Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / DatagridviewDisplayedBandsData.cs / 1305376 / DatagridviewDisplayedBandsData.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { public partial class DataGridView { internal class DisplayedBandsData { private bool dirty; private int firstDisplayedFrozenRow; private int firstDisplayedFrozenCol; private int numDisplayedFrozenRows; private int numDisplayedFrozenCols; private int numTotallyDisplayedFrozenRows; private int firstDisplayedScrollingRow; private int numDisplayedScrollingRows; private int numTotallyDisplayedScrollingRows; private int firstDisplayedScrollingCol; private int numDisplayedScrollingCols; private int lastTotallyDisplayedScrollingCol; private int lastDisplayedScrollingRow; private int lastDisplayedFrozenCol; private int lastDisplayedFrozenRow; private int oldFirstDisplayedScrollingRow; private int oldFirstDisplayedScrollingCol; private int oldNumDisplayedFrozenRows; private int oldNumDisplayedScrollingRows; private bool rowInsertionOccurred, columnInsertionOccurred; public DisplayedBandsData() { this.firstDisplayedFrozenRow = -1; this.firstDisplayedFrozenCol = -1; this.firstDisplayedScrollingRow = -1; this.firstDisplayedScrollingCol = -1; this.lastTotallyDisplayedScrollingCol = -1; this.lastDisplayedScrollingRow = -1; this.lastDisplayedFrozenCol = -1; this.lastDisplayedFrozenRow = -1; this.oldFirstDisplayedScrollingRow = -1; this.oldFirstDisplayedScrollingCol = -1; } public bool ColumnInsertionOccurred { get { return this.columnInsertionOccurred; } } public bool Dirty { get { return this.dirty; } set { this.dirty = value; } } public int FirstDisplayedFrozenCol { set { if (value != this.firstDisplayedFrozenCol) { EnsureDirtyState(); this.firstDisplayedFrozenCol = value; } } } public int FirstDisplayedFrozenRow { set { if (value != this.firstDisplayedFrozenRow) { EnsureDirtyState(); this.firstDisplayedFrozenRow = value; } } } public int FirstDisplayedScrollingCol { get { return this.firstDisplayedScrollingCol; } set { if (value != this.firstDisplayedScrollingCol) { EnsureDirtyState(); this.firstDisplayedScrollingCol = value; } } } public int FirstDisplayedScrollingRow { get { return this.firstDisplayedScrollingRow; } set { if (value != this.firstDisplayedScrollingRow) { EnsureDirtyState(); this.firstDisplayedScrollingRow = value; } } } public int LastDisplayedFrozenCol { set { if (value != this.lastDisplayedFrozenCol) { EnsureDirtyState(); this.lastDisplayedFrozenCol = value; } } } public int LastDisplayedFrozenRow { set { if (value != this.lastDisplayedFrozenRow) { EnsureDirtyState(); this.lastDisplayedFrozenRow = value; } } } public int LastDisplayedScrollingRow { set { if (value != this.lastDisplayedScrollingRow) { EnsureDirtyState(); this.lastDisplayedScrollingRow = value; } } } public int LastTotallyDisplayedScrollingCol { get { return this.lastTotallyDisplayedScrollingCol; } set { if (value != this.lastTotallyDisplayedScrollingCol) { EnsureDirtyState(); this.lastTotallyDisplayedScrollingCol = value; } } } public int NumDisplayedFrozenCols { get { return this.numDisplayedFrozenCols; } set { if (value != this.numDisplayedFrozenCols) { EnsureDirtyState(); this.numDisplayedFrozenCols = value; } } } public int NumDisplayedFrozenRows { get { return this.numDisplayedFrozenRows; } set { if (value != this.numDisplayedFrozenRows) { EnsureDirtyState(); this.numDisplayedFrozenRows = value; } } } public int NumDisplayedScrollingRows { get { return this.numDisplayedScrollingRows; } set { if (value != this.numDisplayedScrollingRows) { EnsureDirtyState(); this.numDisplayedScrollingRows = value; } } } public int NumDisplayedScrollingCols { get { return this.numDisplayedScrollingCols; } set { if (value != this.numDisplayedScrollingCols) { EnsureDirtyState(); this.numDisplayedScrollingCols = value; } } } public int NumTotallyDisplayedFrozenRows { get { return this.numTotallyDisplayedFrozenRows; } set { if (value != this.numTotallyDisplayedFrozenRows) { EnsureDirtyState(); this.numTotallyDisplayedFrozenRows = value; } } } public int NumTotallyDisplayedScrollingRows { get { return this.numTotallyDisplayedScrollingRows; } set { if (value != this.numTotallyDisplayedScrollingRows) { EnsureDirtyState(); this.numTotallyDisplayedScrollingRows = value; } } } public int OldFirstDisplayedScrollingCol { get { return this.oldFirstDisplayedScrollingCol; } } public int OldFirstDisplayedScrollingRow { get { return this.oldFirstDisplayedScrollingRow; } } public int OldNumDisplayedFrozenRows { get { return this.oldNumDisplayedFrozenRows; } } public int OldNumDisplayedScrollingRows { get { return this.oldNumDisplayedScrollingRows; } } public bool RowInsertionOccurred { get { return this.rowInsertionOccurred; } } public void EnsureDirtyState() { if (!this.dirty) { this.dirty = true; this.rowInsertionOccurred = false; this.columnInsertionOccurred = false; SetOldValues(); } } public void CorrectColumnIndexAfterInsertion(int columnIndex, int insertionCount) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingCol != -1 && columnIndex <= this.oldFirstDisplayedScrollingCol) { this.oldFirstDisplayedScrollingCol += insertionCount; } this.columnInsertionOccurred = true; } public void CorrectRowIndexAfterDeletion(int rowIndex) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingRow != -1 && rowIndex <= this.oldFirstDisplayedScrollingRow) { this.oldFirstDisplayedScrollingRow--; } } public void CorrectRowIndexAfterInsertion(int rowIndex, int insertionCount) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingRow != -1 && rowIndex <= this.oldFirstDisplayedScrollingRow) { this.oldFirstDisplayedScrollingRow += insertionCount; } this.rowInsertionOccurred = true; this.oldNumDisplayedScrollingRows += insertionCount; this.oldNumDisplayedFrozenRows += insertionCount; } private void SetOldValues() { this.oldFirstDisplayedScrollingRow = this.firstDisplayedScrollingRow; this.oldFirstDisplayedScrollingCol = this.firstDisplayedScrollingCol; this.oldNumDisplayedFrozenRows = this.numDisplayedFrozenRows; this.oldNumDisplayedScrollingRows = this.numDisplayedScrollingRows; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { public partial class DataGridView { internal class DisplayedBandsData { private bool dirty; private int firstDisplayedFrozenRow; private int firstDisplayedFrozenCol; private int numDisplayedFrozenRows; private int numDisplayedFrozenCols; private int numTotallyDisplayedFrozenRows; private int firstDisplayedScrollingRow; private int numDisplayedScrollingRows; private int numTotallyDisplayedScrollingRows; private int firstDisplayedScrollingCol; private int numDisplayedScrollingCols; private int lastTotallyDisplayedScrollingCol; private int lastDisplayedScrollingRow; private int lastDisplayedFrozenCol; private int lastDisplayedFrozenRow; private int oldFirstDisplayedScrollingRow; private int oldFirstDisplayedScrollingCol; private int oldNumDisplayedFrozenRows; private int oldNumDisplayedScrollingRows; private bool rowInsertionOccurred, columnInsertionOccurred; public DisplayedBandsData() { this.firstDisplayedFrozenRow = -1; this.firstDisplayedFrozenCol = -1; this.firstDisplayedScrollingRow = -1; this.firstDisplayedScrollingCol = -1; this.lastTotallyDisplayedScrollingCol = -1; this.lastDisplayedScrollingRow = -1; this.lastDisplayedFrozenCol = -1; this.lastDisplayedFrozenRow = -1; this.oldFirstDisplayedScrollingRow = -1; this.oldFirstDisplayedScrollingCol = -1; } public bool ColumnInsertionOccurred { get { return this.columnInsertionOccurred; } } public bool Dirty { get { return this.dirty; } set { this.dirty = value; } } public int FirstDisplayedFrozenCol { set { if (value != this.firstDisplayedFrozenCol) { EnsureDirtyState(); this.firstDisplayedFrozenCol = value; } } } public int FirstDisplayedFrozenRow { set { if (value != this.firstDisplayedFrozenRow) { EnsureDirtyState(); this.firstDisplayedFrozenRow = value; } } } public int FirstDisplayedScrollingCol { get { return this.firstDisplayedScrollingCol; } set { if (value != this.firstDisplayedScrollingCol) { EnsureDirtyState(); this.firstDisplayedScrollingCol = value; } } } public int FirstDisplayedScrollingRow { get { return this.firstDisplayedScrollingRow; } set { if (value != this.firstDisplayedScrollingRow) { EnsureDirtyState(); this.firstDisplayedScrollingRow = value; } } } public int LastDisplayedFrozenCol { set { if (value != this.lastDisplayedFrozenCol) { EnsureDirtyState(); this.lastDisplayedFrozenCol = value; } } } public int LastDisplayedFrozenRow { set { if (value != this.lastDisplayedFrozenRow) { EnsureDirtyState(); this.lastDisplayedFrozenRow = value; } } } public int LastDisplayedScrollingRow { set { if (value != this.lastDisplayedScrollingRow) { EnsureDirtyState(); this.lastDisplayedScrollingRow = value; } } } public int LastTotallyDisplayedScrollingCol { get { return this.lastTotallyDisplayedScrollingCol; } set { if (value != this.lastTotallyDisplayedScrollingCol) { EnsureDirtyState(); this.lastTotallyDisplayedScrollingCol = value; } } } public int NumDisplayedFrozenCols { get { return this.numDisplayedFrozenCols; } set { if (value != this.numDisplayedFrozenCols) { EnsureDirtyState(); this.numDisplayedFrozenCols = value; } } } public int NumDisplayedFrozenRows { get { return this.numDisplayedFrozenRows; } set { if (value != this.numDisplayedFrozenRows) { EnsureDirtyState(); this.numDisplayedFrozenRows = value; } } } public int NumDisplayedScrollingRows { get { return this.numDisplayedScrollingRows; } set { if (value != this.numDisplayedScrollingRows) { EnsureDirtyState(); this.numDisplayedScrollingRows = value; } } } public int NumDisplayedScrollingCols { get { return this.numDisplayedScrollingCols; } set { if (value != this.numDisplayedScrollingCols) { EnsureDirtyState(); this.numDisplayedScrollingCols = value; } } } public int NumTotallyDisplayedFrozenRows { get { return this.numTotallyDisplayedFrozenRows; } set { if (value != this.numTotallyDisplayedFrozenRows) { EnsureDirtyState(); this.numTotallyDisplayedFrozenRows = value; } } } public int NumTotallyDisplayedScrollingRows { get { return this.numTotallyDisplayedScrollingRows; } set { if (value != this.numTotallyDisplayedScrollingRows) { EnsureDirtyState(); this.numTotallyDisplayedScrollingRows = value; } } } public int OldFirstDisplayedScrollingCol { get { return this.oldFirstDisplayedScrollingCol; } } public int OldFirstDisplayedScrollingRow { get { return this.oldFirstDisplayedScrollingRow; } } public int OldNumDisplayedFrozenRows { get { return this.oldNumDisplayedFrozenRows; } } public int OldNumDisplayedScrollingRows { get { return this.oldNumDisplayedScrollingRows; } } public bool RowInsertionOccurred { get { return this.rowInsertionOccurred; } } public void EnsureDirtyState() { if (!this.dirty) { this.dirty = true; this.rowInsertionOccurred = false; this.columnInsertionOccurred = false; SetOldValues(); } } public void CorrectColumnIndexAfterInsertion(int columnIndex, int insertionCount) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingCol != -1 && columnIndex <= this.oldFirstDisplayedScrollingCol) { this.oldFirstDisplayedScrollingCol += insertionCount; } this.columnInsertionOccurred = true; } public void CorrectRowIndexAfterDeletion(int rowIndex) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingRow != -1 && rowIndex <= this.oldFirstDisplayedScrollingRow) { this.oldFirstDisplayedScrollingRow--; } } public void CorrectRowIndexAfterInsertion(int rowIndex, int insertionCount) { EnsureDirtyState(); if (this.oldFirstDisplayedScrollingRow != -1 && rowIndex <= this.oldFirstDisplayedScrollingRow) { this.oldFirstDisplayedScrollingRow += insertionCount; } this.rowInsertionOccurred = true; this.oldNumDisplayedScrollingRows += insertionCount; this.oldNumDisplayedFrozenRows += insertionCount; } private void SetOldValues() { this.oldFirstDisplayedScrollingRow = this.firstDisplayedScrollingRow; this.oldFirstDisplayedScrollingCol = this.firstDisplayedScrollingCol; this.oldNumDisplayedFrozenRows = this.numDisplayedFrozenRows; this.oldNumDisplayedScrollingRows = this.numDisplayedScrollingRows; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
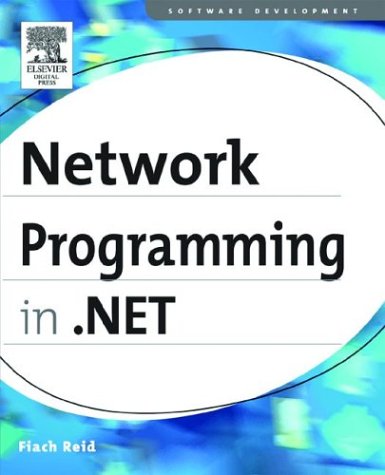
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientConfigurationSystem.cs
- MemoryFailPoint.cs
- DynamicResourceExtensionConverter.cs
- MsdtcWrapper.cs
- LogPolicy.cs
- EdmSchemaAttribute.cs
- ParameterBuilder.cs
- IResourceProvider.cs
- FormParameter.cs
- ThreadExceptionDialog.cs
- ExpressionNode.cs
- DirectionalLight.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- XamlToRtfWriter.cs
- HttpProfileBase.cs
- WebPartVerb.cs
- DiffuseMaterial.cs
- ApplicationHost.cs
- AppDomainUnloadedException.cs
- DataTableTypeConverter.cs
- DetailsViewRow.cs
- IOThreadTimer.cs
- _AutoWebProxyScriptEngine.cs
- DataBinder.cs
- WpfMemberInvoker.cs
- TreeNodeBindingCollection.cs
- TableAdapterManagerHelper.cs
- CodeAssignStatement.cs
- PrimitiveSchema.cs
- UpdateCompiler.cs
- MimeObjectFactory.cs
- ContentPlaceHolder.cs
- FontFamilyConverter.cs
- PartBasedPackageProperties.cs
- Button.cs
- LineBreakRecord.cs
- WebPartZoneDesigner.cs
- MediaEntryAttribute.cs
- ReadOnlyMetadataCollection.cs
- TableRowGroupCollection.cs
- NavigationHelper.cs
- StrongNameUtility.cs
- httpstaticobjectscollection.cs
- DeploymentSectionCache.cs
- MetabaseReader.cs
- ItemChangedEventArgs.cs
- DataGridRowEventArgs.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- TextElement.cs
- BitmapEffectDrawing.cs
- ObjectReferenceStack.cs
- MetabaseServerConfig.cs
- KerberosSecurityTokenParameters.cs
- ValidationEventArgs.cs
- TdsParserSafeHandles.cs
- SymmetricAlgorithm.cs
- ClientOptions.cs
- Stack.cs
- PrePostDescendentsWalker.cs
- DiscreteKeyFrames.cs
- SafeNativeMethods.cs
- OdbcConnectionPoolProviderInfo.cs
- ObjectListCommandsPage.cs
- TextAdaptor.cs
- WebMethodAttribute.cs
- Message.cs
- UIInitializationException.cs
- DataFieldCollectionEditor.cs
- BaseTemplateBuildProvider.cs
- BaseDataListActionList.cs
- SizeChangedEventArgs.cs
- ExpandCollapsePattern.cs
- WmlPanelAdapter.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- TextLineResult.cs
- SafeNativeMethodsOther.cs
- MenuTracker.cs
- DesignColumn.cs
- ErrorHandler.cs
- EncryptedData.cs
- DBConcurrencyException.cs
- XPathNode.cs
- XmlSchemaParticle.cs
- HScrollProperties.cs
- SkipQueryOptionExpression.cs
- DetailsViewUpdateEventArgs.cs
- RegexCompilationInfo.cs
- XmlQueryStaticData.cs
- FormsAuthenticationEventArgs.cs
- HtmlElement.cs
- DiagnosticTraceSource.cs
- NavigationPropertySingletonExpression.cs
- ProfileSettings.cs
- PartitionedStream.cs
- AppDomainProtocolHandler.cs
- DecoderNLS.cs
- SchemaManager.cs
- SecUtil.cs
- Span.cs
- EntityModelBuildProvider.cs