Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / CredentialManagerDialog.cs / 1 / CredentialManagerDialog.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: // CredentialManagerDialog is the Forms dialog that allows users to select RM Creds. // // History: // 08/15/05 - [....] created // //--------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Windows.Forms; using System.Windows.TrustUI; using System.Security; using System.Security.RightsManagement; namespace MS.Internal.Documents { ////// CredentialManagerDialog is used for choose RM credentials. /// internal sealed partial class CredentialManagerDialog : DialogBaseForm { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- ////// The constructor /// internal CredentialManagerDialog( IListaccountList, string defaultAccount, DocumentRightsManagementManager docRightsManagementManager ) { if ((docRightsManagementManager != null)) { _docRightsManagementManager = docRightsManagementManager; } else { throw new ArgumentNullException("DocumentRightsManagementManager"); } //Set the data source for the listbox SetCredentialManagementList(accountList, defaultAccount); //Enable or disable remove button depending on whether there is anything to remove _credListBox_SelectedIndexChanged(this, EventArgs.Empty); } #endregion Constructors #region Public Methods //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- /// /// SetCredentialManagementList. /// internal void SetCredentialManagementList( IListaccountList, string defaultAccount ) { //now we need to refresh everything. //Set the data source _credListBox.DataSource = accountList; if (defaultAccount != null) { //Now we need to get and select the default. _credListBox.SelectedIndex = _credListBox.Items.IndexOf(defaultAccount); } } #endregion Public Methods #region Private Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ /// /// Called when the selected item changes on the listbox. /// private void _credListBox_SelectedIndexChanged(object sender, EventArgs e) { //Check to see if something is selected. if (_credListBox.SelectedIndex >= 0) { _removeButton.Enabled = true; } else { _removeButton.Enabled = false; } } ////// OK button handler. /// ////// Critical - Calls a critical method _docRightsManagementManager::OnCredentialManagementSetDefault. /// TreatAsSafe - The data we are passing into the critical call is strictly user supplied text /// directly from the UI for list box. /// [SecurityCritical, SecurityTreatAsSafe] private void _okButton_Click(object sender, EventArgs e) { //Check to see if something is selected. if (_credListBox.SelectedIndex >= 0) { //Call Manager to set the default. _docRightsManagementManager.OnCredentialManagementSetDefault( (string) _credListBox.Items[_credListBox.SelectedIndex]); } } ////// Remove button handler. /// ////// Critical - Calls a critical method _docRightsManagementManager::OnCredentialManagementRemove. /// TreatAsSafe - The data we are passing into the critical call is strictly user supplied text /// directly from the UI for list box. /// [SecurityCritical, SecurityTreatAsSafe] private void _removeButton_Click(object sender, EventArgs e) { //Check to see if something is selected. if (_credListBox.SelectedIndex >= 0) { //Call Manager to remove selected user. _docRightsManagementManager.OnCredentialManagementRemove( (string)_credListBox.Items[_credListBox.SelectedIndex]); //Enable or disable remove button depending on whether there is anything to remove _credListBox_SelectedIndexChanged(this, EventArgs.Empty); } } ////// Add button handler. /// ////// Critical - Calls a critical method _docRightsManagementManager::OnCredentialManagementShowEnrollment. /// TreatAsSafe - No data is being passed into the critical method and only invoked when the UI button is clicked. /// [SecurityCritical, SecurityTreatAsSafe] private void _addButton_Click(object sender, EventArgs e) { //to add new user just call enrollment _docRightsManagementManager.OnCredentialManagementShowEnrollment(); //Enable or disable remove button depending on whether there is anything to remove _credListBox_SelectedIndexChanged(this, EventArgs.Empty); } #endregion Private Methods #region Protected Methods //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ ////// ApplyResources override. Called to apply dialog resources. /// protected override void ApplyResources() { base.ApplyResources(); _cancelButton.Text = SR.Get(SRID.RMCredManagementCancel); _okButton.Text = SR.Get(SRID.RMCredManagementOk); _addButton.Text = SR.Get(SRID.RMCredManagementAdd); _removeButton.Text = SR.Get(SRID.RMCredManagementRemove); _instructionLabel.Text = SR.Get(SRID.RMCredManagementInstruction); Text = SR.Get(SRID.RMCredManagementDialog); // Setup matching Add/Remove button widths int maxWidth = Math.Max(_addButton.Width, _removeButton.Width); _addButton.Width = maxWidth; _removeButton.Width = maxWidth; } #endregion Protected Methods #region Private Fields //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- private DocumentRightsManagementManager _docRightsManagementManager; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
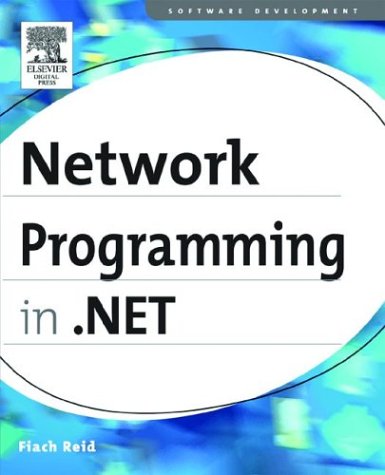
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewGroup.cs
- WebPartCloseVerb.cs
- FragmentNavigationEventArgs.cs
- SEHException.cs
- BlurEffect.cs
- StyleCollectionEditor.cs
- XmlSerializer.cs
- AnnotationElement.cs
- ContextMenu.cs
- ElementHostAutomationPeer.cs
- HtmlPanelAdapter.cs
- SubclassTypeValidator.cs
- DESCryptoServiceProvider.cs
- MessageFormatterConverter.cs
- StickyNoteAnnotations.cs
- PlatformNotSupportedException.cs
- IpcChannelHelper.cs
- AdornedElementPlaceholder.cs
- ModulesEntry.cs
- MarginsConverter.cs
- CultureInfoConverter.cs
- CorePropertiesFilter.cs
- OracleException.cs
- EntityDesignPluralizationHandler.cs
- XDRSchema.cs
- DiscoveryExceptionDictionary.cs
- XmlSchemaAnnotation.cs
- ScriptResourceHandler.cs
- HtmlTernaryTree.cs
- SelectorItemAutomationPeer.cs
- SoapServerMessage.cs
- PasswordBox.cs
- WebEventTraceProvider.cs
- IMembershipProvider.cs
- OrderPreservingPipeliningMergeHelper.cs
- DataServiceQueryOfT.cs
- __ConsoleStream.cs
- EntryWrittenEventArgs.cs
- AdornerHitTestResult.cs
- IdentitySection.cs
- TabControl.cs
- SimpleRecyclingCache.cs
- TreeChangeInfo.cs
- ReflectEventDescriptor.cs
- MetafileHeader.cs
- PropertyValueUIItem.cs
- ProgressBar.cs
- LinqDataSourceSelectEventArgs.cs
- PreviewPrintController.cs
- StylusPointDescription.cs
- WindowsImpersonationContext.cs
- TemplateBindingExtension.cs
- CDSCollectionETWBCLProvider.cs
- MeasureItemEvent.cs
- FileDialogCustomPlace.cs
- SecurityContext.cs
- CollectionsUtil.cs
- ZipIOFileItemStream.cs
- StateMachineSubscription.cs
- ImageListImageEditor.cs
- QilSortKey.cs
- SemanticResolver.cs
- SQLMoneyStorage.cs
- PipeStream.cs
- MemberJoinTreeNode.cs
- TypeUtils.cs
- ArraySortHelper.cs
- WebPartEventArgs.cs
- EqualityArray.cs
- Misc.cs
- SqlDataReaderSmi.cs
- XmlEntity.cs
- SimpleApplicationHost.cs
- SQLInt16.cs
- ApplicationTrust.cs
- FilterRepeater.cs
- MethodExpr.cs
- EntityContainerEmitter.cs
- WS2007FederationHttpBinding.cs
- SchemaElementDecl.cs
- SetterBaseCollection.cs
- _SslSessionsCache.cs
- Pkcs9Attribute.cs
- TypedDataSourceCodeGenerator.cs
- CryptoApi.cs
- CheckBoxList.cs
- ColumnTypeConverter.cs
- FrugalMap.cs
- entityreference_tresulttype.cs
- NumberAction.cs
- Attributes.cs
- iisPickupDirectory.cs
- ContractBase.cs
- WrappedIUnknown.cs
- PersianCalendar.cs
- DiagnosticTraceSource.cs
- HWStack.cs
- ListBindingHelper.cs
- CodeSnippetTypeMember.cs
- HtmlInputFile.cs