Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Fonts.cs / 1 / Fonts.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: The Fonts class provides font enumeration APIs. // See spec at [....]/text/DesignDocsAndSpecs/Font%20Enumeration%20API.htm // // // History: // 03/02/2004 : [....] - Created // //--------------------------------------------------------------------------- using System; using System.Text; using System.IO; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Windows; using MS.Internal; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.PresentationCore; using MS.Internal.Shaping; using System.Security; namespace System.Windows.Media { ////// The FontEmbeddingManager class provides font enumeration APIs. /// public static class Fonts { //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Enumerates font families from an arbitrary location. /// /// An absolute URI of a folder containing fonts or a font file. ///Collection of FontFamily objects from the specified folder or file. ////// The specified location must be an absolute file URI or path, and the caller must have /// FileIOPermission(FileIOPermissionAccess.Read) for the location. Each resulting FontFamily /// object includes the specified location in its friendly name and has no base URI. /// public static ICollectionGetFontFamilies(string location) { if (location == null) throw new ArgumentNullException("location"); return GetFontFamilies(null, location); } /// /// Enumerates font families in the same folder as the specified base URI. /// /// An absolute URI of a folder containing fonts or a resource in that folder. ///Collection of FontFamily objects from the specified folder. ////// The caller must have FileIOPermission(FileIOPermissionAccess.Read) for the folder /// specified by baseUri. Each resulting FontFamily object has the specified Uri as its /// BaseUri property has a friendly name of the form "./#Family Name". /// public static ICollectionGetFontFamilies(Uri baseUri) { if (baseUri == null) throw new ArgumentNullException("baseUri"); return GetFontFamilies(baseUri, null); } /// /// Enumerates font families in the font location specified by a base URI and/or a location string. /// /// Base URI used to determine the font location if the location parameter is not specified /// or is relative, and the value of the BaseUri property of each FontFamily in the resulting collection. This /// parameter can be null if the location parameter specifies an absolute location. /// Optional relative or absolute URI reference. The location is used (with baseUri) to /// determine the font folder and is exposed as part of the Source property of each FontFamily in the resulting /// collection. If location is null or empty then "./" is implied, meaning same folder as the base URI. ///Collection of FontFamily objects from the specified font location. ////// The caller must have FileIOPermission(FileIOPermissionAccess.Read) for the specified font folder. /// Each resulting FontFamily object has the specified base Uri as its BaseUri property and includes the /// specified location as part of the friendly name specified by the Source property. /// public static ICollectionGetFontFamilies(Uri baseUri, string location) { // Both Uri parameters are optional but neither can be relative. if (baseUri != null && !baseUri.IsAbsoluteUri) throw new ArgumentException(SR.Get(SRID.UriNotAbsolute), "baseUri"); // Determine the font location from the base URI and location string. Uri fontLocation; if (!string.IsNullOrEmpty(location) && Uri.TryCreate(location, UriKind.Absolute, out fontLocation)) { // absolute location; make sure we support absolute font family references for this scheme if (!Util.IsSupportedSchemeForAbsoluteFontFamilyUri(fontLocation)) throw new ArgumentException(SR.Get(SRID.InvalidAbsoluteUriInFontFamilyName), "location"); // make sure the absolute location is a valid URI reference rather than a Win32 path as // we don't support the latter in a font family reference location = fontLocation.GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); } else { // relative location; we need a base URI if (baseUri == null) throw new ArgumentNullException("baseUri", SR.Get(SRID.NullBaseUriParam, "baseUri", "location")); // the location part must include a path component, otherwise we'll look in windows fonts and ignore the base URI if (string.IsNullOrEmpty(location)) location = "./"; else if (Util.IsReferenceToWindowsFonts(location)) location = "./" + location; fontLocation = new Uri(baseUri, location); } // Create the font families. return CreateFamilyCollection( fontLocation, // fontLocation baseUri, // fontFamilyBaseUri location // fontFamilyLocationReference ); } /// /// Enumerates typefaces from an arbitrary location. /// /// An absolute URI of a folder containing fonts or a font file. ///Collection of Typeface objects from the specified folder or file. ////// The specified location must be an absolute file URI or path, and the caller must have /// FileIOPermission(FileIOPermissionAccess.Read) for the location. The FontFamily of each /// resulting Typeface object includes the specified location in its friendly name and has /// no base URI. /// public static ICollectionGetTypefaces(string location) { if (location == null) throw new ArgumentNullException("location"); return new TypefaceCollection(GetFontFamilies(null, location)); } /// /// Enumerates typefaces in the same folder as the specified base URI. /// /// An absolute URI of a folder containing fonts or a resource in that folder. ///Collection of Typeface objects from the specified folder. ////// The caller must have FileIOPermission(FileIOPermissionAccess.Read) for the folder /// specified by baseUri. The FontFamily of each resulting Typeface object has the specified /// Uri as its BaseUri property has a friendly name of the form "./#Family Name". /// public static ICollectionGetTypefaces(Uri baseUri) { if (baseUri == null) throw new ArgumentNullException("baseUri"); return new TypefaceCollection(GetFontFamilies(baseUri, null)); } /// /// Enumerates typefaces in the font location specified by a base URI and/or a location string. /// /// Base URI used to determine the font location if the location parameter is not specified /// or is relative, and the value of the BaseUri property of each FontFamily in the resulting collection. This /// parameter can be null if the location parameter specifies an absolute location. /// Optional relative or absolute URI reference. The location is used (with baseUri) to /// determine the font folder and is exposed as part of the Source property of each FontFamily in the resulting /// collection. If location is null or empty then "./" is implied, meaning same folder as the base URI. ///Collection of Typeface objects from the specified font location. ////// The caller must have FileIOPermission(FileIOPermissionAccess.Read) for the specified font folder. /// Each resulting FontFamily object has the specified base Uri as its BaseUri property and includes the /// specified location as part of the friendly name specified by the Source property. /// public static ICollectionGetTypefaces(Uri baseUri, string location) { return new TypefaceCollection(GetFontFamilies(baseUri, location)); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties /// /// Font families from the default system font location. /// ///Collection of FontFamily objects from the default system font location. public static ICollectionSystemFontFamilies { get { return _defaultFontCollection; } } /// /// Type faces from the default system font location. /// ///Collection of Typeface objects from the default system font location. public static ICollectionSystemTypefaces { get { return new TypefaceCollection(_defaultFontCollection); } } #endregion Public Properties //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods /// /// This method enumerates the font families in the specified font location and returns the resulting /// collection of FontFamily objects. /// /// Absolute URI of file or folder containing font data /// Optional base URI, exposed as the BaseUri property of each FontFamily. /// Optional location reference, exposed as part of the Source /// property of each FontFamily. ////// SecurityCritical - Calls the critical FamilyCollection constructor; fontLocation may be critical /// TreatAsSafe - FamilyCollection does security demands for the necessary permissions; fontLocation is /// not exposed by the resulting FontFamily objects /// [SecurityCritical,SecurityTreatAsSafe] private static ICollectionCreateFamilyCollection( Uri fontLocation, Uri fontFamilyBaseUri, string fontFamilyLocationReference ) { // Use reference comparison to determine the critical isWindowsFonts value. We want this // to be true ONLY if we're called internally to enumerate the default family collection. // See the SecurityNote for the FamilyCollection constructor. FamilyCollection familyCollection = new FamilyCollection( fontLocation, object.ReferenceEquals(fontLocation, Util.WindowsFontsUriObject) // isWindowsFonts ); CacheManager.Lookup(familyCollection); FontFamily[] fontFamilyList = new FontFamily[familyCollection.FamilyCount]; int i = 0; foreach (CachedFontFamily family in familyCollection.GetFontFamilies()) { string fontFamilyReference = Util.ConvertFamilyNameAndLocationToFontFamilyReference( family.OrdinalName, fontFamilyLocationReference ); string friendlyName = Util.ConvertFontFamilyReferenceToFriendlyName(fontFamilyReference); fontFamilyList[i++] = new FontFamily(fontFamilyBaseUri, friendlyName); } Debug.Assert(i == familyCollection.FamilyCount); return Array.AsReadOnly (fontFamilyList); } /// /// Creates a collection of font families in the Windows Fonts folder. /// ////// This method is used to initialized the static _defaultFontCollection field. By having this /// safe wrapper for CreateFamilyCollection we avoid having to create a static initializer and /// declare it critical. /// ////// Critical - This method accesses the critical Util.WindowsFontsUriObject /// TreatAsSafe - It specifies null as the BaseUri of the resulting FontFamily objects /// [SecurityCritical, SecurityTreatAsSafe] private static ICollectionCreateDefaultFamilyCollection() { return CreateFamilyCollection( Util.WindowsFontsUriObject, // fontLocation null, // fontFamilyBaseUri, null // fontFamilyLocationReference ); } #endregion Private Methods //----------------------------------------------------- // // Private Classes // //------------------------------------------------------ #region Private Classes private struct TypefaceCollection : ICollection { private IEnumerable _families; public TypefaceCollection(IEnumerable families) { _families = families; } #region ICollection Members public void Add(Typeface item) { throw new NotSupportedException(); } public void Clear() { throw new NotSupportedException(); } public bool Contains(Typeface item) { foreach (Typeface t in this) { if (t.Equals(item)) return true; } return false; } public void CopyTo(Typeface[] array, int arrayIndex) { if (array == null) { throw new ArgumentNullException("array"); } if (array.Rank != 1) { throw new ArgumentException(SR.Get(SRID.Collection_BadRank)); } // The extra "arrayIndex >= array.Length" check in because even if _collection.Count // is 0 the index is not allowed to be equal or greater than the length // (from the MSDN ICollection docs) if (arrayIndex < 0 || arrayIndex >= array.Length || (arrayIndex + Count) > array.Length) { throw new ArgumentOutOfRangeException("arrayIndex"); } foreach (Typeface t in this) { array[arrayIndex++] = t; } } public int Count { get { int count = 0; foreach (Typeface t in this) { ++count; } return count; } } public bool IsReadOnly { get { return true; } } public bool Remove(Typeface item) { throw new NotSupportedException(); } #endregion #region IEnumerable Members public IEnumerator GetEnumerator() { foreach (FontFamily family in _families) { foreach (Typeface typeface in family.GetTypefaces()) { yield return typeface; } } } #endregion #region IEnumerable Members System.Collections.IEnumerator System.Collections.IEnumerable.GetEnumerator() { return ((IEnumerable )this).GetEnumerator(); } #endregion } #endregion Private Classes //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private static readonly ICollection _defaultFontCollection = CreateDefaultFamilyCollection(); #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
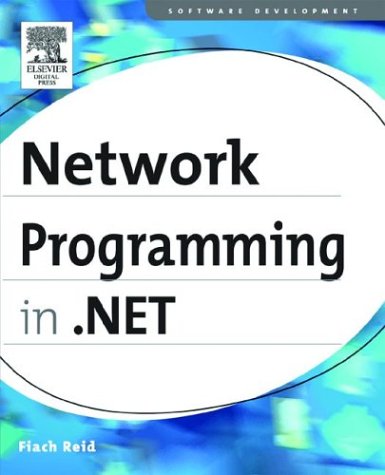
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Transform.cs
- _KerberosClient.cs
- Bitmap.cs
- UIHelper.cs
- TaskHelper.cs
- GeneralTransform.cs
- PropertyMapper.cs
- ClientBuildManager.cs
- LinqDataSource.cs
- PageSettings.cs
- ToolStripMenuItem.cs
- PathSegmentCollection.cs
- ByteConverter.cs
- XmlSerializerNamespaces.cs
- EnumUnknown.cs
- SourceLineInfo.cs
- SizeAnimationClockResource.cs
- JsonCollectionDataContract.cs
- Facet.cs
- HttpApplication.cs
- EntityCommandDefinition.cs
- ProgressBarRenderer.cs
- StreamedFramingRequestChannel.cs
- StyleXamlParser.cs
- IList.cs
- GridViewHeaderRowPresenter.cs
- Control.cs
- BevelBitmapEffect.cs
- IIS7WorkerRequest.cs
- ValidationResult.cs
- XPathDocumentNavigator.cs
- EUCJPEncoding.cs
- ObjectTokenCategory.cs
- AssemblyCache.cs
- Inflater.cs
- EastAsianLunisolarCalendar.cs
- TransportSecurityProtocolFactory.cs
- EditableLabelControl.cs
- ObjectViewQueryResultData.cs
- DataGridViewTopLeftHeaderCell.cs
- SqlNodeTypeOperators.cs
- bindurihelper.cs
- coordinatorscratchpad.cs
- Operator.cs
- XmlDataDocument.cs
- ExpressionParser.cs
- IgnoreFileBuildProvider.cs
- EnumerationRangeValidationUtil.cs
- BitmapPalettes.cs
- KeyConstraint.cs
- InvariantComparer.cs
- TdsParserStateObject.cs
- RectangleConverter.cs
- DocumentViewerAutomationPeer.cs
- WindowsScrollBarBits.cs
- DbDataSourceEnumerator.cs
- DelegatingTypeDescriptionProvider.cs
- ListItemDetailViewAttribute.cs
- MetadataUtilsSmi.cs
- LineInfo.cs
- EntityRecordInfo.cs
- AssemblyCollection.cs
- SiteMapNodeItemEventArgs.cs
- RetriableClipboard.cs
- TagPrefixCollection.cs
- Crc32Helper.cs
- DatatypeImplementation.cs
- DataGridViewColumnEventArgs.cs
- DoneReceivingAsyncResult.cs
- AsnEncodedData.cs
- RuntimeConfigurationRecord.cs
- PerfCounters.cs
- CharKeyFrameCollection.cs
- DataKey.cs
- ellipse.cs
- DataGridViewRowEventArgs.cs
- DoubleAnimationBase.cs
- TreeNodeBindingCollection.cs
- DeclaredTypeElementCollection.cs
- WindowsRebar.cs
- PromptEventArgs.cs
- SingleAnimationBase.cs
- PersonalizationProviderCollection.cs
- HighlightComponent.cs
- OracleInternalConnection.cs
- DiagnosticTraceSource.cs
- ContextMenuStrip.cs
- Parser.cs
- TdsParserStateObject.cs
- DependencyObjectType.cs
- WmlImageAdapter.cs
- WebPartConnectionCollection.cs
- DataGridViewSelectedRowCollection.cs
- OutputBuffer.cs
- OLEDB_Util.cs
- SecuritySessionClientSettings.cs
- QueryRewriter.cs
- DebugView.cs
- DataSourceComponent.cs
- LocalizationComments.cs