Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Design / UITypeEditor.cs / 1 / UITypeEditor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Collections.Generic; using System.Collections.ObjectModel; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class UITypeEditor { ///Provides a base class for editors /// that may provide users with a user interface to visually edit /// the values of the supported type or types. ////// /// In this static constructor we provide default UITypeEditors to /// the TypeDescriptor. /// static UITypeEditor() { Hashtable intrinsicEditors = new Hashtable(); // Our set of intrinsic editors. intrinsicEditors[typeof(DateTime)] = "System.ComponentModel.Design.DateTimeEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Array)] = "System.ComponentModel.Design.ArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(IList)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(ICollection)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(byte[])] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(System.IO.Stream)] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(string[])] = "System.Windows.Forms.Design.StringArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Collection)] = "System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign; // Add our intrinsic editors to TypeDescriptor. // TypeDescriptor.AddEditorTable(typeof(UITypeEditor), intrinsicEditors); } /// /// /// public UITypeEditor() { } ////// Initializes /// a new instance of the ///class. /// /// /// public virtual bool IsDropDownResizable { get { return false; } } ////// Determines if drop-down editors should be resizable by the user. /// ////// /// public object EditValue(IServiceProvider provider, object value) { return EditValue(null, provider, value); } ///Edits the specified value using the editor style /// provided by ///. /// /// public virtual object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { return value; } ///Edits the specified object's value using the editor style /// provided by ///. /// /// public UITypeEditorEditStyle GetEditStyle() { return GetEditStyle(null); } ////// Gets the ////// of the Edit method. /// /// /// public bool GetPaintValueSupported() { return GetPaintValueSupported(null); } ///Gets a value indicating whether this editor supports painting a representation /// of an object's value. ////// /// public virtual bool GetPaintValueSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the specified context supports painting a representation /// of an object's value. ////// /// public virtual UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.None; } ////// Gets the editing style of the Edit method. /// ////// /// public void PaintValue(object value, Graphics canvas, Rectangle rectangle) { PaintValue(new PaintValueEventArgs(null, value, canvas, rectangle)); } ///Paints a representative value of the specified object to the /// specified canvas. ////// /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual void PaintValue(PaintValueEventArgs e) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Paints a representative value of the specified object to the /// provided canvas. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Drawing.Design { using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Collections; using Microsoft.Win32; using System.ComponentModel.Design; using System.Drawing; using System.Collections.Generic; using System.Collections.ObjectModel; ////// /// [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.InheritanceDemand, Name="FullTrust")] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name="FullTrust")] public class UITypeEditor { ///Provides a base class for editors /// that may provide users with a user interface to visually edit /// the values of the supported type or types. ////// /// In this static constructor we provide default UITypeEditors to /// the TypeDescriptor. /// static UITypeEditor() { Hashtable intrinsicEditors = new Hashtable(); // Our set of intrinsic editors. intrinsicEditors[typeof(DateTime)] = "System.ComponentModel.Design.DateTimeEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Array)] = "System.ComponentModel.Design.ArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(IList)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(ICollection)] = "System.ComponentModel.Design.CollectionEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(byte[])] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(System.IO.Stream)] = "System.ComponentModel.Design.BinaryEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(string[])] = "System.Windows.Forms.Design.StringArrayEditor, " + AssemblyRef.SystemDesign; intrinsicEditors[typeof(Collection)] = "System.Windows.Forms.Design.StringCollectionEditor, " + AssemblyRef.SystemDesign; // Add our intrinsic editors to TypeDescriptor. // TypeDescriptor.AddEditorTable(typeof(UITypeEditor), intrinsicEditors); } /// /// /// public UITypeEditor() { } ////// Initializes /// a new instance of the ///class. /// /// /// public virtual bool IsDropDownResizable { get { return false; } } ////// Determines if drop-down editors should be resizable by the user. /// ////// /// public object EditValue(IServiceProvider provider, object value) { return EditValue(null, provider, value); } ///Edits the specified value using the editor style /// provided by ///. /// /// public virtual object EditValue(ITypeDescriptorContext context, IServiceProvider provider, object value) { return value; } ///Edits the specified object's value using the editor style /// provided by ///. /// /// public UITypeEditorEditStyle GetEditStyle() { return GetEditStyle(null); } ////// Gets the ////// of the Edit method. /// /// /// public bool GetPaintValueSupported() { return GetPaintValueSupported(null); } ///Gets a value indicating whether this editor supports painting a representation /// of an object's value. ////// /// public virtual bool GetPaintValueSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the specified context supports painting a representation /// of an object's value. ////// /// public virtual UITypeEditorEditStyle GetEditStyle(ITypeDescriptorContext context) { return UITypeEditorEditStyle.None; } ////// Gets the editing style of the Edit method. /// ////// /// public void PaintValue(object value, Graphics canvas, Rectangle rectangle) { PaintValue(new PaintValueEventArgs(null, value, canvas, rectangle)); } ///Paints a representative value of the specified object to the /// specified canvas. ////// /// [SuppressMessage("Microsoft.Security", "CA2109:ReviewVisibleEventHandlers")] public virtual void PaintValue(PaintValueEventArgs e) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Paints a representative value of the specified object to the /// provided canvas. ///
Link Menu
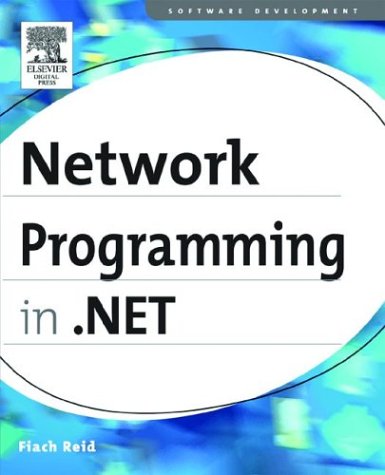
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SimpleTextLine.cs
- TextTreeNode.cs
- ModelPropertyImpl.cs
- dataprotectionpermission.cs
- OrderByBuilder.cs
- QilStrConcatenator.cs
- RegistryPermission.cs
- UpdateException.cs
- TcpServerChannel.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- ProjectionCamera.cs
- LassoSelectionBehavior.cs
- OrCondition.cs
- DataServiceProviderMethods.cs
- AnimationClock.cs
- Matrix3D.cs
- __Filters.cs
- Point.cs
- WebPartsPersonalizationAuthorization.cs
- MergeFilterQuery.cs
- ChildChangedEventArgs.cs
- ViewValidator.cs
- HttpCapabilitiesSectionHandler.cs
- PageRequestManager.cs
- ButtonField.cs
- RSAOAEPKeyExchangeFormatter.cs
- ReflectPropertyDescriptor.cs
- XmlEncoding.cs
- AsnEncodedData.cs
- TdsRecordBufferSetter.cs
- DataTable.cs
- BitmapFrameEncode.cs
- FixedStringLookup.cs
- DocumentViewerBase.cs
- SpellerStatusTable.cs
- Html32TextWriter.cs
- ProfileSection.cs
- SqlDataSourceStatusEventArgs.cs
- RuntimeConfig.cs
- TimeSpan.cs
- MenuAutoFormat.cs
- ToolStripItemClickedEventArgs.cs
- _SpnDictionary.cs
- OdbcParameter.cs
- PathFigureCollection.cs
- ObjectViewQueryResultData.cs
- AppSecurityManager.cs
- DBDataPermission.cs
- ContextMenu.cs
- ObjectPersistData.cs
- DispatchWrapper.cs
- MarkupWriter.cs
- ControlDesignerState.cs
- StringArrayConverter.cs
- EntityKey.cs
- WebBrowserNavigatingEventHandler.cs
- Quack.cs
- BrowserDefinition.cs
- MetadataItem_Static.cs
- PackageProperties.cs
- PassportIdentity.cs
- RectangleHotSpot.cs
- SerializableAttribute.cs
- InputLanguageEventArgs.cs
- HttpInputStream.cs
- OutputCacheSettings.cs
- DataBindingValueUIHandler.cs
- QilFunction.cs
- EntityClientCacheEntry.cs
- AdornerPresentationContext.cs
- SqlConnectionHelper.cs
- PropertyStore.cs
- CoordinationService.cs
- RegexEditorDialog.cs
- FontStyles.cs
- SoapMessage.cs
- baseaxisquery.cs
- ConfigurationErrorsException.cs
- CreateUserWizardAutoFormat.cs
- IsolatedStorage.cs
- SecureConversationDriver.cs
- DynamicFilter.cs
- AbsoluteQuery.cs
- SignedXmlDebugLog.cs
- ProcessManager.cs
- CompModSwitches.cs
- MappingItemCollection.cs
- UIElement3DAutomationPeer.cs
- SequenceFullException.cs
- CalendarTable.cs
- SqlConnectionStringBuilder.cs
- DesignTable.cs
- EmptyControlCollection.cs
- JavaScriptString.cs
- BasicAsyncResult.cs
- ColorConvertedBitmapExtension.cs
- Odbc32.cs
- DataPagerFieldCommandEventArgs.cs
- securitymgrsite.cs
- PointAnimationBase.cs