Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / WinForms / Managed / System / WinForms / DataGridViewAdvancedBorderStyle.cs / 1 / DataGridViewAdvancedBorderStyle.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Diagnostics; using System.ComponentModel; ///public sealed class DataGridViewAdvancedBorderStyle : ICloneable { private DataGridView owner; private bool all = true; private DataGridViewAdvancedCellBorderStyle banned1, banned2, banned3; private DataGridViewAdvancedCellBorderStyle top = DataGridViewAdvancedCellBorderStyle.None; private DataGridViewAdvancedCellBorderStyle left = DataGridViewAdvancedCellBorderStyle.None; private DataGridViewAdvancedCellBorderStyle right = DataGridViewAdvancedCellBorderStyle.None; private DataGridViewAdvancedCellBorderStyle bottom = DataGridViewAdvancedCellBorderStyle.None; /// public DataGridViewAdvancedBorderStyle() : this(null, DataGridViewAdvancedCellBorderStyle.NotSet, DataGridViewAdvancedCellBorderStyle.NotSet, DataGridViewAdvancedCellBorderStyle.NotSet) { } internal DataGridViewAdvancedBorderStyle(DataGridView owner) : this(owner, DataGridViewAdvancedCellBorderStyle.NotSet, DataGridViewAdvancedCellBorderStyle.NotSet, DataGridViewAdvancedCellBorderStyle.NotSet) { } /// /// Creates a new DataGridViewAdvancedBorderStyle. The specified owner will /// be notified when the values are changed. /// internal DataGridViewAdvancedBorderStyle(DataGridView owner, DataGridViewAdvancedCellBorderStyle banned1, DataGridViewAdvancedCellBorderStyle banned2, DataGridViewAdvancedCellBorderStyle banned3) { this.owner = owner; this.banned1 = banned1; this.banned2 = banned2; this.banned3 = banned3; } ///public DataGridViewAdvancedCellBorderStyle All { get { return this.all ? this.top : DataGridViewAdvancedCellBorderStyle.NotSet; } set { // Sequential enum. Valid values are 0x0 to 0x7 if (!ClientUtils.IsEnumValid(value, (int)value, (int)DataGridViewAdvancedCellBorderStyle.NotSet, (int)DataGridViewAdvancedCellBorderStyle.OutsetPartial)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DataGridViewAdvancedCellBorderStyle)); } if (value == DataGridViewAdvancedCellBorderStyle.NotSet || value == this.banned1 || value == this.banned2 || value == this.banned3) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "All")); } if (!this.all || this.top != value) { this.all = true; this.top = this.left = this.right = this.bottom = value; if (this.owner != null) { this.owner.OnAdvancedBorderStyleChanged(this); } } } } /// public DataGridViewAdvancedCellBorderStyle Bottom { get { if (this.all) { return this.top; } return this.bottom; } set { // Sequential enum. Valid values are 0x0 to 0x7 if (!ClientUtils.IsEnumValid(value, (int)value, (int)DataGridViewAdvancedCellBorderStyle.NotSet, (int)DataGridViewAdvancedCellBorderStyle.OutsetPartial)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DataGridViewAdvancedCellBorderStyle)); } if (value == DataGridViewAdvancedCellBorderStyle.NotSet) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Bottom")); } this.BottomInternal = value; } } internal DataGridViewAdvancedCellBorderStyle BottomInternal { set { //Debug.Assert(Enum.IsDefined(typeof(DataGridViewAdvancedCellBorderStyle), value)); //Debug.Assert(value != DataGridViewAdvancedCellBorderStyle.NotSet); if ((this.all && this.top != value) || (!this.all && this.bottom != value)) { if (this.all) { if (this.right == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.right = DataGridViewAdvancedCellBorderStyle.Outset; } } this.all = false; this.bottom = value; if (this.owner != null) { this.owner.OnAdvancedBorderStyleChanged(this); } } } } /// public DataGridViewAdvancedCellBorderStyle Left { get { if (this.all) { return this.top; } return this.left; } set { // Sequential enum. Valid values are 0x0 to 0x7 if (!ClientUtils.IsEnumValid(value, (int)value, (int)DataGridViewAdvancedCellBorderStyle.NotSet, (int)DataGridViewAdvancedCellBorderStyle.OutsetPartial)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DataGridViewAdvancedCellBorderStyle)); } if (value == DataGridViewAdvancedCellBorderStyle.NotSet) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Left")); } this.LeftInternal = value; } } internal DataGridViewAdvancedCellBorderStyle LeftInternal { set { //Debug.Assert(Enum.IsDefined(typeof(DataGridViewAdvancedCellBorderStyle), value)); //Debug.Assert(value != DataGridViewAdvancedCellBorderStyle.NotSet); if ((this.all && this.top != value) || (!this.all && this.left != value)) { if ((this.owner != null && this.owner.RightToLeftInternal) && (value == DataGridViewAdvancedCellBorderStyle.InsetDouble || value == DataGridViewAdvancedCellBorderStyle.OutsetDouble)) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Left")); } if (this.all) { if (this.right == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.right = DataGridViewAdvancedCellBorderStyle.Outset; } if (this.bottom == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.bottom = DataGridViewAdvancedCellBorderStyle.Outset; } } this.all = false; this.left = value; if (this.owner != null) { this.owner.OnAdvancedBorderStyleChanged(this); } } } } /// public DataGridViewAdvancedCellBorderStyle Right { get { if (this.all) { return this.top; } return this.right; } set { // Sequential enum. Valid values are 0x0 to 0x7 if (!ClientUtils.IsEnumValid(value, (int)value, (int)DataGridViewAdvancedCellBorderStyle.NotSet, (int)DataGridViewAdvancedCellBorderStyle.OutsetPartial)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DataGridViewAdvancedCellBorderStyle)); } if (value == DataGridViewAdvancedCellBorderStyle.NotSet) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Right")); } this.RightInternal = value; } } internal DataGridViewAdvancedCellBorderStyle RightInternal { set { //Debug.Assert(Enum.IsDefined(typeof(DataGridViewAdvancedCellBorderStyle), value)); //Debug.Assert(value != DataGridViewAdvancedCellBorderStyle.NotSet); if ((this.all && this.top != value) || (!this.all && this.right != value)) { if ((this.owner != null && !this.owner.RightToLeftInternal) && (value == DataGridViewAdvancedCellBorderStyle.InsetDouble || value == DataGridViewAdvancedCellBorderStyle.OutsetDouble)) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Right")); } if (this.all) { if (this.bottom == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.bottom = DataGridViewAdvancedCellBorderStyle.Outset; } } this.all = false; this.right = value; if (this.owner != null) { this.owner.OnAdvancedBorderStyleChanged(this); } } } } /// public DataGridViewAdvancedCellBorderStyle Top { get { return this.top; } set { // Sequential enum. Valid values are 0x0 to 0x7 if (!ClientUtils.IsEnumValid(value, (int)value, (int)DataGridViewAdvancedCellBorderStyle.NotSet, (int)DataGridViewAdvancedCellBorderStyle.OutsetPartial)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(DataGridViewAdvancedCellBorderStyle)); } if (value == DataGridViewAdvancedCellBorderStyle.NotSet) { throw new ArgumentException(SR.GetString(SR.DataGridView_AdvancedCellBorderStyleInvalid, "Top")); } this.TopInternal = value; } } internal DataGridViewAdvancedCellBorderStyle TopInternal { set { //Debug.Assert(Enum.IsDefined(typeof(DataGridViewAdvancedCellBorderStyle), value)); //Debug.Assert(value != DataGridViewAdvancedCellBorderStyle.NotSet); if ((this.all && this.top != value) || (!this.all && this.top != value)) { if (this.all) { if (this.right == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.right = DataGridViewAdvancedCellBorderStyle.Outset; } if (this.bottom == DataGridViewAdvancedCellBorderStyle.OutsetDouble) { this.bottom = DataGridViewAdvancedCellBorderStyle.Outset; } } this.all = false; this.top = value; if (this.owner != null) { this.owner.OnAdvancedBorderStyleChanged(this); } } } } /// /// public override bool Equals(object other) { DataGridViewAdvancedBorderStyle dgvabsOther = other as DataGridViewAdvancedBorderStyle; if (dgvabsOther == null) { return false; } return dgvabsOther.all == this.all && dgvabsOther.top == this.top && dgvabsOther.left == this.left && dgvabsOther.bottom == this.bottom && dgvabsOther.right == this.right; } /// /// public override int GetHashCode() { return WindowsFormsUtils.GetCombinedHashCodes((int) this.top, (int) this.left, (int) this.bottom, (int) this.right); } /// /// public override string ToString() { return "DataGridViewAdvancedBorderStyle { All=" + this.All.ToString() + ", Left=" + this.Left.ToString() + ", Right=" + this.Right.ToString() + ", Top=" + this.Top.ToString() + ", Bottom=" + this.Bottom.ToString() + " }"; } /// /// object ICloneable.Clone() { DataGridViewAdvancedBorderStyle dgvabs = new DataGridViewAdvancedBorderStyle(this.owner, this.banned1, this.banned2, this.banned3); dgvabs.all = this.all; dgvabs.top = this.top; dgvabs.right = this.right; dgvabs.bottom = this.bottom; dgvabs.left = this.left; return dgvabs; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
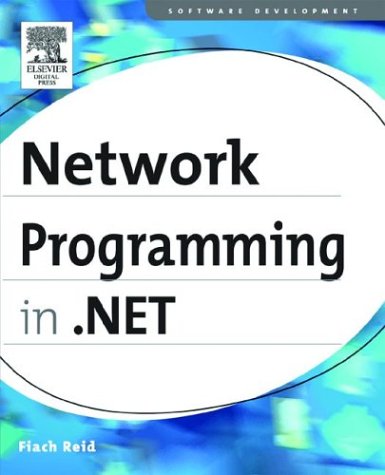
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ThemeDirectoryCompiler.cs
- HierarchicalDataSourceIDConverter.cs
- TraceSource.cs
- BatchParser.cs
- WriteTimeStream.cs
- PropertyToken.cs
- FormatterConverter.cs
- OperationCanceledException.cs
- HtmlElementEventArgs.cs
- FrameworkEventSource.cs
- ArithmeticException.cs
- SymbolMethod.cs
- UnsafeNativeMethods.cs
- ObjectMaterializedEventArgs.cs
- Formatter.cs
- TextServicesPropertyRanges.cs
- TextDocumentView.cs
- BindingExpressionUncommonField.cs
- OdbcConnectionPoolProviderInfo.cs
- CollaborationHelperFunctions.cs
- GroupStyle.cs
- TreeViewImageGenerator.cs
- ListBoxChrome.cs
- ObjectDataSourceStatusEventArgs.cs
- DataControlFieldHeaderCell.cs
- MergePropertyDescriptor.cs
- DefaultDialogButtons.cs
- TagMapCollection.cs
- JsonSerializer.cs
- LayoutEngine.cs
- Peer.cs
- ConfigurationPropertyCollection.cs
- ReadOnlyHierarchicalDataSource.cs
- SqlParameter.cs
- WebRequestModuleElement.cs
- NamedObject.cs
- CatalogZoneDesigner.cs
- InlineUIContainer.cs
- EventArgs.cs
- DataGridPagerStyle.cs
- FileSystemEventArgs.cs
- ArrayList.cs
- ResourceAssociationSetEnd.cs
- PeerHopCountAttribute.cs
- DbRetry.cs
- AssociatedControlConverter.cs
- BamlRecordHelper.cs
- Debug.cs
- propertyentry.cs
- RectangleHotSpot.cs
- EqualityComparer.cs
- XamlPoint3DCollectionSerializer.cs
- XmlUtf8RawTextWriter.cs
- HWStack.cs
- SQLDateTime.cs
- HMACMD5.cs
- TreeViewAutomationPeer.cs
- MessageContractAttribute.cs
- MatrixKeyFrameCollection.cs
- AutomationIdentifier.cs
- DataStorage.cs
- BindingFormattingDialog.cs
- AuthStoreRoleProvider.cs
- ReadWriteObjectLock.cs
- EntryWrittenEventArgs.cs
- XPathAncestorQuery.cs
- SqlDataSourceQueryEditor.cs
- DataViewManager.cs
- StringToken.cs
- WindowsListViewItemCheckBox.cs
- OleDbPermission.cs
- ModuleBuilder.cs
- MsdtcClusterUtils.cs
- Metafile.cs
- CompoundFileIOPermission.cs
- PingReply.cs
- SoapEnumAttribute.cs
- Point3DAnimationBase.cs
- Attributes.cs
- WebHttpBindingCollectionElement.cs
- OverflowException.cs
- StrokeRenderer.cs
- ServicePointManager.cs
- BitmapCodecInfoInternal.cs
- AudioFormatConverter.cs
- CacheChildrenQuery.cs
- CompositeScriptReferenceEventArgs.cs
- HttpResponseInternalWrapper.cs
- Char.cs
- Typography.cs
- ResourcePool.cs
- LeaseManager.cs
- PreservationFileWriter.cs
- WorkflowWebService.cs
- EmptyTextWriter.cs
- X509CertificateTokenFactoryCredential.cs
- RuleSetDialog.cs
- MetadataSerializer.cs
- CharacterString.cs
- CodeLabeledStatement.cs