Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / ComponentModel / Int64Converter.cs / 1 / Int64Converter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] public class Int64Converter : BaseNumberConverter { ///Provides a type converter to convert 64-bit signed integer objects to and /// from various other representations. ////// The Type this converter is targeting (e.g. Int16, UInt32, etc.) /// internal override Type TargetType { get { return typeof(Int64); } } ////// Convert the given value to a string using the given radix /// internal override object FromString(string value, int radix) { return Convert.ToInt64(value, radix); } ////// Convert the given value to a string using the given formatInfo /// internal override object FromString(string value, NumberFormatInfo formatInfo) { return Int64.Parse(value, NumberStyles.Integer, formatInfo); } ////// Convert the given value to a string using the given CultureInfo /// internal override object FromString(string value, CultureInfo culture){ return Int64.Parse(value, culture); } ////// Convert the given value from a string using the given formatInfo /// internal override string ToString(object value, NumberFormatInfo formatInfo) { return ((Int64)value).ToString("G", formatInfo); } } }
Link Menu
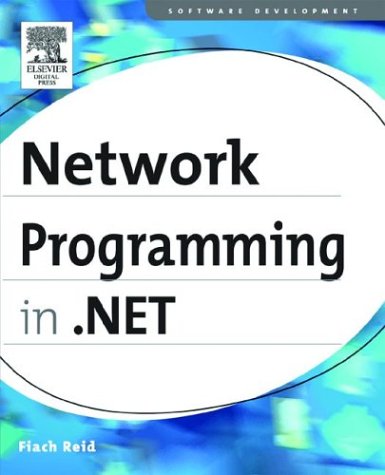
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbInsertCommandTree.cs
- RouteItem.cs
- RegisteredDisposeScript.cs
- XPathNodeList.cs
- DecimalKeyFrameCollection.cs
- MissingFieldException.cs
- DataListItem.cs
- CalendarDateRangeChangingEventArgs.cs
- XmlBaseWriter.cs
- AuthenticationServiceManager.cs
- EntitySetDataBindingList.cs
- CssTextWriter.cs
- NextPreviousPagerField.cs
- XmlExtensionFunction.cs
- StringConverter.cs
- XmlElementAttribute.cs
- TextTreeTextBlock.cs
- KnownColorTable.cs
- UInt16.cs
- StaticFileHandler.cs
- ActivityCodeGenerator.cs
- FontSource.cs
- DataGridViewCellValueEventArgs.cs
- ContentFileHelper.cs
- LifetimeServices.cs
- EntityDataSourceContainerNameConverter.cs
- tooltip.cs
- XamlDesignerSerializationManager.cs
- ConnectionPoolManager.cs
- path.cs
- FolderLevelBuildProviderAppliesToAttribute.cs
- SystemWebCachingSectionGroup.cs
- BrushConverter.cs
- ConstraintManager.cs
- PTProvider.cs
- XPathAxisIterator.cs
- LineSegment.cs
- ErrorFormatterPage.cs
- AnnotationDocumentPaginator.cs
- PropertyGridCommands.cs
- TextSegment.cs
- Italic.cs
- StringFormat.cs
- EntityContainerEntitySet.cs
- GifBitmapEncoder.cs
- LookupBindingPropertiesAttribute.cs
- ResXResourceSet.cs
- FontStyleConverter.cs
- DbModificationCommandTree.cs
- HandlerBase.cs
- RuntimeHelpers.cs
- SectionUpdates.cs
- XPathAncestorQuery.cs
- CreateUserWizard.cs
- FormatterConverter.cs
- WebControlAdapter.cs
- Model3DCollection.cs
- RenderDataDrawingContext.cs
- NamespaceTable.cs
- Stack.cs
- AlphabeticalEnumConverter.cs
- Wildcard.cs
- DelimitedListTraceListener.cs
- XmlNamespaceMappingCollection.cs
- CompositeDataBoundControl.cs
- ObjectIDGenerator.cs
- ProjectedWrapper.cs
- DateTimeOffsetAdapter.cs
- EndOfStreamException.cs
- SqlBulkCopy.cs
- ComponentDesigner.cs
- NetDataContractSerializer.cs
- EDesignUtil.cs
- PropertyGridView.cs
- DefaultSerializationProviderAttribute.cs
- ResourceDictionaryCollection.cs
- configsystem.cs
- HuffmanTree.cs
- SoapSchemaImporter.cs
- SimpleApplicationHost.cs
- AccessedThroughPropertyAttribute.cs
- RectValueSerializer.cs
- ExpressionBindingCollection.cs
- BookmarkResumptionRecord.cs
- SystemResourceHost.cs
- AddIn.cs
- InvalidEnumArgumentException.cs
- ComAwareEventInfo.cs
- RelationalExpressions.cs
- FileUtil.cs
- DataViewListener.cs
- CompilerGeneratedAttribute.cs
- CodeDomSerializerException.cs
- WebContext.cs
- Application.cs
- FontEmbeddingManager.cs
- LinkArea.cs
- PersonalizationState.cs
- HtmlUtf8RawTextWriter.cs
- EventLogPermissionEntryCollection.cs