Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / LocalTransaction.cs / 1305376 / LocalTransaction.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #region Using directives using System; using System.Diagnostics; using System.Transactions; using System.Data; using System.Data.Common; using System.Threading; #endregion namespace System.Workflow.Runtime.Hosting { ////// This class wraps a local transaction (DbTransaction) by implementing IPromotableSinglePhaseNotification: /// It instantiate a DbTransaction and never allows promotion to a DTC transaction. /// internal sealed class LocalTransaction : IPromotableSinglePhaseNotification { readonly DbTransaction transaction; System.Data.Common.DbConnection connection; ManualResetEvent handle; object syncRoot = new Object(); #region Constructor ////// Wraps a local transaction inside /// /// internal LocalTransaction(DbResourceAllocator dbHelper, ManualResetEvent handle) { if (null == handle) throw new ArgumentNullException("handle"); // Open a connection that specifically does not auto-enlist ("Enlist=false" in connection string) // to prevent auto-promotion of the transaction this.connection = dbHelper.OpenNewConnectionNoEnlist(); this.transaction = this.connection.BeginTransaction(); this.handle = handle; } #endregion Constructor #region Accessors public System.Data.Common.DbConnection Connection { get { return this.connection; } } public DbTransaction Transaction { get { return this.transaction; } } #endregion Accessors #region IPromotableSinglePhaseNotification Members public void Initialize() { } public void SinglePhaseCommit(SinglePhaseEnlistment en) { if (en == null) throw new ArgumentNullException("en"); // // Wait until IPendingWork members have completed (WinOE bugs 17580 and 13395) try { handle.WaitOne(); } catch (ObjectDisposedException) { // If an ObjectDisposedException is thrown because // the WaitHandle has already closed, nothing to worry // about. Move on. } lock (syncRoot) { try { this.transaction.Commit(); en.Committed(); } catch (Exception e) { en.Aborted(e); } finally { if ((null != connection) && (ConnectionState.Closed != connection.State)) { connection.Close(); connection = null; } } } } public void Rollback(SinglePhaseEnlistment en) { if (en == null) throw new ArgumentNullException("en"); // // Wait until IPendingWork members have completed (WinOE bugs 17580 and 13395) try { handle.WaitOne(); } catch (ObjectDisposedException) { // If an ObjectDisposedException is thrown because // the WaitHandle has already closed, nothing to worry // about. Move on. } // DbTransaction.Dispose will call Rollback if the DbTransaction is not Zombied. // Not safe to call DbTransaction.Rollback here as the the underlining // DbTransaction may have already been Rolled back lock (syncRoot) { if (null != transaction) transaction.Dispose(); if ((null != connection) && (ConnectionState.Closed != connection.State)) { connection.Close(); connection = null; } en.Aborted(); } } public byte[] Promote() { throw new TransactionPromotionException( ExecutionStringManager.PromotionNotSupported); } #endregion IPromotableSinglePhaseNotification Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- #region Using directives using System; using System.Diagnostics; using System.Transactions; using System.Data; using System.Data.Common; using System.Threading; #endregion namespace System.Workflow.Runtime.Hosting { ////// This class wraps a local transaction (DbTransaction) by implementing IPromotableSinglePhaseNotification: /// It instantiate a DbTransaction and never allows promotion to a DTC transaction. /// internal sealed class LocalTransaction : IPromotableSinglePhaseNotification { readonly DbTransaction transaction; System.Data.Common.DbConnection connection; ManualResetEvent handle; object syncRoot = new Object(); #region Constructor ////// Wraps a local transaction inside /// /// internal LocalTransaction(DbResourceAllocator dbHelper, ManualResetEvent handle) { if (null == handle) throw new ArgumentNullException("handle"); // Open a connection that specifically does not auto-enlist ("Enlist=false" in connection string) // to prevent auto-promotion of the transaction this.connection = dbHelper.OpenNewConnectionNoEnlist(); this.transaction = this.connection.BeginTransaction(); this.handle = handle; } #endregion Constructor #region Accessors public System.Data.Common.DbConnection Connection { get { return this.connection; } } public DbTransaction Transaction { get { return this.transaction; } } #endregion Accessors #region IPromotableSinglePhaseNotification Members public void Initialize() { } public void SinglePhaseCommit(SinglePhaseEnlistment en) { if (en == null) throw new ArgumentNullException("en"); // // Wait until IPendingWork members have completed (WinOE bugs 17580 and 13395) try { handle.WaitOne(); } catch (ObjectDisposedException) { // If an ObjectDisposedException is thrown because // the WaitHandle has already closed, nothing to worry // about. Move on. } lock (syncRoot) { try { this.transaction.Commit(); en.Committed(); } catch (Exception e) { en.Aborted(e); } finally { if ((null != connection) && (ConnectionState.Closed != connection.State)) { connection.Close(); connection = null; } } } } public void Rollback(SinglePhaseEnlistment en) { if (en == null) throw new ArgumentNullException("en"); // // Wait until IPendingWork members have completed (WinOE bugs 17580 and 13395) try { handle.WaitOne(); } catch (ObjectDisposedException) { // If an ObjectDisposedException is thrown because // the WaitHandle has already closed, nothing to worry // about. Move on. } // DbTransaction.Dispose will call Rollback if the DbTransaction is not Zombied. // Not safe to call DbTransaction.Rollback here as the the underlining // DbTransaction may have already been Rolled back lock (syncRoot) { if (null != transaction) transaction.Dispose(); if ((null != connection) && (ConnectionState.Closed != connection.State)) { connection.Close(); connection = null; } en.Aborted(); } } public byte[] Promote() { throw new TransactionPromotionException( ExecutionStringManager.PromotionNotSupported); } #endregion IPromotableSinglePhaseNotification Members } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
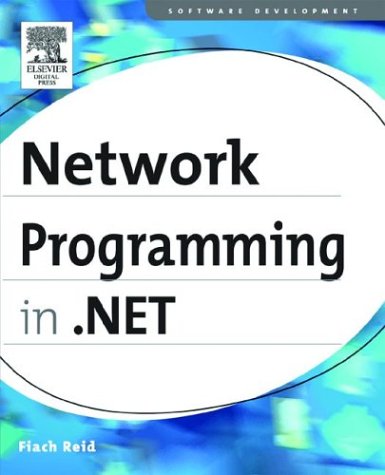
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PrivilegeNotHeldException.cs
- SecUtil.cs
- CodeMethodInvokeExpression.cs
- XmlNotation.cs
- XmlWriterTraceListener.cs
- EnumerationRangeValidationUtil.cs
- BatchServiceHost.cs
- httpserverutility.cs
- UnhandledExceptionEventArgs.cs
- InboundActivityHelper.cs
- Visual3D.cs
- SafeNativeMethods.cs
- OutputCacheSettingsSection.cs
- ClientApiGenerator.cs
- FieldNameLookup.cs
- ImageButton.cs
- DiscoveryInnerClientAdhocCD1.cs
- DbDataReader.cs
- UniformGrid.cs
- ApplicationFileCodeDomTreeGenerator.cs
- ImpersonationContext.cs
- DbProviderFactories.cs
- ExpandoObject.cs
- FormsAuthenticationEventArgs.cs
- ColorPalette.cs
- DbConnectionPoolIdentity.cs
- GridViewRow.cs
- TextTrailingCharacterEllipsis.cs
- PartitionedStreamMerger.cs
- PrivilegedConfigurationManager.cs
- BufferAllocator.cs
- AnonymousIdentificationSection.cs
- Sentence.cs
- KnownBoxes.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- ToolStripDropDownClosingEventArgs.cs
- ParamArrayAttribute.cs
- CodeAttributeDeclarationCollection.cs
- XamlSerializerUtil.cs
- CodeParameterDeclarationExpression.cs
- SqlResolver.cs
- HttpCacheParams.cs
- PasswordBox.cs
- RTLAwareMessageBox.cs
- ResourceDictionaryCollection.cs
- CodeDefaultValueExpression.cs
- FixedBufferAttribute.cs
- FormViewPageEventArgs.cs
- VariableAction.cs
- TextServicesManager.cs
- Events.cs
- TimeIntervalCollection.cs
- AesManaged.cs
- WhitespaceReader.cs
- DocumentViewerHelper.cs
- CompoundFileStreamReference.cs
- ECDiffieHellmanCng.cs
- MessageQueueException.cs
- HttpWriter.cs
- DataListCommandEventArgs.cs
- ServiceNotStartedException.cs
- MemoryRecordBuffer.cs
- Win32PrintDialog.cs
- ClientUrlResolverWrapper.cs
- DesignerEditorPartChrome.cs
- ShaderEffect.cs
- StorageInfo.cs
- ProxyDataContractResolver.cs
- ToolStripLocationCancelEventArgs.cs
- TextEncodedRawTextWriter.cs
- ToolStripOverflowButton.cs
- EntityDataSourceState.cs
- Int64Converter.cs
- BindingValueChangedEventArgs.cs
- TreeNodeCollection.cs
- ToolboxItemAttribute.cs
- DragDropHelper.cs
- AuthenticatedStream.cs
- TimelineGroup.cs
- SplitterCancelEvent.cs
- SafeRightsManagementHandle.cs
- JsonFormatReaderGenerator.cs
- XslCompiledTransform.cs
- TextServicesPropertyRanges.cs
- XmlImplementation.cs
- PolygonHotSpot.cs
- TextServicesContext.cs
- XmlSchemaSimpleTypeList.cs
- Action.cs
- LowerCaseStringConverter.cs
- COM2ColorConverter.cs
- AstTree.cs
- CodeBlockBuilder.cs
- MultipleViewPattern.cs
- StringBuilder.cs
- BooleanExpr.cs
- LongAverageAggregationOperator.cs
- RuleAction.cs
- AccessDataSourceView.cs
- GrammarBuilderRuleRef.cs