Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / Security / Principal / NTAccount.cs / 1 / NTAccount.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System; using System.Runtime.InteropServices; using System.Globalization; using System.Security.Permissions; namespace System.Security.Principal { [System.Runtime.InteropServices.ComVisible(false)] public sealed class NTAccount : IdentityReference { #region Private members private readonly string _Name; // // Limit for nt account names for users is 20 while that for groups is 256 // internal const int MaximumAccountNameLength = 256; // // Limit for dns domain names is 255 // internal const int MaximumDomainNameLength = 255; #endregion #region Constructors public NTAccount( string domainName, string accountName ) { if ( accountName == null ) { throw new ArgumentNullException( "accountName" ); } if ( accountName.Length == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Argument_StringZeroLength" ), "accountName" ); } if ( accountName.Length > MaximumAccountNameLength ) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_AccountNameTooLong" ), "accountName"); } if ( domainName != null && domainName.Length > MaximumDomainNameLength ) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_DomainNameTooLong" ), "domainName"); } if ( domainName == null || domainName.Length == 0 ) { _Name = accountName; } else { _Name = domainName + "\\" + accountName; } } public NTAccount( string name ) { if ( name == null ) { throw new ArgumentNullException( "name" ); } if ( name.Length == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Argument_StringZeroLength" ), "name" ); } if ( name.Length > ( MaximumDomainNameLength + 1 /* '\' */ + MaximumAccountNameLength )) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_AccountNameTooLong" ), "name"); } _Name = name; } #endregion #region Inherited properties and methods #if false public override string Scheme { get { return "ms-ntaccount"; } } #endif public override string Value { get { return ToString(); } } public override bool IsValidTargetType( Type targetType ) { if ( targetType == typeof( SecurityIdentifier )) { return true; } else if ( targetType == typeof( NTAccount )) { return true; } else { return false; } } public override IdentityReference Translate( Type targetType ) { if ( targetType == null ) { throw new ArgumentNullException( "targetType" ); } if ( targetType == typeof( NTAccount )) { return this; // assumes that NTAccount objects are immutable } else if ( targetType == typeof( SecurityIdentifier )) { IdentityReferenceCollection irSource = new IdentityReferenceCollection( 1 ); irSource.Add( this ); IdentityReferenceCollection irTarget; irTarget = NTAccount.Translate( irSource, targetType, true ); return irTarget[0]; } else { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_MustBeIdentityReference" ), "targetType" ); } } public override bool Equals( object o ) { if ( o == null ) { return false; } NTAccount nta = o as NTAccount; if ( nta == null ) { return false; } return ( this == nta ); // invokes operator== } public override int GetHashCode() { return StringComparer.InvariantCultureIgnoreCase.GetHashCode(_Name); } public override string ToString() { return _Name; } internal static IdentityReferenceCollection Translate( IdentityReferenceCollection sourceAccounts, Type targetType, bool forceSuccess) { bool SomeFailed = false; IdentityReferenceCollection Result; Result = Translate( sourceAccounts, targetType, out SomeFailed ); if (forceSuccess && SomeFailed) { IdentityReferenceCollection UnmappedIdentities = new IdentityReferenceCollection(); foreach (IdentityReference id in Result) { if (id.GetType() != targetType) { UnmappedIdentities.Add(id); } } throw new IdentityNotMappedException(Environment.GetResourceString("IdentityReference_IdentityNotMapped"), UnmappedIdentities); } return Result; } internal static IdentityReferenceCollection Translate( IdentityReferenceCollection sourceAccounts, Type targetType, out bool someFailed ) { if ( sourceAccounts == null ) { throw new ArgumentNullException( "sourceAccounts" ); } if ( targetType == typeof( SecurityIdentifier )) { return TranslateToSids( sourceAccounts, out someFailed ); } throw new ArgumentException( Environment.GetResourceString( "IdentityReference_MustBeIdentityReference" ), "targetType" ); } #endregion #region Operators public static bool operator==( NTAccount left, NTAccount right ) { object l = left; object r = right; if ( l == null && r == null ) { return true; } else if ( l == null || r == null ) { return false; } else { return ( left.ToString().Equals(right.ToString(), StringComparison.OrdinalIgnoreCase)); } } public static bool operator!=( NTAccount left, NTAccount right ) { return !( left == right ); // invoke operator== } #endregion #region Private methods private static IdentityReferenceCollection TranslateToSids( IdentityReferenceCollection sourceAccounts, out bool someFailed ) { // // Check if the lsa api is supported // if (!Win32.LsaApisSupported) { throw new PlatformNotSupportedException( Environment.GetResourceString( "PlatformNotSupported_Win9x" )); } SafeLsaPolicyHandle LsaHandle = SafeLsaPolicyHandle.InvalidHandle; SafeLsaMemoryHandle ReferencedDomainsPtr = SafeLsaMemoryHandle.InvalidHandle; SafeLsaMemoryHandle SidsPtr = SafeLsaMemoryHandle.InvalidHandle; int i = 0; if ( sourceAccounts == null ) { throw new ArgumentNullException( "sourceAccounts" ); } if ( sourceAccounts.Count == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Arg_EmptyCollection" ), "sourceAccounts" ); } try { // // Construct an array of unicode strings // Win32Native.UNICODE_STRING[] Names = new Win32Native.UNICODE_STRING[ sourceAccounts.Count ]; foreach ( IdentityReference id in sourceAccounts ) { NTAccount nta = id as NTAccount; if ( nta == null ) { throw new ArgumentException( Environment.GetResourceString( "Argument_ImproperType" ), "sourceAccounts" ); } Names[i].Buffer = nta.ToString(); if ( Names[i].Buffer.Length * 2 + 2 > ushort.MaxValue ) { // this should never happen since we are already validating account name length in constructor and // it is less than this limit BCLDebug.Assert(false, "NTAccount::TranslateToSids - source account name is too long."); throw new SystemException(); } Names[i].Length = ( ushort )( Names[i].Buffer.Length * 2 ); Names[i].MaximumLength = ( ushort )( Names[i].Length + 2 ); i++; } // // Open LSA policy (for lookup requires it) // LsaHandle = Win32.LsaOpenPolicy( null, PolicyRights.POLICY_LOOKUP_NAMES ); // // Now perform the actual lookup // someFailed = false; uint ReturnCode; if ( Win32.LsaLookupNames2Supported ) { ReturnCode = Win32Native.LsaLookupNames2( LsaHandle, 0, sourceAccounts.Count, Names, ref ReferencedDomainsPtr, ref SidsPtr ); } else { ReturnCode = Win32Native.LsaLookupNames( LsaHandle, sourceAccounts.Count, Names, ref ReferencedDomainsPtr, ref SidsPtr ); } // // Make a decision regarding whether it makes sense to proceed // based on the return code and the value of the forceSuccess argument // if ( ReturnCode == Win32Native.STATUS_NO_MEMORY || ReturnCode == Win32Native.STATUS_INSUFFICIENT_RESOURCES ) { throw new OutOfMemoryException(); } else if ( ReturnCode == Win32Native.STATUS_ACCESS_DENIED ) { throw new UnauthorizedAccessException(); } else if ( ReturnCode == Win32Native.STATUS_NONE_MAPPED || ReturnCode == Win32Native.STATUS_SOME_NOT_MAPPED ) { someFailed = true; } else if ( ReturnCode != 0 ) { int win32ErrorCode = Win32Native.LsaNtStatusToWinError(unchecked((int)ReturnCode)); BCLDebug.Assert( false, string.Format( CultureInfo.InvariantCulture, "Win32Native.LsaLookupNames(2) returned unrecognized error {0}", win32ErrorCode )); throw new SystemException(Win32Native.GetMessage(win32ErrorCode)); } // // Interpret the results and generate SID objects // IdentityReferenceCollection Result = new IdentityReferenceCollection( sourceAccounts.Count ); if ( ReturnCode == 0 || ReturnCode == Win32Native.STATUS_SOME_NOT_MAPPED ) { if ( Win32.LsaLookupNames2Supported ) { for ( i = 0; i < sourceAccounts.Count; i++ ) { Win32Native.LSA_TRANSLATED_SID2 Lts; SidNameUse Use; Lts = ( Win32Native.LSA_TRANSLATED_SID2 )Marshal.PtrToStructure( new IntPtr(( long )SidsPtr.DangerousGetHandle() + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRANSLATED_SID2 ))), typeof( Win32Native.LSA_TRANSLATED_SID2 )); Use = ( SidNameUse )Lts.Use; // // Only some names are recognized as NTAccount objects // switch ( Use ) { case SidNameUse.User: goto case SidNameUse.WellKnownGroup; case SidNameUse.Group: goto case SidNameUse.WellKnownGroup; case SidNameUse.Alias: goto case SidNameUse.WellKnownGroup; case SidNameUse.Computer: goto case SidNameUse.WellKnownGroup; case SidNameUse.WellKnownGroup: Result.Add( new SecurityIdentifier( Lts.Sid, true )); break; default: someFailed = true; Result.Add( sourceAccounts[i] ); break; } } } else { Win32Native.LSA_REFERENCED_DOMAIN_LIST rdl = ( Win32Native.LSA_REFERENCED_DOMAIN_LIST )Marshal.PtrToStructure( ReferencedDomainsPtr.DangerousGetHandle(), typeof( Win32Native.LSA_REFERENCED_DOMAIN_LIST )); SecurityIdentifier[] ReferencedDomains = new SecurityIdentifier[ rdl.Entries ]; for ( i = 0; i < rdl.Entries; i++ ) { Win32Native.LSA_TRUST_INFORMATION ti = ( Win32Native.LSA_TRUST_INFORMATION )Marshal.PtrToStructure( new IntPtr(( long )rdl.Domains + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRUST_INFORMATION ))), typeof( Win32Native.LSA_TRUST_INFORMATION )); ReferencedDomains[i] = new SecurityIdentifier( ti.Sid, true ); } for ( i = 0; i < sourceAccounts.Count; i++ ) { Win32Native.LSA_TRANSLATED_SID Lts; SidNameUse Use; Lts = ( Win32Native.LSA_TRANSLATED_SID )Marshal.PtrToStructure( new IntPtr(( long )SidsPtr.DangerousGetHandle() + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRANSLATED_SID ))), typeof( Win32Native.LSA_TRANSLATED_SID )); Use = ( SidNameUse )Lts.Use; switch ( Use ) { case SidNameUse.User: goto case SidNameUse.WellKnownGroup; case SidNameUse.Group: goto case SidNameUse.WellKnownGroup; case SidNameUse.Alias: goto case SidNameUse.WellKnownGroup; case SidNameUse.Computer: goto case SidNameUse.WellKnownGroup; case SidNameUse.WellKnownGroup: Result.Add( new SecurityIdentifier( ReferencedDomains[ Lts.DomainIndex ], Lts.Rid )); break; default: someFailed = true; Result.Add( sourceAccounts[i] ); break; } } } } else { for ( i = 0; i < sourceAccounts.Count; i++ ) { Result.Add( sourceAccounts[i] ); } } return Result; } finally { LsaHandle.Dispose(); ReferencedDomainsPtr.Dispose(); SidsPtr.Dispose(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using Microsoft.Win32; using Microsoft.Win32.SafeHandles; using System; using System.Runtime.InteropServices; using System.Globalization; using System.Security.Permissions; namespace System.Security.Principal { [System.Runtime.InteropServices.ComVisible(false)] public sealed class NTAccount : IdentityReference { #region Private members private readonly string _Name; // // Limit for nt account names for users is 20 while that for groups is 256 // internal const int MaximumAccountNameLength = 256; // // Limit for dns domain names is 255 // internal const int MaximumDomainNameLength = 255; #endregion #region Constructors public NTAccount( string domainName, string accountName ) { if ( accountName == null ) { throw new ArgumentNullException( "accountName" ); } if ( accountName.Length == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Argument_StringZeroLength" ), "accountName" ); } if ( accountName.Length > MaximumAccountNameLength ) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_AccountNameTooLong" ), "accountName"); } if ( domainName != null && domainName.Length > MaximumDomainNameLength ) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_DomainNameTooLong" ), "domainName"); } if ( domainName == null || domainName.Length == 0 ) { _Name = accountName; } else { _Name = domainName + "\\" + accountName; } } public NTAccount( string name ) { if ( name == null ) { throw new ArgumentNullException( "name" ); } if ( name.Length == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Argument_StringZeroLength" ), "name" ); } if ( name.Length > ( MaximumDomainNameLength + 1 /* '\' */ + MaximumAccountNameLength )) { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_AccountNameTooLong" ), "name"); } _Name = name; } #endregion #region Inherited properties and methods #if false public override string Scheme { get { return "ms-ntaccount"; } } #endif public override string Value { get { return ToString(); } } public override bool IsValidTargetType( Type targetType ) { if ( targetType == typeof( SecurityIdentifier )) { return true; } else if ( targetType == typeof( NTAccount )) { return true; } else { return false; } } public override IdentityReference Translate( Type targetType ) { if ( targetType == null ) { throw new ArgumentNullException( "targetType" ); } if ( targetType == typeof( NTAccount )) { return this; // assumes that NTAccount objects are immutable } else if ( targetType == typeof( SecurityIdentifier )) { IdentityReferenceCollection irSource = new IdentityReferenceCollection( 1 ); irSource.Add( this ); IdentityReferenceCollection irTarget; irTarget = NTAccount.Translate( irSource, targetType, true ); return irTarget[0]; } else { throw new ArgumentException( Environment.GetResourceString( "IdentityReference_MustBeIdentityReference" ), "targetType" ); } } public override bool Equals( object o ) { if ( o == null ) { return false; } NTAccount nta = o as NTAccount; if ( nta == null ) { return false; } return ( this == nta ); // invokes operator== } public override int GetHashCode() { return StringComparer.InvariantCultureIgnoreCase.GetHashCode(_Name); } public override string ToString() { return _Name; } internal static IdentityReferenceCollection Translate( IdentityReferenceCollection sourceAccounts, Type targetType, bool forceSuccess) { bool SomeFailed = false; IdentityReferenceCollection Result; Result = Translate( sourceAccounts, targetType, out SomeFailed ); if (forceSuccess && SomeFailed) { IdentityReferenceCollection UnmappedIdentities = new IdentityReferenceCollection(); foreach (IdentityReference id in Result) { if (id.GetType() != targetType) { UnmappedIdentities.Add(id); } } throw new IdentityNotMappedException(Environment.GetResourceString("IdentityReference_IdentityNotMapped"), UnmappedIdentities); } return Result; } internal static IdentityReferenceCollection Translate( IdentityReferenceCollection sourceAccounts, Type targetType, out bool someFailed ) { if ( sourceAccounts == null ) { throw new ArgumentNullException( "sourceAccounts" ); } if ( targetType == typeof( SecurityIdentifier )) { return TranslateToSids( sourceAccounts, out someFailed ); } throw new ArgumentException( Environment.GetResourceString( "IdentityReference_MustBeIdentityReference" ), "targetType" ); } #endregion #region Operators public static bool operator==( NTAccount left, NTAccount right ) { object l = left; object r = right; if ( l == null && r == null ) { return true; } else if ( l == null || r == null ) { return false; } else { return ( left.ToString().Equals(right.ToString(), StringComparison.OrdinalIgnoreCase)); } } public static bool operator!=( NTAccount left, NTAccount right ) { return !( left == right ); // invoke operator== } #endregion #region Private methods private static IdentityReferenceCollection TranslateToSids( IdentityReferenceCollection sourceAccounts, out bool someFailed ) { // // Check if the lsa api is supported // if (!Win32.LsaApisSupported) { throw new PlatformNotSupportedException( Environment.GetResourceString( "PlatformNotSupported_Win9x" )); } SafeLsaPolicyHandle LsaHandle = SafeLsaPolicyHandle.InvalidHandle; SafeLsaMemoryHandle ReferencedDomainsPtr = SafeLsaMemoryHandle.InvalidHandle; SafeLsaMemoryHandle SidsPtr = SafeLsaMemoryHandle.InvalidHandle; int i = 0; if ( sourceAccounts == null ) { throw new ArgumentNullException( "sourceAccounts" ); } if ( sourceAccounts.Count == 0 ) { throw new ArgumentException( Environment.GetResourceString( "Arg_EmptyCollection" ), "sourceAccounts" ); } try { // // Construct an array of unicode strings // Win32Native.UNICODE_STRING[] Names = new Win32Native.UNICODE_STRING[ sourceAccounts.Count ]; foreach ( IdentityReference id in sourceAccounts ) { NTAccount nta = id as NTAccount; if ( nta == null ) { throw new ArgumentException( Environment.GetResourceString( "Argument_ImproperType" ), "sourceAccounts" ); } Names[i].Buffer = nta.ToString(); if ( Names[i].Buffer.Length * 2 + 2 > ushort.MaxValue ) { // this should never happen since we are already validating account name length in constructor and // it is less than this limit BCLDebug.Assert(false, "NTAccount::TranslateToSids - source account name is too long."); throw new SystemException(); } Names[i].Length = ( ushort )( Names[i].Buffer.Length * 2 ); Names[i].MaximumLength = ( ushort )( Names[i].Length + 2 ); i++; } // // Open LSA policy (for lookup requires it) // LsaHandle = Win32.LsaOpenPolicy( null, PolicyRights.POLICY_LOOKUP_NAMES ); // // Now perform the actual lookup // someFailed = false; uint ReturnCode; if ( Win32.LsaLookupNames2Supported ) { ReturnCode = Win32Native.LsaLookupNames2( LsaHandle, 0, sourceAccounts.Count, Names, ref ReferencedDomainsPtr, ref SidsPtr ); } else { ReturnCode = Win32Native.LsaLookupNames( LsaHandle, sourceAccounts.Count, Names, ref ReferencedDomainsPtr, ref SidsPtr ); } // // Make a decision regarding whether it makes sense to proceed // based on the return code and the value of the forceSuccess argument // if ( ReturnCode == Win32Native.STATUS_NO_MEMORY || ReturnCode == Win32Native.STATUS_INSUFFICIENT_RESOURCES ) { throw new OutOfMemoryException(); } else if ( ReturnCode == Win32Native.STATUS_ACCESS_DENIED ) { throw new UnauthorizedAccessException(); } else if ( ReturnCode == Win32Native.STATUS_NONE_MAPPED || ReturnCode == Win32Native.STATUS_SOME_NOT_MAPPED ) { someFailed = true; } else if ( ReturnCode != 0 ) { int win32ErrorCode = Win32Native.LsaNtStatusToWinError(unchecked((int)ReturnCode)); BCLDebug.Assert( false, string.Format( CultureInfo.InvariantCulture, "Win32Native.LsaLookupNames(2) returned unrecognized error {0}", win32ErrorCode )); throw new SystemException(Win32Native.GetMessage(win32ErrorCode)); } // // Interpret the results and generate SID objects // IdentityReferenceCollection Result = new IdentityReferenceCollection( sourceAccounts.Count ); if ( ReturnCode == 0 || ReturnCode == Win32Native.STATUS_SOME_NOT_MAPPED ) { if ( Win32.LsaLookupNames2Supported ) { for ( i = 0; i < sourceAccounts.Count; i++ ) { Win32Native.LSA_TRANSLATED_SID2 Lts; SidNameUse Use; Lts = ( Win32Native.LSA_TRANSLATED_SID2 )Marshal.PtrToStructure( new IntPtr(( long )SidsPtr.DangerousGetHandle() + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRANSLATED_SID2 ))), typeof( Win32Native.LSA_TRANSLATED_SID2 )); Use = ( SidNameUse )Lts.Use; // // Only some names are recognized as NTAccount objects // switch ( Use ) { case SidNameUse.User: goto case SidNameUse.WellKnownGroup; case SidNameUse.Group: goto case SidNameUse.WellKnownGroup; case SidNameUse.Alias: goto case SidNameUse.WellKnownGroup; case SidNameUse.Computer: goto case SidNameUse.WellKnownGroup; case SidNameUse.WellKnownGroup: Result.Add( new SecurityIdentifier( Lts.Sid, true )); break; default: someFailed = true; Result.Add( sourceAccounts[i] ); break; } } } else { Win32Native.LSA_REFERENCED_DOMAIN_LIST rdl = ( Win32Native.LSA_REFERENCED_DOMAIN_LIST )Marshal.PtrToStructure( ReferencedDomainsPtr.DangerousGetHandle(), typeof( Win32Native.LSA_REFERENCED_DOMAIN_LIST )); SecurityIdentifier[] ReferencedDomains = new SecurityIdentifier[ rdl.Entries ]; for ( i = 0; i < rdl.Entries; i++ ) { Win32Native.LSA_TRUST_INFORMATION ti = ( Win32Native.LSA_TRUST_INFORMATION )Marshal.PtrToStructure( new IntPtr(( long )rdl.Domains + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRUST_INFORMATION ))), typeof( Win32Native.LSA_TRUST_INFORMATION )); ReferencedDomains[i] = new SecurityIdentifier( ti.Sid, true ); } for ( i = 0; i < sourceAccounts.Count; i++ ) { Win32Native.LSA_TRANSLATED_SID Lts; SidNameUse Use; Lts = ( Win32Native.LSA_TRANSLATED_SID )Marshal.PtrToStructure( new IntPtr(( long )SidsPtr.DangerousGetHandle() + i * Marshal.SizeOf( typeof( Win32Native.LSA_TRANSLATED_SID ))), typeof( Win32Native.LSA_TRANSLATED_SID )); Use = ( SidNameUse )Lts.Use; switch ( Use ) { case SidNameUse.User: goto case SidNameUse.WellKnownGroup; case SidNameUse.Group: goto case SidNameUse.WellKnownGroup; case SidNameUse.Alias: goto case SidNameUse.WellKnownGroup; case SidNameUse.Computer: goto case SidNameUse.WellKnownGroup; case SidNameUse.WellKnownGroup: Result.Add( new SecurityIdentifier( ReferencedDomains[ Lts.DomainIndex ], Lts.Rid )); break; default: someFailed = true; Result.Add( sourceAccounts[i] ); break; } } } } else { for ( i = 0; i < sourceAccounts.Count; i++ ) { Result.Add( sourceAccounts[i] ); } } return Result; } finally { LsaHandle.Dispose(); ReferencedDomainsPtr.Dispose(); SidsPtr.Dispose(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
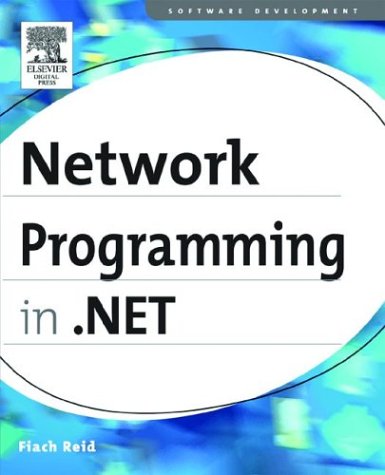
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NetSectionGroup.cs
- WindowsTitleBar.cs
- DynamicValueConverter.cs
- OutArgumentConverter.cs
- XmlQueryTypeFactory.cs
- WindowsEditBoxRange.cs
- StringArrayConverter.cs
- BuildResultCache.cs
- SoapSchemaMember.cs
- ChildTable.cs
- XmlTextWriter.cs
- DataGridItemCollection.cs
- Scripts.cs
- Rotation3D.cs
- StagingAreaInputItem.cs
- InertiaRotationBehavior.cs
- RegexFCD.cs
- ToolboxDataAttribute.cs
- Speller.cs
- TextTreeDeleteContentUndoUnit.cs
- SymmetricKey.cs
- LineServices.cs
- Verify.cs
- UnsafeNativeMethods.cs
- EmptyEnumerator.cs
- DbCommandTree.cs
- LingerOption.cs
- ContractMethodParameterInfo.cs
- ConfigurationValidatorAttribute.cs
- ParentUndoUnit.cs
- IndicCharClassifier.cs
- PageParser.cs
- ConfigXmlText.cs
- AnonymousIdentificationModule.cs
- DetailsViewPagerRow.cs
- PropertyGeneratedEventArgs.cs
- Math.cs
- DataViewSettingCollection.cs
- CodeCatchClause.cs
- ListView.cs
- ExpressionVisitor.cs
- TextContainerHelper.cs
- SQLBinary.cs
- BindingEntityInfo.cs
- X509UI.cs
- StyleSheetComponentEditor.cs
- TextTrailingCharacterEllipsis.cs
- odbcmetadatacollectionnames.cs
- SrgsDocumentParser.cs
- CultureInfoConverter.cs
- Selection.cs
- ActivityExecutorOperation.cs
- XamlPointCollectionSerializer.cs
- SharedUtils.cs
- DLinqDataModelProvider.cs
- OleAutBinder.cs
- EncoderExceptionFallback.cs
- ExpressionPrefixAttribute.cs
- AccessControlEntry.cs
- QilPatternFactory.cs
- DataGridViewCellStyleBuilderDialog.cs
- AnnotationResource.cs
- DbCommandTree.cs
- CodeSubDirectory.cs
- CheckBoxStandardAdapter.cs
- ObjectListSelectEventArgs.cs
- basemetadatamappingvisitor.cs
- hebrewshape.cs
- DiscoveryClientBindingElement.cs
- ImageFormat.cs
- OracleTransaction.cs
- XmlEncApr2001.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- DateTimeFormat.cs
- RemotingException.cs
- LinkLabelLinkClickedEvent.cs
- TcpChannelHelper.cs
- EdmComplexTypeAttribute.cs
- SizeLimitedCache.cs
- WebPartActionVerb.cs
- OrderByBuilder.cs
- FragmentQuery.cs
- ReadOnlyAttribute.cs
- SspiWrapper.cs
- EntityExpressionVisitor.cs
- LayoutDump.cs
- WrapPanel.cs
- TransformedBitmap.cs
- HttpsHostedTransportConfiguration.cs
- Logging.cs
- ConfigurationCollectionAttribute.cs
- TextPenaltyModule.cs
- MenuCommands.cs
- ConfigurationUtility.cs
- StringBlob.cs
- DBProviderConfigurationHandler.cs
- SamlAuthenticationStatement.cs
- TargetControlTypeAttribute.cs
- ReadOnlyCollection.cs
- ControlFilterExpression.cs