SqlFlattener.cs
- XmlComment.cs
- ContextStack.cs
- SemaphoreFullException.cs
- PartialTrustVisibleAssemblyCollection.cs
- ZoneMembershipCondition.cs
- XmlComplianceUtil.cs
- IndexedGlyphRun.cs
- StylusDownEventArgs.cs
- StackSpiller.Generated.cs
- ConfigurationSectionCollection.cs
- ParagraphResult.cs
- DomainConstraint.cs
- Grammar.cs
- InputLanguageCollection.cs
- ToolboxDataAttribute.cs
- LongSumAggregationOperator.cs
- MulticastOption.cs
- CompositionAdorner.cs
- ExpressionPrefixAttribute.cs
- QEncodedStream.cs
- BindingOperations.cs
- ProcessHostConfigUtils.cs
- SQLDoubleStorage.cs
- IssuedTokenParametersElement.cs
- ProxyGenerationError.cs
- Typeface.cs
- SqlNode.cs
- Thickness.cs
- ArgumentNullException.cs
- XpsImage.cs
- ContentDisposition.cs
- RegisteredScript.cs
- PointIndependentAnimationStorage.cs
- BidirectionalDictionary.cs
- SmiMetaData.cs
- LessThan.cs
- TextEndOfLine.cs
- PersonalizationAdministration.cs
- FixedSOMLineCollection.cs
- ExtentKey.cs
- CharConverter.cs
- OpacityConverter.cs
- Group.cs
- SmiEventStream.cs