Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / DescendentsWalkerBase.cs / 1 / DescendentsWalkerBase.cs
using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Utility; namespace System.Windows { ////// This is a base class to the DescendentsWalker. It is factored out so that /// FrameworkContextData can store and retrieve it from context local storage /// in a type agnostic manner. /// internal class DescendentsWalkerBase { #region Construction protected DescendentsWalkerBase(TreeWalkPriority priority) { _startNode = null; _priority = priority; _recursionDepth = 0; _nodes = new FrugalStructList(); } #endregion Construction internal bool WasVisited(DependencyObject d) { DependencyObject ancestor = d; while ((ancestor != _startNode) && (ancestor != null)) { DependencyObject logicalParent; if (FrameworkElement.DType.IsInstanceOfType(ancestor)) { FrameworkElement fe = ancestor as FrameworkElement; logicalParent = fe.Parent; // FrameworkElement DependencyObject dependencyObjectParent = VisualTreeHelper.GetParent(fe); if (dependencyObjectParent != null && logicalParent != null && dependencyObjectParent != logicalParent) { return _nodes.Contains(ancestor); } // Follow visual tree if not null otherwise we follow logical tree if (dependencyObjectParent != null) { ancestor = dependencyObjectParent; continue; } } else { // FrameworkContentElement FrameworkContentElement ancestorFCE = ancestor as FrameworkContentElement; logicalParent = (ancestorFCE != null) ? ancestorFCE.Parent : null; } ancestor = logicalParent; } return (ancestor != null); } internal DependencyObject _startNode; internal TreeWalkPriority _priority; internal FrugalStructList _nodes; internal int _recursionDepth; internal const int MAX_TREE_DEPTH = 250; } /// /// Enum specifying whether visual tree needs /// to be travesed first or the logical tree /// internal enum TreeWalkPriority { ////// Traverse Logical Tree first /// LogicalTree, ////// Traverse Visual Tree first /// VisualTree } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections; using System.Diagnostics; using System.Windows; using System.Windows.Media; using MS.Utility; namespace System.Windows { ////// This is a base class to the DescendentsWalker. It is factored out so that /// FrameworkContextData can store and retrieve it from context local storage /// in a type agnostic manner. /// internal class DescendentsWalkerBase { #region Construction protected DescendentsWalkerBase(TreeWalkPriority priority) { _startNode = null; _priority = priority; _recursionDepth = 0; _nodes = new FrugalStructList(); } #endregion Construction internal bool WasVisited(DependencyObject d) { DependencyObject ancestor = d; while ((ancestor != _startNode) && (ancestor != null)) { DependencyObject logicalParent; if (FrameworkElement.DType.IsInstanceOfType(ancestor)) { FrameworkElement fe = ancestor as FrameworkElement; logicalParent = fe.Parent; // FrameworkElement DependencyObject dependencyObjectParent = VisualTreeHelper.GetParent(fe); if (dependencyObjectParent != null && logicalParent != null && dependencyObjectParent != logicalParent) { return _nodes.Contains(ancestor); } // Follow visual tree if not null otherwise we follow logical tree if (dependencyObjectParent != null) { ancestor = dependencyObjectParent; continue; } } else { // FrameworkContentElement FrameworkContentElement ancestorFCE = ancestor as FrameworkContentElement; logicalParent = (ancestorFCE != null) ? ancestorFCE.Parent : null; } ancestor = logicalParent; } return (ancestor != null); } internal DependencyObject _startNode; internal TreeWalkPriority _priority; internal FrugalStructList _nodes; internal int _recursionDepth; internal const int MAX_TREE_DEPTH = 250; } /// /// Enum specifying whether visual tree needs /// to be travesed first or the logical tree /// internal enum TreeWalkPriority { ////// Traverse Logical Tree first /// LogicalTree, ////// Traverse Visual Tree first /// VisualTree } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
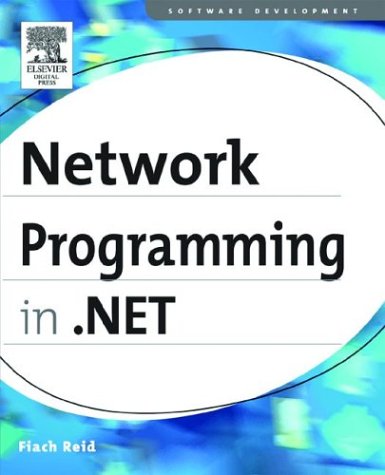
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LoginView.cs
- SafeFreeMibTable.cs
- Calendar.cs
- SqlIdentifier.cs
- SectionUpdates.cs
- DataPager.cs
- HttpDictionary.cs
- XmlAttributeProperties.cs
- AssemblyAttributesGoHere.cs
- VectorAnimation.cs
- dataprotectionpermission.cs
- SerializationObjectManager.cs
- MachineKeyConverter.cs
- SiteMapHierarchicalDataSourceView.cs
- AttachedPropertyMethodSelector.cs
- ClockController.cs
- FixedTextContainer.cs
- XmlSerializableReader.cs
- ContentControl.cs
- BrowserDefinition.cs
- WindowsFormsLinkLabel.cs
- XmlSchemaAnyAttribute.cs
- ConnectionInterfaceCollection.cs
- ToolboxService.cs
- JapaneseCalendar.cs
- PopupRootAutomationPeer.cs
- ObjectListComponentEditor.cs
- ScriptingJsonSerializationSection.cs
- SingleAnimation.cs
- XmlIlVisitor.cs
- RegexWriter.cs
- x509utils.cs
- oledbconnectionstring.cs
- ValueTypeFixupInfo.cs
- XamlReader.cs
- LineBreakRecord.cs
- Point4DConverter.cs
- login.cs
- EpmTargetTree.cs
- JsonWriter.cs
- WebZone.cs
- documentsequencetextview.cs
- TagNameToTypeMapper.cs
- ProgressBarHighlightConverter.cs
- SqlInternalConnectionTds.cs
- AuthStoreRoleProvider.cs
- PeerValidationBehavior.cs
- EnumerableRowCollectionExtensions.cs
- DrawingVisualDrawingContext.cs
- IdnElement.cs
- DefaultAssemblyResolver.cs
- SqlMethodAttribute.cs
- SourceChangedEventArgs.cs
- DataSourceControlBuilder.cs
- EncryptedKey.cs
- CollectionType.cs
- FixedPageProcessor.cs
- NavigationWindowAutomationPeer.cs
- PrefixHandle.cs
- ImageCollectionEditor.cs
- ByteViewer.cs
- DataGridViewDataConnection.cs
- CompilerWrapper.cs
- ChangeDirector.cs
- MemberInfoSerializationHolder.cs
- Token.cs
- DashStyles.cs
- TemplateXamlTreeBuilder.cs
- SqlStream.cs
- UserCancellationException.cs
- BasicCellRelation.cs
- Setter.cs
- NTAccount.cs
- TransactionsSectionGroup.cs
- TrackingServices.cs
- NumberFunctions.cs
- HttpResponse.cs
- ComponentResourceKey.cs
- Symbol.cs
- Policy.cs
- RectValueSerializer.cs
- BrowserCapabilitiesFactory.cs
- InArgumentConverter.cs
- PageAdapter.cs
- FileLogRecord.cs
- SecurityProtocol.cs
- ConfigurationCollectionAttribute.cs
- Metafile.cs
- DataBindEngine.cs
- FirewallWrapper.cs
- ImageList.cs
- HideDisabledControlAdapter.cs
- XmlWriterTraceListener.cs
- ReturnEventArgs.cs
- PolyQuadraticBezierSegment.cs
- DLinqTableProvider.cs
- SettingsPropertyNotFoundException.cs
- FamilyCollection.cs
- RegisteredHiddenField.cs
- MaterializeFromAtom.cs