Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CompMod / System / Diagnostics / TypedElement.cs / 1 / TypedElement.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Configuration; using System; using System.Reflection; using System.Globalization; namespace System.Diagnostics { internal class TypedElement : ConfigurationElement { protected static readonly ConfigurationProperty _propTypeName = new ConfigurationProperty("type", typeof(string), String.Empty, ConfigurationPropertyOptions.IsRequired); protected static readonly ConfigurationProperty _propInitData = new ConfigurationProperty("initializeData", typeof(string), String.Empty, ConfigurationPropertyOptions.None); protected ConfigurationPropertyCollection _properties; protected object _runtimeObject = null; private Type _baseType; public TypedElement(Type baseType) : base() { _properties = new ConfigurationPropertyCollection(); _properties.Add(_propTypeName); _properties.Add(_propInitData); _baseType = baseType; } [ConfigurationProperty("initializeData", DefaultValue = "")] public string InitData { get { return (string) this[_propInitData]; } // This is useful when the OM becomes public. In the meantime, this can be utilized via reflection set { this[_propInitData] = value; } } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("type", IsRequired = true, DefaultValue = "")] public virtual string TypeName { get { return (string) this[_propTypeName]; } set { this[_propTypeName] = value; } } protected object BaseGetRuntimeObject() { if (_runtimeObject == null) _runtimeObject = TraceUtils.GetRuntimeObject(TypeName, _baseType, InitData); return _runtimeObject; } } }
Link Menu
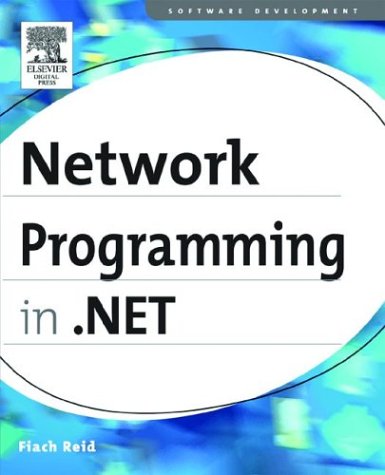
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AlternationConverter.cs
- ApplicationServiceManager.cs
- PkcsUtils.cs
- SafeNativeMethods.cs
- AppLevelCompilationSectionCache.cs
- ResourceKey.cs
- Token.cs
- Win32.cs
- StrongNameMembershipCondition.cs
- SerializationInfoEnumerator.cs
- COM2PropertyDescriptor.cs
- MethodToken.cs
- TypeConverterHelper.cs
- PropertyStore.cs
- DateTimeOffsetStorage.cs
- __ConsoleStream.cs
- AuthStoreRoleProvider.cs
- DataGridBoolColumn.cs
- SqlDataSourceCommandEventArgs.cs
- HotCommands.cs
- PropertyBuilder.cs
- Point3DCollection.cs
- XmlSchemaFacet.cs
- GeneralTransform3D.cs
- Canvas.cs
- RowParagraph.cs
- ToolboxItemWrapper.cs
- HtmlMeta.cs
- Select.cs
- Parameter.cs
- AlphabeticalEnumConverter.cs
- TableStyle.cs
- TextRunCache.cs
- BufferModeSettings.cs
- InputLangChangeRequestEvent.cs
- SqlInternalConnectionTds.cs
- Win32KeyboardDevice.cs
- WeakRefEnumerator.cs
- CodeParameterDeclarationExpressionCollection.cs
- MsmqAppDomainProtocolHandler.cs
- OleDbDataReader.cs
- safelink.cs
- SafeBitVector32.cs
- SelectionItemPattern.cs
- ReverseInheritProperty.cs
- precedingsibling.cs
- ConfigurationManagerInternalFactory.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- DataListCommandEventArgs.cs
- CompatibleComparer.cs
- OdbcHandle.cs
- ImageSource.cs
- JsonReaderDelegator.cs
- WebServiceEnumData.cs
- TextPenaltyModule.cs
- RowVisual.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- WebPartZoneAutoFormat.cs
- Classification.cs
- SByteStorage.cs
- AutomationIdentifier.cs
- StringFreezingAttribute.cs
- SchemaImporterExtension.cs
- XmlSchemaIdentityConstraint.cs
- MarkupCompilePass1.cs
- RegexRunner.cs
- InfiniteIntConverter.cs
- UInt16.cs
- EditorBrowsableAttribute.cs
- Substitution.cs
- ActionItem.cs
- PropertyExpression.cs
- Timer.cs
- AuthenticationModulesSection.cs
- CardSpaceSelector.cs
- Camera.cs
- DictionaryContent.cs
- DataGridViewComboBoxCell.cs
- StackOverflowException.cs
- XmlAutoDetectWriter.cs
- ReturnEventArgs.cs
- HttpWrapper.cs
- StringExpressionSet.cs
- PointKeyFrameCollection.cs
- QueueProcessor.cs
- EventWaitHandleSecurity.cs
- _SafeNetHandles.cs
- ListItemCollection.cs
- HtmlTable.cs
- BitmapImage.cs
- ProcessStartInfo.cs
- RestHandlerFactory.cs
- NoneExcludedImageIndexConverter.cs
- FormViewDeletedEventArgs.cs
- HybridDictionary.cs
- RMPublishingDialog.cs
- MethodToken.cs
- Vector3DKeyFrameCollection.cs
- FlowDocumentReaderAutomationPeer.cs
- CodeLabeledStatement.cs