Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Security / PermissionSetTriple.cs / 5 / PermissionSetTriple.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: PermissionSetTriple ** ** Purpose: Container class for holding an AppDomain's Grantset and Refused sets. ** Also used for CompressedStacks which brings in the third PermissionSet. ** Hence, the name PermissionSetTriple. ** =============================================================================*/ namespace System.Security { using IEnumerator = System.Collections.IEnumerator; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; [Serializable()] sealed internal class PermissionSetTriple { unsafe static private RuntimeMethodHandle s_emptyRMH = new RuntimeMethodHandle(null); static private PermissionToken s_zoneToken; static private PermissionToken s_urlToken; internal PermissionSet AssertSet; internal PermissionSet GrantSet; internal PermissionSet RefusedSet; internal PermissionSetTriple() { Reset(); } internal PermissionSetTriple(PermissionSetTriple triple) { this.AssertSet = triple.AssertSet; this.GrantSet = triple.GrantSet; this.RefusedSet = triple.RefusedSet; } internal void Reset() { AssertSet = null; GrantSet = null; RefusedSet = null; } internal bool IsEmpty() { return (AssertSet == null && GrantSet == null && RefusedSet == null); } private PermissionToken ZoneToken { get { if (s_zoneToken == null) s_zoneToken = PermissionToken.GetToken(typeof(ZoneIdentityPermission)); return s_zoneToken; } } private PermissionToken UrlToken { get { if (s_urlToken == null) s_urlToken = PermissionToken.GetToken(typeof(UrlIdentityPermission)); return s_urlToken; } } internal bool Update(PermissionSetTriple psTriple, out PermissionSetTriple retTriple) { retTriple = null; // Special case: unrestricted assert. Note: dcs.Assert.IsUnrestricted => dcs.Grant.IsUnrestricted if (psTriple.AssertSet != null && psTriple.AssertSet.IsUnrestricted()) { return true; // stop construction } retTriple = UpdateAssert(psTriple.AssertSet); UpdateGrant(psTriple.GrantSet); UpdateRefused(psTriple.RefusedSet); return false; } internal PermissionSetTriple UpdateAssert(PermissionSet in_a) { PermissionSetTriple retTriple = null; if (in_a != null) { BCLDebug.Assert((!in_a.IsUnrestricted()), "Cannot be unrestricted here"); // if we're already asserting in_a, nothing to do if (in_a.IsSubsetOf(AssertSet)) return null; PermissionSet retPs; if (GrantSet != null) retPs = in_a.Intersect(GrantSet); // Restrict the assert to what we've already been granted else { GrantSet = new PermissionSet(true); retPs = in_a.Copy(); // Currently unrestricted Grant: assert the whole assert set } bool bFailedToCompress; // removes anything that is already in the refused set from the assert set retPs = PermissionSet.RemoveRefusedPermissionSet(retPs, RefusedSet, out bFailedToCompress); if (!bFailedToCompress) bFailedToCompress = PermissionSet.IsIntersectingAssertedPermissions(retPs, AssertSet); if (bFailedToCompress) { retTriple = new PermissionSetTriple(this); this.Reset(); this.GrantSet = retTriple.GrantSet.Copy(); } if (AssertSet == null) AssertSet = retPs; else AssertSet.InplaceUnion(retPs); } return retTriple; } internal void UpdateGrant(PermissionSet in_g, out ZoneIdentityPermission z,out UrlIdentityPermission u) { z = null; u = null; if (in_g != null) { if (GrantSet == null) GrantSet = in_g.Copy(); else GrantSet.InplaceIntersect(in_g); z = (ZoneIdentityPermission)in_g.GetPermission(ZoneToken); u = (UrlIdentityPermission)in_g.GetPermission(UrlToken); } } internal void UpdateGrant(PermissionSet in_g) { if (in_g != null) { if (GrantSet == null) GrantSet = in_g.Copy(); else GrantSet.InplaceIntersect(in_g); } } internal void UpdateRefused(PermissionSet in_r) { if (in_r != null) { if (RefusedSet == null) RefusedSet = in_r.Copy(); else RefusedSet.InplaceUnion(in_r); } } static bool CheckAssert(PermissionSet pSet, CodeAccessPermission demand, PermissionToken permToken) { if (pSet != null) { pSet.CheckDecoded(demand, permToken); CodeAccessPermission perm = (CodeAccessPermission)pSet.GetPermission(demand); // If the assert set does contain the demanded permission, halt the stackwalk try { if ((pSet.IsUnrestricted() && demand.CanUnrestrictedOverride()) || demand.CheckAssert(perm)) { return SecurityRuntime.StackHalt; } } catch (ArgumentException) { } } return SecurityRuntime.StackContinue; } static bool CheckAssert(PermissionSet assertPset, PermissionSet demandSet, out PermissionSet newDemandSet) { newDemandSet = null; if (assertPset!= null) { assertPset.CheckDecoded(demandSet); // If this frame asserts a superset of the demand set we're done if (demandSet.CheckAssertion(assertPset)) return SecurityRuntime.StackHalt; PermissionSet.RemoveAssertedPermissionSet(demandSet, assertPset, out newDemandSet); } return SecurityRuntime.StackContinue; } internal bool CheckDemand(CodeAccessPermission demand, PermissionToken permToken, RuntimeMethodHandle rmh) { if (CheckAssert(AssertSet, demand, permToken) == SecurityRuntime.StackHalt) return SecurityRuntime.StackHalt; CodeAccessSecurityEngine.CheckHelper(GrantSet, RefusedSet, demand, permToken, rmh, null, SecurityAction.Demand, true); return SecurityRuntime.StackContinue; } internal bool CheckSetDemand(PermissionSet demandSet , out PermissionSet alteredDemandset, RuntimeMethodHandle rmh) { alteredDemandset = null; if (CheckAssert(AssertSet, demandSet, out alteredDemandset) == SecurityRuntime.StackHalt) return SecurityRuntime.StackHalt; if (alteredDemandset != null) demandSet = alteredDemandset; // note that this does not modify demandSet external to this function. CodeAccessSecurityEngine.CheckSetHelper(GrantSet, RefusedSet, demandSet, rmh, null, SecurityAction.Demand, true); return SecurityRuntime.StackContinue; } internal bool CheckDemandNoThrow(CodeAccessPermission demand, PermissionToken permToken) { BCLDebug.Assert(AssertSet == null, "AssertSet not null"); return CodeAccessSecurityEngine.CheckHelper(GrantSet, RefusedSet, demand, permToken, s_emptyRMH, null, SecurityAction.Demand, false); } internal bool CheckSetDemandNoThrow(PermissionSet demandSet) { BCLDebug.Assert(AssertSet == null, "AssertSet not null"); return CodeAccessSecurityEngine.CheckSetHelper(GrantSet, RefusedSet, demandSet, s_emptyRMH, null, SecurityAction.Demand, false); } ////// Check to see if the triple satisfies a demand for the permission represented by the flag. /// ////// If the triple asserts for one of the bits in the flags, it is zeroed out. /// /// set of flags to check (See PermissionType) internal bool CheckFlags(ref int flags) { if (AssertSet != null) { // remove any permissions which were asserted for int assertFlags = SecurityManager.GetSpecialFlags(AssertSet, null); if ((flags & assertFlags) != 0) flags = flags & ~assertFlags; } return (SecurityManager.GetSpecialFlags(GrantSet, RefusedSet) & flags) == flags; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** Class: PermissionSetTriple ** ** Purpose: Container class for holding an AppDomain's Grantset and Refused sets. ** Also used for CompressedStacks which brings in the third PermissionSet. ** Hence, the name PermissionSetTriple. ** =============================================================================*/ namespace System.Security { using IEnumerator = System.Collections.IEnumerator; using System.Security; using System.Security.Permissions; using System.Runtime.InteropServices; [Serializable()] sealed internal class PermissionSetTriple { unsafe static private RuntimeMethodHandle s_emptyRMH = new RuntimeMethodHandle(null); static private PermissionToken s_zoneToken; static private PermissionToken s_urlToken; internal PermissionSet AssertSet; internal PermissionSet GrantSet; internal PermissionSet RefusedSet; internal PermissionSetTriple() { Reset(); } internal PermissionSetTriple(PermissionSetTriple triple) { this.AssertSet = triple.AssertSet; this.GrantSet = triple.GrantSet; this.RefusedSet = triple.RefusedSet; } internal void Reset() { AssertSet = null; GrantSet = null; RefusedSet = null; } internal bool IsEmpty() { return (AssertSet == null && GrantSet == null && RefusedSet == null); } private PermissionToken ZoneToken { get { if (s_zoneToken == null) s_zoneToken = PermissionToken.GetToken(typeof(ZoneIdentityPermission)); return s_zoneToken; } } private PermissionToken UrlToken { get { if (s_urlToken == null) s_urlToken = PermissionToken.GetToken(typeof(UrlIdentityPermission)); return s_urlToken; } } internal bool Update(PermissionSetTriple psTriple, out PermissionSetTriple retTriple) { retTriple = null; // Special case: unrestricted assert. Note: dcs.Assert.IsUnrestricted => dcs.Grant.IsUnrestricted if (psTriple.AssertSet != null && psTriple.AssertSet.IsUnrestricted()) { return true; // stop construction } retTriple = UpdateAssert(psTriple.AssertSet); UpdateGrant(psTriple.GrantSet); UpdateRefused(psTriple.RefusedSet); return false; } internal PermissionSetTriple UpdateAssert(PermissionSet in_a) { PermissionSetTriple retTriple = null; if (in_a != null) { BCLDebug.Assert((!in_a.IsUnrestricted()), "Cannot be unrestricted here"); // if we're already asserting in_a, nothing to do if (in_a.IsSubsetOf(AssertSet)) return null; PermissionSet retPs; if (GrantSet != null) retPs = in_a.Intersect(GrantSet); // Restrict the assert to what we've already been granted else { GrantSet = new PermissionSet(true); retPs = in_a.Copy(); // Currently unrestricted Grant: assert the whole assert set } bool bFailedToCompress; // removes anything that is already in the refused set from the assert set retPs = PermissionSet.RemoveRefusedPermissionSet(retPs, RefusedSet, out bFailedToCompress); if (!bFailedToCompress) bFailedToCompress = PermissionSet.IsIntersectingAssertedPermissions(retPs, AssertSet); if (bFailedToCompress) { retTriple = new PermissionSetTriple(this); this.Reset(); this.GrantSet = retTriple.GrantSet.Copy(); } if (AssertSet == null) AssertSet = retPs; else AssertSet.InplaceUnion(retPs); } return retTriple; } internal void UpdateGrant(PermissionSet in_g, out ZoneIdentityPermission z,out UrlIdentityPermission u) { z = null; u = null; if (in_g != null) { if (GrantSet == null) GrantSet = in_g.Copy(); else GrantSet.InplaceIntersect(in_g); z = (ZoneIdentityPermission)in_g.GetPermission(ZoneToken); u = (UrlIdentityPermission)in_g.GetPermission(UrlToken); } } internal void UpdateGrant(PermissionSet in_g) { if (in_g != null) { if (GrantSet == null) GrantSet = in_g.Copy(); else GrantSet.InplaceIntersect(in_g); } } internal void UpdateRefused(PermissionSet in_r) { if (in_r != null) { if (RefusedSet == null) RefusedSet = in_r.Copy(); else RefusedSet.InplaceUnion(in_r); } } static bool CheckAssert(PermissionSet pSet, CodeAccessPermission demand, PermissionToken permToken) { if (pSet != null) { pSet.CheckDecoded(demand, permToken); CodeAccessPermission perm = (CodeAccessPermission)pSet.GetPermission(demand); // If the assert set does contain the demanded permission, halt the stackwalk try { if ((pSet.IsUnrestricted() && demand.CanUnrestrictedOverride()) || demand.CheckAssert(perm)) { return SecurityRuntime.StackHalt; } } catch (ArgumentException) { } } return SecurityRuntime.StackContinue; } static bool CheckAssert(PermissionSet assertPset, PermissionSet demandSet, out PermissionSet newDemandSet) { newDemandSet = null; if (assertPset!= null) { assertPset.CheckDecoded(demandSet); // If this frame asserts a superset of the demand set we're done if (demandSet.CheckAssertion(assertPset)) return SecurityRuntime.StackHalt; PermissionSet.RemoveAssertedPermissionSet(demandSet, assertPset, out newDemandSet); } return SecurityRuntime.StackContinue; } internal bool CheckDemand(CodeAccessPermission demand, PermissionToken permToken, RuntimeMethodHandle rmh) { if (CheckAssert(AssertSet, demand, permToken) == SecurityRuntime.StackHalt) return SecurityRuntime.StackHalt; CodeAccessSecurityEngine.CheckHelper(GrantSet, RefusedSet, demand, permToken, rmh, null, SecurityAction.Demand, true); return SecurityRuntime.StackContinue; } internal bool CheckSetDemand(PermissionSet demandSet , out PermissionSet alteredDemandset, RuntimeMethodHandle rmh) { alteredDemandset = null; if (CheckAssert(AssertSet, demandSet, out alteredDemandset) == SecurityRuntime.StackHalt) return SecurityRuntime.StackHalt; if (alteredDemandset != null) demandSet = alteredDemandset; // note that this does not modify demandSet external to this function. CodeAccessSecurityEngine.CheckSetHelper(GrantSet, RefusedSet, demandSet, rmh, null, SecurityAction.Demand, true); return SecurityRuntime.StackContinue; } internal bool CheckDemandNoThrow(CodeAccessPermission demand, PermissionToken permToken) { BCLDebug.Assert(AssertSet == null, "AssertSet not null"); return CodeAccessSecurityEngine.CheckHelper(GrantSet, RefusedSet, demand, permToken, s_emptyRMH, null, SecurityAction.Demand, false); } internal bool CheckSetDemandNoThrow(PermissionSet demandSet) { BCLDebug.Assert(AssertSet == null, "AssertSet not null"); return CodeAccessSecurityEngine.CheckSetHelper(GrantSet, RefusedSet, demandSet, s_emptyRMH, null, SecurityAction.Demand, false); } ////// Check to see if the triple satisfies a demand for the permission represented by the flag. /// ////// If the triple asserts for one of the bits in the flags, it is zeroed out. /// /// set of flags to check (See PermissionType) internal bool CheckFlags(ref int flags) { if (AssertSet != null) { // remove any permissions which were asserted for int assertFlags = SecurityManager.GetSpecialFlags(AssertSet, null); if ((flags & assertFlags) != 0) flags = flags & ~assertFlags; } return (SecurityManager.GetSpecialFlags(GrantSet, RefusedSet) & flags) == flags; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
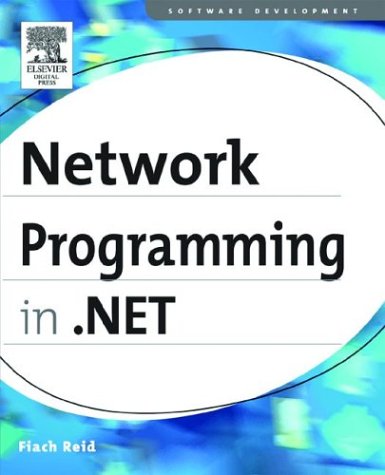
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClipboardProcessor.cs
- ExportOptions.cs
- ProviderCommandInfoUtils.cs
- SamlAttributeStatement.cs
- ProcessHostFactoryHelper.cs
- ToolStripStatusLabel.cs
- HandlerBase.cs
- DBBindings.cs
- NativeRightsManagementAPIsStructures.cs
- RemoteDebugger.cs
- DeferredSelectedIndexReference.cs
- DefaultTextStoreTextComposition.cs
- QilPatternVisitor.cs
- TextElementEditingBehaviorAttribute.cs
- FamilyCollection.cs
- WebUtil.cs
- HttpListenerRequest.cs
- ClientTargetCollection.cs
- SocketElement.cs
- PropertyEntry.cs
- Geometry.cs
- ItemsPresenter.cs
- PrintDocument.cs
- TimeSpanValidator.cs
- SiteMapDataSource.cs
- TextEffectResolver.cs
- ComplexBindingPropertiesAttribute.cs
- GAC.cs
- CodeTypeMemberCollection.cs
- DrawingContextDrawingContextWalker.cs
- FileLevelControlBuilderAttribute.cs
- BitmapMetadataEnumerator.cs
- WebConfigurationHost.cs
- HttpRequestBase.cs
- ResourcePart.cs
- AttributeData.cs
- RegexGroup.cs
- PrivilegedConfigurationManager.cs
- EntityDataSourceSelectingEventArgs.cs
- MissingMethodException.cs
- HttpRequestWrapper.cs
- AddInPipelineAttributes.cs
- MSAAWinEventWrap.cs
- SQLInt32.cs
- RectAnimationUsingKeyFrames.cs
- EncoderBestFitFallback.cs
- TableLayoutCellPaintEventArgs.cs
- DuplexChannelBinder.cs
- ImportedNamespaceContextItem.cs
- XmlWriter.cs
- SoapFault.cs
- StringFormat.cs
- RequestCachePolicy.cs
- PlanCompilerUtil.cs
- GlobalProxySelection.cs
- SymbolType.cs
- PropertyDescriptorGridEntry.cs
- PageCatalogPart.cs
- CompilationRelaxations.cs
- DeclarativeCatalogPart.cs
- PrintPreviewGraphics.cs
- XmlReader.cs
- ClientApiGenerator.cs
- BaseTemplateBuildProvider.cs
- ReadOnlyNameValueCollection.cs
- NavigationPropertyEmitter.cs
- StylusPoint.cs
- EpmCustomContentSerializer.cs
- DataObjectSettingDataEventArgs.cs
- Frame.cs
- ZipPackage.cs
- BeginEvent.cs
- LocalizationParserHooks.cs
- HttpApplication.cs
- HtmlWindowCollection.cs
- Lasso.cs
- WindowsListViewItemStartMenu.cs
- XPathSingletonIterator.cs
- ToolStripItemCollection.cs
- DataGridViewCellMouseEventArgs.cs
- UInt32.cs
- TemplateComponentConnector.cs
- Pen.cs
- WebZone.cs
- CommonRemoteMemoryBlock.cs
- SpeechEvent.cs
- DataGridRelationshipRow.cs
- ComboBox.cs
- GeneralTransformGroup.cs
- XmlDomTextWriter.cs
- SystemException.cs
- WebPartConnectionsDisconnectVerb.cs
- CallSiteOps.cs
- ComEventsHelper.cs
- mediaeventshelper.cs
- ProfileEventArgs.cs
- ColumnReorderedEventArgs.cs
- RuntimeIdentifierPropertyAttribute.cs
- BindingCompleteEventArgs.cs
- QueryPageSettingsEventArgs.cs