Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / BuildTasks / MS / Internal / MarkupCompiler / CompilationUnit.cs / 1 / CompilationUnit.cs
using System; using System.Security.Permissions; namespace MS.Internal { ////// The CompilationUnit class /// internal class CompilationUnit { #region Constructors ///constructor public CompilationUnit(string assemblyName, string language, string defaultNamespace, string [] fileList) { _assemblyName = assemblyName; _language = language; _fileList = fileList; _defaultNamespace = defaultNamespace; } #endregion Constructors #region Properties internal bool Pass2 { get { return _pass2; } set { _pass2 = value; } } ///Name of the assembly the package is compiled into public string AssemblyName { get { return _assemblyName; } } ///Name of the CLR language the package is compiled into public string Language { get { return _language; } } ///path to the project root public string SourcePath { get { return _sourcePath; } set { _sourcePath = value; } } ///Default CLR Namespace of the project public string DefaultNamespace { get { return _defaultNamespace; } } ///Application definition file (relative to SourcePath) public string ApplicationFile { get { return _applicationFile; } set { _applicationFile = value; } } ///A list of relative (to SourcePath) file path names comprising the package to be compiled public string [] FileList { get { return _fileList; } } #endregion Properties #region Private Data private bool _pass2 = false; private string _assemblyName = string.Empty; private string _language = string.Empty; private string _sourcePath = string.Empty; private string _defaultNamespace = string.Empty; private string _applicationFile = string.Empty; private string [] _fileList; #endregion Private Data } #region ErrorEvent ////// Delegate for the Error event. /// internal delegate void MarkupErrorEventHandler(Object sender, MarkupErrorEventArgs e); ////// Event args for the Error event /// internal class MarkupErrorEventArgs : EventArgs { #region Constructors ////// constructor /// internal MarkupErrorEventArgs(Exception e, int lineNum, int linePos, string fileName) { _e = e; _lineNum = lineNum; _linePos = linePos; _fileName = fileName; } #endregion Constructors #region Properties ////// The Error Message /// public Exception Exception { get { return _e; } } ////// The line number at which the compile Error occured /// public int LineNumber { get { return _lineNum; } } ////// The character position in the line at which the compile Error occured /// public int LinePosition { get { return _linePos; } } ////// The xaml file in which the compile Error occured /// public string FileName { get { return _fileName; } } #endregion Properties #region Private Data private int _lineNum; private int _linePos; private Exception _e; private string _fileName; #endregion Private Data } #endregion ErrorEvent #region SourceFileResolveEvent ////// Delegate for the SourceFileResolve Event. /// internal delegate void SourceFileResolveEventHandler(Object sender, SourceFileResolveEventArgs e); ////// Event args for the Error event /// internal class SourceFileResolveEventArgs: EventArgs { #region Constructors ////// constructor /// internal SourceFileResolveEventArgs(string filePath) { _sourceFileInfo = new SourceFileInfo(filePath); } #endregion Constructors #region Properties // // FileInfo // internal SourceFileInfo SourceFileInfo { get { return _sourceFileInfo; } } #endregion Properties #region Private Data private SourceFileInfo _sourceFileInfo; #endregion Private Data } #endregion SourceFileResolveEvent #region ReferenceAssembly //// Reference Assembly // Passed by CodeGenertation Task. // Consumed by Parser. // internal class ReferenceAssembly : MarshalByRefObject { #region Constructor //// Constructor // internal ReferenceAssembly() { _path = null; _assemblyName = null; } //// Constructor // // // internal ReferenceAssembly(string path, string assemblyname) { Path = path; AssemblyName = assemblyname; } #endregion Constructor #region Internal Properties //// The path for the assembly. // The path must end with "\", but not include any Assembly Name. // //internal string Path { get { return _path; } set { _path = value; } } // // AssemblyName without any Extension part. // //internal string AssemblyName { get { return _assemblyName; } set { _assemblyName = value;} } #endregion Internal Properties #region private fields private string _path; private string _assemblyName; #endregion private fields } #endregion ReferenceAssembly } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security.Permissions; namespace MS.Internal { /// /// The CompilationUnit class /// internal class CompilationUnit { #region Constructors ///constructor public CompilationUnit(string assemblyName, string language, string defaultNamespace, string [] fileList) { _assemblyName = assemblyName; _language = language; _fileList = fileList; _defaultNamespace = defaultNamespace; } #endregion Constructors #region Properties internal bool Pass2 { get { return _pass2; } set { _pass2 = value; } } ///Name of the assembly the package is compiled into public string AssemblyName { get { return _assemblyName; } } ///Name of the CLR language the package is compiled into public string Language { get { return _language; } } ///path to the project root public string SourcePath { get { return _sourcePath; } set { _sourcePath = value; } } ///Default CLR Namespace of the project public string DefaultNamespace { get { return _defaultNamespace; } } ///Application definition file (relative to SourcePath) public string ApplicationFile { get { return _applicationFile; } set { _applicationFile = value; } } ///A list of relative (to SourcePath) file path names comprising the package to be compiled public string [] FileList { get { return _fileList; } } #endregion Properties #region Private Data private bool _pass2 = false; private string _assemblyName = string.Empty; private string _language = string.Empty; private string _sourcePath = string.Empty; private string _defaultNamespace = string.Empty; private string _applicationFile = string.Empty; private string [] _fileList; #endregion Private Data } #region ErrorEvent ////// Delegate for the Error event. /// internal delegate void MarkupErrorEventHandler(Object sender, MarkupErrorEventArgs e); ////// Event args for the Error event /// internal class MarkupErrorEventArgs : EventArgs { #region Constructors ////// constructor /// internal MarkupErrorEventArgs(Exception e, int lineNum, int linePos, string fileName) { _e = e; _lineNum = lineNum; _linePos = linePos; _fileName = fileName; } #endregion Constructors #region Properties ////// The Error Message /// public Exception Exception { get { return _e; } } ////// The line number at which the compile Error occured /// public int LineNumber { get { return _lineNum; } } ////// The character position in the line at which the compile Error occured /// public int LinePosition { get { return _linePos; } } ////// The xaml file in which the compile Error occured /// public string FileName { get { return _fileName; } } #endregion Properties #region Private Data private int _lineNum; private int _linePos; private Exception _e; private string _fileName; #endregion Private Data } #endregion ErrorEvent #region SourceFileResolveEvent ////// Delegate for the SourceFileResolve Event. /// internal delegate void SourceFileResolveEventHandler(Object sender, SourceFileResolveEventArgs e); ////// Event args for the Error event /// internal class SourceFileResolveEventArgs: EventArgs { #region Constructors ////// constructor /// internal SourceFileResolveEventArgs(string filePath) { _sourceFileInfo = new SourceFileInfo(filePath); } #endregion Constructors #region Properties // // FileInfo // internal SourceFileInfo SourceFileInfo { get { return _sourceFileInfo; } } #endregion Properties #region Private Data private SourceFileInfo _sourceFileInfo; #endregion Private Data } #endregion SourceFileResolveEvent #region ReferenceAssembly //// Reference Assembly // Passed by CodeGenertation Task. // Consumed by Parser. // internal class ReferenceAssembly : MarshalByRefObject { #region Constructor //// Constructor // internal ReferenceAssembly() { _path = null; _assemblyName = null; } //// Constructor // // // internal ReferenceAssembly(string path, string assemblyname) { Path = path; AssemblyName = assemblyname; } #endregion Constructor #region Internal Properties //// The path for the assembly. // The path must end with "\", but not include any Assembly Name. // //internal string Path { get { return _path; } set { _path = value; } } // // AssemblyName without any Extension part. // //internal string AssemblyName { get { return _assemblyName; } set { _assemblyName = value;} } #endregion Internal Properties #region private fields private string _path; private string _assemblyName; #endregion private fields } #endregion ReferenceAssembly } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
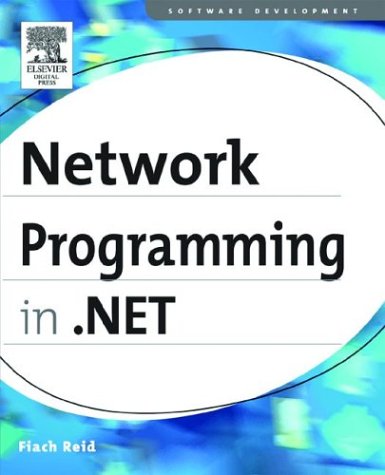
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PartitionResolver.cs
- EncoderBestFitFallback.cs
- ListManagerBindingsCollection.cs
- SchemaSetCompiler.cs
- BuildResultCache.cs
- PositiveTimeSpanValidator.cs
- EntityClassGenerator.cs
- _LazyAsyncResult.cs
- XmlSchemaProviderAttribute.cs
- WebPartMinimizeVerb.cs
- BinaryMethodMessage.cs
- DelayedRegex.cs
- SqlBulkCopy.cs
- OdbcConnectionOpen.cs
- ImageListStreamer.cs
- DataSourceNameHandler.cs
- ComponentDispatcher.cs
- DataGridHelper.cs
- Html32TextWriter.cs
- EpmHelper.cs
- FileSystemInfo.cs
- DesignOnlyAttribute.cs
- BackStopAuthenticationModule.cs
- CacheAxisQuery.cs
- SafeEventLogWriteHandle.cs
- ListComponentEditor.cs
- SerializationSectionGroup.cs
- RuleSettingsCollection.cs
- ToolStripDropDownButton.cs
- PointValueSerializer.cs
- AppDomainFactory.cs
- ServiceModelSecurityTokenTypes.cs
- ArithmeticException.cs
- InstanceCreationEditor.cs
- SqlCacheDependencySection.cs
- MeasureData.cs
- InternalPermissions.cs
- RightsManagementEncryptionTransform.cs
- Camera.cs
- DownloadProgressEventArgs.cs
- FontStretch.cs
- MailDefinition.cs
- OdbcReferenceCollection.cs
- RequestCachePolicy.cs
- ReadOnlyDictionary.cs
- ImageClickEventArgs.cs
- ErrorTableItemStyle.cs
- ComEventsHelper.cs
- CaseInsensitiveComparer.cs
- BounceEase.cs
- ExecutionEngineException.cs
- DurableEnlistmentState.cs
- FileDialog.cs
- PointKeyFrameCollection.cs
- ConfigurationValidatorBase.cs
- PromptEventArgs.cs
- IPHostEntry.cs
- HtmlControlPersistable.cs
- ResumeStoryboard.cs
- AssociatedControlConverter.cs
- SynchronizationLockException.cs
- Splitter.cs
- ResourceManagerWrapper.cs
- WebRequest.cs
- HttpHostedTransportConfiguration.cs
- TextRunTypographyProperties.cs
- sortedlist.cs
- ProgressBarBrushConverter.cs
- PreProcessor.cs
- UInt32Converter.cs
- EventLogger.cs
- ICspAsymmetricAlgorithm.cs
- COAUTHINFO.cs
- PassportAuthenticationModule.cs
- WindowsProgressbar.cs
- GridLength.cs
- ToolStripItemClickedEventArgs.cs
- SqlDataReaderSmi.cs
- BackgroundFormatInfo.cs
- OdbcPermission.cs
- GroupStyle.cs
- SettingsBindableAttribute.cs
- AuthenticatingEventArgs.cs
- OleDbWrapper.cs
- _SingleItemRequestCache.cs
- ProfileModule.cs
- StyleSelector.cs
- WinFormsSecurity.cs
- RowToFieldTransformer.cs
- CharacterBufferReference.cs
- Base64Stream.cs
- ThaiBuddhistCalendar.cs
- sqlpipe.cs
- PeerResolverMode.cs
- storepermissionattribute.cs
- _CommandStream.cs
- GroupItemAutomationPeer.cs
- BitmapEffectDrawingContextWalker.cs
- CodeDomSerializerBase.cs
- UniqueSet.cs