Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / HierarchicalDataSourceDesigner.cs / 1 / HierarchicalDataSourceDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System.ComponentModel; using System.Collections; using System.ComponentModel.Design; using System.Design; using System.Drawing; ///[System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public class HierarchicalDataSourceDesigner : ControlDesigner, IHierarchicalDataSourceDesigner { private event EventHandler _dataSourceChangedEvent; private event EventHandler _schemaRefreshedEvent; private int _suppressEventsCount; private bool _raiseDataSourceChangedEvent; private bool _raiseSchemaRefreshedEvent; /// public override DesignerActionListCollection ActionLists { get { DesignerActionListCollection actionLists = new DesignerActionListCollection(); actionLists.AddRange(base.ActionLists); actionLists.Add(new HierarchicalDataSourceDesignerActionList(this)); return actionLists; } } /// /// /// Indicates whether the Configure() method can be called. /// public virtual bool CanConfigure { get { return false; } } ////// /// Indicates whether the RefreshSchema() method can be called. /// public virtual bool CanRefreshSchema { get { return false; } } ////// Raised when the properties of a DataControl have changed. This allows /// a data-bound control designer to take actions to refresh its /// control in the designer. /// public event EventHandler DataSourceChanged { add { _dataSourceChangedEvent += value; } remove { _dataSourceChangedEvent -= value; } } ////// Raised when the schema of the DataSource has changed. This notifies /// a data-bound control designer that the available schema fields have /// changed. /// public event EventHandler SchemaRefreshed { add { _schemaRefreshedEvent += value; } remove { _schemaRefreshedEvent -= value; } } protected bool SuppressingDataSourceEvents { get { return (_suppressEventsCount > 0); } } ////// /// Launches the data source's configuration wizard. /// This method should only be called if the CanConfigure property is true. /// public virtual void Configure() { throw new NotSupportedException(); } ////// /// Gets the design-time HTML. /// public override string GetDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(); } public virtual DesignerHierarchicalDataSourceView GetView(string viewPath) { return null; } ////// Raises the DataSourceChanged ecent. /// protected virtual void OnDataSourceChanged(EventArgs e) { if (SuppressingDataSourceEvents) { _raiseDataSourceChangedEvent = true; return; } if (_dataSourceChangedEvent != null) { _dataSourceChangedEvent(this, e); } _raiseDataSourceChangedEvent = false; } ////// Raises the SchemaRefreshed event. /// protected virtual void OnSchemaRefreshed(EventArgs e) { if (SuppressingDataSourceEvents) { _raiseSchemaRefreshedEvent = true; return; } if (_schemaRefreshedEvent != null) { _schemaRefreshedEvent(this, e); } _raiseSchemaRefreshedEvent = false; } public virtual void RefreshSchema(bool preferSilent) { throw new NotSupportedException(); } public virtual void ResumeDataSourceEvents() { if (_suppressEventsCount == 0) { throw new InvalidOperationException(SR.GetString(SR.DataSource_CannotResumeEvents)); } _suppressEventsCount--; if (_suppressEventsCount == 0) { // If this is the last call to resume, we raise the events if necessary if (_raiseDataSourceChangedEvent) { OnDataSourceChanged(EventArgs.Empty); } if (_raiseSchemaRefreshedEvent) { OnSchemaRefreshed(EventArgs.Empty); } } } public virtual void SuppressDataSourceEvents() { _suppressEventsCount++; } private class HierarchicalDataSourceDesignerActionList : DesignerActionList { private HierarchicalDataSourceDesigner _parent; public HierarchicalDataSourceDesignerActionList(HierarchicalDataSourceDesigner parent) : base(parent.Component) { _parent = parent; } public override bool AutoShow { get { return true; } set { } } public void Configure() { _parent.Configure(); } public void RefreshSchema() { _parent.RefreshSchema(false); } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection items = new DesignerActionItemCollection(); if (_parent.CanConfigure) { DesignerActionMethodItem methodItem = new DesignerActionMethodItem(this, "Configure", SR.GetString(SR.DataSourceDesigner_ConfigureDataSourceVerb), SR.GetString(SR.DataSourceDesigner_DataActionGroup), SR.GetString(SR.DataSourceDesigner_ConfigureDataSourceVerbDesc), true); methodItem.AllowAssociate = true; items.Add(methodItem); } if (_parent.CanRefreshSchema) { DesignerActionMethodItem methodItem = new DesignerActionMethodItem(this, "RefreshSchema", SR.GetString(SR.DataSourceDesigner_RefreshSchemaVerb), SR.GetString(SR.DataSourceDesigner_DataActionGroup), SR.GetString(SR.DataSourceDesigner_RefreshSchemaVerbDesc), false); methodItem.AllowAssociate = true; items.Add(methodItem); } return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
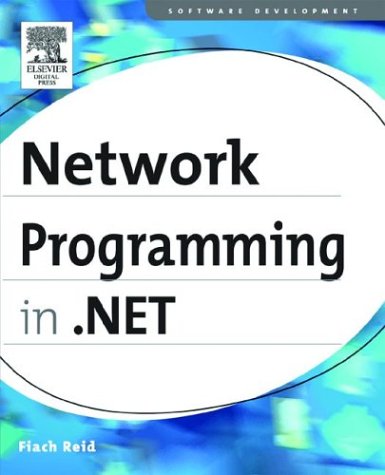
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreePropertyUndoUnit.cs
- WindowsFont.cs
- SchemaImporter.cs
- ConnectionInterfaceCollection.cs
- BitHelper.cs
- TextEditorContextMenu.cs
- Ipv6Element.cs
- CellLabel.cs
- EdmType.cs
- TypeUsage.cs
- UserValidatedEventArgs.cs
- MyContact.cs
- HttpRequest.cs
- ReachPageContentSerializerAsync.cs
- ConnectionManagementSection.cs
- SqlProviderServices.cs
- X509Certificate.cs
- CompilerErrorCollection.cs
- ObjectReferenceStack.cs
- MobileControlsSection.cs
- WsdlWriter.cs
- RangeValidator.cs
- ToolboxItemFilterAttribute.cs
- ToggleButtonAutomationPeer.cs
- Timer.cs
- ImpersonateTokenRef.cs
- SourceSwitch.cs
- DiagnosticTrace.cs
- WaitForChangedResult.cs
- CharacterString.cs
- TemplatePropertyEntry.cs
- MemberAccessException.cs
- XamlFxTrace.cs
- ReferenceEqualityComparer.cs
- Terminate.cs
- CodeTypeDelegate.cs
- ObjectAnimationBase.cs
- TextProperties.cs
- SamlEvidence.cs
- InvokeBinder.cs
- ExtendedPropertyDescriptor.cs
- DetailsViewInsertEventArgs.cs
- RelationalExpressions.cs
- Queue.cs
- ControlDesigner.cs
- DirectoryInfo.cs
- SamlConstants.cs
- OracleException.cs
- VScrollBar.cs
- RtfControls.cs
- ModelFunction.cs
- BuildManager.cs
- ComponentEvent.cs
- ColorAnimation.cs
- OrderingQueryOperator.cs
- AsyncResult.cs
- ConfigXmlText.cs
- LineProperties.cs
- NameTable.cs
- FormsAuthentication.cs
- CompilerGeneratedAttribute.cs
- EventLogInformation.cs
- XmlFormatExtensionAttribute.cs
- CollectionConverter.cs
- XPathScanner.cs
- ComboBoxItem.cs
- GlobalProxySelection.cs
- StandardToolWindows.cs
- ProcessProtocolHandler.cs
- SqlNodeTypeOperators.cs
- DeviceSpecificDialogCachedState.cs
- URLBuilder.cs
- SoapFormatter.cs
- IODescriptionAttribute.cs
- FakeModelItemImpl.cs
- XmlSchemaNotation.cs
- Signature.cs
- BaseAddressElement.cs
- UserPreferenceChangedEventArgs.cs
- RepeaterCommandEventArgs.cs
- BooleanFunctions.cs
- ErrorHandler.cs
- InputScopeNameConverter.cs
- ContainerControl.cs
- DbMetaDataFactory.cs
- QuerySelectOp.cs
- ClientBuildManager.cs
- MinimizableAttributeTypeConverter.cs
- EventLogRecord.cs
- StateRuntime.cs
- BrowserTree.cs
- SrgsRulesCollection.cs
- EditorOptionAttribute.cs
- SecurityHelper.cs
- ClientOptions.cs
- DataGridSortCommandEventArgs.cs
- XamlWrappingReader.cs
- ConsoleKeyInfo.cs
- NetCodeGroup.cs
- FormViewInsertEventArgs.cs