Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Caching / MetadataCache.cs / 1305376 / MetadataCache.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to cache metadata information. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Caching { using System; using System.Collections.Generic; using System.Data.EntityClient; using System.Data.Objects; using System.Diagnostics; using System.Threading; ////// Use this class to cache metadata through MetadataCacheItem instances. /// internal static class MetadataCache { ///AppDomain-wide cache for metadata items. private static Dictionarycache = new Dictionary (new MetadataCacheKey.Comparer()); /// Reader/writer lock for AppDomain private static ReaderWriterLockSlim cacheLock = new ReaderWriterLockSlim(LockRecursionPolicy.NoRecursion); ///. Adds a new cache item, and returns the item that is put in the cache. /// Type of service with metadata being cached. /// /// Data context instance being cached, possibly segmenting the cache /// space for. /// /// Item being added. /// The item being put in the cache (possibly an existing one). ///This method is thread-safe but not re-entrant. internal static MetadataCacheItem AddCacheItem(Type serviceType, object dataContextInstance, MetadataCacheItem item) { Debug.Assert(serviceType != null, "serviceType != null"); Debug.Assert(dataContextInstance != null, "dataContextInstance != null"); Debug.Assert(item != null, "item != null"); MetadataCacheKey key = new MetadataCacheKey(serviceType, dataContextInstance as ObjectContext); MetadataCacheItem result; cacheLock.EnterWriteLock(); try { // If another thread beat the current thread, we return the // previously created item, which has a higher chance of // having survived a garbage collection already. if (!cache.TryGetValue(key, out result)) { cache.Add(key, item); result = item; } } finally { cacheLock.ExitWriteLock(); } Debug.Assert(result != null, "result != null -- a null item is never returned."); Debug.Assert( result == TryLookup(serviceType, dataContextInstance), "result == TryLookup(serviceType, dataContextInstance) -- instance from cache is being returned."); return result; } ///Tries to look up metadata for the specifed service type and context instance. /// Type of service with metadata being cached. /// /// Data context instance being cached, possibly segmenting the cache /// space for. /// /// The cached metadata item, if one exists. ///This method is thread-safe but not re-entrant. internal static MetadataCacheItem TryLookup(Type serviceType, object dataContextInstance) { Debug.Assert(serviceType != null, "serviceType != null"); Debug.Assert(dataContextInstance != null, "dataContextInstance != null"); MetadataCacheKey key = new MetadataCacheKey(serviceType, dataContextInstance as ObjectContext); MetadataCacheItem result; cacheLock.EnterReadLock(); try { cache.TryGetValue(key, out result); } finally { cacheLock.ExitReadLock(); } return result; } ///This type is used as the key in the metadata cache. internal struct MetadataCacheKey { ///Connection string used to segment service type. private readonly string dataContextConnection; ///Hash code for this instance. private readonly int hashCode; ///Service type. private readonly Type serviceType; ///Initializes a new MetadataCacheKey instance. /// Service type for key. /// Data context instace for key, possibly null. internal MetadataCacheKey(Type serviceType, ObjectContext dataContextInstance) { Debug.Assert(serviceType != null, "serviceType != null"); this.serviceType = serviceType; this.dataContextConnection = null; this.hashCode = this.serviceType.GetHashCode(); if (dataContextInstance != null) { EntityConnection connection = dataContextInstance.Connection as EntityConnection; if (connection != null) { this.dataContextConnection = new EntityConnectionStringBuilder(connection.ConnectionString).Metadata; this.hashCode ^= this.dataContextConnection.GetHashCode(); } } } ///Comparer for metadata cache keys. internal class Comparer : IEqualityComparer{ /// Compares the specified keys. /// First key. /// Second key. ///true if public bool Equals(MetadataCacheKey x, MetadataCacheKey y) { return x.dataContextConnection == y.dataContextConnection && x.serviceType == y.serviceType; } ///equals , false otherwise. Gets the hash code for the object. /// Object. ///The hash code for this key. public int GetHashCode(MetadataCacheKey obj) { return obj.hashCode; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to cache metadata information. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Caching { using System; using System.Collections.Generic; using System.Data.EntityClient; using System.Data.Objects; using System.Diagnostics; using System.Threading; ////// Use this class to cache metadata through MetadataCacheItem instances. /// internal static class MetadataCache { ///AppDomain-wide cache for metadata items. private static Dictionarycache = new Dictionary (new MetadataCacheKey.Comparer()); /// Reader/writer lock for AppDomain private static ReaderWriterLockSlim cacheLock = new ReaderWriterLockSlim(LockRecursionPolicy.NoRecursion); ///. Adds a new cache item, and returns the item that is put in the cache. /// Type of service with metadata being cached. /// /// Data context instance being cached, possibly segmenting the cache /// space for. /// /// Item being added. /// The item being put in the cache (possibly an existing one). ///This method is thread-safe but not re-entrant. internal static MetadataCacheItem AddCacheItem(Type serviceType, object dataContextInstance, MetadataCacheItem item) { Debug.Assert(serviceType != null, "serviceType != null"); Debug.Assert(dataContextInstance != null, "dataContextInstance != null"); Debug.Assert(item != null, "item != null"); MetadataCacheKey key = new MetadataCacheKey(serviceType, dataContextInstance as ObjectContext); MetadataCacheItem result; cacheLock.EnterWriteLock(); try { // If another thread beat the current thread, we return the // previously created item, which has a higher chance of // having survived a garbage collection already. if (!cache.TryGetValue(key, out result)) { cache.Add(key, item); result = item; } } finally { cacheLock.ExitWriteLock(); } Debug.Assert(result != null, "result != null -- a null item is never returned."); Debug.Assert( result == TryLookup(serviceType, dataContextInstance), "result == TryLookup(serviceType, dataContextInstance) -- instance from cache is being returned."); return result; } ///Tries to look up metadata for the specifed service type and context instance. /// Type of service with metadata being cached. /// /// Data context instance being cached, possibly segmenting the cache /// space for. /// /// The cached metadata item, if one exists. ///This method is thread-safe but not re-entrant. internal static MetadataCacheItem TryLookup(Type serviceType, object dataContextInstance) { Debug.Assert(serviceType != null, "serviceType != null"); Debug.Assert(dataContextInstance != null, "dataContextInstance != null"); MetadataCacheKey key = new MetadataCacheKey(serviceType, dataContextInstance as ObjectContext); MetadataCacheItem result; cacheLock.EnterReadLock(); try { cache.TryGetValue(key, out result); } finally { cacheLock.ExitReadLock(); } return result; } ///This type is used as the key in the metadata cache. internal struct MetadataCacheKey { ///Connection string used to segment service type. private readonly string dataContextConnection; ///Hash code for this instance. private readonly int hashCode; ///Service type. private readonly Type serviceType; ///Initializes a new MetadataCacheKey instance. /// Service type for key. /// Data context instace for key, possibly null. internal MetadataCacheKey(Type serviceType, ObjectContext dataContextInstance) { Debug.Assert(serviceType != null, "serviceType != null"); this.serviceType = serviceType; this.dataContextConnection = null; this.hashCode = this.serviceType.GetHashCode(); if (dataContextInstance != null) { EntityConnection connection = dataContextInstance.Connection as EntityConnection; if (connection != null) { this.dataContextConnection = new EntityConnectionStringBuilder(connection.ConnectionString).Metadata; this.hashCode ^= this.dataContextConnection.GetHashCode(); } } } ///Comparer for metadata cache keys. internal class Comparer : IEqualityComparer{ /// Compares the specified keys. /// First key. /// Second key. ///true if public bool Equals(MetadataCacheKey x, MetadataCacheKey y) { return x.dataContextConnection == y.dataContextConnection && x.serviceType == y.serviceType; } ///equals , false otherwise. Gets the hash code for the object. /// Object. ///The hash code for this key. public int GetHashCode(MetadataCacheKey obj) { return obj.hashCode; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
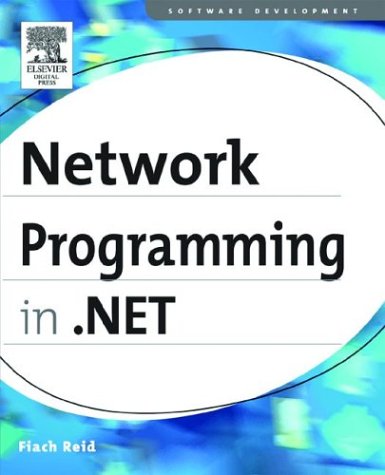
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransformValueSerializer.cs
- ReturnValue.cs
- XmlSchemaSimpleContentExtension.cs
- QuaternionKeyFrameCollection.cs
- FatalException.cs
- TabRenderer.cs
- SafeLibraryHandle.cs
- SharedPersonalizationStateInfo.cs
- RectAnimationClockResource.cs
- SimpleHandlerFactory.cs
- HttpWrapper.cs
- Visual.cs
- KerberosSecurityTokenAuthenticator.cs
- TableHeaderCell.cs
- StrokeNodeOperations.cs
- IPAddressCollection.cs
- DataTableExtensions.cs
- ToolStripItemTextRenderEventArgs.cs
- XamlToRtfParser.cs
- PaintValueEventArgs.cs
- WarningException.cs
- PasswordBox.cs
- NavigationWindowAutomationPeer.cs
- ColorContextHelper.cs
- PreviewPageInfo.cs
- Version.cs
- SaveWorkflowCommand.cs
- TextEndOfSegment.cs
- CommentAction.cs
- VisualStyleInformation.cs
- Publisher.cs
- CodeExporter.cs
- DynamicPropertyHolder.cs
- PanelStyle.cs
- BuildManagerHost.cs
- CqlErrorHelper.cs
- XmlException.cs
- PenThreadPool.cs
- LogRestartAreaEnumerator.cs
- XsdSchemaFileEditor.cs
- SmiSettersStream.cs
- SqlException.cs
- Stroke.cs
- IisTraceWebEventProvider.cs
- ToolboxCategory.cs
- StringCollection.cs
- designeractionlistschangedeventargs.cs
- DesignTimeVisibleAttribute.cs
- ContentType.cs
- GenericAuthenticationEventArgs.cs
- PointCollection.cs
- ComponentEditorPage.cs
- InputLanguageProfileNotifySink.cs
- ProtectedConfigurationProviderCollection.cs
- TableLayoutRowStyleCollection.cs
- CachedPathData.cs
- ColorContext.cs
- DocumentXmlWriter.cs
- ProfileModule.cs
- DataControlField.cs
- DocumentReference.cs
- ListViewCancelEventArgs.cs
- BuildProvider.cs
- XpsResourceDictionary.cs
- ActivityBindForm.Designer.cs
- TranslateTransform3D.cs
- BypassElement.cs
- ManipulationDevice.cs
- Typeface.cs
- RNGCryptoServiceProvider.cs
- FtpRequestCacheValidator.cs
- ClickablePoint.cs
- PeerApplicationLaunchInfo.cs
- NewArrayExpression.cs
- HotCommands.cs
- MatrixAnimationUsingPath.cs
- StylusPlugin.cs
- ScriptReferenceEventArgs.cs
- XmlReader.cs
- SpecialFolderEnumConverter.cs
- PreProcessInputEventArgs.cs
- TableLayoutPanel.cs
- SqlMethodTransformer.cs
- FlowLayoutSettings.cs
- MetafileHeaderEmf.cs
- AssemblyNameProxy.cs
- DependencyObjectCodeDomSerializer.cs
- XsltQilFactory.cs
- ToolStripContentPanel.cs
- EnumerationRangeValidationUtil.cs
- MaskedTextBox.cs
- GeneralTransform.cs
- WSFederationHttpBindingElement.cs
- XmlElement.cs
- PropertyMap.cs
- DependencyPropertyHelper.cs
- ItemAutomationPeer.cs
- ListBindingHelper.cs
- TrustLevelCollection.cs
- DataGridViewElement.cs