Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Serializer / QueueSurrogate.cs / 1305376 / QueueSurrogate.cs
namespace System.Workflow.ComponentModel.Serialization { using System; using System.Xml; using System.Runtime.Serialization; using System.Reflection; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Collections; using System.Collections.Generic; #region QueueSurrogate internal sealed class QueueSurrogate : ISerializationSurrogate { internal QueueSurrogate() { } void ISerializationSurrogate.GetObjectData(object obj, SerializationInfo info, StreamingContext context) { object[] items = ((Queue)obj).ToArray(); if (items.Length == 1) info.AddValue("item", items[0]); else info.AddValue("items", items); info.SetType(typeof(QRef)); } object ISerializationSurrogate.SetObjectData(object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { return null; } #region QRef [Serializable] private sealed class QRef : IObjectReference, IDeserializationCallback { [OptionalField] private IList items = null; [OptionalField] private object item = null; [NonSerialized] private Queue queue = null; Object IObjectReference.GetRealObject(StreamingContext context) { if (this.queue == null) { this.queue = new Queue(); } return this.queue; } void IDeserializationCallback.OnDeserialization(Object sender) { if (this.queue != null) { if (this.items != null) { for (int n = 0; n < this.items.Count; n++) this.queue.Enqueue(items[n]); } else { this.queue.Enqueue(this.item); } this.queue = null; } } } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Serialization { using System; using System.Xml; using System.Runtime.Serialization; using System.Reflection; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Collections; using System.Collections.Generic; #region QueueSurrogate internal sealed class QueueSurrogate : ISerializationSurrogate { internal QueueSurrogate() { } void ISerializationSurrogate.GetObjectData(object obj, SerializationInfo info, StreamingContext context) { object[] items = ((Queue)obj).ToArray(); if (items.Length == 1) info.AddValue("item", items[0]); else info.AddValue("items", items); info.SetType(typeof(QRef)); } object ISerializationSurrogate.SetObjectData(object obj, SerializationInfo info, StreamingContext context, ISurrogateSelector selector) { return null; } #region QRef [Serializable] private sealed class QRef : IObjectReference, IDeserializationCallback { [OptionalField] private IList items = null; [OptionalField] private object item = null; [NonSerialized] private Queue queue = null; Object IObjectReference.GetRealObject(StreamingContext context) { if (this.queue == null) { this.queue = new Queue(); } return this.queue; } void IDeserializationCallback.OnDeserialization(Object sender) { if (this.queue != null) { if (this.items != null) { for (int n = 0; n < this.items.Count; n++) this.queue.Enqueue(items[n]); } else { this.queue.Enqueue(this.item); } this.queue = null; } } } #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
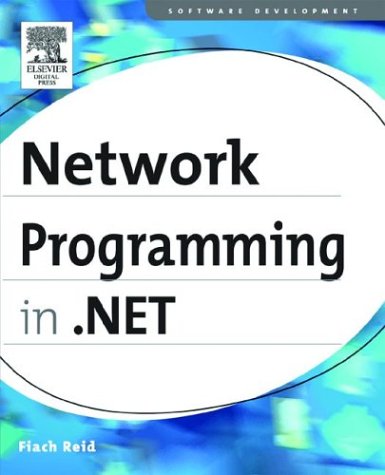
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbTransaction.cs
- MessageEventSubscriptionService.cs
- SoapException.cs
- KnownColorTable.cs
- SelectionRange.cs
- HttpCookieCollection.cs
- MetadataSerializer.cs
- BitmapMetadataEnumerator.cs
- XPathSingletonIterator.cs
- DelegateBodyWriter.cs
- DeviceFilterDictionary.cs
- ItemCollection.cs
- DefaultExpressionVisitor.cs
- OleDbStruct.cs
- SerializationAttributes.cs
- DataListComponentEditor.cs
- NativeDirectoryServicesQueryAPIs.cs
- PasswordRecovery.cs
- ObjectDisposedException.cs
- SystemColorTracker.cs
- DictionaryBase.cs
- UndoManager.cs
- ToggleButtonAutomationPeer.cs
- ComplusEndpointConfigContainer.cs
- ExpressionBuilder.cs
- LinkButton.cs
- PreloadHost.cs
- DateBoldEvent.cs
- SecUtil.cs
- IsolatedStorageFile.cs
- GridEntryCollection.cs
- MSHTMLHostUtil.cs
- CheckedListBox.cs
- OnOperation.cs
- Resources.Designer.cs
- ObjectViewFactory.cs
- KeyNotFoundException.cs
- SqlCommand.cs
- InstanceCreationEditor.cs
- WindowsRichEditRange.cs
- AsyncPostBackTrigger.cs
- HttpsChannelFactory.cs
- SecurityDocument.cs
- HtmlPhoneCallAdapter.cs
- IISUnsafeMethods.cs
- Matrix3D.cs
- ClientSideQueueItem.cs
- OdbcReferenceCollection.cs
- SecurityManager.cs
- TableRow.cs
- NetworkCredential.cs
- CryptoApi.cs
- TypeSystem.cs
- SHA512.cs
- PriorityRange.cs
- ConnectionStringsExpressionBuilder.cs
- AssemblyInfo.cs
- SqlFacetAttribute.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- RelativeSource.cs
- CodeDomSerializerException.cs
- XmlSchemaNotation.cs
- SrgsElementList.cs
- StylusPointProperties.cs
- FrameworkContentElement.cs
- Model3D.cs
- XmlWriterTraceListener.cs
- ThreadAttributes.cs
- TextRangeBase.cs
- ArgIterator.cs
- XmlSchemaAny.cs
- BooleanProjectedSlot.cs
- SpAudioStreamWrapper.cs
- EventsTab.cs
- StyleHelper.cs
- StaticTextPointer.cs
- OutOfProcStateClientManager.cs
- NullableIntAverageAggregationOperator.cs
- JsonObjectDataContract.cs
- FileChangeNotifier.cs
- HyperLinkStyle.cs
- DataListItemCollection.cs
- WinFormsUtils.cs
- CorePropertiesFilter.cs
- PrintDialog.cs
- DispatchWrapper.cs
- FileRecordSequenceHelper.cs
- PolyBezierSegment.cs
- XsltArgumentList.cs
- _NegotiateClient.cs
- InsufficientExecutionStackException.cs
- ContainerVisual.cs
- hwndwrapper.cs
- XmlReaderSettings.cs
- InputLangChangeRequestEvent.cs
- UseLicense.cs
- AssociationTypeEmitter.cs
- AccessibleObject.cs
- TranslateTransform3D.cs
- XmlSchemaProviderAttribute.cs