Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / xsp / System / Web / UI / PersistChildrenAttribute.cs / 1 / PersistChildrenAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class PersistChildrenAttribute : Attribute { ////// Indicates whether /// the contents within a tag representing a custom /// or Web control should be treated as literal text. Web controls supporting complex properties, like /// templates, and /// so on, typically mark themselves as "literals", thereby letting the designer /// infra-structure deal with the persistence of those attributes. ////// public static readonly PersistChildrenAttribute Yes = new PersistChildrenAttribute(true); ///Indicates that the children of a control should be persisted at design-time. /// ////// public static readonly PersistChildrenAttribute No = new PersistChildrenAttribute(false); ///Indicates that the children of a control should not be persisted at design-time. ////// This marks the default child persistence behavior for a control at design time. (equal to Yes.) /// public static readonly PersistChildrenAttribute Default = Yes; private bool _persist; private bool _usesCustomPersistence; ////// public PersistChildrenAttribute(bool persist) { _persist = persist; } public PersistChildrenAttribute(bool persist, bool usesCustomPersistence) : this(persist) { _usesCustomPersistence = usesCustomPersistence; } ////// public bool Persist { get { return _persist; } } ///Indicates whether the children of a control should be persisted at design-time. /// This property is read-only. ////// public bool UsesCustomPersistence { get { // if persist is true, we don't use custom persistence. return !_persist && _usesCustomPersistence; } } ///Indicates whether the control does custom persistence. /// This property is read-only. ////// ///public override int GetHashCode() { return Persist.GetHashCode(); } /// /// ///public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is PersistChildrenAttribute)) { return ((PersistChildrenAttribute)obj).Persist == _persist; } return false; } /// /// ///public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.ComponentModel; using System.Security.Permissions; ////// [AttributeUsage(AttributeTargets.Class)] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class PersistChildrenAttribute : Attribute { ////// Indicates whether /// the contents within a tag representing a custom /// or Web control should be treated as literal text. Web controls supporting complex properties, like /// templates, and /// so on, typically mark themselves as "literals", thereby letting the designer /// infra-structure deal with the persistence of those attributes. ////// public static readonly PersistChildrenAttribute Yes = new PersistChildrenAttribute(true); ///Indicates that the children of a control should be persisted at design-time. /// ////// public static readonly PersistChildrenAttribute No = new PersistChildrenAttribute(false); ///Indicates that the children of a control should not be persisted at design-time. ////// This marks the default child persistence behavior for a control at design time. (equal to Yes.) /// public static readonly PersistChildrenAttribute Default = Yes; private bool _persist; private bool _usesCustomPersistence; ////// public PersistChildrenAttribute(bool persist) { _persist = persist; } public PersistChildrenAttribute(bool persist, bool usesCustomPersistence) : this(persist) { _usesCustomPersistence = usesCustomPersistence; } ////// public bool Persist { get { return _persist; } } ///Indicates whether the children of a control should be persisted at design-time. /// This property is read-only. ////// public bool UsesCustomPersistence { get { // if persist is true, we don't use custom persistence. return !_persist && _usesCustomPersistence; } } ///Indicates whether the control does custom persistence. /// This property is read-only. ////// ///public override int GetHashCode() { return Persist.GetHashCode(); } /// /// ///public override bool Equals(object obj) { if (obj == this) { return true; } if ((obj != null) && (obj is PersistChildrenAttribute)) { return ((PersistChildrenAttribute)obj).Persist == _persist; } return false; } /// /// ///public override bool IsDefaultAttribute() { return this.Equals(Default); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
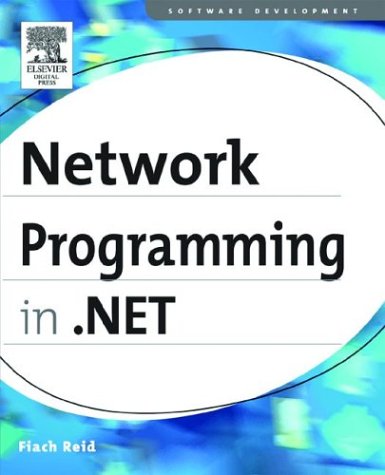
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityDataSourceMemberPath.cs
- XmlHelper.cs
- DataViewManagerListItemTypeDescriptor.cs
- RegexGroup.cs
- SqlSelectStatement.cs
- PathParser.cs
- Char.cs
- ReverseInheritProperty.cs
- RegexCode.cs
- SchemaTypeEmitter.cs
- UTF32Encoding.cs
- ProviderBase.cs
- DatatypeImplementation.cs
- ClientSponsor.cs
- XmlSchemaAnyAttribute.cs
- DataGridParentRows.cs
- TaskResultSetter.cs
- DeferredElementTreeState.cs
- NullableBoolConverter.cs
- TableCellCollection.cs
- shaperfactoryquerycachekey.cs
- RegexTypeEditor.cs
- SqlDataSourceConfigureSortForm.cs
- VirtualDirectoryMappingCollection.cs
- Item.cs
- MarkupObject.cs
- MulticastDelegate.cs
- RowBinding.cs
- WebConfigurationHostFileChange.cs
- Trace.cs
- XmlReflectionMember.cs
- XmlSchemaInclude.cs
- GACIdentityPermission.cs
- DataServiceResponse.cs
- XmlSchemaResource.cs
- Rect.cs
- NameSpaceExtractor.cs
- SqlDelegatedTransaction.cs
- ExpressionBuilder.cs
- ContentFilePart.cs
- ParserOptions.cs
- TriggerCollection.cs
- BamlTreeUpdater.cs
- GridViewRowEventArgs.cs
- DataGridCell.cs
- ConvertEvent.cs
- LiteralControl.cs
- XmlSchemaExporter.cs
- KnownBoxes.cs
- XmlSerializableReader.cs
- IChannel.cs
- WsdlBuildProvider.cs
- NavigatingCancelEventArgs.cs
- TargetParameterCountException.cs
- safelink.cs
- LogReservationCollection.cs
- OracleRowUpdatedEventArgs.cs
- PropertyChangedEventArgs.cs
- Page.cs
- SchemeSettingElement.cs
- PolygonHotSpot.cs
- DataObjectEventArgs.cs
- ExitEventArgs.cs
- FirstMatchCodeGroup.cs
- SspiSecurityTokenProvider.cs
- ResourcePool.cs
- SafeRightsManagementEnvironmentHandle.cs
- SvcMapFile.cs
- Stack.cs
- PointLightBase.cs
- ExistsInCollection.cs
- Light.cs
- SiteMapDataSourceView.cs
- PageThemeParser.cs
- FontStyles.cs
- ComponentChangedEvent.cs
- RoleGroup.cs
- DictionarySectionHandler.cs
- SafeLibraryHandle.cs
- EntityClientCacheKey.cs
- Paragraph.cs
- TargetInvocationException.cs
- InvalidComObjectException.cs
- ExcCanonicalXml.cs
- HtmlInputButton.cs
- DecimalKeyFrameCollection.cs
- ProviderManager.cs
- MarginCollapsingState.cs
- HyperlinkAutomationPeer.cs
- DetailsViewInsertEventArgs.cs
- RelatedPropertyManager.cs
- FlowDocumentFormatter.cs
- Ops.cs
- DataBinding.cs
- NetMsmqSecurityMode.cs
- FontNamesConverter.cs
- SHA512Managed.cs
- SoapAttributeAttribute.cs
- EventLogPermission.cs
- SecurityHelper.cs