Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / CqlBlock.cs / 1305376 / CqlBlock.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.ObjectModel; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that holds an expression of the form SELECT .. FROM .. WHERE // Essentially, it is a structure allows us to generate Cql query in a // localized manner, i.e., all global decisions about nulls, constants, // case statements, etc have already been made internal abstract class CqlBlock : InternalBase { // effects: Initializes a CqlBlock with the SELECT (slotinfos), FROM // (children), WHERE (whereClause), AS (blockAliasNum) protected CqlBlock(SlotInfo[] slotInfos, Listchildren, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) { m_slots = new ReadOnlyCollection (slotInfos); m_children = new ReadOnlyCollection (children); m_whereClause = whereClause; m_blockAlias = identifiers.GetBlockAlias(blockAliasNum); } #region Fields private ReadOnlyCollection m_slots; // essentially, SELECT private ReadOnlyCollection m_children; // FROM private BoolExpression m_whereClause; // WHERE private string m_blockAlias; // AS ... for cql generation #endregion #region Properties // effects: Returns the slot being projected at slotNum. If no slot // is being projected, returns null internal ProjectedSlot ProjectedSlot(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].SlotValue; } // effects: Returns the whereclause of this block (WHERE) protected BoolExpression WhereClause { get { return m_whereClause; } } // effects: Returns all the child blocks of this block (FROM) protected ReadOnlyCollection Children { get { return m_children; } } // effects: Returns all the slots for this block (SELECT) internal ReadOnlyCollection Slots { get { return m_slots; } set { m_slots = value; } } #endregion #region Abstract Methods // effects: Returns a string corresponding to the Cql representation // of this block (and its children below). internal abstract StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel); #endregion #region Methods // effects: Returns a string that has an alias for the FROM part of // this block or the whole block itself that can be used for "AS" internal string CqlAlias { get { return m_blockAlias; } } // effects: Returns true iff slotNum is being projected by this block internal bool IsProjected(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].IsProjected; } protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, bool asForCaseStatementsOnly) { GenerateProjectedtList(builder, indentLevel, blockAlias, CellQuery.SelectDistinct.No, asForCaseStatementsOnly); } // effects: Generates a "SELECT A, B, C, .." for all the slots in this // if asForCaseStatementsOnly is true, generates aliases, ie., foo AS // bar for CaseStatements only. Otherwise, generates them for all slots protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, CellQuery.SelectDistinct selectDistinct, bool asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("SELECT "); if (selectDistinct == CellQuery.SelectDistinct.Yes) { builder.Append("DISTINCT "); } List projectedExpressions = new List (); bool isFirst = true; foreach (SlotInfo slotInfo in Slots) { if (false == slotInfo.IsRequiredByParent) { // Ignore slots that are not needed continue; } if (isFirst == false) { builder.Append(", "); } // Note: We call AsCql and not AliasName on // slotInfo since we do want to pick the right reference to // the slot from the appropriate child if (false == asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel + 1); } slotInfo.AsCql(builder, blockAlias, indentLevel); // Print the field alias for constants (booleans and regular constants). Also // case statements need the alias as well. // We also add it for extent cql blocks for all fields and // finally we need IS NOT NULL for boolean conditions if (slotInfo.SlotValue is CaseStatementProjectedSlot || slotInfo.SlotValue is BooleanProjectedSlot || slotInfo.SlotValue is ConstantProjectedSlot || asForCaseStatementsOnly == false || slotInfo.IsEnforcedNotNull) { builder.Append(" AS ") .Append(slotInfo.CqlFieldAlias); } isFirst = false; } // Get the slots as A, B, C, ... StringUtil.ToSeparatedString(builder, projectedExpressions, ", ", null); StringUtil.IndentNewLine(builder, indentLevel); } internal override void ToCompactString(StringBuilder builder) { for (int i = 0; i < m_slots.Count; i++) { StringUtil.FormatStringBuilder(builder, "{0}: ", i); m_slots[i].ToCompactString(builder); builder.Append(' '); } m_whereClause.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.Structures; using System.Collections.ObjectModel; using System.Text; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A class that holds an expression of the form SELECT .. FROM .. WHERE // Essentially, it is a structure allows us to generate Cql query in a // localized manner, i.e., all global decisions about nulls, constants, // case statements, etc have already been made internal abstract class CqlBlock : InternalBase { // effects: Initializes a CqlBlock with the SELECT (slotinfos), FROM // (children), WHERE (whereClause), AS (blockAliasNum) protected CqlBlock(SlotInfo[] slotInfos, Listchildren, BoolExpression whereClause, CqlIdentifiers identifiers, int blockAliasNum) { m_slots = new ReadOnlyCollection (slotInfos); m_children = new ReadOnlyCollection (children); m_whereClause = whereClause; m_blockAlias = identifiers.GetBlockAlias(blockAliasNum); } #region Fields private ReadOnlyCollection m_slots; // essentially, SELECT private ReadOnlyCollection m_children; // FROM private BoolExpression m_whereClause; // WHERE private string m_blockAlias; // AS ... for cql generation #endregion #region Properties // effects: Returns the slot being projected at slotNum. If no slot // is being projected, returns null internal ProjectedSlot ProjectedSlot(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].SlotValue; } // effects: Returns the whereclause of this block (WHERE) protected BoolExpression WhereClause { get { return m_whereClause; } } // effects: Returns all the child blocks of this block (FROM) protected ReadOnlyCollection Children { get { return m_children; } } // effects: Returns all the slots for this block (SELECT) internal ReadOnlyCollection Slots { get { return m_slots; } set { m_slots = value; } } #endregion #region Abstract Methods // effects: Returns a string corresponding to the Cql representation // of this block (and its children below). internal abstract StringBuilder AsCql(StringBuilder builder, bool isTopLevel, int indentLevel); #endregion #region Methods // effects: Returns a string that has an alias for the FROM part of // this block or the whole block itself that can be used for "AS" internal string CqlAlias { get { return m_blockAlias; } } // effects: Returns true iff slotNum is being projected by this block internal bool IsProjected(int slotNum) { Debug.Assert(slotNum < m_slots.Count, "Slotnum too high"); return m_slots[slotNum].IsProjected; } protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, bool asForCaseStatementsOnly) { GenerateProjectedtList(builder, indentLevel, blockAlias, CellQuery.SelectDistinct.No, asForCaseStatementsOnly); } // effects: Generates a "SELECT A, B, C, .." for all the slots in this // if asForCaseStatementsOnly is true, generates aliases, ie., foo AS // bar for CaseStatements only. Otherwise, generates them for all slots protected void GenerateProjectedtList(StringBuilder builder, int indentLevel, string blockAlias, CellQuery.SelectDistinct selectDistinct, bool asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel); builder.Append("SELECT "); if (selectDistinct == CellQuery.SelectDistinct.Yes) { builder.Append("DISTINCT "); } List projectedExpressions = new List (); bool isFirst = true; foreach (SlotInfo slotInfo in Slots) { if (false == slotInfo.IsRequiredByParent) { // Ignore slots that are not needed continue; } if (isFirst == false) { builder.Append(", "); } // Note: We call AsCql and not AliasName on // slotInfo since we do want to pick the right reference to // the slot from the appropriate child if (false == asForCaseStatementsOnly) { StringUtil.IndentNewLine(builder, indentLevel + 1); } slotInfo.AsCql(builder, blockAlias, indentLevel); // Print the field alias for constants (booleans and regular constants). Also // case statements need the alias as well. // We also add it for extent cql blocks for all fields and // finally we need IS NOT NULL for boolean conditions if (slotInfo.SlotValue is CaseStatementProjectedSlot || slotInfo.SlotValue is BooleanProjectedSlot || slotInfo.SlotValue is ConstantProjectedSlot || asForCaseStatementsOnly == false || slotInfo.IsEnforcedNotNull) { builder.Append(" AS ") .Append(slotInfo.CqlFieldAlias); } isFirst = false; } // Get the slots as A, B, C, ... StringUtil.ToSeparatedString(builder, projectedExpressions, ", ", null); StringUtil.IndentNewLine(builder, indentLevel); } internal override void ToCompactString(StringBuilder builder) { for (int i = 0; i < m_slots.Count; i++) { StringUtil.FormatStringBuilder(builder, "{0}: ", i); m_slots[i].ToCompactString(builder); builder.Append(' '); } m_whereClause.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
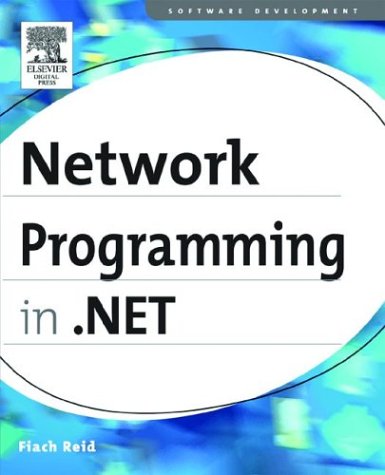
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SendMailErrorEventArgs.cs
- ToolStripButton.cs
- TextWriterTraceListener.cs
- _UriSyntax.cs
- NameTable.cs
- Panel.cs
- PeerNameRegistration.cs
- DetailsViewPagerRow.cs
- TypedElement.cs
- GetImportedCardRequest.cs
- MethodToken.cs
- ValidationSummary.cs
- ExtentJoinTreeNode.cs
- DataView.cs
- SoapExtensionStream.cs
- Win32MouseDevice.cs
- StreamGeometry.cs
- UnaryExpression.cs
- WebPartDisplayModeEventArgs.cs
- PageThemeCodeDomTreeGenerator.cs
- XmlSerializationGeneratedCode.cs
- NamespaceInfo.cs
- OptimisticConcurrencyException.cs
- StorageSetMapping.cs
- Pkcs7Recipient.cs
- PackageStore.cs
- WebCategoryAttribute.cs
- Process.cs
- dbenumerator.cs
- CaseInsensitiveHashCodeProvider.cs
- ProviderConnectionPointCollection.cs
- WasEndpointConfigContainer.cs
- ProcessInfo.cs
- AnimationClockResource.cs
- Freezable.cs
- CssStyleCollection.cs
- FrameworkContentElement.cs
- PrintPreviewDialog.cs
- TextSpanModifier.cs
- Brush.cs
- SerializationAttributes.cs
- ErrorsHelper.cs
- DetailsViewCommandEventArgs.cs
- CheckPair.cs
- ConnectionPoolManager.cs
- IPEndPointCollection.cs
- CmsInterop.cs
- X509ChainPolicy.cs
- ScriptResourceHandler.cs
- SinglePageViewer.cs
- BamlCollectionHolder.cs
- WinEventQueueItem.cs
- DataMemberFieldEditor.cs
- WorkItem.cs
- StringExpressionSet.cs
- IRCollection.cs
- NetworkAddressChange.cs
- StringConcat.cs
- arabicshape.cs
- CngKeyCreationParameters.cs
- PropertyMappingExceptionEventArgs.cs
- TypeDescriptionProvider.cs
- FrugalList.cs
- RawTextInputReport.cs
- DispatchWrapper.cs
- ToolStripLocationCancelEventArgs.cs
- AssociationType.cs
- LexicalChunk.cs
- SendKeys.cs
- SemaphoreSecurity.cs
- JsonXmlDataContract.cs
- TypeExtensionSerializer.cs
- Crc32.cs
- SignatureToken.cs
- WebPartZone.cs
- ButtonPopupAdapter.cs
- MimeFormatter.cs
- GridItemProviderWrapper.cs
- ThumbAutomationPeer.cs
- SessionEndedEventArgs.cs
- UnescapedXmlDiagnosticData.cs
- Menu.cs
- XmlSchemaImporter.cs
- ServiceHostFactory.cs
- input.cs
- RadialGradientBrush.cs
- HostingEnvironmentSection.cs
- FunctionGenerator.cs
- ChangeProcessor.cs
- DeploymentSection.cs
- WorkflowNamespace.cs
- TextFormatter.cs
- DirectoryRedirect.cs
- FontStretchConverter.cs
- BuiltInExpr.cs
- HyperLink.cs
- HttpContextBase.cs
- PrinterUnitConvert.cs
- TemplateAction.cs
- ActivationArguments.cs