Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Net / System / Net / Mail / DelegatedStream.cs / 1 / DelegatedStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Net.Sockets; using System.IO; internal class DelegatedStream : Stream { Stream stream; NetworkStream netStream; protected DelegatedStream() { } protected DelegatedStream(Stream stream) { if (stream == null) throw new ArgumentNullException("stream"); this.stream = stream; netStream = stream as NetworkStream; } protected Stream BaseStream { get { return this.stream; } } public override bool CanRead { get { return this.stream.CanRead; } } public override bool CanSeek { get { return this.stream.CanSeek; } } public override bool CanWrite { get { return this.stream.CanWrite; } } public override long Length { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Length; } } public override long Position { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Position; } set { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.Position = value; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginRead (buffer, offset, count, callback, state); } else{ result = this.stream.BeginRead (buffer, offset, count, callback, state); } return result; } public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginWrite(buffer, offset, count, callback, state); } else{ result = this.stream.BeginWrite (buffer, offset, count, callback, state); } return result; } //This calls close on the inner stream //however, the stream may not be actually closed, but simpy flushed public override void Close() { this.stream.Close(); } public override int EndRead(IAsyncResult asyncResult) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.EndRead (asyncResult); return read; } public override void EndWrite(IAsyncResult asyncResult) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.EndWrite (asyncResult); } public override void Flush() { this.stream.Flush(); } public override int Read(byte[] buffer, int offset, int count) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.Read(buffer, offset, count); return read; } public override long Seek(long offset, SeekOrigin origin) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); long position = this.stream.Seek(offset, origin); return position; } public override void SetLength(long value) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.SetLength(value); } public override void Write(byte[] buffer, int offset, int count) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.Write(buffer, offset, count); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.Net.Sockets; using System.IO; internal class DelegatedStream : Stream { Stream stream; NetworkStream netStream; protected DelegatedStream() { } protected DelegatedStream(Stream stream) { if (stream == null) throw new ArgumentNullException("stream"); this.stream = stream; netStream = stream as NetworkStream; } protected Stream BaseStream { get { return this.stream; } } public override bool CanRead { get { return this.stream.CanRead; } } public override bool CanSeek { get { return this.stream.CanSeek; } } public override bool CanWrite { get { return this.stream.CanWrite; } } public override long Length { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Length; } } public override long Position { get { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); return this.stream.Position; } set { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.Position = value; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginRead (buffer, offset, count, callback, state); } else{ result = this.stream.BeginRead (buffer, offset, count, callback, state); } return result; } public override IAsyncResult BeginWrite(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); IAsyncResult result = null; if(netStream != null){ result = this.netStream.UnsafeBeginWrite(buffer, offset, count, callback, state); } else{ result = this.stream.BeginWrite (buffer, offset, count, callback, state); } return result; } //This calls close on the inner stream //however, the stream may not be actually closed, but simpy flushed public override void Close() { this.stream.Close(); } public override int EndRead(IAsyncResult asyncResult) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.EndRead (asyncResult); return read; } public override void EndWrite(IAsyncResult asyncResult) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.EndWrite (asyncResult); } public override void Flush() { this.stream.Flush(); } public override int Read(byte[] buffer, int offset, int count) { if (!CanRead) throw new NotSupportedException(SR.GetString(SR.ReadNotSupported)); int read = this.stream.Read(buffer, offset, count); return read; } public override long Seek(long offset, SeekOrigin origin) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); long position = this.stream.Seek(offset, origin); return position; } public override void SetLength(long value) { if (!CanSeek) throw new NotSupportedException(SR.GetString(SR.SeekNotSupported)); this.stream.SetLength(value); } public override void Write(byte[] buffer, int offset, int count) { if (!CanWrite) throw new NotSupportedException(SR.GetString(SR.WriteNotSupported)); this.stream.Write(buffer, offset, count); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
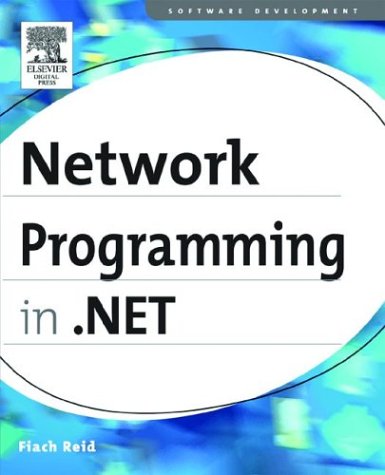
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedDocument.cs
- CharacterShapingProperties.cs
- QueueProcessor.cs
- SafeSecurityHandles.cs
- EventManager.cs
- MenuAutomationPeer.cs
- TypeUsage.cs
- FocusWithinProperty.cs
- BigInt.cs
- ReliableSessionBindingElement.cs
- UnaryQueryOperator.cs
- XmlSchemaProviderAttribute.cs
- VisualStyleTypesAndProperties.cs
- LayoutUtils.cs
- XmlReflectionImporter.cs
- ByteStreamGeometryContext.cs
- Msmq4SubqueuePoisonHandler.cs
- ScriptServiceAttribute.cs
- XmlSchemaNotation.cs
- SmtpMail.cs
- XmlExceptionHelper.cs
- DBNull.cs
- SqlMetaData.cs
- DefaultSerializationProviderAttribute.cs
- Vector3DValueSerializer.cs
- LinkAreaEditor.cs
- ComponentResourceKeyConverter.cs
- XomlCompilerResults.cs
- XPathException.cs
- Condition.cs
- MouseDevice.cs
- IntranetCredentialPolicy.cs
- SimpleWebHandlerParser.cs
- EditorPartChrome.cs
- DataBinding.cs
- AnnotationAuthorChangedEventArgs.cs
- StatusBarDrawItemEvent.cs
- OleDbInfoMessageEvent.cs
- SerializationTrace.cs
- DataGridViewCellCancelEventArgs.cs
- StrokeNodeEnumerator.cs
- Single.cs
- NumberFunctions.cs
- httpapplicationstate.cs
- TimeSpanSecondsConverter.cs
- XamlSerializer.cs
- BamlBinaryWriter.cs
- EventLogRecord.cs
- IApplicationTrustManager.cs
- PasswordTextContainer.cs
- OpenFileDialog.cs
- SessionStateUtil.cs
- OrderedHashRepartitionStream.cs
- DispatcherExceptionEventArgs.cs
- GradientStop.cs
- DocumentCollection.cs
- SchemaCollectionCompiler.cs
- PreviewPageInfo.cs
- AnnotationStore.cs
- OutputCacheSection.cs
- StaticExtension.cs
- MsmqIntegrationAppDomainProtocolHandler.cs
- FixedTextPointer.cs
- DataControlCommands.cs
- VideoDrawing.cs
- TitleStyle.cs
- InstancePersistenceCommandException.cs
- CqlWriter.cs
- Perspective.cs
- RootNamespaceAttribute.cs
- EmbeddedMailObject.cs
- CommandEventArgs.cs
- XmlWhitespace.cs
- XmlSerializableServices.cs
- CharEntityEncoderFallback.cs
- AttributeQuery.cs
- QueryResult.cs
- AttributeProviderAttribute.cs
- SingleTagSectionHandler.cs
- AssemblyBuilder.cs
- HttpDictionary.cs
- FilterException.cs
- WebBrowserProgressChangedEventHandler.cs
- AnnotationHelper.cs
- SerializationBinder.cs
- CompositeScriptReference.cs
- streamingZipPartStream.cs
- _NestedSingleAsyncResult.cs
- TrustLevelCollection.cs
- SelectionProcessor.cs
- KeyFrames.cs
- CodeDomLocalizationProvider.cs
- DefaultTextStoreTextComposition.cs
- DbExpressionVisitor.cs
- PeerCollaboration.cs
- GridViewCellAutomationPeer.cs
- PowerModeChangedEventArgs.cs
- InsufficientMemoryException.cs
- TreeNode.cs
- _LoggingObject.cs