Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / DbUpdateCommandTree.cs / 2 / DbUpdateCommandTree.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Represents a single-row update operation expressed as a canonical command tree. /// When the [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbUpdateCommandTree : DbModificationCommandTree { private DbExpression _predicate; private DbExpression _returning; IListproperty is set, the command returns a reader; otherwise, /// it returns a scalar indicating the number of rows affected. /// _setClauses; internal DbUpdateCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) : base(metadata, dataSpace) { } /// /// Gets the list of update set clauses that define the update operation. /// public IListSetClauses { get { return _setClauses; } } /// /// Gets an ///that specifies a projection of results to be returned based on the modified rows. /// If null, indicates no results should be returned from this command. /// /// The returning projection includes only the following elements: /// public DbExpression Returning { get { return _returning; } internal set { _returning = value; } } //////
///- NewInstance expression
///- Property expression
////// Gets an ///that specifies the predicate used to determine which members of the target collection should be updated. /// /// The predicate includes only the following elements: /// public DbExpression Predicate { get { return _predicate; } internal set { _predicate = value; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Update; } } internal override bool HasReader { get { return null != Returning; } } internal override void DumpStructure(ExpressionDumper dumper) { base.DumpStructure(dumper); if (this.Predicate != null) { dumper.Dump(this.Predicate, "Predicate"); } dumper.Begin("SetClauses", null); foreach (DbModificationClause clause in this.SetClauses) { if (null != clause) { clause.DumpStructure(dumper); } } dumper.End("SetClauses"); dumper.Dump(this.Returning, "Returning"); } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer replacer) { using (new EntityBid.ScopeAuto("///
///- Equality expression
///- Constant expression
///- IsNull expression
///- Property expression
///- Reference expression to the target
///- And expression
///- Or expression
///- Not expression
///%d#", this.ObjectId)) { throw EntityUtil.NotSupported(); } } /// /// Initializes set clauses on this command tree. /// /// Set clauses. internal void InitializeSetClauses(ListsetClauses) { Debug.Assert(null != setClauses, "setClauses cannot be null"); _setClauses = setClauses.AsReadOnly(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Data.Common.Utils; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Represents a single-row update operation expressed as a canonical command tree. /// When the [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbUpdateCommandTree : DbModificationCommandTree { private DbExpression _predicate; private DbExpression _returning; IListproperty is set, the command returns a reader; otherwise, /// it returns a scalar indicating the number of rows affected. /// _setClauses; internal DbUpdateCommandTree(MetadataWorkspace metadata, DataSpace dataSpace) : base(metadata, dataSpace) { } /// /// Gets the list of update set clauses that define the update operation. /// public IListSetClauses { get { return _setClauses; } } /// /// Gets an ///that specifies a projection of results to be returned based on the modified rows. /// If null, indicates no results should be returned from this command. /// /// The returning projection includes only the following elements: /// public DbExpression Returning { get { return _returning; } internal set { _returning = value; } } //////
///- NewInstance expression
///- Property expression
////// Gets an ///that specifies the predicate used to determine which members of the target collection should be updated. /// /// The predicate includes only the following elements: /// public DbExpression Predicate { get { return _predicate; } internal set { _predicate = value; } } internal override DbCommandTreeKind CommandTreeKind { get { return DbCommandTreeKind.Update; } } internal override bool HasReader { get { return null != Returning; } } internal override void DumpStructure(ExpressionDumper dumper) { base.DumpStructure(dumper); if (this.Predicate != null) { dumper.Dump(this.Predicate, "Predicate"); } dumper.Begin("SetClauses", null); foreach (DbModificationClause clause in this.SetClauses) { if (null != clause) { clause.DumpStructure(dumper); } } dumper.End("SetClauses"); dumper.Dump(this.Returning, "Returning"); } internal override string PrintTree(ExpressionPrinter printer) { return printer.Print(this); } internal override void Replace(ExpressionReplacer replacer) { using (new EntityBid.ScopeAuto("///
///- Equality expression
///- Constant expression
///- IsNull expression
///- Property expression
///- Reference expression to the target
///- And expression
///- Or expression
///- Not expression
///%d#", this.ObjectId)) { throw EntityUtil.NotSupported(); } } /// /// Initializes set clauses on this command tree. /// /// Set clauses. internal void InitializeSetClauses(ListsetClauses) { Debug.Assert(null != setClauses, "setClauses cannot be null"); _setClauses = setClauses.AsReadOnly(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
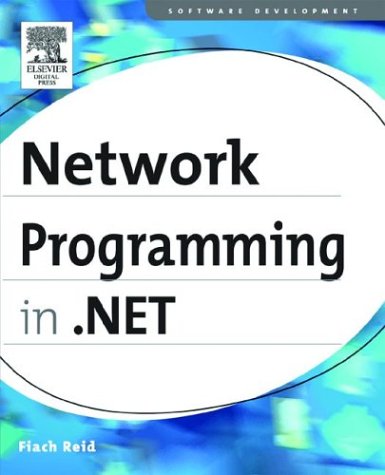
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DebugView.cs
- BulletedListEventArgs.cs
- XamlPathDataSerializer.cs
- StatusBar.cs
- SerializableAttribute.cs
- Delegate.cs
- BezierSegment.cs
- GenericUriParser.cs
- ColumnMapProcessor.cs
- DisplayMemberTemplateSelector.cs
- DispatcherExceptionEventArgs.cs
- DataGridViewAdvancedBorderStyle.cs
- _UriSyntax.cs
- ObjectIDGenerator.cs
- TextPointer.cs
- DeobfuscatingStream.cs
- ImageAnimator.cs
- CFStream.cs
- Rule.cs
- UserNameSecurityTokenParameters.cs
- SetterBase.cs
- DisplayMemberTemplateSelector.cs
- OutputCache.cs
- VisemeEventArgs.cs
- ClassHandlersStore.cs
- CodePropertyReferenceExpression.cs
- ColumnResizeAdorner.cs
- DBParameter.cs
- ProcessThreadCollection.cs
- CatalogZone.cs
- DesignBindingPropertyDescriptor.cs
- ResourceSetExpression.cs
- XmlValueConverter.cs
- DecimalAverageAggregationOperator.cs
- SystemIcmpV4Statistics.cs
- ConfigurationValues.cs
- Rectangle.cs
- CodeIdentifiers.cs
- InvokeProviderWrapper.cs
- Base64Stream.cs
- SessionChannels.cs
- DescendentsWalker.cs
- WSSecurityOneDotOneReceiveSecurityHeader.cs
- DataTableReaderListener.cs
- ConfigurationManagerHelper.cs
- EntityDesignerBuildProvider.cs
- RowUpdatedEventArgs.cs
- SoapHeaderAttribute.cs
- FastEncoderWindow.cs
- SerializationStore.cs
- XmlNodeWriter.cs
- XPathPatternBuilder.cs
- SafeNativeMethods.cs
- DbModificationClause.cs
- Mapping.cs
- MediaTimeline.cs
- ListControl.cs
- BoolExpr.cs
- CqlWriter.cs
- MenuEventArgs.cs
- Registry.cs
- CodeParameterDeclarationExpression.cs
- TextRunTypographyProperties.cs
- Double.cs
- TcpStreams.cs
- ProviderCollection.cs
- ComplexPropertyEntry.cs
- Emitter.cs
- Brush.cs
- HttpListenerPrefixCollection.cs
- InkPresenterAutomationPeer.cs
- ApplyTemplatesAction.cs
- BaseCollection.cs
- HealthMonitoringSection.cs
- WebContext.cs
- ApplicationServicesHostFactory.cs
- BaseDataBoundControl.cs
- Hyperlink.cs
- InternalConfigRoot.cs
- DataGridCellInfo.cs
- DSACryptoServiceProvider.cs
- MemberRelationshipService.cs
- CallTemplateAction.cs
- FullTextBreakpoint.cs
- ProtocolsConfigurationHandler.cs
- LinkDescriptor.cs
- RequestCachePolicy.cs
- MethodRental.cs
- RemotingConfigParser.cs
- EdmConstants.cs
- IsolationInterop.cs
- CompositeDataBoundControl.cs
- CommittableTransaction.cs
- InputReport.cs
- COM2Properties.cs
- UrlMappingsSection.cs
- BuildDependencySet.cs
- ErrorCodes.cs
- DragDrop.cs
- AttributeData.cs