Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / MS / Internal / TextFormatting / TextPenaltyModule.cs / 1 / TextPenaltyModule.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextPenaltyModule.cs // // Contents: Critical handle wrapping unmanaged text penalty module for // penalty calculation of optimal paragraph vis PTS direct access. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 4-4-2006 Worachai Chaoweeraprasit (Wchao) // //----------------------------------------------------------------------- using System; using System.Security; using System.Windows.Media; using System.Windows.Media.TextFormatting; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// Critical handle wrapper of unmanaged text penalty module. This class /// is used exclusively by Framework thru friend-access. It provides direct /// access to the underlying dangerous handle to the unmanaged resource whose /// lifetime is bound to the the underlying LS context. /// [FriendAccessAllowed] // used by Framework internal sealed class TextPenaltyModule : IDisposable { private SecurityCriticalDataForSet_ploPenaltyModule; // Pointer to LS penalty module private bool _isDisposed; /// /// This constructor is called by PInvoke when returning the critical handle /// ////// Critical - as this calls the setter of _ploPenaltyModule. /// Safe - as it does not set the value arbitrarily from the value it receives from caller. /// [SecurityCritical, SecurityTreatAsSafe] internal TextPenaltyModule(SecurityCriticalDataForSetploc) { IntPtr ploPenaltyModule; LsErr lserr = UnsafeNativeMethods.LoAcquirePenaltyModule(ploc.Value, out ploPenaltyModule); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.AcquirePenaltyModuleFailure, lserr), lserr); } _ploPenaltyModule.Value = ploPenaltyModule; } /// /// Finalize penalty module /// ~TextPenaltyModule() { Dispose(false); } ////// Explicitly clean up penalty module /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Critical - as this calls method to dispose unmanaged penalty module. /// Safe - as it does not arbitrarily set critical data. /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { if (_ploPenaltyModule.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposePenaltyModule(_ploPenaltyModule.Value); _ploPenaltyModule.Value = IntPtr.Zero; _isDisposed = true; GC.KeepAlive(this); } } ////// This method should only be called by Framework to authorize direct access to /// unsafe LS penalty module for exclusive use of PTS during optimal paragraph /// penalty calculation. /// ////// Critical - as this returns pointer to unmanaged memory owned by LS. /// [SecurityCritical] internal IntPtr DangerousGetHandle() { if (_isDisposed) { throw new ObjectDisposedException(SR.Get(SRID.TextPenaltyModuleHasBeenDisposed)); } IntPtr penaltyModuleInternalHandle; LsErr lserr = UnsafeNativeMethods.LoGetPenaltyModuleInternalHandle(_ploPenaltyModule.Value, out penaltyModuleInternalHandle); if (lserr != LsErr.None) TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.GetPenaltyModuleHandleFailure, lserr), lserr); GC.KeepAlive(this); return penaltyModuleInternalHandle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextPenaltyModule.cs // // Contents: Critical handle wrapping unmanaged text penalty module for // penalty calculation of optimal paragraph vis PTS direct access. // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 4-4-2006 Worachai Chaoweeraprasit (Wchao) // //----------------------------------------------------------------------- using System; using System.Security; using System.Windows.Media; using System.Windows.Media.TextFormatting; using System.Runtime.InteropServices; using MS.Internal.PresentationCore; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.TextFormatting { ////// Critical handle wrapper of unmanaged text penalty module. This class /// is used exclusively by Framework thru friend-access. It provides direct /// access to the underlying dangerous handle to the unmanaged resource whose /// lifetime is bound to the the underlying LS context. /// [FriendAccessAllowed] // used by Framework internal sealed class TextPenaltyModule : IDisposable { private SecurityCriticalDataForSet_ploPenaltyModule; // Pointer to LS penalty module private bool _isDisposed; /// /// This constructor is called by PInvoke when returning the critical handle /// ////// Critical - as this calls the setter of _ploPenaltyModule. /// Safe - as it does not set the value arbitrarily from the value it receives from caller. /// [SecurityCritical, SecurityTreatAsSafe] internal TextPenaltyModule(SecurityCriticalDataForSetploc) { IntPtr ploPenaltyModule; LsErr lserr = UnsafeNativeMethods.LoAcquirePenaltyModule(ploc.Value, out ploPenaltyModule); if (lserr != LsErr.None) { TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.AcquirePenaltyModuleFailure, lserr), lserr); } _ploPenaltyModule.Value = ploPenaltyModule; } /// /// Finalize penalty module /// ~TextPenaltyModule() { Dispose(false); } ////// Explicitly clean up penalty module /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ////// Critical - as this calls method to dispose unmanaged penalty module. /// Safe - as it does not arbitrarily set critical data. /// [SecurityCritical, SecurityTreatAsSafe] private void Dispose(bool disposing) { if (_ploPenaltyModule.Value != IntPtr.Zero) { UnsafeNativeMethods.LoDisposePenaltyModule(_ploPenaltyModule.Value); _ploPenaltyModule.Value = IntPtr.Zero; _isDisposed = true; GC.KeepAlive(this); } } ////// This method should only be called by Framework to authorize direct access to /// unsafe LS penalty module for exclusive use of PTS during optimal paragraph /// penalty calculation. /// ////// Critical - as this returns pointer to unmanaged memory owned by LS. /// [SecurityCritical] internal IntPtr DangerousGetHandle() { if (_isDisposed) { throw new ObjectDisposedException(SR.Get(SRID.TextPenaltyModuleHasBeenDisposed)); } IntPtr penaltyModuleInternalHandle; LsErr lserr = UnsafeNativeMethods.LoGetPenaltyModuleInternalHandle(_ploPenaltyModule.Value, out penaltyModuleInternalHandle); if (lserr != LsErr.None) TextFormatterContext.ThrowExceptionFromLsError(SR.Get(SRID.GetPenaltyModuleHandleFailure, lserr), lserr); GC.KeepAlive(this); return penaltyModuleInternalHandle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
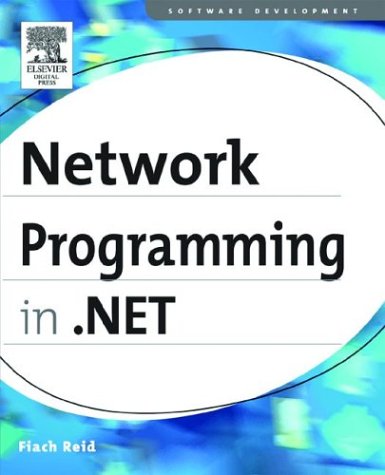
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionPrinter.cs
- DoubleLinkList.cs
- PagesChangedEventArgs.cs
- BoolExpressionVisitors.cs
- ConnectionOrientedTransportChannelFactory.cs
- InvalidOperationException.cs
- DirectionalLight.cs
- InkCanvasFeedbackAdorner.cs
- AtlasWeb.Designer.cs
- ContentTextAutomationPeer.cs
- SafeLocalMemHandle.cs
- AuthorizationRule.cs
- HttpListener.cs
- ExceptionUtility.cs
- Mappings.cs
- SearchExpression.cs
- EntityRecordInfo.cs
- RemotingConfiguration.cs
- ImpersonateTokenRef.cs
- AdapterDictionary.cs
- ResourceAttributes.cs
- CodeAttributeDeclarationCollection.cs
- BasicHttpMessageSecurity.cs
- DiagnosticsConfigurationHandler.cs
- MenuItem.cs
- formatter.cs
- CopyNodeSetAction.cs
- WpfWebRequestHelper.cs
- PackageFilter.cs
- ScrollPattern.cs
- SystemResourceHost.cs
- KeyTimeConverter.cs
- PackagePartCollection.cs
- TypeNameConverter.cs
- Panel.cs
- NamespaceEmitter.cs
- CheckBoxRenderer.cs
- ClockGroup.cs
- RadioButtonAutomationPeer.cs
- HyperLinkStyle.cs
- DebuggerService.cs
- __Filters.cs
- HashFinalRequest.cs
- RawUIStateInputReport.cs
- EntityConnection.cs
- IntegerCollectionEditor.cs
- XmlAttributeCache.cs
- Model3D.cs
- BitmapEffectGeneralTransform.cs
- DataGridColumn.cs
- ArraySortHelper.cs
- WebBrowserNavigatingEventHandler.cs
- Vector3DIndependentAnimationStorage.cs
- StringWriter.cs
- XmlAnyAttributeAttribute.cs
- TypeConverterHelper.cs
- PreloadHost.cs
- SeekStoryboard.cs
- TextSimpleMarkerProperties.cs
- SectionXmlInfo.cs
- XPathNodeList.cs
- FileNotFoundException.cs
- GeneralTransform3DGroup.cs
- DataKeyPropertyAttribute.cs
- HtmlControl.cs
- _SpnDictionary.cs
- HelpEvent.cs
- GenerateTemporaryTargetAssembly.cs
- GridSplitterAutomationPeer.cs
- MemberPath.cs
- diagnosticsswitches.cs
- ListBoxItemAutomationPeer.cs
- CallbackDebugBehavior.cs
- EmptyCollection.cs
- MemberDescriptor.cs
- SynchronousSendBindingElement.cs
- GACIdentityPermission.cs
- DifferencingCollection.cs
- ScrollItemProviderWrapper.cs
- SupportingTokenChannel.cs
- _NtlmClient.cs
- DynamicHyperLink.cs
- PartitionResolver.cs
- cookiecollection.cs
- StylusPoint.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- UrlParameterWriter.cs
- XomlCompilerError.cs
- PointAnimationBase.cs
- JumpItem.cs
- ActiveXSite.cs
- CryptoHandle.cs
- InputLanguageCollection.cs
- RoleGroupCollection.cs
- _NetRes.cs
- HandoffBehavior.cs
- DisplayMemberTemplateSelector.cs
- ToggleButton.cs
- SynchronizationContext.cs
- ButtonFieldBase.cs